23 Adapter Design Pattern Javascript
"A design pattern names, abstracts, and identifies the key aspects of a common design structure that make it useful for creating a reusable object-oriented design. The design pattern identifies the participating classes and their instances, their roles and collaborations, and the distribution of responsibilities. In software engineering, the adapter pattern is a software design pattern that allows the interface of an existing class to be used as another interface. It is often used to make existing classes...
Adapter Design Pattern In Automated Testing
Every design pattern consists of many properties or components when it is defined like pattern name, description, context outline, problem statement, solution, design, implementation, illustrations, examples, co-requisites, relations, known usage, and discussions. Types of JavaScript Design Pattern: Below are the types of design pattern. 1.
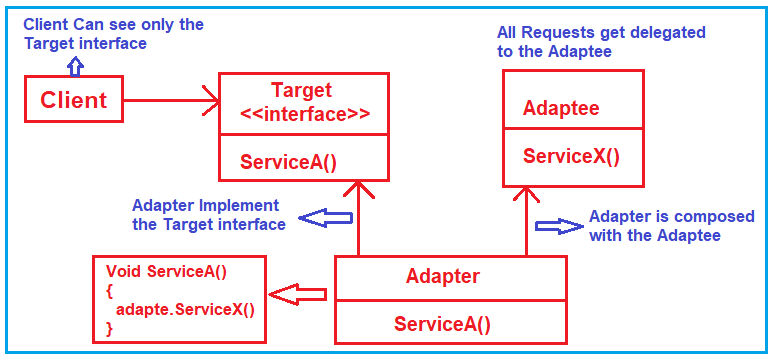
Adapter design pattern javascript. Adapter in TypeScript Adapter is a structural design pattern, which allows incompatible objects to collaborate. The Adapter acts as a wrapper between two objects. It catches calls for one object and transforms them to format and interface recognizable by the second object. Jul 03, 2018 - In the last post we saw the creational patterns. If you missed out on that here is the link : Creational Patterns Intent: Convert the interface of a class into something different that another client… Adapter design pattern is a structural design pattern. It helps one interface of an object or class to be translated into another interface to make it compatible with the system. It has three participants, adapter, adaptee and the target. The target and the adaptee initially are incompatible in this case so we introduce adapter which is able to ...
Adapter is a structural design pattern that allows objects with incompatible interfaces to collaborate. Problem. Imagine that you're creating a stock market monitoring app. The app downloads the stock data from multiple sources in XML format and then displays nice-looking charts and diagrams for the user. JavaScript Design Patterns: Adapter 2011/12/26. Another post, another JavaScript design pattern. This time we're featuring the Adapter pattern. If you'd like to view the previous posts from this series, you can check out the links at the bottom of this page. This mean, green, fighting machine can transform interfaces. Jul 17, 2019 - The Adapter class CalcAdapterwraps the NewCalculator to add the operation method to the public-facing API while using its own addition and subtraction implementation under the hood. ... This is a structural design pattern that composes objects into tree-like structures to represent whole-part ...
The adapter pattern converts the interface of a class to another expected interface, making two incompatible interfaces work together. With the adapter pattern, you might need to show the data from a 3rd party library with the bar chart representation, but the data formats of the 3rd party library API and the display bar chart are different. • Adapter [7] to translate messages from the objects used in the JavaScript application to the format used for communication with the server, and vice versa Design Patterns Specific to JavaScript • JavaScript Module Pattern [8] to create a distinct scope and namespace for Oct 16, 2015 - The adapter design pattern is very useful in JavaScript when it comes to data fetching and persistence. The travel adapter is the most common comparison for…
The Adapter Design Pattern in JavaScript. Contribute to gergob/jsAdapter development by creating an account on GitHub. Nov 25, 2016 - Learn how and when to use the Adapter Design Pattern through JavaScript code example for node.js Oct 04, 2019 - Understanding when to use these design patterns and why you should use them helps you gain their benefits and improve the quality of your code.
Adapter Design Pattern The adaptor design pattern is mainly used for different designs of objects to work together in a single interface. Consider that we are building a library that accepts structures and formats in JSON. 1.Adapter design pattern The adapter is a structural design pattern that allows objects with incompatible interfaces to collaborate just like HDMI <-> DVI adapter The problem : Design patterns are solutions to recurring problems in software application development. ... A classic example of an adapter pattern will be a differently shaped power socket. Sometimes, the socket and device plug doesn't fit. ... Singleton and Builder design patterns do not go well in javascript.
This lesson discusses the adapter pattern in detail using a coding example. Jun 05, 2020 - This is the first article in a series I am planning to make about Design Patterns in JavaScript. This time we are going to dive into Adapter pattern. Which is one of the simplest but really powerful… Prototype Design Pattern. The prototype design pattern lets us create clones of the existing objects. This is similar to the prototypal inheritance in JavaScript. All of the properties and methods of an object can be made available on any other object by leveraging the power of the __proto__ property.
Một số Design Patterns có thể sử dụng trong Javascript - Phần 1. Trong bài viết này, mình sẽ chỉ cho các bạn một số design pattern được sử dụng trong javascript, để giúp các bạn viết code tốt hơn, dễ đọc hơn, dễ bảo trì hơn. Để đọc và hiểu bài viết này, thì các bạn ... In software engineering, the adapter pattern is a software design pattern that allows the interface of an existing class to be used as another interface. It is often used to make existing classes work with others without modifying their source code. The Adapter design pattern converts a class interface into another one the client expects. This pattern lets classes work together otherwise they couldn't because their interfaces are incompatible. The essence of the pattern is transferring an interface (object's properties and methods) to another.
Mar 22, 2019 - There are 23 classic design patterns, ... book, `Design Patterns: Elements of Reusable Object-Oriented Software`. These patterns provide solutions to particular problems, often repeated in the software development. In this article, I am going to describe the how the **Adapter Pattern; and** how and when it should be applied. Tagged with javascript, typescript, ... The adapter pattern introduces an intermediary piece of code that makes two parts of a system compatible with one another. It also injects an element of lose coupling by keeping the two pieces of code separate. It means that you can write your code however you want without the need to take the other piece of code into consideration. An Adapter Pattern says that just "converts the interface of a class into another interface that a client wants". In other words, to provide the interface according to client requirement while using the services of a class with a different interface. The Adapter Pattern is also known as Wrapper. Advantage of Adapter Pattern
Oct 06, 2017 - Definition The Adapter design pattern is a strtuctural design pattern used to make two unrelated and incompatible interfaces or classes work together that could not be achieved otherwise due to mismatched interfaces. It wraps an existing class with a new interface, which is why it is also known ... The Adapter Pattern The Adapter pattern translates an interface for an object or class into an interface compatible with a specific system. Adapters basically allow objects or classes to function … - Selection from Learning JavaScript Design Patterns [Book] "In software engineering, the adapter pattern is a software design pattern that allows the interface of an existing class to be used from another interface. It is often used to make existing classes work with others without modifying their source code." A great example of the adapter pattern in JavaScript is Ember Data.
The code contains also another fascinating design pattern - adapter for Facebook API. Let's take a closer look at this bad boy. The idea behind this adapter is to abstract the implementation of the performed operation (communication with Facebook API) and isolate the rest of the code from details. Here is what the adapter looks like: Before we dive into Adapter pattern example in JavaScript, let's define what it is at all. As you probably know from a real domestic world, adapter is used to connect two or more things which can't be connected with original sockets. Like for example UK plug can't be used with European socket, so you need an adapter. This is the second article in JavaScript Design Patterns series, where I explain Design Patterns with simple words. There is a ton of information out there on the internet, which is incredibly confusing, dry and boring. My goal is to explain complex things in a simple and enjoyable manner.
The adapter pattern convert the interface of a class into another interface clients expect. Adapter lets classes work together that couldn't otherwise because of incompatible interfaces. Jul 26, 2019 - Another fascinating design pattern - adapter for Facebook API. Let's take a closer look at this bad boy. JavaScript Adapter Design Pattern with Example Code.
The Adapter Design Pattern adapts an interface to look like a different interface. When we expect a particular interface but have an object that implements a certain other interface, we can use the adapter design pattern to make the object appear as if it is implementing the first interface. Thus, we can use the object where the first interface ... JavaScript Adapter The Adapter pattern translates one interface (an object's properties and methods) to another. Adapters allows programming components to work together that otherwise wouldn&lstqup;t because of mismatched interfaces. The Adapter pattern is also referred to as the Wrapper Pattern. Oct 16, 2015 - The adapter design pattern is very useful in JavaScript when it comes to data fetching and persistence. The travel adapter is the most common comparison for this pattern. If you have a three-pronged electrical plug, it won’t fit in a two-prong wall outlet. Instead, you need to use a travel ...
Adapter Pattern An Adapter pattern acts as a connector between two incompatible interfaces that otherwise cannot be connected directly. An Adapter wraps an existing class with a new interface so that it becomes compatible with the client's interface. The Adapter Design Pattern is a useful design pattern for when you have two things you'd like to use together, but the interfaces don't match. This Javascrip...
Adapter Design Pattern In Java And Python En Proft Me
Adapter Design Pattern C Codeproject
Javascript Design Patterns The Adapter Pattern By Arthur
Adapter Design Pattern In Javascript
Javascript Adapter Pattern Explained
Design Patterns In Javascript The Adapter Design Pattern In Javascript
Design Patterns Explained Adapter Pattern With Code
Adapter Design Pattern Structural Patterns Dinesh On Java
Javascript Design Patterns The Adapter Pattern By Arthur
Design Patterns Adapter Dev Community
A Comprehensive Guide To Javascript Design Patterns
Design Patterns Adapter Dev Community
Design Patterns Adapter Dev Community
Javascript Adapter Design Pattern Dofactory
Adapter Pattern In Javascript Jsmanifest
Adapter Design Pattern In C With Examples Dot Net Tutorials
Adapter Pattern In Java Java Design Pattern
Javascript Design Patterns Javatpoint
0 Response to "23 Adapter Design Pattern Javascript"
Post a Comment