32 Construct Html In Javascript
Aug 16, 2016 - As I learn JavaScript I've been looking around the web and seen numerous references to constructs in Javascript, but I can't seem to find a complete definition of what they are and what they are not, The HTML <script> Tag The HTML <script> tag is used to define a client-side script (JavaScript). The <script> element either contains script statements, or it points to an external script file through the src attribute. Common uses for JavaScript are image manipulation, form validation, and dynamic changes of content.
A Practical Guide To Typescript How To Build A Pokedex App
We will save this file in the same directory as our index.html file. Table of Contents hide. 1 Fetching the JSON data. 2 Displaying the JSON data. 2.1 Step 1 - Get the div element from the body. 2.2 Step 2 - Loop through every object in our JSON object. 2.3 Step 3 - Append each person to our HTML page.
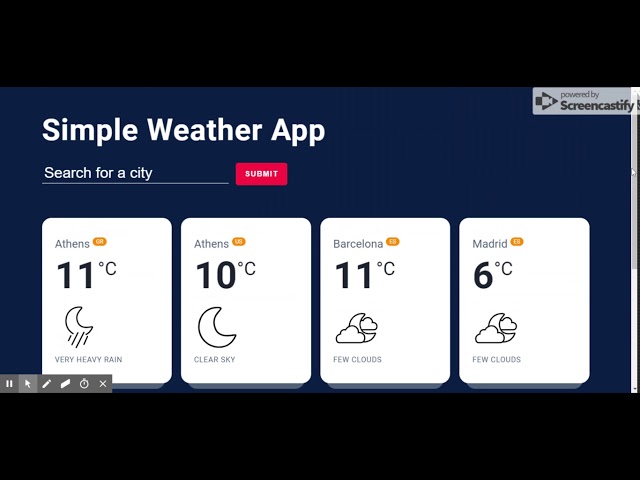
Construct html in javascript. Aug 01, 2020 - Scirra announced today that they will be adding JavaScript language support to their currently codeless cross platform game engine, Construct 3. We did a hands-on video on Construct 3 shortly after it was released, and the lack of scripting support was one of my biggest complaints.Details of ... In this tutorial you'll learn how to create HTML elements using JavaScript. In other words, we'll dynamically create HTML elements with JavaScript code. Get... Jan 09, 2019 - The majority of JS frameworks uses some sort of techniques to handle or manipulate the Document Object Model (DOM) tree. The DOM makes it possible for programs such as JavaScript to change the…
Object Definitions Object Properties ... Object Constructors Object Prototypes Object Iterables Object Sets Object Maps Object Reference ... Function Definitions Function Parameters Function Invocation Function Call Function Apply Function Closures ... DOM Intro DOM Methods DOM Document DOM Elements DOM HTML DOM Forms ... In this module, you'll learn how to: Create a basic web page using HTML. Apply styles to page elements using CSS. Create themes using CSS. Add support for switching between themes using JavaScript. Inspect the website using browser developer tools. Start. Object Definitions Object Properties ... Object Constructors Object Prototypes Object Iterables Object Sets Object Maps Object Reference ... Function Definitions Function Parameters Function Invocation Function Call Function Apply Function Closures ... DOM Intro DOM Methods DOM Document DOM Elements DOM HTML DOM Forms ...
How to add JavaScript to html with javascript tutorial, introduction, javascript oops, application of javascript, loop, variable, objects, map, typedarray etc. ... Now let's create separate JavaScript file. Hello.js. Output. Both of the above programs are saved in the same folder, but you can also store JavaScript code in a separate folder, all ... The Constructor Method. The constructor method is a special method: It has to have the exact name "constructor" It is executed automatically when a new object is created; It is used to initialize object properties; If you do not define a constructor method, JavaScript will add an empty constructor method. Among the many awesome qualities of this library is the ability to easily create DOM elements and place them in the document without the normally verbose syntax of native JavaScript. But there is a little-known feature of JavaScript that allows you to create option elements with fairly minimal effort. This feature is the Option() constructor.
Here, in this article I’ll show you how to convert JSON data to an HTML table dynamically using JavaScript. In addition, you will learn how you can dynamically create a table in JavaScript using createElement() Method. JavaScript Create Element. Creating DOM elements using js, adding text to the new element and appending the child to the Html body is not a hard task, it can be done in just a couple of lines of code. Let's see in the next example: var div = document.createElement ('div'); //creating element div.textContent = "Hello, World"; //adding text on ... This tutorial is all about creating form using JavaScript. In this tutorial, we are going to see how to create simple html form using JavaScript step by step. So if you want to create a form dynamically with javascript, you must read this tutorial.
Mar 24, 2020 - by Kaashan Hussain We all deal with objects in one way or another while writing code in a programming language. In JavaScript, objects provide a way for us to store, manipulate, and send data over the network. There are many ways in which objects in JavaScript differ from objects in HTML Code Explanation: Here we added different buttons in the document, which will get the power to perform some tasks we give to it with the help of JavaScript. We have added buttons for changing the font-weight of the input string, font style, text alignment of the string, and are going to transform the string using the Document Object Model. Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document:
8/7/2010 · There are basically two ways to do this. You can build one long HTML string and append it: var data = ['a', 'bunch', 'of', 'things', 'to', 'insert'] var html = '' for (var i = 0; i < data.length; i++) { html += '' + data + '' } $('#tablerow').append(html) Or you can use an array which is typically a little faster. To begin coding the game, create a new folder in your documents. Use your favorite text editor to open that folder, then create three new files and name them: index.html, style.css, and script.js.It's possible to do everything in one file with HTML5, but it's more organized to keep everything separate. Create a list of bookmarks. In this example, we are going to create a list of bookmarks, append bookmarks to parent element and then present it in HTML using plain JavaScript. The starting point is an index.html file that consist of a div element with an ID of bookmarks. The ID is an important and necessary factor in order to locate the element ...
Object Definitions Object Properties ... Object Constructors Object Prototypes Object Reference Object Map() Object Set() ... Function Definitions Function Parameters Function Invocation Function Call Function Apply Function Closures ... DOM Intro DOM Methods DOM Document DOM Elements DOM HTML DOM Forms ... 9/2/2015 · our Construct 2 port to html and in particular to ELGG. We feel they will benefit the community. The primary goals are: jsob - adds JavaScript object and generalize array object, btb - adds more of JavaScript, html - adds an arbitrary html element. and a bit more ... We are using them routinely for half a … You can create new HTML elements or any other element you want with createElement. For example, if you want to create a new <p> element as a child of the <body> element, you can use the myBody in the previous example and append a new element node. To create a node call document.createElement ("tagname").
The way to create an "object type", is to use an object constructor function. In the example above, function Person () is an object constructor function. Objects of the same type are created by calling the constructor function with the new keyword: const myFather = new Person ("John", "Doe", 50, "blue"); proto. The object which should be the prototype of the newly-created object. propertiesObject Optional. If specified and not undefined, an object whose enumerable own properties (that is, those properties defined upon itself and not enumerable properties along its prototype chain) specify property descriptors to be added to the newly-created object, with the corresponding property names. We can take advantage of the JavaScript syntax to select a field of the loginForm form as formElement.nameOfField, where formElement is your HTML <form> and nameOfField is the value given to the...
You can create an element using document.createElement. After creation you can add attributes. If you want the element to show up in your document, you have to insert in into the DOM-tree of the document. Try playing around with this code: Nov 02, 2017 - Loops are used in programming to automate repetitive tasks. In this tutorial, we will learn about the for statement, including the for...of and for...in statements, which are essential elements of the JavaScript programming language. Constructing HTML with templates. Using JavaScript makes it possible to dynamically add and remove HTML content in the browser. This article shows an approach to adding HTML content that is common, but results in poor readability. It then gradually modifies the code to introduce templates as a better way to generate and display new HTML content.
Object.prototype.constructor. The constructor property returns a reference to the Object constructor function that created the instance object. Note that the value of this property is a reference to the function itself, not a string containing the function's name. The value is only read-only for primitive values such as 1, true, and "test". Though this is a beginner tutorial, you'll still need to be familiar with the basics of both HTML and JavaScript to understand it. If you're new to JavaScript programming, you may want to take this JavaScript beginners course before we start off. The A Link Tag in HTML. The A tag in HTML is used to create hyperlinks on a webpage. Another feature of template literals or template strings is the ability have multi-line strings without any funny business.. Previously with regular string we would have escape the new lines like so: var text = " hello there, \n how are you + \n ";. That gets to be quite a task, to be able to do that.
The following code improperly initializes the proxy. The target in Proxy initialization must itself be a valid constructor for the new operator. The static Reflect.construct() method acts like the new operator, but as a function. It is equivalent to calling new target(...args). It gives also the added option to specify a different prototype. According to the W3C File API specification, the File constructor requires 2 (or 3) parameters. So to create a empty file do: var f = new File([""], "filename"); The first argument is the data provided as an array of lines of text; The second argument is the filename ; The third argument looks like:
Lines 5 and 6 create a new paragraph element with some simple content, and then lines 8-12 handle inserting the new paragraph into the new document. Line 16 pulls the contentDocument of the frame; this is the document into which we'll be injecting the new content. May 28, 2019 - This section covers some details about how Javascript code is run in Construct. Construct's event sheets fundamentally work differently to code. Consequently ob... Note: A new HTMLElement is returned if the document is an HTMLDocument, which is the most common case. Otherwise a new Element is returned. ... This creates a new <div> and inserts it before the element with the ID "div1".
Dynamically create a HTML form with Javascript. Ask Question Asked 11 years, 1 month ago. Active 2 months ago. Viewed 84k times 19 7. how can I go about creating a form with javascript? I have NO idea on how to proceed, I have been googling my face off but there is nothing definitive that can show me how to dynamically create forms with it. ... Nov 18, 2016 - Another feature of template literals or template strings is the ability have multi-line strings without any funny business. Previously… The constructor() method is a special method for creating and initializing objects created within a class. The constructor() method is called automatically when a class is initiated, and it has to have the exact name "constructor", in fact, if you do not have a constructor method, JavaScript will add an invisible and empty constructor method.
A constructor enables you to provide any custom initialization that must be done before any other methods can be called on an instantiated object. If you don't provide your own constructor, then a default constructor will be supplied for you. If your class is a base class, the default constructor is empty: If your class is a derived class, the ... How To Create a Login Form or page with HTML CSS and Javascript. All source code can be found in the description. All links for source code in the descriptio...
Web Design 101 How Html Css And Javascript Work
How To Make A Website Using Html Css And Javascript Step By Step Website Design Tutorial
How To Create A Simple Web App Using Javascript By Belle
How To Create An Image Element Dynamically Using Javascript
Migrate Sharepoint Javascript Customizations To Sharepoint
Html Building Blocks Javatpoint
Build A Weather App With Vanilla Javascript By Using Openweathermap Api Amp Custom Icons
Build A Dynamic Jamstack App With Gatsbyjs And Faunadb Css
How Does A Browser Create A Web Page Oncrawl
Esp8266 Web Server Fast Development Of Html Js With Node
Client Server Overview Learn Web Development Mdn
Pure Javascript Building A Real World Application From
How To Populate Html Table From Array Using Javascript With Source Code
How To Manage Text Input And Output With Javascript For Html5
Get The Benefits Of Jsx From React In Vanilla Js Toptal
Shiny Build Your Entire Ui With Html
Build A Simple Website Using Html Css And Javascript
The Document Object Model Eloquent Javascript
How To Create Interactive Websites With Javascript By
How Javascript Works The Internals Of Shadow Dom How To
Using The Html Checkbox Amp Managing The Check State With
Programming Foundations With Javascript Html And Css Coursera
Web Design 101 How Html Css And Javascript Work
Custom Elements Defining New Elements In Html Html5 Rocks
10 Best Javascript Certification Courses Classes Online 2021
An Introduction To Building And Sending Html Email For Web
How To Pick Up React From A Python Flask And Html Background
How To Talk To The Page Your Game Sits In Free Tutorial
Programmers Sample Guide Dynamically Generate Html Table
0 Response to "32 Construct Html In Javascript"
Post a Comment