25 Reusable Code In Javascript
In this challenge we are introduced to functions. Functions allow us to reuse code as much times as we want. When we want to use some code we wrote in java... Functions — reusable blocks of code. Another essential concept in coding is functions, which allow you to store a piece of code that does a single task inside a defined block, and then call that code whenever you need it using a single short command — rather than having to type out the same code multiple times.
9 5 10 Learning Functional Javascript With Ramda
There are huge amount of JavaScript tutorials out there, but you seldom see a tutorial that teach you how you should organize your code into reusable chunks. i came from c# background where classes provide encapsulation , in JS there is no proper encapsulation in functions. searching as deep as i can i found ecmascript modules.
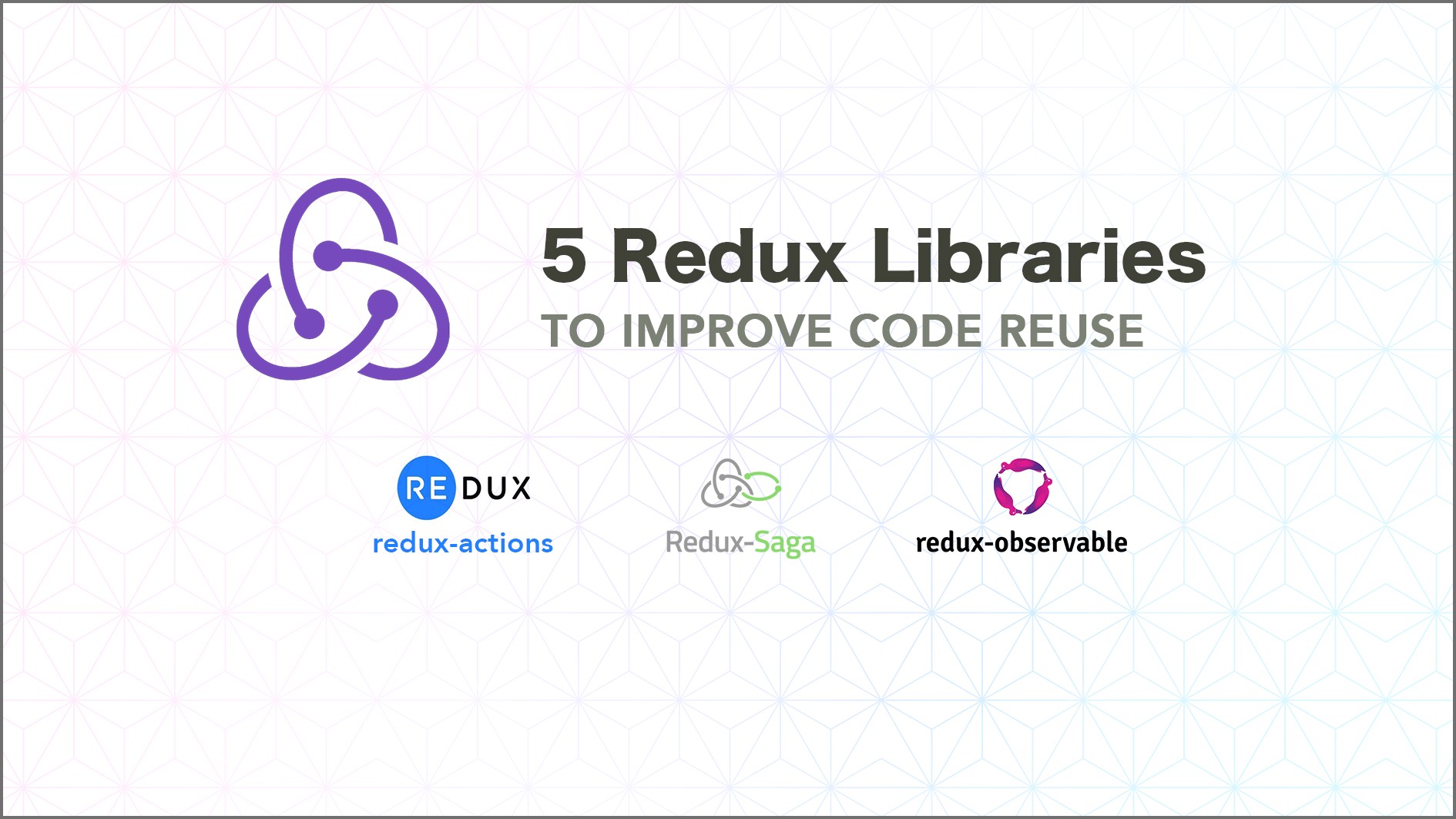
Reusable code in javascript. A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it). Example. function myFunction (p1, p2) {. return p1 * p2; // The function returns the product of p1 and p2. } There are probably endless ways to create reusable components in Javascript. But let me do a quick compilation and generalization: The "traditional way" - Grouping functions and objects together. Javascript Modules - Importing and exporting, or require in the "NodeJS way". Creating Reusable Behaviors with Javascript Functions. Javascript functions are an important part of the Javascript standard. They allow you to create reusable blocks of code with specific names and behaviors so you don't have to repeat the same thing multiple times, which is extremely helpful for code readability and maintenance.
Since the JavaScript code is placed at the top and HTML gets loaded from top to bottom, this fixes the problem of running JavaScript code on html elements that haven't been loaded. 4. Add <div>... Let the code inside the attack function create a monster and add it to the global array, array.push(monster). Having said this, please post the relevant code, what you have tried, so that it can be corrected and improved.. - BatScream Jan 2 '15 at 7:52 N.B. Chunk in this writing could mean functions, variables, objects, arrays or basically reusable blocks of code. In the early days of the web, there was no standardized method of distributing and sharing reusable code across various files and as a result, JavaScript programs were mostly written entirely in one file and loaded into a script tag.
Every programming language focus on the 'DRY - Do not Repeat Yourself' or the Reusability principle. JavaScript also focuses on the same. As a developer identify the reusable code and move them to functions. So, that it can be reuse, test once, avoid errors, save time, and have consistency. 11/4/2021 · JavaScript modules had been launched in 2009, with the ES5 model of the programming language. Using modules builders had been now in a position to create customized items of code and export them to be utilized in different sections of a JavaScript software. The Basic Structure of the Module Pattern (operate() HTML templates. One of the least recognized, but most powerful features of the Web Components specification is the <template> element. In the first article of this series, we defined the template element as, "user-defined templates in HTML that aren't rendered until called upon." In other words, a template is HTML that the browser ignores until told to do otherwise.
This reduces wasted DOM calls, can improve the speed of your JavaScript code and makes code more reusable. The following code snippet caches the current selected DOM element as a jQuery object using the variable name $$. Secondly, the plugin makes its settings available to the Metadata plugin ‡ which is best practice within jQuery plugins. Functions are very useful blocks of code that are reusable throughout your application. Javascript functions are only executed if they are called on. This is useful if you have a few lines of code that need to be used in multiple places in your application. This can save alot of time when writing code. 25/3/2021 · **Your code so far** function reusableFunction() { console.log("Hi World"); } **Your browser information:** User Agent is: Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.90 Safari/537.36. Challenge: Write Reusable JavaScript with Functions. Link to the challenge: freecodecamp
The Javascript standard provides the ability to create reusable code in the form of Javascript objects. Javascript objects provide complex functionality with minimal code, making new scripts easy to maintain and fast to load. Object-oriented Javascript Programming Explained Javascript objects follow a strategy called object-oriented programming. 1. Use only the good parts (Amazon : JavaScript: The Good Parts (9780596517748): Douglas Crockford: Books). 2. Use JSHint to rigorously maintain clean code (JSHint - Documentation) 3. Organize javascript code into self-contained AMD modules w... JavaScript Algorithms and Data Structures. Basic JavaScript. Write Reusable JavaScript with Functions. In JavaScript, we can divide up our code into reusable parts called functions. Here's an example of a function: function functionName {console. log ("Hello World");}
JavaScript - Functions, A function is a group of reusable code which can be called anywhere in your program. This eliminates the need of writing the same code again and again. It helps 1. #3 Professional JavaScript Development Creating Reusable Code Doc. v. 0.1 - 26/03/09 Wildan Maulana | wildan [at] tobethink . 2. When developing code with other programmers, which is standard for most corporate or team projects, it becomes fundamentally important to maintain good authoring practices in order maintaom your sanity. 1/6/2021 · Your code so far // Example function ourReusableFunction() { console.log("Heyya, World"); } ourReusableFunction(); // Only change code below this line function reusableFunction() { console.log("Hi World"); } Your browser information: User Agent is: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/80.0.3987.116 Safari/537.36.
15/12/2015 · Code example: index.html - how I call f1.js in the html page (my code) <script type="text/javascript" src="f1.js"> </script>. f1.js - my code. function hello () { Math.seedrandom ("1"); // this is the method I want to reuse from f2.js alert ("hello: " + Math.random ()); } Today we go about using reusable JavaScript code to write functions. This may be the most important tutorial thus far. Writing clean, reusable function is ou... makeuseof - Understanding how to use design patterns will enable you to use reusable code in JavaScript. Here's what you need to know. If you ever want to create …
But what if your application grows and you need to repeat the same code for the header and footer 5, 10, or say 15 times? Remember the DRY (Don't Repeat Yourself) principle of software development. With the introduction of Web Components, it becomes easy to solve this problem and to create reusable HTML components. add (eggs); boil (5*60*1000); // 5 mins. And you can keep iteration this, but not too far, to not create too many abstractions. In conclusion, you have all "implementations details of entities" separated and reusable, and "application's flow (scenario)" which uses that entities - also clean and cheap for change. JavaScript supports user-defined functions, which is highly advantageous for developing a library of reusable code. You can place code you think you might reuse into one or more functions and save...
Writing reusable JavaScript. ... this helps when we need to debug and allows us to reuse this block of code: ... We have split the original callback into 2 reusable functions. 1/10/2020 · This is one of the major problems things like React or Handlebars.js solve: any code, especially structural things like a header or footer, can be written once and easily reused throughout a project. Until recently it wasn't possible to use components in vanilla HTML and JavaScript. 11/4/2021 · If you ever want to create reusable JavaScript code or collaborate with a team of developers, then you need to know how to use and identify the different design patterns in the language. In JavaScript, the term design pattern refers to a specific way of writing code and is often thought of as a programming template.
The 3 Essential Rules Of Really Reusable Javascript
Using The Children Prop For Dynamic And Reusable React
Write Reusable Javascript With Functions Free Code Camp
Professional Javascript Development Creating Reusable Code
Joel Thoms Javascript On Twitter Tip Abstract Code
Hire Dedicated React Native Mobile Developers Top React
Vanilla Javascript Code Snippets Smashing Magazine Hostulum
5 Key Redux Libraries To Improve Code Reuse Logrocket Blog
Javascript Four Principles Of Object Oriented Programming
Increase Code Reuse Reduce Overhead By Jonathan Saring
Must Know Reusable Module Vs Component In Vue 3 Composition
Challenge Won T Pass Javascript The Freecodecamp Forum
Typescript For Javascript Developers Migrate From Javascript
How To Maximize Your Ability To Reuse Code Across Projects
Reusable Html Components How To Reuse A Header And Footer
Basic Javascript Write Reusable Javascript With Functions
How To Declare Functions In Javascript
Building Reusable Components Using React Buttercms
How To Reduce Reuse And Recycle Your Code By Amy Medium
Functions In Javascript A Function Is A Group Of Reusable
Functions In Javascript A Function Is A Group Of Reusable
Java Vs Javascript Most Important Differences You Must Know
Easy Learning Design Patterns Es6 Javascript 2 Edition
1 Javascript Shadi Banitaan Outline Introduction Java Script
0 Response to "25 Reusable Code In Javascript"
Post a Comment