29 Javascript Unique Array Of Objects
Oct 12, 2019 - This is an ancient question, a million years old. Why raising this antique question again? Feb 14, 2019 - Extract unique objects by attribute from array of objects. Understanding basic JavaScript codes.
Javascript Lesson 26 Nested Array Object In Javascript
With JavaScript 1.6/ ECMAScript 5you can use the native filtermethod of an Array in the following way to get an array with unique values: function onlyUnique(value, index, self) { return self.indexOf(value) === index;
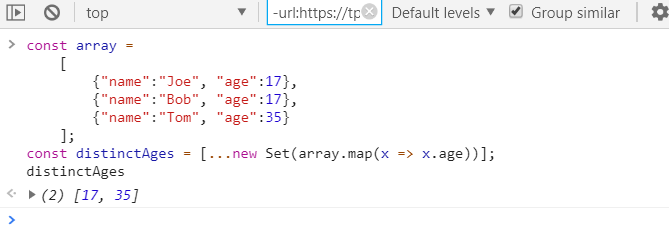
Javascript unique array of objects. Mar 29, 2016 - Browse other questions tagged javascript arrays unique or ask your own question. ... The full data set for the 2021 Developer Survey now available! Podcast 371: Exploring the magic of instant python refactoring with Sourcery ... Extract an elelment's property without repetitions from an array of objects... May 04, 2016 - If you have an array of objects and you want to filter the array to remove duplicate objects but do so based on a provided key/property, this might prove to be a problem you would expect Javascript to address natively. If you are using Lodash then you have many methods at your disposal that ... Sep 24, 2020 - Here are a few ways to filter unique values in javascript. ... Set is a new data structure introduced in ES6. It is somewhat similar to an array but it does not allow us to store duplicate values. To filter unique values we pass an array to the Set constructor and we frame back an array from the Set object ...
Produces a duplicate-free version of the array, using === to test object equality. If you know in advance that the array is sorted, passing true for isSorted will run a much faster algorithm. If you want to compute unique items based on a transformation, pass an iterator function. There are two common ways (in ES5 and ES6, respectively) of getting unique values in JavaScript arrays of primitive values. Basically, in ES5, you first define a distinct callback to check if a value first occurs in the original array, then filter the original array so that only first-occurred elements are kept. In ES6, the code is much simpler. Jul 18, 2020 - //ES6 let array = [ { "name": "Joe", "age": 17 }, { "name": "Bob", "age": 17 }, { "name": "Carl", "age": 35 } ]; array.map(item => item.age) .filter((value, index, self) => self.indexOf(value) === index) > [17, 35]
If it means that instead of "array" being an array of objects, but a "map" of objects with some unique key (i.e. "1,2,3") that would be okay too. Im just looking for the most performance efficient way. Delphi queries related to “get unique values from array javascript” ... Create a function unique(arr) that should return an array with unique items of arr. Jan 04, 2021 - Let’s find the distinct values for a given property among all of the JavaScript objects in an array in this short tutorial. Dr. Derek Austin 🥳 ... Set only accepts one copy of each primitive value added to it, so it’s used to find unique values in JavaScript.
If you are looking for a solution for how to get a unique javascript array, then your answer is […new Set (arr)] method. The triple dot (…) is spread operator. Primitive values in JavaScript are immutable values except for objects. Types of primitive values include Null, Undefined, Boolean, Number, Symbol, and String. Here are 3 ways to filter out duplicates from an array and return only the unique values... Javascript queries related to "get distinct values from list of objects typescript" array unique by key js; js only unique keys; javascript get unique values from key
5/8/2021 · JavaScript Set. The Set object lets you store unique values of any type, whether primitive values or object references. The key word to make note of in the description is unique. The syntax to create a new Set is, new Set([iterable]); Iterable is an Object JavaScript can iterate, or move, through; such as an Array. What Not To Do javascript - Generating an array of unique values of a specific property in an object array - Code Review Stack Exchange Given a table represented as a javascript array of objects, I would like create a list of unique values for a specific property. How to get only unique values from array in JavaScript. In this tutorial, you will learn how to get unique values from json array in javascript with examples. This example will teach you three javascript methods or functions to get unique values from arrays in JavaScript ES6. Three methods to get unique values from arrays in ES6. Using Set
Below is a general function to obtain a unique array of objects (arr) based on a specific property (prop). Note that in the case of duplicates, only the first object with the property value will be retained. ... javascript - find unique objects in array based on multiple properties. 0. filter distinct/map array of objects in javascript? 0. const array = [ { "name": "Joe", "age": 17 }, { "name": "Bob", "age": 17 }, { "name": "Carl", "age": 35 } ] const key = 'age'; const arrayUniqueByKey = [...new Map(array.map(item => [item[key], item])).values()]; console.log(arrayUniqueByKey); /*OUTPUT [ { "name": "Bob", "age": 17 }, { "name": ... You can use array#reduce and create an object with objectId as the key and the object as the values. Once, you have your object, extract all values to get the unique objects using Object.values ().
A Set is a unique collection of items, and it has the advantage over JavaScript objects that you can iterate through the items of a Set in insertion order. In other words, you can loop through a ... Set objects are collections of values. You can iterate through the elements of a set in insertion order. A value in the Set may only occur once; it is unique in the Set's collection. The rule for unique values is one we can use to our advantage here. Mar 27, 2021 - How to Find Unique Values of a Specific Key in an Array of Objects · A simple JavaScript function to find all unique values ... In a previous article — Three Easy Ways to Remove Duplicate Array Values in JavaScript—we covered three different algorithms to create an array of unique values.
Merging arrays in JavaScript isn't all that tricky. Getting unique values in merged arrays can be. We'll look at some different methods and approaches in JavaScript that create unique arrays, including the shiny Set object. filter () method takes a callback function in which we can provide the condition to filter the unique items. If the condition is true then the item will be added to the new array. It return's the new array with unique items. And then we call uniq on the returned array to get the unique values from that returned array. So we get the same result for uniques as the other examples. Conclusion. We can use native JavaScript array methods or Lodash to extract distinct values from a property from an array of objects.
3/7/2021 · In the example above, we want to create a unique array of objects based on the ‘name’ property of each object set. You can see that there is a duplicate property in positions 1 and 4 with key ‘name’ and value, ‘Alessia Medina’ that we need to remove. You can also change the key to either the ‘character’ or ‘episodes’ property. Feb 16, 2020 - How to find the duplicates though? In my case there were 100 unique objects in the array, so while combing through them manually certainly wasn't impossible, it was going to be a tedious task. The solution was to use JavaScript's map method and Set functionality. How can I use Array.filter() to return unique id with name? My scenario is slightly different than the solutions I have researched in that I have an array of objects. Every example I find contains a flat array of single values.
The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Therefore, you can use this method to filter an array of objects by a specific property's value, for example, in the following way: If a match is not found then the Array.prototype.filter () method will return ... From this array of objects, if you wish to extract the values of all object properties called "name" for example into a new array, you can do so in the following ways: Using Array.prototype.map() You can use the Array.prototype.map() method to create a new array consisting of only specific key's values. One way to get distinct values from an array of JavaScript objects is to use the array's map method to get an array with the values of a property in each object. Then we can remove the duplicate values with the Set constructor. And then we can convert the set back to an array with the spread operator.
javascript create variable containing an object that will contain three properties that store the length of each side of the box · javascript check if elements of one array are in another Sep 28, 2018 - It’s a collection of unique values. We put into that the list of property values we get from using map(), which how we used it will return this array: ... Passing through Set, we’ll remove the duplicates. ... is the spread operator, which will expand the set values into an array. Download my free JavaScript ... Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift.
Remove duplicates from js array (ES5/ES6). GitHub Gist: instantly share code, notes, and snippets. 5/1/2021 · When you have an array of objects in JavaScript, you usually want to work with the unique objects — you don’t want any duplicate objects that could cause bugs or a poor user experience. However, JavaScript has two definitions of what makes a unique object: An object’s reference (its variable name or location in memory) For most Arrays, we can fix this problem using Set, which is a JavaScript global object that forces each of its values to be unique and discards any duplicates. See the example below: However, if we use Set on an array of JSON Objects, it doesn't remove the duplicates.
Remove Object From An Array Of Objects In Javascript
Javascript Array Distinct Ever Wanted To Get Distinct
Creating Objects In Javascript 4 Different Ways Geeksforgeeks
How To Find Unique Values By Property In An Array Of Objects
How To Deep Clone An Array In Javascript Dev Community
Converting Object To An Array Samanthaming Com
Javascript Get Unique Array Elements Of Objects Remove
How To Count Unique Items In Javascript Arrays Thisdavej
How To Create Own Custom Array Implementation In Python
Js Array From An Array Like Object Dzone Web Dev
Creating A List Of Objects Inside A Javascript Object Stack
Group Array Objects Using Javascript
Get A Unique List Of Objects In An Array Of Object In
How To Find Unique Dates In An Array In Javascript By Dr
How To Create Array Of Objects In Java Javatpoint
Javascript Merge Array Of Objects By Key Es6 Reactgo
Javascript Array Distinct Ever Wanted To Get Distinct
Javascript Array Distinct Ever Wanted To Get Distinct
Remove Duplicate Values From Js Array Stack Overflow
Get Unique Values From Array Of Objects Javascript Lodash
How To Find Unique Objects In An Array In Javascript By
The Easy Way To Create A Unique Array Of Json Objects In
Javascript Sort Array Of Objects Code Example
5 Way To Append Item To Array In Javascript Samanthaming Com
Javascript Array Contain Same Object Multiples Times When I
10 Rendering Lists Part 2 Iterating Over Objects Java
How To Update An Array Of Objects Property Value In
0 Response to "29 Javascript Unique Array Of Objects"
Post a Comment