32 Javascript When To Use Var
JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ... Mar 02, 2020 - When we declare a new variable in JavaScript, we can either use const, let, or var. Each of these is used for something different and some might be needed in specific situations depending on what you’re working with. Today, we will take a look at the main differences between const, let, and ...
Javascript For Loop By Examples
1 week ago - Get access to ad-free content, doubt assistance and more! ... Understanding basic JavaScript codes. ... var and let are both used for variable declaration in javascript but the difference between them is that var is function scoped and let is block scoped. It can be said that a variable declared ...
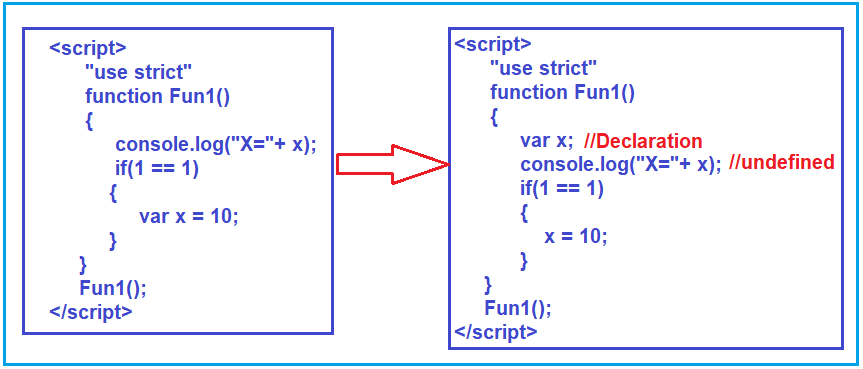
Javascript when to use var. var is the keyword that tells JavaScript you're declaring a variable. x is the name of that variable. = is the operator that tells JavaScript a value is coming up next. 100 is the value for the variable to store. Using variables. After you declare a variable, you can reference it by name elsewhere in your code. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...) The code to be executed, by the function ... Before ES6, the var keyword was used to declare a variable in JavaScript. The var keyword has been around since the inception of JavaScript, and it's what you will see in any pre ES6 code. Variables declared using the var keyword are either globally or functionally scoped, they do not support block-level scope.
A JavaScript Function is a JavaScript Variable until it is executed (evaluated). Read on for a full explanation. ... Having declared a function just makes it available for use in your program. It ... 38 answersScoping rules. The main difference is scoping rules. Variables declared by var keyword are scoped to the immediate function body (hence the function scope) ... Creating JavaScript Variables. Any of the following are valid variable declarations: var car; var house; var lawnmower; var tea; var year; The keyword var is part of the JavaScript language and is used to declare a variable. We simply use the keyword var followed by the name we want to use to identify our spot in memory that will hold our data. We are free to name our variables anything we ...
Using var lets you hide external variables that have the same name. In this way you can simulate a "private" variable, but that's another topic. A rule of thumb is to always use var, because otherwise you run the risk of introducing subtle bugs. EDIT: After the critiques I received, I would like to emphasize the following: Note: In JavaScript, var is function scoped and let is block-scoped. If you try to use var c = 'hello'; inside the if statement in the above program, the whole program works, as c is treated as a local variable. To learn more about let versus var, visit JavaScript let vs var. Variables are used to store values (name = "John") or expressions (sum = x + y). Declare Variables in JavaScript. Before using a variable, you first need to declare it. You have to use the keyword var to declare a variable like this: var name; Assign a Value to the Variable
For a start, if you write a multiline JavaScript program that declares and initializes a variable, you can actually declare a variable with var after you initialize it and it will still work. Nov 17, 2016 - I’ve been writing JavaScript using the ES2015 (ES6) syntax for awhile now and have grown to appreciate many of the language changes for their elegance and simplicity. One of the first and easiest changes I adapted to was using let/const instead of var. However, I took for granted the benefit ... Nov 28, 2017 - Now, if you, like me, want to stick with using semicolons in your JavaScript, here is how to know where they should go: Semicolons are needed at the end of JavaScript 'statements' Lots of things qualify as a statement in Javascript. Here are some examples: // variable declaration (assigning ...
Nov 12, 2011 - Maybe pretty easy question. Where should I use var keyword in JavaScript. It seems to me using it or not have the same effect ( but of course I'm still learning the language ) For instance thes... 1 week ago - `var` is now the weakest signal available when you define a variable in JavaScript. The variable may or may not be reassigned, and the variable may or may not be used for an entire function, or just for the purpose of a block or loop. ... With `let` and `const` in ES6, it’s no longer safe to ... Feb 10, 2020 - We all start somewhere. I learned JavaScript declaring variables with the var keyword. It was simple and it worked, but I’ve changed. If you write code like var x = 5 you need to stop. Well, I’ll be…
In the above code, we have passed the regex pattern as an argument to the replace() method instead of that we can store the regex pattern in a variable and pass it to the replace method.. Note: Regex can be created in two ways first one is regex literal and the second one is regex constructor method (new RegExp()).If we try to pass a variable to the regex literal pattern it won't work. The var statement declares a variable. Variables are containers for storing information. Creating a variable in JavaScript is called "declaring" a variable: var carName; After the declaration, the variable is empty (it has no value). To assign a value to the variable, use the equal sign: carName = "Volvo" ; You can also assign a value to the ... Apr 14, 2020 - Are You Read for the Great JavaScript Battle of var vs let vs const? Variables declared using var can be done anywhere in a function. But instead of being limited to block, like a for loop, in the function the variables are hoisted to the top of the function. This is why the best practice is to declare all the variables used ...
The "use strict" Directive. The "use strict" directive was new in ECMAScript version 5. It is not a statement, but a literal expression, ignored by earlier versions of JavaScript. The purpose of "use strict" is to indicate that the code should be executed in "strict mode". With strict mode, you can not, for example, use undeclared variables. JavaScript variables are boxes for storing values. We must declare a variable in the JavaScript program before using it. A variable can be a number, a single character, string, or value indicating whether something true or false. Variable allows us to store, retrieve and change the stored information. Use Basic Formatting to Insert Variable Into String in JavaScript Another way of neatly inserting the variable values into our string is by using the basic formatting supported in JavaScript. Using the Javascript console.log (), we can avoid concatenation and add placeholders in the targetted string.
Nov 26, 2017 - Quality Weekly Reads About Technology Infiltrating Everything Feb 28, 2019 - Javascript variables; should you use let, var or const? ... Ever wondered when to use either of the three? Why were the other two introduced into javascript? Lets try get some answers Jan 01, 2019 - The next difference has to do with Hoisting. Earlier we said that the definition of hoisting was “The JavaScript interpreter will assign variable declarations a default value of undefined during what’s called the ‘Creation’ phase.” We even saw this in action by logging a variable ...
JavaScript variables are loosely typed, that is to say, variables can hold values with any type of data. Variables are just named placeholders for values. Declare JavaScript variables using var keyword To declare a variable, you use the var keyword followed by the variable name as follows: var declarations are processed when the function starts (or script starts for globals). In other words, var variables are defined from the beginning of the function, no matter where the definition is (assuming that the definition is not in the nested function). In programming, just like in algebra, we use variables (like price1) to hold values. In programming, just like in algebra, we use variables in expressions (total = price1 + price2). From the example above, you can calculate the total to be 11. JavaScript variables are containers for storing data values.
When to use const keyword over var in JavaScript How to convert a number to a string in JavaScript Clearing the input field on focus in JavaScript How to remove duplicate elements from array JavaScript Getting the protocol, domain, port from a URL with JavaScript Jul 20, 2021 - The property created on the global object for global variables, is set to be non-configurable because the identifier is to be treated as a variable, rather than a straightforward property of the global object. JavaScript has automatic memory management, and it would make no sense to be able to use ... Sep 21, 2009 - Then the client started getting specific about the design content. Suddenly there’s buttons to click, things that hide or display, live updating of various bits of content… the JavaScript has grown from a nice small file to a monster (although it’s still smaller than loading jQuery).
Agreed, this is my favorite answer as it specifically addresses JavaScript variable assignment concerns. Additionally, if you choose to use a ternary as one of the subsequent variables to test for assignment (after the operator) you must wrap the ternary in parentheses for assignment evaluation to work properly. 30/11/2020 · How to use the var keyword in JavaScript. Keywords in JavaScript are reserved words. When you use the var keyword, you’re telling JavaScript you’ll be declaring a variable. When using the var keyword, variables can be reassigned. We’ll demonstrate this by first declaring a new variable, name, and assigning it to the value of Madison. May 06, 2019 - If you used to code in Javascript in the old days, you used the var keyword a lot. There was no other way to declare a variable. It was really simple. The only thing you needed to do was just…
Babel's guide to ES6 says:. let is the new var.. Apparently the only difference is that var gets scoped to the current function, while let gets scoped to the current block.There are some good examples in this answer.. I can't see any reason to use var in ES6 code. Even if you want to scope a given variable to the whole function, you can do so with let by putting the declaration at the top of ... HTML CSS JAVASCRIPT SQL PYTHON PHP BOOTSTRAP HOW TO W3.CSS JAVA JQUERY C++ C# R React Kotlin ... JS HOME JS Introduction JS Where To JS Output JS Statements JS Syntax JS Comments JS Variables JS Let JS Const JS Operators JS Arithmetic JS Assignment JS Data Types JS Functions JS ... Feb 13, 2018 - Most Javascript experts agree var shouldn’t be used. Douglas Crockford, the man who popularized JSON, is against the use of var. He indicates that, “var might possibly still be useful in an extreme case like machine-generated code, but I’m stretching hard there.
ES6 introduced two important new JavaScript keywords: let and const. These two keywords provide Block Scope in JavaScript. Variables declared inside a { } block cannot be accessed from outside the block: Example. {. let x = 2; } // x can NOT be used here. Variables declared with the var keyword can NOT have block scope. When using var to declare variables JavaScript initiates the variable at the top of the current scope regardless of where it has been included in the code. So the fact that we have declared d locally within the conditional is irrelevant. Essentially JavaScript is reinterpreting this code as:- When I first started learning Javascript I had a hard time wrapping my head around when to use the var, let, and const keywords. I was used to writing native iOS apps in Swift (and Objective-C when…
Variable means anything that can vary. In JavaScript, a variable stores the data value that can be changed later on. Use the reserved keyword var to declare a variable in JavaScript. A variable is a "named storage" for data. We can use variables to store goodies, visitors, and other data. To create a variable in JavaScript, use the let keyword. The statement below creates (in other words: declares) a variable with the name "message": let message; Now, we can put some data into it by using the assignment operator =: A variable defined using var is known throughout the function it is defined in. function doSomething () { // x is know throughout this function var x = 0; // rest of the code } If the variable is defined outside a function, it means it is a global variable and has global scope. It is known throughout the whole code.
Const Vs Let Vs Var In Javascript Which One Should You Use
What Are The Differences Between Var Let And Const
Difference Between Var And Let In Javascript Geeksforgeeks
Using Variables And Variables Types In Javascript Var Let
In Javascript How Declaring A Variable With Let Is
How To Loading Objects Using Sharepoint Jsom Incworx
Empty Quotes Assigned To Variable Explanation Solved
Learn How To Get Current Date Amp Time In Javascript
Handling Common Javascript Problems Learn Web Development Mdn
Why Is My Variable Unaltered After I Modify It Inside Of A
How To Use Let Var And Const In Javascript By Dr Derek
Difference Between Var And Let In Javascript Geeksforgeeks
Understanding Hoisting In Javascript Digitalocean
We Need To Minimize The Use Of Global Variables In Javascript
Use Php Variable As Javascript Function Parameters Stack
Goodmodule On Twitter I Don 39 T Use Var In Es6 I Use
Javascript Hoisting With Examples Dot Net Tutorials
Javascript Scope Of Variables Huey2672 博客园
Javascript Es6 Function Scope Amp Block Scope Nerd For Tech
Javascript Variables A To Z Guide For A Newbie In
Variables In Javascripts The Engineering Projects
Javascript Hoisting Var Let And Const Variables Vojtech
How To Get Attachments Url In All Folder Use Javascript
Javascript Var Let Or Const Which One Should You Use
A Guide To Javascript Variable Hoisting With Let And Const
Javascript Basics Variables In Javascript You Can Use
Javascript 5 Let Const And Var
Var Vs Let Vs Const In Javascript Ui Dev
Javascript Var Vs Let Which One Should You Use Dev Community
0 Response to "32 Javascript When To Use Var"
Post a Comment