23 Javascript Create Array Of Strings
Aug 02, 2017 - An element inside an array can ... can be of different types : string, boolean, even objects or other arrays. This means that it’s possible to create an array that has a string in the first position, a number in the second, an object in the third, and so on. Arrays in Javascript are zero-based, ... The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string.
How To Easily Convert A Javascript Array Into A Comma
You should use objects when you want the element names to be strings (text). You should use arrays when you want the element names to be numbers. ... JavaScript has a buit in array constructor new Array(). But you can safely use [] instead. These two different statements both create a new empty ...
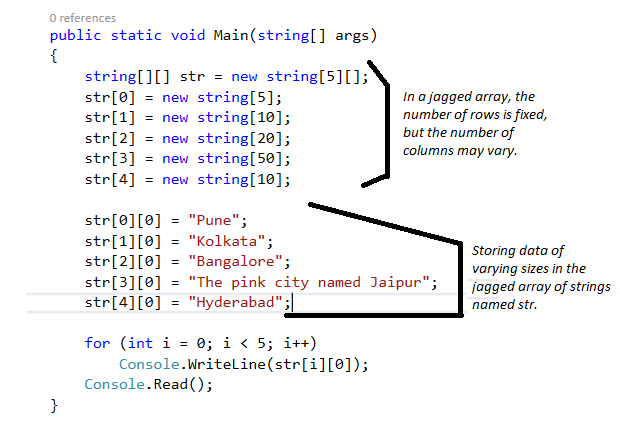
Javascript create array of strings. May 13, 2021 - Asks the user for values using prompt and stores the values in the array. Finishes asking when the user enters a non-numeric value, an empty string, or presses “Cancel”. Calculates and returns the sum of array items. JavaScript does not provide the multidimensional array natively. However, you can create a multidimensional array by defining an array of elements, where each element is also another array. For this reason, we can say that a JavaScript multidimensional array is an array of arrays. An array in javascript can hold many any types of data that is available in the language. We will see different ways to form a string from different types of array. Using toString() method to create a string from array. Using toString() method on an array returns the string representation of the array.
Convert the string to an array. Elements will be separated by the defined SEPARATOR. Click Here: Array.from(STRING, FUNCTION) Convert the given string, to an array. The mapping FUNCTION is optional. Click Here: Object.assign(TARGET, SOURCE) Assigns the SOURCE to the TARGET. Click Here: JSON.parse(STRING) Parse a JSON encoded string back into an ... In this tutorial, we will learn how to create arrays; how they are indexed; how to add, modify, remove, or access items in an array; and how to loop through arrays. Creating an Array. There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword. Learn what is an array and how to use it in JavaScript. Also, learn how to access values from an array.
Jun 30, 2020 - Get code examples like "initialize javascript string array" instantly right from your google search results with the Grepper Chrome Extension. The syntax of creating an array in Java using new keyword −. type [] reference = new type [10]; Where, type is the data type of the elements of the array. reference is the reference that holds the array. And, if you want to populate the array by assigning values to all the elements one by one using the index −. Hacks for Creating JavaScript Arrays Insightful tips for creating and cloning arrays in JavaScript. Original Photo by Markus Spiske on Unsplash. A very important aspect of every programming language is the data types and structures available in the language. Most programming languages provide data types for representing and working with complex ...
You can also use the new keyword to create an array of strings in JavaScript − var animals = new Array ("Time", "Money", "Work"); The Array parameter is a list of strings or integers. When you specify a single numeric parameter with the Array constructor, you specify the initial length of the array. In JavaScript, we can create a string in a couple of ways: Using the string literal as a. In general, a string represents a sequence of characters in a programming language. Let's look at an example of a string created using a sequence of characters, ... method combined with other string and array methods. Let's see one here. It could be a ... Oct 06, 2017 - If you had wanted to add more than one item then badArray would be initialized correctly since Javascript would then be smart enough to know that you were initializing the array instead of stating how many elements you wanted to add. Is what the authors trying to say is Array(10) creates an array ...
However, you can create an associative array in JavaScript, where the array index can be a string representing a keyword, mapping that string to a given value. In the solution, the array index is the identifier given the array element, and the actual array value is the form element value. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions. 1 week ago - The 2 in years[2] is coerced into a string by the JavaScript engine through an implicit toString conversion. As a result, '2' and '02' would refer to two different slots on the years object, and the following example could be true: ... A JavaScript array's length property and numerical properties are connected. Several of ...
Extracts a section of an array and returns a new array. 16. some() Returns true if at least one element in this array satisfies the provided testing function. 17. sort() Sorts the elements of an array. 18. splice() Adds and/or removes elements from an array. 19. toString() Returns a string representing the array and its elements. 20. unshift() Jul 21, 2021 - An array in JavaScript can hold different elements We can store Numbers, Strings and Boolean in a single array. Example: Accessing Array Elements Array in JavaScript are indexed from 0 so we can access array elements as follows: Length property of an Array Length property of an Array returns ... Definition and Usage The toString () method returns a string with all array values separated by commas. toString () does not change the original array.
All of the above three ways are used to initialize the String Array and have the same value. The 3 rd method is a specific size method. In this, the value of the index can be found using the ( arraylength - 1) formula if we want to access the elements more than the index 2 in the above Array.It will throw the Java.lang.ArrayIndexOutOfBoundsException exception. CodinGame is a challenge-based training platform for programmers where you can play with the hottest programming topics. Solve games, code AI bots, learn from your peers, have fun. 15/5/2020 · You can also use the Object.assign () method to convert a string into a characters array as shown below: const str = 'JavaScript'; const chars = Object.assign([], str); console.log( chars); Be careful while using Object.assign () to split a string into an array. This …
Browse other questions tagged javascript arrays string or ask your own question. ... Creating multiline strings in JavaScript. 5722. How do I include a JavaScript file in another JavaScript file? 4283. How do I make the first letter of a string uppercase in JavaScript? 4912. Firstly by using the add method as above. Secondly by passing an array to the constructor ( new Set () ): let arraySet1 = new Set( [3,2,1]); // Set (3) {3, 2, 1} Enter fullscreen mode. Exit fullscreen mode. Duplicated values are also removed if they are included in the initial array: Sep 11, 2020 - For example, you can have an array that stores the number and string, and boolean values. Second, the length of an array is dynamically sized and auto-growing. In other words, you don’t need to specify the array size upfront. ... JavaScript provides you with two ways to create an array.
Given an array containing array elements and here we will join all the array elements to make a single string. To join the array elements we use arr.join () method. This method is used to join the elements of an array into a string. The elements of the string will be separated by a specified separator and its default value is a comma (,). The Object.assign () method copies all enumerable own properties from one or more source objects to a target object The key there is "copies all enumerable own properties". So what we're doing here Object.assign ([], string) it copying ALL of our string properties over to our new array. Which means we have an Array PLUS some string methods. Oct 27, 2020 - The Array.from() static method creates a new, shallow-copied Array instance from an array-like or iterable object.
Viewed 41k times. 23. Is there an easy way to create an array of empty strings in javascript? Currently the only way I can think to do it is with a loop: var empty = new Array (someLength); for (var i=0;i<empty.length;i++) { empty [i] = ''; } but I'm wondering if there is some way to do this in one line using either regular javascript or ... Dec 07, 2018 - In this case, we are creating an empty object with a length property set to 10. The second argument is a function that fills the array with whatever the result of our function is — in our case it is our hello object. ... The array spread syntax allows you to expand an array or string and create ... Nov 07, 2011 - Is there a way to create a dynamic array of strings on Javascript? What I mean is, on a page the user can enter one number or thirty numbers, then he/she presses the OK button and the next page sho...
Oct 14, 2017 - Not the answer you're looking for? Browse other questions tagged javascript arrays string or ask your own question. ... Hello, Toroidal Earth! Cartoon-like characters on the side of the Tianwen-1 Mars lander? In this article, you will learn how to create an array from a string in Javascript. Let's say you have a string variable named 'a' with value "codesource". var a = "codesource"; In order to create an array from the string, you can use the Array.from() method. In this example, you will be creating an array of characters from the string ... Elegant way to convert string of Array of Arrays into a Javascript array of arrays? Ask Question ... to convert that string into an array, as long as you wrap it in some brackets ... greatly on the context and what can appear in place of Name - probably in this case you just want to do something like create a 2D array by extracting all ...
21/6/2021 · There are two methods to do this, which are given below: Method 1: Array traversal and typecasting: In this method, we traverse an array of strings and add it to a new array of numbers by typecasting it to an integer using the parseInt () function. Arrays in JSON are almost the same as arrays in JavaScript. In JSON, array values must be of type string, number, object, array, boolean or null. In JavaScript, array values can be all of the above, plus any other valid JavaScript expression, including functions, dates, and undefined. Java Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets: We have now declared a variable that holds an array of strings. To insert values to it, we can use an array literal - place the values in a comma ...
An array is a collection of the same type variable. Whereas a string is a sequence of Unicode characters or array of characters. Therefore arrays of strings is an array of arrays of characters. Here, string array and arrays of strings both are same term. For Example, if you want to store the name of students of a class then you can use the arrays of strings. Creating an array using the result of a match The result of a match between a RegExpand a string can create a JavaScript array. This array has properties and elements which provide information about the match. Such an array is returned by RegExp.exec(), String.match(), and String.replace(). The sort( ) method accepts an optional argument which is a function that compares two elements of the array.. If the compare function is omitted, then the sort( ) method will sort the element based on the elements values.. Elements values rules: 1.If compare (a,b) is less than zero, the sort( ) method sorts a to a lower index than b.In other words, a will come first.
ARRAY.toString() Convert array to string, elements will be separated by commas. Click Here: ARRAY.JOIN(SEPARATOR) Convert array to string, elements will be separated by defined SEPARATOR. Click Here: ARRAY.flat(LEVEL) Flatten the array to the specified LEVEL. Click Here: JSON.stringify(ARRAY) JSON encode an array or object into a string. Click ... 4 weeks ago - The join() method creates and returns a new string by concatenating all of the elements in an array (or an array-like object), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator. Mar 01, 2021 - JavaScript support various types of array, string array is one of them. String array is nothing but it’s all about the array of the string. The array is a variable that stores the multiple values of similar types. In the context of a string array, it stores the string value only.
The Beginner S Guide To Javascript Array With Examples
Array Of Pointers To Strings In C C Programming Tutorial
Data Structures Objects And Arrays Eloquent Javascript
Introduction To Arrays In Bigquery By Chiefhustler
How To Declare An Empty Array In Java Code Example
Here S Why Mapping A Constructed Array In Javascript Doesn T
How To Implement Array Like Functionality In Sql Server
How To Append An Item To An Array In Javascript
Load Array Of Strings From Config File Help Uipath
How To Remove Commas From Array In Javascript
String Array In Javascript Type Of Array In Javascript With
Excel Cells Amp Ranges Working With Arrays
Gtmtips Create String From Multiple Object Properties Simo
Jquery Convert Json String To Array Sitepoint
How To Remove Array Duplicates In Es6 By Samantha Ming
How To Work With Jagged Arrays In C Infoworld
Javascript Array Distinct Ever Wanted To Get Distinct
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
How To Apply Regular Expression On Array Of Strings String
How To Declare String Array And Assign Value In Array In For
0 Response to "23 Javascript Create Array Of Strings"
Post a Comment