24 How To Select All Class In Javascript
22/3/2019 · document.querySelectorAll() selects all elements of given class name and store them in an array-like object. It is possible to loop through the objects instead of accessing them manually. var elements = document.querySelectorAll('.className'); for(var i = 0; i < elements.length; i++){ var str = elements[i].innerHTML; elements[i].innerHTML = str.replace(":smile:", "<i class='em em-smile'></i>"); } Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
Document Object Model Dom The Document Object Model Is A
In JavaScript, the standard way of selecting an element is to use the document.getElementById("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows:
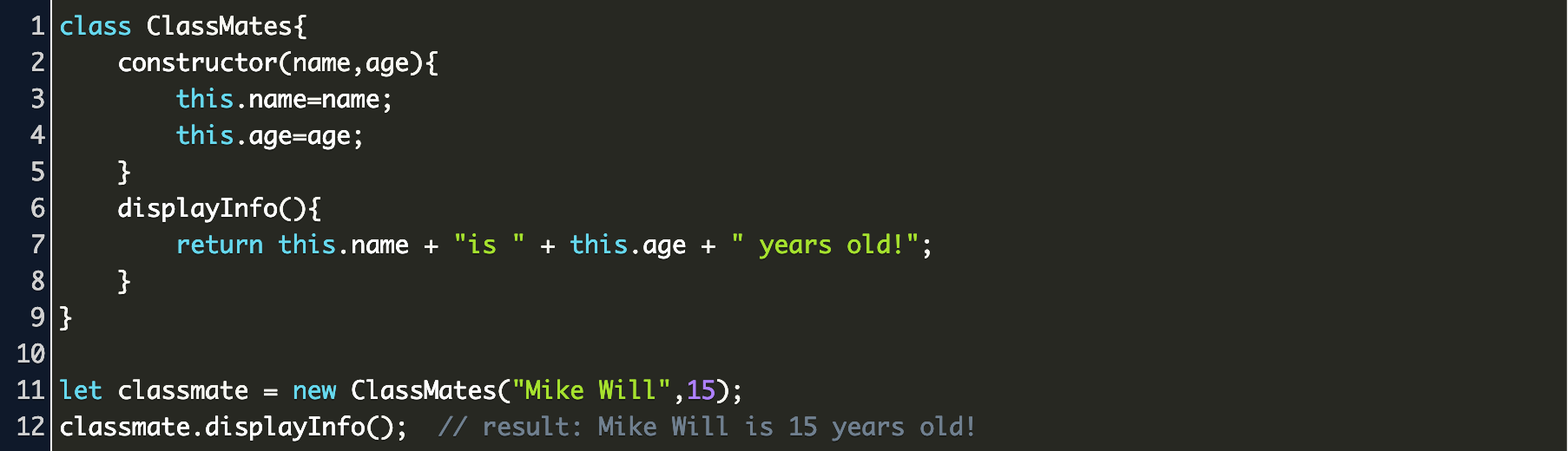
How to select all class in javascript. 20/11/2020 · How to Select All <div> Elements with JavaScript J avaScript has a built-in function called document.getElementsByTagName () that will select all the instances of a certain HTML element on the current webpage based on its tag name, i.e. <div>. Query Selector All. Want to select a list of elements that match the specified selectors? Use querySelectorAll() method instead. This method takes multiple CSS selectors as input and returns a NodeList — a list of DOM elements that match the given selectors. Let us select all elements with a class of either bird or animal from the above HTML ... Sometimes, we want to select an item with a class within a div with JavaScript. In this article, we'll look at how to select an item with a class within a div with JavaScript. Use document.querySelector. We can use document.querySelector on to select a div and then select an element within it with the given class after that.
Selecting Elements by Class Name Similarly, you can use the getElementsByClassName () method to select all the elements having specific class names. This method returns an array-like object of all child elements which have all of the given class names. Let's check out the following example: Class methods are non-enumerable. A class definition sets enumerable flag to false for all methods in the "prototype". That's good, because if we for..in over an object, we usually don't want its class methods. Classes always use strict. All code inside the class construct is automatically in strict mode. Introduction to Select Class in Selenium. In Selenium, the Select class provides the implementation of the HTML SELECT tag. A Select tag provides the helper methods with select and deselect options. As Select is an ordinary class, its object is created by the keyword New and also specifies the location of the web element.
Check total options and total selected options if it is equal then set Select All checkbox checked otherwise unchecked it. When working with HTML, you'll use classes to give elements a particular style with CSS. In this video, you'll learn to select elements with the same class, using <code>document.getElementsByClassName</code>. On the other hand, the classList property returns a live DOMTokenList collection of all the classes applied to a DOM element. It can be used to easily add, remove, toggle, and iterate through all the classes. Read Next: Hide and show DOM elements using a CSS class in JavaScript. ️ Like this article? Follow me on Twitter and LinkedIn. You can ...
The class syntax does not introduce the new object-oriented inheritance model to JavaScript. Define a class in Javascript. One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code. A cross browser compatible JavaScript function to select or de-select all checkboxes in a form. The check all function finds all checkboxes in a designated form, regardless of the checkbox name. JQuery version also available You can select an HTML element using the class with jQuery on a page. Use the dot (.) sign before the class to access the required element. A class name not required to be unique and you can use the same class name for many HTML elements. This applies the script to all the elements with the same class name. Test it Live.
How to get CSS values in JavaScript 27th Jun 2018. CSS alone is not enough sometimes. You might need to control your CSS values with JavaScript. But how do you get ... Here we take advantage of the query methods' ability to specify everything from html tags, classes, child elements etc. A bit overkill in this case but you get the idea. This would return the paragraphs with the class of "theLastParagraph" that are descendents of a div tag. In the case above there is of course only one. The querySelector () is a method of the Element interface. The querySelector () allows you to find the first element that matches one or more CSS selectors. You can call the querySelector () method on the document or any HTML element. The following illustrates the syntax of the querySelector () method: In this syntax, the selector is a CSS ...
Toggling the class means if there is no class name assigned to the element, then a class name can be assigned to it dynamically or if a certain class is already present, then it can be removed dynamically by just using the toggle() or by using contains(), add(), remove() methods of DOMTokenList object within JavaScript.. Properties of HTML elements being used: The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name (s). When called on the document object, the complete document is searched, including the root node. Definition and Usage. The .class selector selects all elements with the specific class. The class refers to the class attribute of an HTML element. The class attribute is used to set a particular style for several HTML elements. Note: Do not start a class attribute with a number. It may cause problems in some browsers.
9/12/2006 · all, but I only know how to select one element at a time, e.g. Element.hide('element). How can I do this to multiple elements with the same id or class name? No you can't with same id because id is unique on a same page :-(If elements are with same class : You can create a special class for the body which will say to this class: hop! hide ! A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {} Copy. const y = class {} version added: 1.0 jQuery ( ".class" ) class: A class to search for. An element can have multiple classes; only one of them must match. For class selectors, jQuery uses JavaScript's native getElementsByClassName () function if the browser supports it.
In JavaScript, you can use the.getElementsByTagName () method to get all the <li> elements in <ul>. In-addition, you can use the.querySelectorAll () method also to get all the <li>. I have shared few simple examples here explaining how you can use the method to extract all the LI elements. In JavaScript its easy : Let say we have a div having class clsPanel and inside that dive other elements resides with the same class name. Then you can select the div by following code: var selectAllClass= document.getElementsByClassName(clsPanel)... Thanks! I did actually end up taking the opportunity to test all sorts of combos for quite a while to get a better understanding. As for my particular issue, I recall it being some kind of silly mistake like trying to select class when it was a tag, or a typo, or something silly like that.
Using jQuery Selector, you can target or select HTML elements based on their IDs, classes, types, attributes and much more than a DOM. Simple Defination of jQuery Selector jQuery selectors are used to selecting one or more HTML elements using jQuery, and once you select the element you can take various actions on it. Since the getElementsByClassName () is a method of the Element, you can select elements with a given class inside a container. The following example shows only the innerHTML of the element with the CSS class note inside the container: node.textContent = text Property values: It contains single value text which specifies the text content of the specified node. Return value: It returns a string, representing the text of node and all its descendants. It returns null if the element is a document, a document type, or a notation. HTML DOM innerText Property: This property set/return the text content of defined node, and all its ...
class Car {. constructor (name, year) {. this.name = name; this.year = year; } } The example above creates a class named "Car". The class has two initial properties: "name" and "year". A JavaScript class is not an object. d3.select(".myclass").classed("myanotherclass"); This will return true, if any element in the selection has the class. Use d3.select for single element selection. Toggle class − To flip a class to the opposite state - remove it if it exists already, add it if it does not yet exist - you can do one of the following. In this article, we will learn how we can access an element (s) based on its class. GetElementsByClassName () method is used to retrieve a collection or array of all the HTML elements that are child nodes of the element on which this method is called and have the class as mentioned in the parameter of this method.
Select With Class Name Selector Myclass In Jquery
Omotola Shogunle Talks Us Through How To Build Your Very Own
The Provided Project S Output Class Has Two Methods Chegg Com
Id Classes Attributes Tags Dev Community
How To Select All Child Elements Recursively Using Css
How To Add Options To A Select Element Using Jquery
Work With Fields Using Js On Plumsail Forms Public Forms
How To Create Function For Select Multiple Row In Javascript
Adding Methods To A Class In Javascript Code Example
Github D3 D3 Selection Transform The Dom By Selecting
Add Or Remove Multiple Classes In Javascript For Dom Element
How To Select Value From Dropdown Using Selenium Webdriver
Javascript Preprocessor And Javascript Postprocessor
How To Render Js Variable In A Kendo Ui S Itemtemplate When
How To Add And Remove Classes In Vanilla Javascript
Css Naming Conventions That Will Save You Hours Of Debugging
Select All A Elements From A Page Stack Overflow
Click Ctrl Key To Select A Row And Then Add A Css Class To
Select Elements In Sequence By Group Class Name Stack
How To Select Text By Class Name And Copy Questions
How To Select A Div With A Certain Class Using Jquery
In Javascript Consider The Following Selector Chegg Com
0 Response to "24 How To Select All Class In Javascript"
Post a Comment