34 How To Do Asynchronous Calls In Javascript
Javascript make asynchronous calls inside a loop and pause /block loop between calls. Returning a response from an asynchronous call. There are many ways to return the response from an async call in JavaScript, callbacks, and promises. Let's say you are making an asynchronous call and you want the result of the call to be from the function, this can be done using async/await, let's explain this further in the code below:
Managing Asychronous Calls Aws Sdk For Javascript
Nov 07, 2018 - If your code contains blocking code it is better to make it an async function. By doing this you are making sure that somebody else can use your function asynchronously. By making async functions out of blocking code, you are enabling the user who will call your function to decide on the level ...
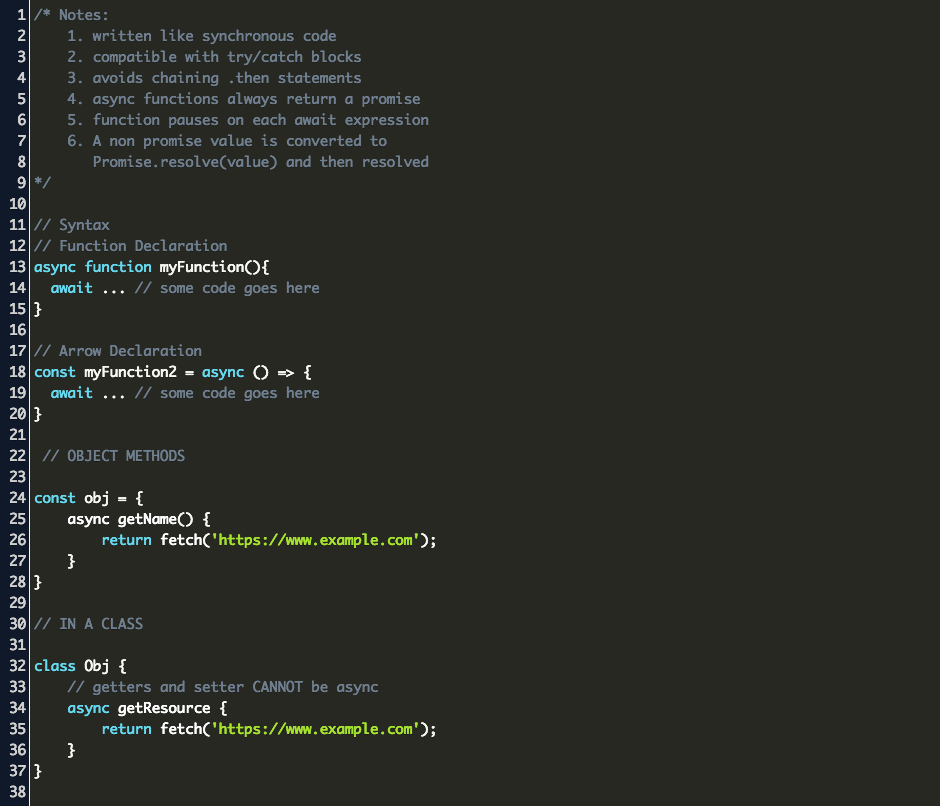
How to do asynchronous calls in javascript. Mar 16, 2021 - If it’s an error, the exception is generated — same as if throw error were called at that very place. Otherwise, it returns the result. Together they provide a great framework to write asynchronous code that is easy to both read and write. Feb 26, 2018 - Discover the modern approach to asynchronous functions in JavaScript. JavaScript evolved in a very short time from callbacks to Promises, and since ES2017 asynchronous JavaScript is even simpler with the async/await syntax Solution 1: Making Synchronous AJAX Calls The first solution has already been mentioned above. You can write asynchronous AJAX calls so that it waits for the response before moving on to the next statements. If you are using jQuery, you can easily do this by setting the async option to false.
The async and await keywords are a great addition to Javascript. They make it easier to read (and write) code that runs asynchronously. But they can still be confusing. Asynchronous programming is hard. Anyone who tells you differently is either lying or selling something. But there are some simple patterns you can learn that will make life easier. In this article, we will discuss how to deal with asynchronous calls in all of the above-mentioned ways. We will use the request module provided in Node.js to request JSON data from the website DOG CEO API which returns an URL link for a randomized dog picture in JSON format. Using a Callback function: const request = require ('request'); Jul 07, 2021 - Now that we have a basic idea about the call stack, and how the synchronous JavaScript works, let’s get back to the asynchronous JavaScript. ... Let’s suppose we are doing an image processing or a network request in a synchronous way. For example:
Javascript is a single threaded language. This means it has one call stack and one memory heap. As expected, it executes code in order and must finish executing a piece code before moving onto the next. It's synchronous, but at times that can be harmful. For example, if a function takes awhile to execute or has to wait on something, it freezes ... Any Async function returns a Promise implicitly, and the resolved value of the Promise will be whatever returns from your function. Our function has an async keyword on its definition (which says... Mar 04, 2019 - The underlying HTTP(s) request is an asynchronous operation and does not block the execution of the rest of the JavaScript code. The callback function is put on a sort of queue called the “event loop” until it will be executed with a result from the request.
This is a typical asynchronous programming challenge, and how you choose to deal with asynchronous calls will, in large part, make or break your app, and by extension potentially your entire startup. Synchronizing asynchronous tasks in JavaScript was a serious issue for a very long time. JavaScript, itself, has basically no asynchronous nature. It's frequently used in environmentsthat do, like the browser, or NodeJS. - T.J. CrowderJun 8 '16 at 16:38 I'd suggest reading up on Promises. Ajax is a technology that allows developers to make asynchronous HTTP requests without the need for a full page refresh. To make the process less cumbersome than it would be in pure JavaScript ...
JavaScript async/await step by step Enter async / await . These functions let you work with promises without all of the then and catch syntax and makes your asynchronous code readable. Sep 25, 2019 - Then when the time is right a callback will spring these asynchronous requests into action. ... This will actually log “1 3 2”, since the “2” is on a setTimeout which will only execute, by this example, after two seconds. Your application does not hang waiting for the two seconds to finish. JavaScript environments typically implement this style of programming using callbacks, functions that are called when the actions complete. An event loop schedules such callbacks to be called when appropriate, one after the other, so that their execution does not overlap. Programming asynchronously ...
To fix the problematic fetch () example and make the three console.log () statements appear in the desired order, you could make the third console.log () statement run async as well. This can be done by moving it inside another.then () block chained onto the end of the second one, or by moving it inside the second then () block. But now you hopefully have a grasp on how JavaScript works with asynchronous code in the browser, and a stronger grasp over both promises and async / await. If you enjoyed this article, you might also enjoy my youtube channel . myCallback (sum); } myCalculator (5, 5, myDisplayer); Try it Yourself ». In the example above, myDisplayer is the name of a function. It is passed to myCalculator () as an argument. In the real world, callbacks are most often used with asynchronous functions. A typical example is JavaScript …
Asynchronous calls refer to calls that are moved off of JavaScript's execution stack and do some work elsewhere. These are calls to an API. In Node's case, they are calls to a C++ API in Node. Once the work is done, there is a function put in the event queue. Then when JavaScript's execution stack is empty, the event loop pulls the ... 8/2/2021 · The verifyUser method should be defined using an async function as only inside these functions; it is possible to await promises. This small change enables you to await promises without making any other changes to the other existing methods. Async functions are the subsequent logical step in the evolution of asynchronous programming in JavaScript. AJAX is a misleading name. AJAX applications might use XML to transport data, but it is equally common to transport data as plain text or JSON text. AJAX allows web pages to be updated asynchronously by exchanging data with a web server behind the scenes. This means that it is possible to update parts of a web page, without reloading the whole ...
At the point when the "Async" call is made, the JavaScript execution thread is free to perform any additional client-side processing (although none are shown in the diagram). When the "Async" method returns, the callback resumes execution on the thread, and the add-in can the access data, do something with it, and display the result. Jan 31, 2019 - However, when dealing with asynchronous code (e.g. reading from the file system, accessing external APIs) we need to handle it in a special way to be able to deal with the results which this code is delivering. JavaScript offers various possibilities of doing so. With callbacks, Promises and ... In order to mimic something like an asynchronous call we can use the 'setTimeout' method. This takes two arguments; the first is a function which will be a callback function, and the second is the...
The JavaScript code triggering such an operation registers a callback, which is invoked with the result once the operation is finished. The invocation is handled via the task queue. This style of delivering a result is called asynchronous because the caller doesn't wait until the results are ready. JavaScript Async Functions. Async and await are built on promises. The keyword "async" accompanies the function, indicating that it returns a promise. Within this function, the await keyword is applied to the promise being returned. The await keyword ensures that the function waits for the promise to resolve. 16/11/2020 · The first and oldest way to write asynchronous JavaScript code is by using callbacks. A callback is an asynchronous function that is passed as argument to other function when you call it. When that function you called finishes its execution it “calls back” the callback function. Until this happens, the callback function is not invoked.
async function getFile () {. let myPromise = new Promise (function(myResolve, myReject) {. let req = new XMLHttpRequest (); req.open('GET', "mycar.html"); req.onload = function() {. if (req.status == 200) {myResolve (req.response);} else {myResolve ("File not Found");} An async function is a function declared with the async keyword, and the await keyword is permitted within them. The async and await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains. Async functions may also be defined as expressions. More complex asynchronous JavaScript operations, such as looping through asynchronous calls, is an even bigger challenge. In fact, there is no trivial way of doing this with callbacks. This is why JavaScript Promise libraries like Bluebird and Q got so much traction.
2/2/2012 · But here's how you can do this:./calling-file.js. var createClient = require('sync-rpc'); var mySynchronousCall = createClient(require.resolve('./my-asynchronous-call'), 'init data'); var param1 = 'test data' var data = mySynchronousCall(param1); console.log(data); // prints: received "test data" after "init data" ./my-asynchronous-call.js The Practical Client. Simple Asynchronous Processing for Web Service Calls. You don't need to write ugly code to synchronize your AJAX calls. Instead, you can leverage await/async and the JQueryXHR object to simplify the code around your AJAX calls while still getting the benefits of concurrent processing. Jun 11, 2019 - Promises were introduced to solve the famous callback dilemma, but they introduced complexity on their own, and syntax complexity. ... Since ES2017 asynchronous JavaScript is even simpler with the async/await syntax. ... In a more comfortable fashion, they reduce the boilerplate around promises, and the “don...
Introduction. XMLHTTPRequest is an object which is used to perform the Asynchronous HTTP call using JavaScript. Usually, we call it an AJAX call. It is a browser object which is supported by all modern browsers and basically, it can handle any type of data which is used to communicate between the client and server using HTTP. In "How do I return the response from an asynchronous call in Javascript?" I answer a FAQ in Javascript. Before Async/await functions, JavaScript code that relied on lots of asynchronous events (for example: code that made lots of calls to APIs) would end up in what some called “callback hell” - A chain of functions and callbacks that was very difficult to read and understand.
1 2 3 When an asynchronous Web API is used, the rules become more complicated. A built-in API that you can test this with is setTimeout, which sets a timer and performs an action after a specified amount of time.setTimeout needs to be asynchronous, otherwise the entire browser would remain frozen during the waiting, which would result in a poor user experience. Mar 12, 2020 - This means you can pass functions around as arguments, and return functions from functions. Handy. When JavaScript was first released, callbacks were the standard approach for handling asynchronous operations. Callbacks are conceptually simple — you pass a function as a parameter that should ... This is why understanding JavaScript callbacks is essential to understanding asynchronous programming in JavaScript. In the client browser callbacks enable JavaScript code to make a call that might take a long time to respond, like a web service API call, without "freezing" the web page while it waits for a response.
May 30, 2021 - More recent additions to the JavaScript language are async functions and the await keyword, added in ECMAScript 2017. These features basically act as syntactic sugar on top of promises, making asynchronous code easier to write and to read afterwards. They make async code look more like old-school ... Asynchronous JavaScript: Asynchronous code allows the program to be executed immediately where the synchronous code will block further execution of the remaining code until it finishes the current one. This may not look like a big problem but when you see it in a bigger picture you realize that it may lead to delaying the User Interface. Apr 22, 2018 - If you don’t know what Promises are, you should read above part first, You can’t understand Async/Await in JavaScript until you understand Promises. ... The newest way to write asynchronous code in JavaScript. It is non blocking (just like promises and callbacks).
When you try to do this in asynchronous code, it's easy to run into callback hell, a common problem where you have callback functions deeply nested inside of each other. Node.js code and front-end applications with lots of AJAX calls are particularly susceptible to end-up looking something like this: Oct 09, 2017 - Synchronous operations in JavaScript entails having each step of an operation waits for the previous step to execute completely. This means no matter how long a previous process takes, subsquent process won't kick off until the former is completed. Asynchronous operations, on the other hand, defe
How To Use Async Await To Write Better Javascript Code
How To Return Ajax Response From Asynchronous Javascript Call
Javascript Goes Asynchronous And It S Awesome Sitepoint
How To Use Fetch With Async Await
Async Await In Node Js How To Master It Risingstack
Asynchronous Programming In Javascript
How To Use Async Await To Properly Link Multiple Functions In
Asynchronous Adventures In Javascript Async Await By
Deeply Understanding Javascript Async And Await With Examples
Callback Vs Promises Vs Async Await Loginradius Engineering
How Typescript S Async Await Makes Your Life Easier Time
Calling Bind On An Async Function Partially Works Stack
Asynchronous Programming Eloquent Javascript
Why Is My Variable Unaltered After I Modify It Inside Of A
How To Run Async Javascript Functions In Sequence Or Parallel
Asynchronous Javascript Async Await Tutorial Toptal
Using Async Await With A Foreach Loop Stack Overflow
Async Await Vs Promises A Guide And Cheat Sheet By Kait
What Every Programmer Should Know About Synchronous Vs
How To Learn Javascript Promises And Async Await In 20 Minutes
Asynchronous Javascript With Promises Amp Async Await In
Cleaning Up Asynchronous Javascript With Async Await Keywords
Asynchronous Javascript From Callback Hell To Async And
How To Force Javascript To Await Async Call Code Example
What Is The Difference Between Microtask Queue And Callback
Async Await For Beginners Understanding Asynchronous Code In
Node Js Async Await Tutorial With Asynchronous Javascript
Callback Hell Promises And Async Await
How To Run Async Javascript Functions In Sequence Or Parallel
Asynchronous Javascript Using Promises With Rest Apis In Node Js
Aborting A Signal How To Cancel An Asynchronous Task In
Understanding Async Await In Javascript By Gemma Stiles
0 Response to "34 How To Do Asynchronous Calls In Javascript"
Post a Comment