34 Remove Property From Object Javascript
The JavaScript delete operator removes a property from an object; if no more references to the same property are held, it is eventually released automatically. Using delete operator to remove an property delete operator is used to remove key from an object, and its key and value removed from an object.
How To Remove A Key From An Object In Javascript By Dr
This tutorial discusses two ways of removing a property from an object. The first way is using the delete operator, and the second way is object destructuring which is useful to remove multiple object properties through a single line of code.. Method 1 - Using delete Operator. The delete operator removes the given property from the object. This operation is mutable, and the value of the ...
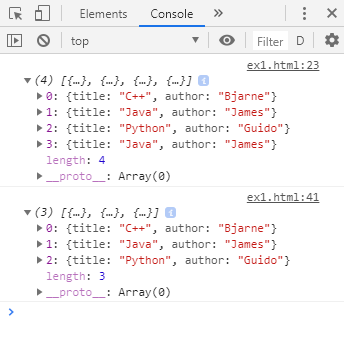
Remove property from object javascript. In this short post, we are going to discuss how to remove property from an array of objects in JavaScript. Removing fields from an array of objects is necessary for sensitive data like password, credit card numbers etc. Let's take this array as an example. From this array, I would like to remove the address field alone. // for enumerable and non-enumerable properties Object.getOwnPropertyNames(obj).forEach(function (prop) { delete obj[prop]; }); ES6. And ES6 solution can be: // for enumerable and non-enumerable properties for (const prop of Object.getOwnPropertyNames(obj)) { delete obj[prop]; } Performance JavaScript object operations - How to add / remove item from a JavaScript object Getting into JavaScript frameworks like vue.js and react.js, now it's time to review some of the JavaScript fundamentals.
Javascript delete is an inbuilt operator that removes the property from the object. The delete operator removes both the value of the property and the property itself. If there are no more references to the same property, then it is eventually released automatically. The delete operator also has the return value. Before destructuring, we would typically use the delete keyword to remove properties from an object. The issue with delete is that it's a mutable operation, physically changing the object and potentially causing unwanted side-effects due to the way JavaScript handles objects references. The delete operator in JavaScript has a different function to that of the keyword in C and C++ − It does not directly free memory. Instead, its sole purpose is to remove properties from objects.
To delete a property of an object, delete key word should be used.Delete key word can be used with both the methods such as Dot method and Bracket method.. syntax delete object.property; Example. In the following example initially when the property "country" is executed its value "England" is displayed in the output.But when that property is deleted using delete keyword,instead of "England ... # Deleting an Object Property Using the delete operator, we can remove a property from an object. Although this has great browser support, beware that this would modify the existing object (i.e. it is mutable). It returns true if the property is successfully deleted, and false if the property can't be deleted. In an earlier article, we looked at how to add a property to an object in JavaScript. But what if you want to remove a specific property from an object? JavaScript provides the delete operator to remove a property from an object. On successful deletion, it will return true, otherwise false:
Use the delete Operator to Remove a Property From an Object in JavaScript One method to remove any of the properties of any object is the delete operator. This operator removes the property from the object. For example, we have an object myObject with the properties as id, subject, and grade, and we need to remove the grade property. Delete operator is designed to used on object properties. It can not be used on variables or functions. Delete operator should not be used on predefined JavaScript object properties. It can cause problems. Example 1: This example deletes the address property of an object. <!DOCTYPE html>. Answer: Use the delete Operator You can use the delete operator to completely remove the properties from the JavaScript object. Deleting is the only way to actually remove a property from an object. Setting the property to undefined or null only changes the value of the property.
How to remove a property from a JavaScript object There are various ways to remove a property from a JavaScript object. Find out the alternatives and the suggested solution. Published May 22, 2018. The semantically correct way to remove a property from an object is to use the delete keyword. Objects in JavaScript can be thought of as maps between keys and values. The deleteoperator is used to remove these keys, more commonly known as object properties, one at a time. var obj = { myProperty: 1 I needed to remove a particular property from a list (just a plain object) stored in state. I have the property id, so shouldn't be too difficult. As we're working in a reducer, we need to be wary of state mutation and side effects of our functions. i.e. we want to return the new and updated state without mutating the original state.
The delete operator is technically the correct way to remove a property from a JavaScript object. Unlike the previous option, delete will completely remove the property from the object, but it will still cause a mutation. const pet = { species: 'dog', age: 3, name: 'celeste', gender: 'female' }; delete pet.gender; Object.keys( pet); This post will discuss how to remove a property from an object in JavaScript. 1. Using Delete Operator. The delete operator removes a given property from an object, returning true or false as appropriate on success and failure. In JavaScript, there are 2 common ways to remove properties from an object. The first mutable approach is to use the delete object.property operator. The second approach, which is immutable since it doesn't modify the original object, is to invoke the object destructuring and spread syntax: const {property,...rest} = object.
JavaScript offers various methods to remove the property from the object either by using built-in methods or by implementing our own logic. In this lesson, we are going to discuss different methods to remove the property from the object. Using delete operator. We can remove the property from the JavaScript object by deleting it. 22/8/2021 · This is the oldest and most used way to delete property from an object in javascript. You can simply use the delete operator to remove property from an object. If you want to delete multiple properties, you have to use the delete operator multiple times in the same function. const obj = { 'first': 'one', 'second': 'two', 'third': 'three' } delete obj.first; // OR delete obj['first']; // obj = { 'second': 'two', … How TO - Remove a Property from an Object Previous Next Learn how to remove a property from a JavaScript object. Remove Property from an Object. The delete operator deletes a property from an object: Example. var person = { firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"
In JavaScript, we can use the 'delete' operator to completely remove the properties from the JavaScript object. Deleting is the way to remove a property from an object. When you remove a property from an object property set to undefined or null. Delete operator not work when you used on predefined JavaScript object properties. JavaScript: Remove a Property From an Object. Abhilash Kakumanu. Introduction. An object in JavaScript is a collection of key-value pairs. One of these key-value pairs is called an object property. Both keys and values of properties can be of any data type - Number, String, Array, Object, etc. In the above program, the delete operator is used to remove a property from an object. You can use the delete operator with. or [ ] to remove the property from an object. Note: You should not use the delete operator on predefined JavaScript object properties.
How TO - Remove a Property from an Object Previous Next Learn how to remove a property from a JavaScript object. Remove Property from an Object. The delete operator deletes a property from an object: Example. var person = { firstName:"John", lastName:"Doe", age:50, eyeColor:"blue" Deleting a property of an object with ES5 JavaScript The delete functionality of properties is also given in ES5 JavaScript. The keyword to use is delete and can be used similar to what the adding of a property looks like. 1 To remove a property from an object, we can use the the delete keyword in JavaScript. Here is an example, that removes the property b from the following object: const obj ={ a:1, b:2, c:3 } delete obj.b. Output: const obj ={ a:1, c:3 } The delete keyword deletes the object property and its value. Share:
Remove A Property Of An Object Immutably In Redux Javascript
Use Strict In Javascript If You Are New To Js Use Strict
Remove Item From Object Javascript Code Example
Remove A Property From An Object In Javascript With This
How Do I Remove A Property From A Javascript Object Stack
How To Find Unique Values By Property In An Array Of Objects
Javascript Delete Operator How To Remove Property Of Object
Add Or Delete A Property Of An Object In Javascript
How To Remove Duplicates From An Array Of Objects Using
How To Remove A Property From Javascript Object Geeksforgeeks
Javascript Delete How To Remove Property Of Object In Js
How To Create Modify And Loop Through Objects In Javascript
Deep Notes Javascript Javascript Object Oriented And
Removing Items From An Array In Javascript Ultimate Courses
How Can I Remove Properties From Javascript Objects O Reilly
Details Of The Object Model Javascript Mdn
How To Remove Last Property In An Object In Es6 Code Example
Javascript Dom Document Object Model Guide For Novice
How To Remove A Key From An Object In Javascript By Dr
Javascript Full Stack Javascriptfullstack Profile Pinterest
Remove Property From Object Javascript Es6 Code Example
Lodash Remove Throws Object Doesn T Support Property Or
How To Remove An Object That Matches A Filter From A
How To Remove Css Property Using Javascript Geeksforgeeks
Javascript Object Rename Key Stack Overflow
Remove Object From An Array Of Objects In Javascript
How To Add Remove Property From A Json Object Dynamically
How To Remove A Property From An Object In Javascript
3 Ways To Shallow Clone Objects In Javascript W Bonuses
Remove Item From An Array Of Objects By Obj Property Renat
0 Response to "34 Remove Property From Object Javascript"
Post a Comment