24 Javascript Array Count Values
12/5/2020 · Object.defineProperties(Array.prototype, { count: { value: function(query) { /* Counts number of occurrences of query in array, an integer >= 0 Uses the javascript == notion of equality. */ var count = 0; for(let i=0; i<this.length; i++) if (this[i]==query) count++; return count; } } }); 1/6/2020 · Use array methods. JavaScript includes a bunch of helpful methods when working with arrays. Each one can be chained to an array and passed different parameters to work with while iterating through the elements in the array. The two we'll look at are filter() and reduce(). filter()
Javascript Array Distinct Ever Wanted To Get Distinct
JavaScript fundamental (ES6 Syntax): Exercise-70 with Solution. Write a JavaScript program to count the occurrences of a value in an array. Use Array.prototype.reduce () to increment a counter each time the specific value is encountered inside the array. This Pen is owned by w3resource on CodePen .
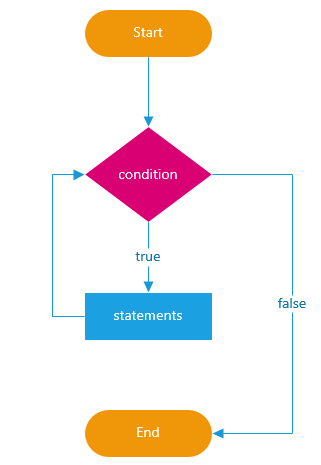
Javascript array count values. reason: When next().done=true or currentIndex>length the for..of loop ends. See Iteration protocols.. Value: there are no values stored in the array Iterator object; instead it stores the address of the array used in its creation and so depends on the values stored in that array. If you need to find the index of a value, use Array.prototype.indexOf (). (It's similar to findIndex (), but checks each element for equality with the value instead of using a testing function.) If you need to find if a value exists in an array, use Array.prototype.includes (). The array we are going to count property-values from. Now we can use the map method. WHAT IS THE MAP METHOD? Well, it’s something that creates a new array out of the existing array. As an example, if we have an array like: Atomic = [78,79,47]; we can create a new array like so: Atomic.map(x => x*2)
In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf() method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.. indexOf() Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf() method. count how many times each value appears in an array js count number of times an element is occuring in an array in javascriptjavascript number of times an item is in an array js how to find the most common elements in array javascript There are two common ways (in ES5 and ES6, respectively) of getting unique values in JavaScript arrays of primitive values. Basically, in ES5, you first define a distinct callback to check if a value first occurs in the original array, then filter the original array so that only first-occurred elements are kept. In ES6, the code is much simpler.
Creates a new array with the result of calling a function for each array element: pop() Removes the last element of an array, and returns that element: push() Adds new elements to the end of an array, and returns the new length: reduce() Reduce the values of an array to a single value (going left-to-right) reduceRight() Reduce the values of an ... Answer 7. I think this is the simplest way how to count occurrences with same value in array. var a = [true, false, false, false]; a.filter(function(value) { return value === false; }).length. Answer 8. You can have an object that contains counts. Walk over the list and increment the count for each element: Count how many empty values is has: (length - length after removing the empty values) var empties = arr.length - arr.filter(function(x){ return true }).length; // return 3 or something like this
The length property of an object which is an instance of type Array sets or returns the number of elements in that array. The value is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array. Returns the array item at the given index. Accepts negative integers, which count back from the last item. Array.prototype.concat() Returns a new array that is this array joined with other array(s) and/or value(s). Array.prototype.copyWithin() Copies a sequence of array elements within the array. Array.prototype.entries() The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana").
4/4/2019 · function array_count_values (arr) {//returns array let a = [], prev; arr.sort (); for ( let i = 0; i < arr.length; i++ ) { if ( arr [i] !== prev ) { a.push (1) } else { a [a.length-1]++; } prev = arr [i]; } return a; } And another that returns an object: In JavaScript, the array is a single variable that is used to store different elements. It is usually used once we need to store a list of parts and access them by one variable. We can store key => value array in JavaScript Object using methods discussed below: Method 1: In this method we will use Object to store key => value in JavaScript ... Finding Max and Min Values in an Array. There are no built-in functions for finding the highest or lowest value in a JavaScript array. You will learn how you solve this problem in the next chapter of this tutorial.
Asks the user for values using prompt and stores the values in the array. Finishes asking when the user enters a non-numeric value, an empty string, or presses "Cancel". Calculates and returns the sum of array items. P.S. A zero 0 is a valid number, please don't stop the input on zero. Run the demo If start is negative, it is treated as array.length + start.; If end is negative, it is treated as array.length + end.; fill is intentionally generic: it does not require that its this value be an Array object.; fill is a mutator method: it will change the array itself and return it, not a copy of it.; If the first parameter is an object, each slot in the array will reference that object. 24/11/2020 · Counting below / par elements from an array - JavaScript; How to add new array elements at the beginning of an array in JavaScript? Counting duplicates and aggregating array of objects in JavaScript; Turning a 2D array into a sparse array of arrays in JavaScript; Add two consecutive elements from the original array and display the result in a ...
1. Array contains a primitive value. A primitive value in JavaScript is a string, number, boolean, symbol, and special value undefined. The easiest way to determine if an array contains a primitive value is to use array.includes() ES2015 array method: Count Duplicate Values in an Array in JavaScript with forEach We can use the JavaScript array forEach method to loop through the array we want to count the duplicates for and add the count of each item into an object. For instance, we can write: Javascript get array length Example. Let's see the simple example of How to JavaScript check array length. On Clicking a button "myFunction()" function will call. In a function, the first script will create an array and assigning value to the count variable.
The array.values () function is an inbuilt function in JavaScript which is used to returns a new array Iterator object that contains the values for each index in the array i.e, it prints all the elements of the array. Syntax: arr.values () Return values: It returns a new array iterator object i.e, elements of the given array. An array can hold many values under a single name, and you can access the values by referring to an index number. JavaScript Array length ... Return Value: A Number, representing the number of elements in the array object: JavaScript Version: ECMAScript 1: Related Pages. Array Tutorial. Array Const. Array Methods. Sorting Arrays. Array Iterations
Group array by equal values JavaScript. Let's say, we have an array of string / number literals that contains some duplicate values like this −. We are required to write a function groupSimilar () that takes in this array and returns a new array where all the repeating entries are group together in a subarray as the first element and their ... @AntoineNedelec The initial value is a new Map object; see the second argument of the reduce.Map.prototype.set returns the map object, and Map.prototype.get returns undefined or the value of whatever key is supplied to it. This lets us get the current count of each letter (or 0 if undefined), then increment that by one, then set that letter's count to the new count, which returns the map and ...
How To Get Last Element Of An Array In Javascript
How To Find Even Numbers In An Array Using Javascript
Java Program To Count Positive And Negative Numbers In An Array
Using Node Js To Read Really Really Large Datasets Amp Files
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Javascript Fundamental Es6 Syntax Count The Occurrences Of
Creating Indexing Modifying Looping Through Javascript
Java67 How To Remove A Number From An Integer Array In Java
How To Get Unique Values In An Array Stack Overflow
Count Repeated Values In Array Javascript
How To Replace An Element In Array In Java Code Example
Javascript Fundamental Es6 Syntax Count The Occurrences Of
Vuejs Count Array Of Total Items Example Pakainfo
5 Examples Of How To Get Python List Length Array Tuple
Java Program To Count Even And Odd Numbers In An Array
Javascript While Loop By Examples
Javarevisited 3 Ways To Find Duplicate Elements In An Array
Most Efficient Way To Create A Zero Filled Javascript Array
Javascript Array Splice Vs Slice Stack Overflow
Javascript Function Letter Count Within A String W3resource
Javascript Arrays How To Count Poperty Values Including
0 Response to "24 Javascript Array Count Values"
Post a Comment