27 Javascript Api Post Example
Making a POST Request in JavaScript This is an important part of REST API in JavaScript, Now learn to make a POST request in Javascript. Time to try our hands-on making a POST request. Since this is not the default method in the fetch API we need to add it in the fetch() call. When it comes to retrieving data client-side using Javascript the Dynamics 365 Web API is the best way to do this. In this post I'm going to share a reusable Javascript function I've written that makes using the Web API and retrieving data a breeze. I'll also step through an example based on the lead form and the existing contact field.
Here Are The Most Popular Ways To Make An Http Request In
Dec 07, 2017 - A big part of working with JavaScript is knowing how to connect to APIs. As a fledgling developer, you may have been told at some point to…
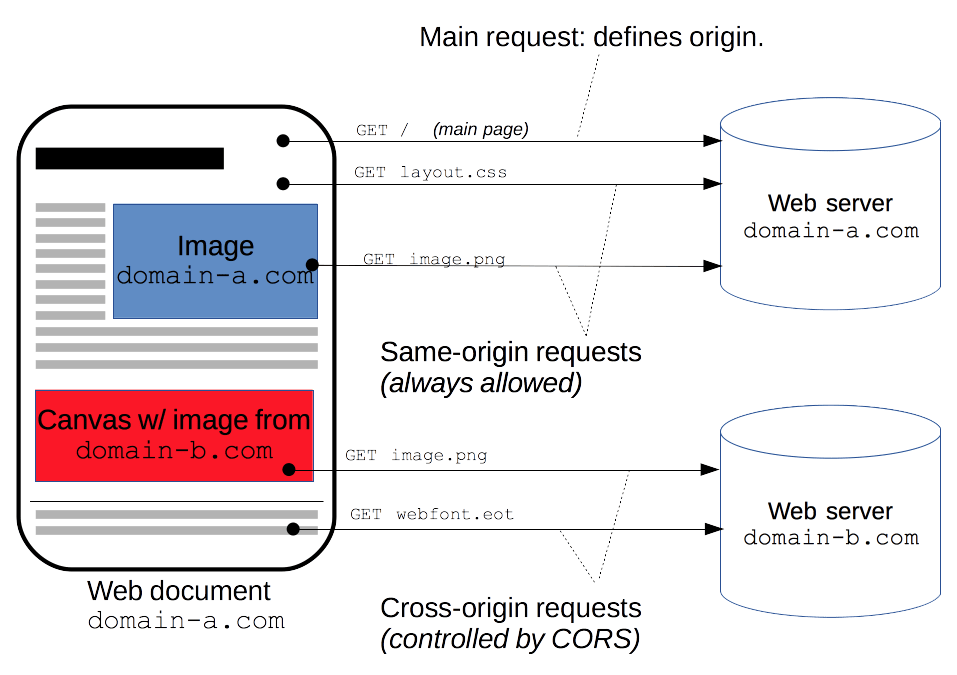
Javascript api post example. Jul 07, 2020 - In JavaScript, it was really important to know how to make HTTP requests and retrieve the dynamic data from the server/database. JavaScript provides some built-in browser objects and some external… As mentioned above, name of the action methods in the Web API controller plays an important role. Action method name can be the same as HTTP verbs like Get, Post, Put, Patch or Delete as shown in the Web API Controller example above. However, you can append any suffix with HTTP verbs for more readability. Javascript HTTP Post Request Fetch API Example | JSONPlaceholder API ExampleWelcome Folks My name is Gautam and Welcome to Coding Shiksha a Place for All Pro...
This example is very simple and does not support the GET method. If you are interesting by more sophisticated examples, please have a look at the excellent MDN documentation. See also similar answer about XMLHttpRequest to Post HTML Form. ArcGIS API for JavaScript; ArcGIS Runtime API for Android; ArcGIS Runtime API for iOS; ArcGIS Runtime API for .NET; ArcGIS Runtime API for Java; ArcGIS Runtime API for Qt; ArcGIS API for Python; Open Source APIs. Esri Leaflet; ArcGIS REST JS; 3rd Party API Clients. Mapbox GL JS; OpenLayers; Extending ArcGIS. ArcGIS Pro SDK; ArcGIS Enterprise ... May 14, 2017 - Here is a complete example: After spending hours tinkering with incomplete code snippets I finally managed to post some json from javascript, pick it up using php on a server, added a data field and finally updated the original web page. Here is the HTML, the PHP and the JS.
Check it out the Fetch API demo.. Summary. The Fetch API allows you to asynchronously request for a resource. Use the fetch() method to return a promise that resolves into a Response object. To get the actual data, you call one of the methods of the Response object e.g., text() or json().These methods resolve into the actual data. Mar 23, 2020 - I just completely redesigned my APIs & JavaScript pocket guide with modern ES6 approaches, including Promises and fetch(). This is an excerpt. (The examples below use the JSON Placeholder API.) Specifying the HTTP method with fetch() The fetch() method accepts an optional second argument: an ... Nov 04, 2018 - If you look back at the ... is a JavaScript object. This provides an easy way to get values from the body of the request, but it’s not something that happens automatically. The API you are building, expects clients to send JSON formatted data in the body of POST and PUT ...
21/8/2019 · Here is an example of a POST request: const url = 'https://reqres.in/api/users' ; // post body data const user = { first_name : 'John' , last_name : 'Doe' , job_title : 'Blogger' } ; // request options const options = { method : 'POST' , body : JSON . stringify ( user ) , headers : { 'Content-Type' : 'application/json' } } // send POST request fetch ( url , options ) . then ( res => res . json ( ) ) . then ( … Returning a promise allows us to chain these sample operations together in a way that is preferred by many developers to traditional callback functions. For more information about promise, see JavaScript Promise. See also. Use the Dataverse Web API Web API Samples Web API Samples (C#) May 07, 2020 - In this article, we are going to ... in JavaScript. ... Ajax is the traditional way to make an asynchronous HTTP request. Data can be sent using the HTTP POST method and received using the HTTP GET method. Let’s take a look and make a GET request. I’ll be using JSONPlaceholder, a free online REST API for developers ...
Stack Exchange network consists of 178 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.. Visit Stack Exchange The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch () method that provides an easy, logical way to fetch resources asynchronously across the network. This kind of functionality was previously achieved using XMLHttpRequest. 22/8/2020 · GET — Get data from the API. For example, get a twitter user based on their username. POST — Push data to the API. For example, create a new user record with name, age, and email address. PUT — Update an existing record with new data. For example, update a user’s email address. DELETE — Remove a record.
This example fetches the requested HTML snippet and inserts it on the page. Pages fetched with POST are never cached, so the cache and ifModified options in jQuery.ajaxSetup() have no effect on these requests. The jqXHR Object. As of jQuery 1.5, all of jQuery's Ajax methods return a superset of the XMLHTTPRequest object. This post describes how to make API calls in Typescript, and how we can support types and interfaces while calling REST APIs. If you just want to see the example code, go here Typescript helps developers by providing static analysis and autocomplete features for their Javascript code. One of the best features of jQuery AJAX Method is to load data from external website by calling APIs, and get the response in JSON or XML formats. In this example I will show you how easy it is to make such API calls in jQuery AJAX. OpenWeatherMap API. The OpenWeatherMap API provides the complete weather information for any location on Earth including over 200,000 cities.
Call the web API with JavaScript 2.1. In this section, an HTML page is added that uses JavaScript to call the web API. jQuery initiates the request. JavaScript updates the page with the details from the web API's response. Configure the app to serve static files and enable default file mapping by updating Startup.cs with the following ... This sample shows how the Fetch API can make POST requests. May 22, 2017 - I am trying to send an Http post request to parse server through Rest API keys. Not sure if I am doing it right as below. The following is my whole script and makes a button which should trigge...
Specifies the data type expected of the server response. By default jQuery performs an automatic guess. Possible types: "xml" - An XML document. "html" - HTML as plain text. "text" - A plain text string. "script" - Runs the response as JavaScript, and returns it as plain text. "json" - Runs the response as JSON, and returns a JavaScript object. The jQuery $.post() method allows you to post data to the server in a single line. This is a simple wrapper for the more advanced $.ajax method. Below is an example of sending JSON to the ReqBin echo URL with jQuery ajax method. 26/8/2021 · JavaScript/AJAX REST API POST Example. To send data to the REST API server using JavaScript/AJAX, you must send an HTTP POST request and include the POST data in the request's body. You also need to provide the Content-Type: application/json and Content-Length request headers. Below is an example of a REST API POST request to a ReqBin REST API endpoint.
POST request using fetch API: The post request is widely used to submit forms to the server. Fetch also supports the POST method call. To do a POST request we need to specify additional parameters with the request such as method, headers, etc. In this example, we'll do a POST request on the same JSONPlaceholder and add a post in the posts. The Post Method in Web API application allows us to create a new item. Here, we want to add a new Employee to the Employees table. First, Include the following Post () method within the EmployeesController. Notice that the Employee object is being passed as a parameter to the Post method. The Employee parameter is decorated with the [FromBody ... This example fetches the requested HTML snippet and inserts it on the page. Pages fetched with POST are never cached, so the cache and ifModified options in
If you are looking for how to consume restful web services in javascript, call rest service from HTML page, jquery rest API post, ajax API, call example, jquery API call, calling web API from jquery ajax, javascript call rest API JSON. This tutorial solves your all queries related to calling APIs (web services). It can be named either Post or with any suffix e.g. POST (), Post (), PostNewStudent (), PostStudents () are valid names for an action method that handles HTTP POST request. The following example demonstrates Post action method to handle HTTP POST request. Example: Post Method in Web API Controller Sep 21, 2020 - Now, JavaScript has its own built-in way to make API requests. This is the Fetch API, a new standard to make server requests with promises, but includes many other features. In this tutorial, you will create both GET and POST requests using the Fetch API.
Jun 28, 2019 - A simple POST request using the fetch API. GitHub Gist: instantly share code, notes, and snippets. Issue an HTTP POST request. // Using a binary array as body. Make sure to open() the file as binary For each of these actions, JAAS API provides a corresponding endpoint. Browse APIs In order to demonstrate the entire CRUD functionality in JavaScript, we will complete the following steps: Make a POST request for the API used to create the object. We will save object id which was received in the answer.
XMLHttpRequest is the safest and most reliable way to make HTTP requests. To send form data with XMLHttpRequest, prepare the data by URL-encoding it, and obey the specifics of form data requests. Let's look at an example: And now the JavaScript: const btn = document.querySelector('button'); function sendData( data ) { console.log( 'Sending data ... Mar 02, 2021 - It’s a common task for JavaScript developers to send GET and POST requests to retrieve or submit data. There are libraries like Axios that help you do that with beautiful syntax. However, you can achieve the same result with a very similar syntax with Fetch API, which is supported in all ... 8/4/2020 · I also pass a content-type to set the content as application/json and an authorization header to authenticate my request with a Bearer token I was assigned through the API dashboard. XHR. The first code example is XHR, which we can use in the browser natively, and in Node.js using https://www.npmjs /package/xmlhttprequest:
Axios tutorial shows how to generage requests in JavaScript using Axios client library. Axios is a promise based HTTP client for the browser and Node.js. However, always use POST requests when: A cached file is not an option (update a file or database on the server). Sending a large amount of data to the server (POST has no size limitations). Sending user input (which can contain unknown characters), POST is more robust and secure than GET.
Node Js Rest Apis Example With Express Sequelize Amp Mysql
Asynchronous Javascript Using Promises With Rest Apis In Node Js
Post Response Body Javascript Code Example
Example Of Vanilla Javascript Fetch Post Api In Laravel 5
Adding Batch Or Bulk Endpoints To Your Rest Api
Axios Post Request To Send Form Data Stack Overflow
Angular 8 Tutorial Rest Api And Httpclient Examples
Get And Post Method Using Fetch Api Geeksforgeeks
Put Vs Post What S The Difference
Sharepoint 2013 Rest Api Javascript Example Archives Global
The Same Post Api Call In Various Javascript Libraries
Step 4 Request Example Api Reference Tutorial
How To Use An Api With Javascript Beginner S Guide
Javascript How To Make Jquery Ajax Post Request With
Javascript Fetch Api Tutorial With Js Fetch Post And Header
Write Api Tests For Http Post Method
Cross Origin Resource Sharing Cors Http Mdn
How Http Post Request Work In Nodejs Geeksforgeeks
Building A Restful Api Using Node Js And Mongodb Nordic Apis
What Are Get Post Put Patch Delete A Walkthrough With
Tutorial Host Restful Api With Cors Azure App Service
Talking To Python From Javascript Flask And The Fetch Api
Get Vs Post Difference Between Get And Post Method Edureka
Esp32 Http Get And Http Post With Arduino Ide Random Nerd
0 Response to "27 Javascript Api Post Example"
Post a Comment