21 Javascript Regular Expression Replace
Jan 18, 2015 - What is the fastest way to replace all instances of a string/character in a string in JavaScript? A while, a for-loop, a regular expression? Sep 15, 2014 - We're going to cover how to use the regular expression driven replace(..) with JavaScript string values. All string values have a replace(..) method available to them. This method allows you to pass a regular expression (or a string that will be interpreted as the pattern for a dynamically-created ...
Regexr Learn Build Amp Test Regex
JavaScript's Regular Expression Flavor In JavaScript source code, a regular expression is written in the form of /pattern/modifiers where "pattern" is the regular expression itself, and "modifiers" are a series of characters indicating various options. The "modifiers" part is optional. This syntax is borrowed from Perl.
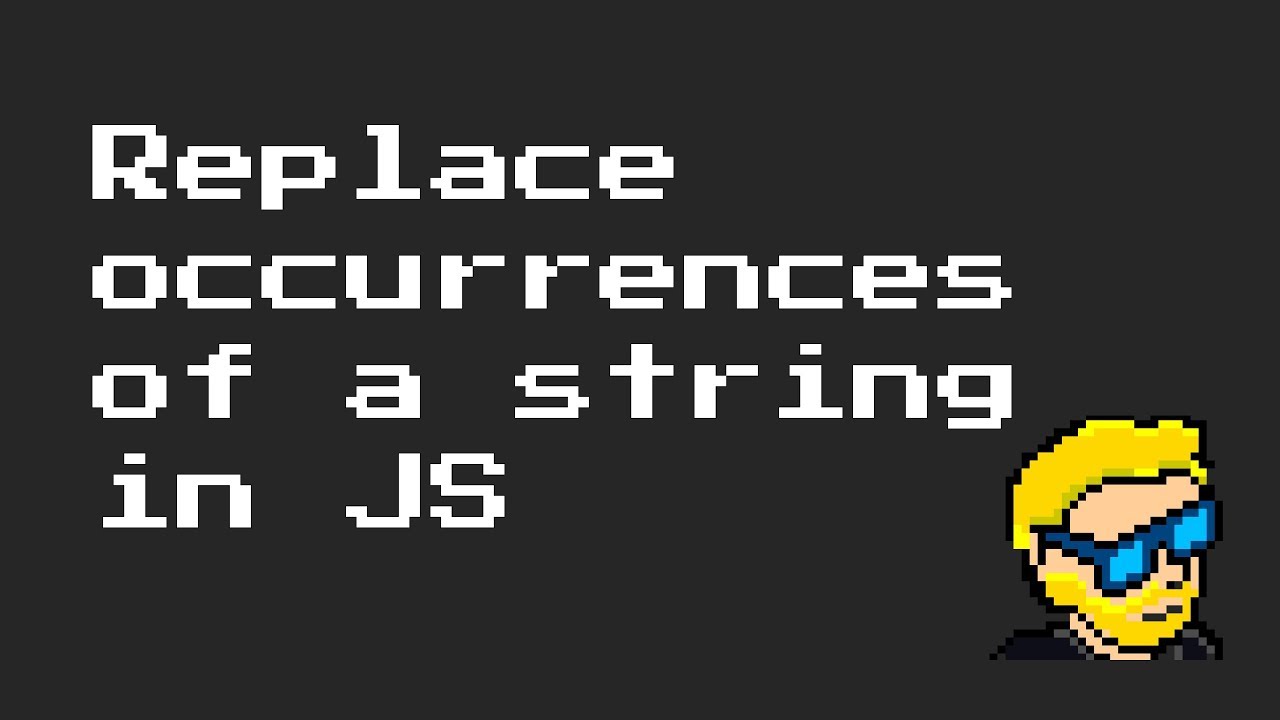
Javascript regular expression replace. 1 week ago - If using the RegExp constructor ... in the regular expression, you need to escape it at the string literal level. /a\*b/ and new RegExp("a\\*b") create the same expression, which searches for "a" followed by a literal "*" followed by "b". If escape strings are not already part of your pattern you can add them using String.replace... In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor. In JavaScript, regular expressions are objects. JavaScript provides the built-in RegExp type that allows you to work with regular expressions effectively. Regular expressions are useful for searching and replacing strings that match a pattern. They have many useful applications.
15/6/2013 · Javascript string replace and regular expression / The replace method in JavaScript is pretty much the same as in the other languages. Replace part/s of a string with something else. However, there are some tricky moments and I wanted to write this article, because I met this problem several times in the past. You need to double escape any RegExp characters (once for the slash in the string and once for the regexp): "$TESTONE $TESTONE".replace (new RegExp ("\\$TESTONE","gm"),"foo") Otherwise, it looks for the end of the line and 'TESTONE' (which it never finds). Personally, I'm not a big fan of building regexp's using strings for this reason. Regular expressions, abbreviated as regex, or sometimes regexp, are one of those concepts that you probably know is really powerful and useful. But they can be daunting, especially for beginning programmers. It doesn't have to be this way. JavaScript includes several helpful methods that make using regular expressions much more manageable.
Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The.replace method is used on strings in JavaScript to replace parts of string with characters. The rule of thumb is that simple regular expressions are simple to read and write, while complex regular expressions can quickly turn into a mess if you don't deeply grasp the basics. How does a Regular Expression look like. In JavaScript, a regular expression is an object, which can be defined in two ways. Javascript Web Development Object Oriented Programming Let's say the following are our strings with commas − "My Favorite subject is," "My Favorite subject is, and teacher name is Adam Smith" "My Favorite subject is, and got the marks 89" To replace commas, use replace and in that, use Regular Expression.
Nov 19, 2020 - We all know the replace() function for JavaScript Strings and that it is possible to do really fancy things by using regular expressions to replace a (sub)string. What I didn't know was that there are special $ references you can use in the replace() function. RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details. Validate patterns with suites of Tests. Save & share expressions with others. The JavaScript String replace () method returns a new string with a substring (substr) replaced by a new one (newSubstr). Note that the replace () method doesn't change the original string. It returns a new string. JavaScript String replace () examples
If the first argument is a string, it replaces all occurences of the string, while replace replaces only the first occurence. If the first argument is a regular expression without the g flag, there'll be an error. With g flag, it works the same as replace. The main use case for replaceAll is replacing all occurences of a string. A regular expression is a sequence of characters that forms a search pattern. The search pattern can be used for text search and text to replace operations. A regular expression can be a single character or a more complicated pattern. Regular expressions can be used to perform all types of text search and text replace operations. Mostly a regular expression is used to validate a string against a pattern or extract a specific substring out of a string or check if a string contains a certain substring, and for checking this, we use the RegExp.test() function.. JavaScript String test(), replace() and match() Functions:. The test() function is used along with a regular expression pattern providing a string as an argument.
a.replace (/ (f)/, "$1".toUpperCase ()) In this example you pass a string to the replace function. Since you are using the special replace syntax ($N grabs the Nth capture) you are simply giving the same value. JavaScript's String type has 4 built-in methods: search(), replace(), split(), and match(), all of which accept regular expressions as an argument to help them perform their respective operations. When searching in a given string, you can specify the pattern you're looking for. Arnd Issler pointed out, that you can not talk about backreferences in regular expression without mentioning the references when using String.prototype.replace.So, here we go. 😊. Replacement references for capture groups. You can reference included capture groups using $1, $2, etc. in the replacement pattern.. MDN provides a good example to swap words using references.
This online Regex Replace tool helps you to replace string using regular expression (Javascript RegExp). Coding.Tools. Regex Replace Online Tool. Regular Expression. ... Replace regular expression matches in a string using Python: import re regex_replacer = re pile("test", re.IGNORECASE) test_string = "This is a Test string." ... Regular expressions are patterns that provide a powerful way to search and replace in text. In JavaScript, they are available via the RegExp object, as well as being integrated in methods of strings. This can be done by wrapping them in parentheses and including their groups in the replacement string ($1, $2). Groups that are not matched will be replaced by nothing. ... Write an expression that matches only JavaScript-style numbers. It must support an optional minus or plus sign in front ...
Aug 26, 2020 - It can not only replace plain strings, but patterns too. These patterns are known as Regular Expressions. Regular expressions exist in JavaScript and most other programming languages. Regex (for short) are a very powerful tool to help you find simple as well as complex search patterns. Jul 20, 2021 - The [@@replace]() method replaces some or all matches of a this pattern in a string by a replacement, and returns the result of the replacement as a new string. The replacement can be a string or a function to be called for each match. JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex
Sep 21, 2017 - Example of how to use the JavaScript RegExp Object. Test your regular expressions online in your web browser. Regular expressions are built-in tools like grep, sed, text editors like vi, emacs, programming languages like JavaScript, Perl, and Python. JavaScript regular expression. In JavaScript, we build regular expressions either with slashes // or RegExp object. A pattern is a regular expression that defines the text we are searching for or ... In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String
In JavaScript, a regular expression is simply a type of object that is used to match character combinations in strings. Creating Regex in JS. There are two ways to create a regular expression: Regular Expression Literal — This method uses slashes ( / ) to enclose the Regex pattern: var regexLiteral = /cat/; In JavaScript, replace () is a string method that is used to replace occurrences of a specified string or regular expression with a replacement string. Because the replace () method is a method of the String object, it must be invoked through a particular instance of the String class. Definition and Usage The replace () method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Note: If you are replacing a value (and not a regular expression), only the first instance of the value will be replaced.
how to use JavaScript regular expression to remove special characters or regular expression to escape special characters in JavaScript or regex to replace sp... The replace () method returns a new string with some or all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. If pattern is a string, only the first occurrence will be replaced. The original string is left unchanged. The string method string.replace (regExpSearch, replaceWith) searches and replaces the occurrences of the regular expression regExpSearch with replaceWith string. To make the method replace () replace all occurrences of the pattern you have to enable the global flag on the regular expression:
Aug 02, 2019 - While the built-in functions in most languages are usually sufficient to perform search and replace operations on strings, more complex operations — such as validating text inputs — often require the use of regular expressions. Regular expressions have been part of the JavaScript language ...
Using Regular Expression In Javascript Codeproject
Check If String Matches Regex Typescript Code Example
Regular Expressions And Perl Ppt Download
Javascript Replace Forward Slash In String Parallelcodes
How To Find Patterns Of Text In Javascript By Anh Dang
How To Replace All Occurrences Of A String In Javascript
Java Email Regex Examples Mkyong Com
Javascript String Replace How To Replace String In Javascript
Javascript Replace A Step By Step Guide Career Karma
Find And Replace Using Regular Expression In Visual Studio
Replace Data With Regular Expression My Expression Regular
Javascript Replace Html Tags Replace And Regex Example
Regular Expressions In Java Tutorial
Timetalks Understanding Javascript S Match Exec And
How To Globally Replace A Forward Slash In A Javascript
Everything You Need To Know About Regular Expressions By
Javascript String Replace Example With Regex
Everything You Need To Know About Regular Expressions By
Data Sheet Python Regex Cheatsheet Free Download Activestate
Javascript Regular Expression How To Create Amp Write Them In
0 Response to "21 Javascript Regular Expression Replace"
Post a Comment