27 Javascript Check If Function
3. Write a JavaScript function that generates all combinations of a string. Go to the editor. Example string : 'dog'. Expected Output : d,do,dog,o,og,g. Click me to see the solution. 4. Write a JavaScript function that returns a passed string with letters in alphabetical order. Go to the editor. 4 weeks ago - The hasOwnProperty() method returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it).
Javascript Math Check If A Number Is A Whole Number Or Has A
29/4/2020 · To check if a particular function name has been defined, you can use the typeof operator: Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) if (typeof myFunctionName === 'function') { myFunctionName (); } In the given case, the typeof operator will return undefined because myFunctionName () has not been defined.
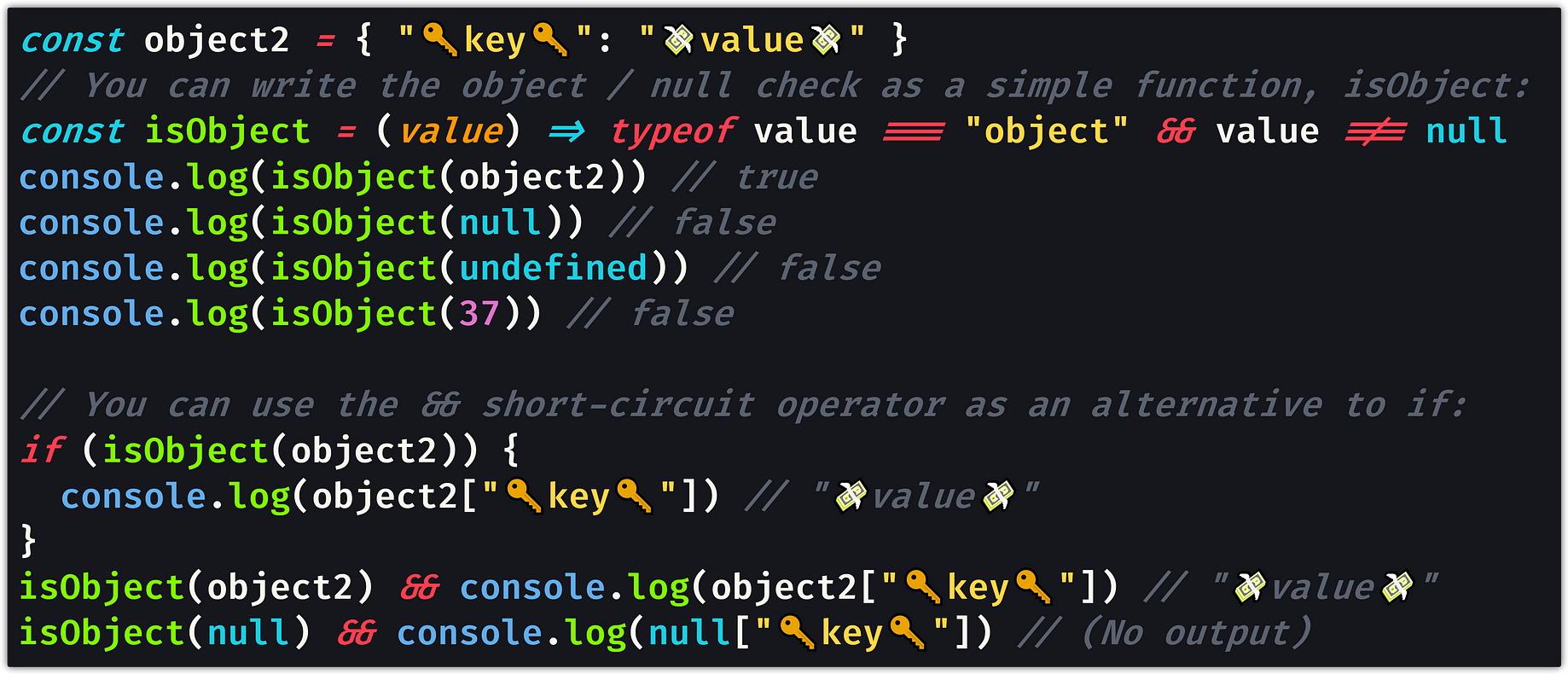
Javascript check if function. Jan 02, 2020 - Sometimes you might want to call a function in Javascript but check if the function exists before calling it. This is very simple and is covered in this post. In the following examples, a JavaScript function is used to check a valid date format against a regular expression. Later we take each part of the string supplied by user (i.e. dd, mm and yyyy) and check whether dd is a valid date, mm is a valid month or yyyy is a valid year. We have also checked the leap year factor for the month of February. Jan 30, 2019 - In Chrome typeof(obj) === 'function' appears to be the fastest by far; however, in Firefox obj instanceof Function is the clear winner. ... Regarding the part: typeof should only be used for checking if variables or properties are undefined. At javascript.info/tutorial/type-detection in the ...
JavaScript Functions¶ JavaScript functions are the main blocks of code that are designed to perform a particular task. Functions allow the code to be called many times without repetition. Variables that are defined inside a function cannot be accessed from anywhere outside that function, as it is defined only in the scope of the function. JavaScript Check If Checkbox is Checked Demo. With the help of onClick event, JavaScript function and this keyword, we can pretty easily check If the checkbox is checked. It also allows us to add any condition we want within our if and else statement. JavaScript Multiple Checkboxes Onclick Example. Aug 05, 2020 - Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance:
Aug 25, 2020 - Write a function that checks whether a person can watch an MA15+ rated movie javascript One of the following two conditions is required for admittance: Jun 22, 2021 - The has() method returns a boolean indicating whether an element with the specified key exists or not. 27/3/2021 · To prevent the error, you can first check if a function exists in your current JavaScript environment by using a combination of an if statement and the typeof operator on the function you want to call. Only when the typeof returns "function" will you call that function to execute it. Let’s look at the following example:
Compared to typeof approach, the try/catch is more precise because it determines solely if the variable is not defined, despite being initialized or uninitialized.. 4. Using window.hasOwnProperty(). Finally, to check for the existence of global variables, you can go with a simpler approach. Each global variable is stored as a property on the global object (window in a browser environment ... Jul 20, 2021 - The has() method returns a boolean indicating whether an element with the specified value exists in a Set object or not. In JavaScript you have 0 to 11 for the months, so the month of June would be #5. In the IF condition we added "&& z==5" to check and see if we were in the month of June. If these conditions were met we display, "Its the weekend in the month of June". Next we added a "else if" statement to check for another condition.
terrific, you are on 1st place in google for javascript check it function exists, thank you. Reply. Darin Reid March 26, 2013 at 5:04 am. Hey neighbor, imagine my surprise when I googled this tonight. See you at coffee! Reply. jeffreifman March 26, 2013 at 5:26 am. HA! We should cowork 🙂 Email me your email again … as I can't seem to ... Jul 20, 2018 - A couple of days ago I shared a technique for checking if a function exists before trying to run it. The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed. The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions.
Jul 20, 2021 - The instanceof operator tests to see if the prototype property of a constructor appears anywhere in the prototype chain of an object. The return value is a boolean value. The truth about truthiness In ifstatements, JavaScript evaluates the statement to a boolean trueor false, and acts accordingly. But when certain values are encountered, JavaScript "coerces" them to a boolean value. For example, these statements are all correct: In the first implementation of JavaScript, JavaScript values were represented as a type tag and a value. The type tag for objects was 0. null was represented as the NULL pointer (0x00 in most platforms). Consequently, null had 0 as type tag, hence the typeof return value "object".
This seems like a bad idea to me. You shouldn't ever need to know if a function is asynchronous, because it can be treated the same as a non-async function that returns a Promise, and all functions can be treated as if they return a Promise by enclosing the call in Promise.resolve().I can't think of a single circumstance when this would actually be a sensible way to achieve a goal. The Array.isArray() method determines whether the passed value is an Array. Here is a working and simple solution for checking existence of a function and triggering that function dynamically by another function; Trigger function function runDynamicFunction(functionname){ if (typeof window[functionname] == "function") { //check availability window[functionname]("this is from the function it"); // run function and pass a parameter to it } }
Y ou can check for a function using the typeof keyword, which will return "function" if given the name of any JavaScript function. How To Check Data Types in JavaScript using typeof Check for one of nine strings: undefined, object (null), boolean, number, bigint, string, symbol, function, or object… JavaScript: check if function parameter is a string or an object. Filed under: JavaScript— Tagged with: jquery. If a function takes a DOM element as a parameter, it can be a string, or a jQuery object, for example. Or why not both? With a small conditional check it's possible. The if (...) statement evaluates a condition in parentheses and, if the result is true, executes a block of code.
An IF statement can be used to compare if the returned value is of the type 'Function'. //javascript check if function-Using object.prototype.toString <script > // Declare a variable and initialize it with an anonymous function var exampleVar = function() { /* A set of statements */ }; // to check a variable is of function type or not function checkFunction(x) { if (Object.prototype.toString.call(x) == '[object Function]') { document.write("Variable is of function … Jul 20, 2021 - The logical NOT (!) operator (logical complement, negation) takes truth to falsity and vice versa. It is typically used with Boolean (logical) values. When used with non-Boolean values, it returns false if its single operand can be converted to true; otherwise, returns true. The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false. This function is different from the Number specific Number.isNaN () method.
We have various ways to check if a value is a number. The first is isNaN (), a global variable, assigned to the window object in the browser: const value = 2 isNaN(value) //false isNaN('test') //true isNaN({}) //true isNaN(1.2) //false If isNaN () returns false, the value is a number. In the above program, the checkPalindrome() function takes input from the user. The length of the string is calculated using the length property. The for loop is used to iterate up to the half of the string. The if condition is used to check if the first and the corresponding last characters are the same. This loop continues till the half of ... In this article, we learned how to check if a variable in JavaScript is a number. The Number.isNaN () function is only suitable if we know our variable is a number and need to verify if it specifically NaN or otherwise. The typeof () function is suitable if your code can work with NaN, Infinity or -Infinity as well as other numbers.
JavaScript Program to Check If a Variable is of Function Type JavaScript Program to Check If a Variable is of Function Type In this example, you will learn to write a JavaScript program that will check if a variable is of function type. To understand this example, you should have the knowledge of the following JavaScript programming topics: Well, if you're not on the homepage and there is no slider, but you're calling it in your javascript, you'll get an uncaught Object error in your console. So, what Chris is saying, is check to see if that function exists somewhere, if it is, then let it through, if not, don't call it. To check if a JavaScript function is defined or not, checks it with "undefined".ExampleYou can try to run the following example to check for a function is d ...
We create two different variables - a function type variable and a string type variable. We create a custom function called isFunction, which checks to see if a JS variable is of type "function" or not. This returns TRUE or FALSE. We then test both of our variables and log the results to the console. Code language: JavaScript (javascript) When you click the button, you can call the check () function to select all checkboxes. Next time, when you click the button, it should uncheck all the checkboxes. To do this switch, you need to reassign the click event handler whenever the click event fires. Check if a JavaScript function exists before calling it. To check if a particular function name has been defined, you can use JavaScript's typeof operator: //Use the typeof operator to check if a JS function exists. if (typeof bad_function_call === "function") { bad_function_call (); }
1 week ago - The if statement executes a statement if a specified condition is truthy. If the condition is falsy, another statement can be executed. Variable is of function type Using Strict Equal (===) operator: In JavaScript, '===' Operator is used to check whether two entities are of equal values as well as of equal type provides a boolean result. In this example, we use the '===' operator. This operator, called the Strict Equal operator, checks if the operands are of the same type. Jul 20, 2021 - The global undefined property represents the primitive value undefined. It is one of JavaScript's primitive types.
Python Conditional Statements If Else Elif Amp Switch Case
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check If A Variable In Javascript Is Undefined Or Null
How To Check If An Object Has A Property Properly In
How To Call Javascript Function In Html Javatpoint
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check For A Function In Javascript By Dr Derek
Devcurry Jquery Check If Function Exists Before Calling
Check If A String Is A Not A Number With Isnan Function In
How To Check For An Object In Javascript Object Null Check
How To Check If A String Contains A Substring In Javascript
How To Use Vlookup And If Functions Together Excelchat
Check If All Values In Array Are True Javascript Code Example
Javascript Programming With Visual Studio Code
Differentiability At A Point Algebraic Function Is
Formula Or Function For If Statement Based On Cell Color
How To Use Excel If Statement With Multiple Conditions Range
Javascript Check If Object Exists In Array Code Example
Index Match Functions Used Together In Excel
How To Check Nan In Javascript Weekly Webtips
Javascript Function Check Whether A Passed String Is
Check If Javascript Function Exists Or Is Defined
How To Check If A Javascript Promise Has Been Fulfilled
Check If A String Contains A Substring In Javascript
Check If One Column Value Exists In Another Column Excelchat
How To Check If A Function In Windows Parent Exists In
0 Response to "27 Javascript Check If Function"
Post a Comment