32 How To Convert Json Object To Array In Javascript
var obj = JSON.parse(data); obj.notes.push(addition); var json = JSON.stringify(obj, null, 2); In the following code, we declare a variable named obj that parses the data from the code.json file. We then "push" the object addition into the notes array. Next, in the same above code, we stringify i.e. create an object into a JSON string. Now ... To convert an object into an array in Javascript, you can use different types of methods. Some of the methods are Object.keys (), Object.values (), and Object.entries (). Consider the below examples to understand the above methods. Method 1: Object.keys ()
Js Function To Convert Json Object To Json Array Code Example
Summary: in this tutorial, you will learn how to convert an object to an array using Object’s methods.. To convert an object to an array you use one of three methods: Object.keys(), Object.values(), and Object.entries().. Note that the Object.keys() method has been available since ECMAScript 2015 or ES6, and the Object.values() and Object.entries() have been available since ECMAScript 2017.
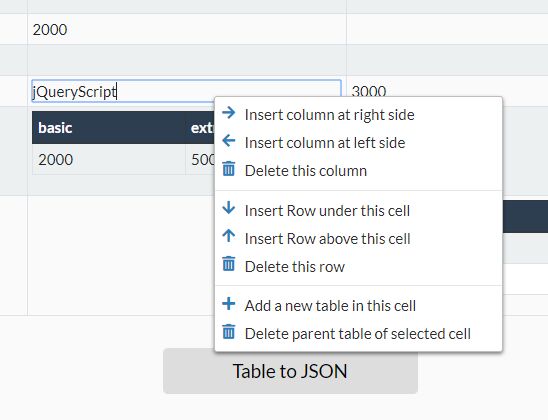
How to convert json object to array in javascript. Luckily, this works the same way as in the browser - just use JSON.stringify() to convert JavaScript object literals or arrays into a JSON string: const newJoke = { categories: ['dev'], value: "Chuck Norris's keyboard is made up entirely of Cmd keys because Chuck Norris is always in command." 20/1/2013 · I need to convert JSON object string to a JavaScript array. This my JSON object: {"2013-01-21":1,"2013-01-22":7} And I want to have: var data = new google.visualization.DataTable(); data.addCol... Convert the JSON string to the JavaScript object using eval () method and then take out the values of the object and push them to the array using push () method.
[code]var JSONItems = []; $.getJSON( "js/settings.json", function( data){ JSONItems = data; console.log(JSONItems); }); [/code]Or [code]var JSONItems = []; $.get( "js ... Find centralized, trusted content and collaborate around the technologies you use most. Learn more A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse(), and the data becomes a JavaScript object.
22/10/2020 · Build tree array from JSON in JavaScript; How to turn a JSON object into a JavaScript array in JavaScript ? How to convert JSON text to JavaScript JSON object? JSON group object in JavaScript; Transform data from a nested array to an object in JavaScript; JavaScript: create an array of JSON objects from linking two arrays; Retrieve key and ... Convert and Loop through JSON with PHP and JavaScript Arrays/Objects | json to array php ; json decode in jquery - How to decode and encode JSON Data in JavaScript? How to find the Json Object Length in Javascript? VueJS json array object string parse Example ; Laravel Convert object Array JSON String Example ; Converting JSON Object into ... How to turn a JSON object into a JavaScript array in JavaScript ? ... Strip quotes with JavaScript to convert into JSON object? From JSON object to an array in JavaScript; How do I turn a string in dot notation into a nested object with a value - JavaScript? Previous Page Print Page.
Convert JSON object to javascript array. by leealdrich. on Jun 9, 2016 at 15:51 UTC. Solved Web Development. 1. Next: Do i need SSl certification for integrate? Get answers from your peers along with millions of IT pros who visit Spiceworks. Join Now. Hi. Please can you help with the following. ... var personObject = JSON.parse(jsonPerson); //parse json string into JS object. convert json object to array javascript. javascript by Creepy Chinchilla on Mar 27 2020 Comment. 0. var as = JSON.parse (jstring); xxxxxxxxxx. 1. var as = JSON.parse(jstring); The JSON Format Evaluates to JavaScript Objects. The JSON format is syntactically identical to the code for creating JavaScript objects. Because of this similarity, a JavaScript program can easily convert JSON data into native JavaScript objects.
Convert JSON String to PHP Array or Object. PHP >= 5.2.0 features a function, json_decode, that decodes a JSON string into a PHP variable. By default it returns an object. The second parameter accepts a boolean that when set as true, tells it to return the objects as associative arrays. You can learn more about the json_decode function from PHP ... To convert the array from the local JSON file to the JavaScript-based object, you can use the ES6 import statement to import the local JSON file and use it in the existing components. Any JSON file can be imported from the local directory. 1 import Students from "./Students"; jsx. Here in the above import statement, the Students will represent ... The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ...
You convert the whole array to JSON as one object by calling JSON.stringify () on the array, which results in a single JSON string. To convert back to an array from JSON, you'd call JSON.parse () on the string, leaving you with the original array. To convert each item in an array into JSON format, then you'll want to loop over the array. If you want to convert an object or array which contains a function, the JSON.stringify () function will remove the function. So you have to convert the function into a string before converting the object or array into JSON, and at the receiver, you can restore it to a function. How to Convert JSON to Array Objects Created JSON object and Empty Array Object using a for…in loop, Iterated each element of the JSON object. Add each element to Array using the push method
JSON is a commonly used data transfer format for representing objects in javascript. We use JSON format as a standard format in most client-server communications for transferring data. The JSON notation is easy to use and interpret as it is a human-readable format of a Javascript object. It is easy to convert a javascript object to a JSON format. Convert JSON file to Array using jQuery.getJSON () Method Now, let̢۪s see how you can accomplish the same task, that is, converting data in JSON file to an array, using jQuery. The jQuery.getJSON () method is ideal for this kind of task. That̢۪s what I am using here in my second example. The below approaches can be followed to solve the problem. Approach 1: In this approach, we create an empty object and use the Array.forEach () method to iterate over the array. On every iteration, we insert the first item of the child array into the object as a key and the second item as the value. Then it returns the object after the iterations.
JSON (JavaScript Object Notation) is a lightweight data-interchange format. As its name suggests, JSON is derived from the JavaScript programming language, but it's available for use by many languages including Python, Ruby, PHP, and Java and hence, it can be said as language-independent. - How to convert JavaScript Array to JSON. how can I convert an array to object where the array is [0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0] and ... Code language: JavaScript (javascript) The JSON.stringify () method converts a JavaScript object, array, or value to a JSON string that can be sent over the wire using the Fetch API (or another communication library). Weird Answer - Array to JSON with indexes as keys
Use the JavaScript function JSON.stringify () to convert it into a string. const myJSON = JSON.stringify(obj); The result will be a string following the JSON notation. myJSON is now a string, and ready to be sent to a server: Example. const obj = {name: "John", age: 30, city: "New York"}; const myJSON = JSON.stringify(obj); When converting an object to an array, we'll use the.entries () method from the Object class. This will convert our object to an array of arrays. Each nested array is a two-value list where the first item is the key and the second item is the value.
How To Iterate Through Jsonarray In Javascript Crunchify
Convert Json String To Data Array Using Javascript Or C
Convert And Loop Through Json With Php And Javascript Arrays
Json Handling With Php How To Encode Write Parse Decode
How To Parse Custom Json Data Using Excel The Excel Club
Java67 3 Ways To Convert String To Json Object In Java Examples
Converting Json Object Into Javascript Php Array Pakainfo
Jquery Convert Json String To Array Sitepoint
Convert Json Array String To Json Object Javascript
Golang How To Convert Json To Map In Go
Convert Array To Json Object Javascript Tuts Make
How To Convert Mongodb Response Array From Javascript Object
How To Parse Json In Sql Server
How To Convert Json String To Json Object In Javascript
How To Get Array From Json Object Stack Overflow
Solved Converting Json Object To Array Power Platform
Json Structures Studio Pro 9 Guide Mendix Documentation
Javascript Convert An Array To Json Geeksforgeeks
Convert Json To Swift C Typescript Objective C Go Java
How To Import Export Json Data Using Sql Server 2016
Java67 How To Parse Json To From Java Object Using Jackson
Convert Json Array Into Editable Table Jsoneditor Js Free
Convert Json Data Dynamically To Html Table Using Javascript
Convert Java Object To Json String Using Jackson Api
How To Convert Json Array To Arraylist In Java Javatpoint
How To Convert Json Data To A Html Table Using Javascript
Working With Json Data In Python Python Guides
3 Ways To Convert Datatable To Json String In Asp Net C
Java67 3 Ways To Ignore Null Fields While Converting Java
Convert Json Object To Lowercase Code Example
0 Response to "32 How To Convert Json Object To Array In Javascript"
Post a Comment