22 Javascript Websocket Send Json
In this tutorial we will check how to setup a HTTP web server on the ESP32, which will have a websocket endpoint and will serve a HTML page. The HTML page will run a simple JavaScript application that will connect to the server using websockets and periodically receive simulated temperature measurements from the server. StreamExplorer pushing findings as JSON messages to a WebSocket channel for live HTML Dashboard updates Leveraging RESTful Services from Java applications using Jersey (Introduction) JavaOne 2014: Roadmaps for the near future of Java Make HTTP POST request from Java SE - no frills, no libraries, just plain Java Reflections after Oracle OpenWorld 2015 - Integration
Publish Data To Websocket Server In Json Format Simulink
Nov 21, 2020 - Unlike HTTP servers, WebSockets ones don’t have any routes by default because it is just not needed. In this protocol, you just use a string to send and receive information (a good practice is to send a JSON object serialized to a string). That’s why the configuration is so simple, still, ...
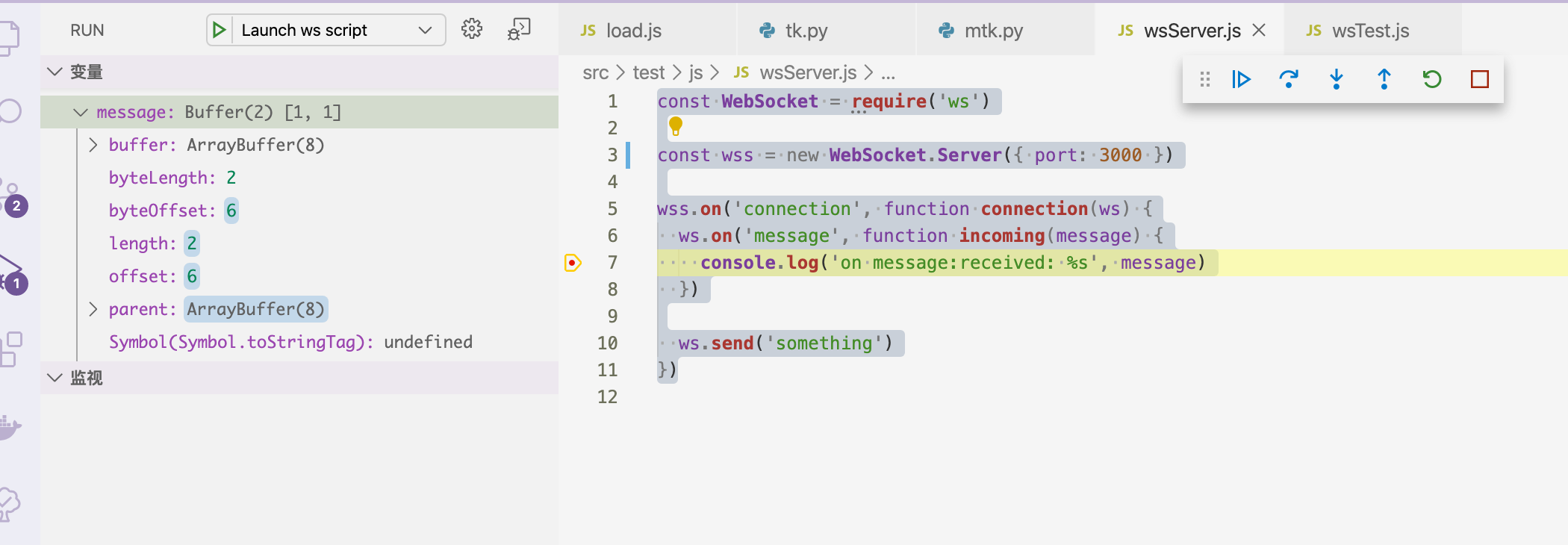
Javascript websocket send json. WebSockets support sending and receiving: strings, typed arrays (ArrayBuffer) and Blobs. Javascript objects must be serialized to one of the above types before sending. To send an object as a string you can use the builtin JSON support: ws.send(JSON.stringify(object)); ASP.NET Core SignalR supports two protocols for encoding messages: JSON and MessagePack. Each protocol has serialization configuration options. JSON serialization can be configured on the server using the AddJsonProtocol extension method, which can be added after AddSignalR in your Startup.ConfigureServices method. You can connect to the Qlik Engine JSON API using the following WebSocket URIs:. wss://server.domain :4747/app/ (connect directly to the Qlik Engine JSON API; requires certificates) wss://server.domain [/virtual proxy]/app/ (connect via the Qlik Sense Proxy Service) These URIs will result in a WebSocket that is opened with global context (that is, you will be able to call only methods ...
Writing to a WebSocket can be done just like writing to any file descriptor. In this case, we use writeall() so that we can send the whole JSON object in one packet. Otherwise, the JSON object might be sent as several smaller packets, which doesn't play nice with JavaScript on the browser side. 4. Using JavaScript to establish a WebSocket ... WebSockets - Send & Receive Messages. The Message event takes place usually when the server sends some data. Messages sent by the server to the client can include plain text messages, binary data, or images. Whenever data is sent, the onmessage function is fired. This event acts as a client's ear to the server. The connection in using Websocket. For the moment, I can send a String from the server to the web page. I want to send a JSON object to the web code. how to this ? Is it better to convert the JSOn in a string in my C# server and parse the String to JSON in the web page or is there a way to do it without using the string ?
JavaScript Object Notation (JSON). It is a lightweight data transferring format. It is very easy to understand by human as well as machine. It is commonly used to send data from or to server. Nowadays it is widely used in API integration because of its advantages and simplicity. Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! Returns. WebSocketSubject<T>: Subject which allows to both send and receive messages via WebSocket connection. Description. Subject that communicates with a server via WebSocket. webSocket is a factory function that produces a WebSocketSubject, which can be used to make WebSocket connection with an arbitrary endpoint.webSocket accepts as an argument either a string with url of WebSocket ...
Mar 23, 2019 - The underlying method is called “send” in the WebSocket API, but I’ve chosen to call it “trigger” so we stay close to the idea of events. I like that because Javascript in the browser is all about events (“click”, “mouseover”, “submit”) and I really like the idea of treating ... Over in the client it needs to juggle both the websocket connection and a timer to send the JSON at intervals. Based on the ws-rs guide, I've made a struct that represents the established connection. The Handler trait lets me respond to messages and all the websocket lifecycle events by implementing the relevant functions. This is a continuation of my previous video. This time I show how to send communicate objects between an HTML5 client and a node.js server using websockets ...
Aug 19, 2017 - It is best to use Binary data only when JSON or XML is not an option due to performance reasons. ... Leading to data being written over the wrong section of buffer. WebSockets can mix and match binary data and text data. So I can have the best of both worlds. Infrequently I need to send complex ... wss.on('connection', function ... { ws.send(JSON.stringify({ type: 'server', method: req.method, headers: req.headers, body: msg }, null, '\t')); }); }); ... /** * @param {string} message */ send(message) { if (this._ws.readyState === WebSocket.OPEN) { protocolDebug('>', ... This tutorial details the use of WebSocket to remotely interact with an ESP32 that exposes a web application through an HTTP server. The WebSocket protocol opens a two-way communication channel between the client browser and the HTTP server run by the ESP32. With this API you can send messages to a server and receive its responses on an event-driven basis without having to go to the server for ...
The wsURI URI scheme is part of the WebSocket protocol and specifies the path to the endpoint for the application. Right-click the project node in the Projects window and choose New > Other. Select JavaScript File in the Web category of the New File wizard. Click Next. Type websocket for the JavaScript File Name. Apr 13, 2016 - PHP WebSockets. Another simple framework without documentation or complete demonstration. ... Whatever the framework used, the client-side code is the same as in the JavaScript example, but PHP side, it is necessary to convert a JSON string to object to access the content in key-value format. 2 Jan 2019 — How can I send json to client in websockets using js and print the value? javascript jquery websocket. I want to parse a json like this from the ...
Apr 29, 2016 - IIRC there is an alternative lib that is purpose built to be faster than JSON in JavaScript but I can't remember the name off the top of my head... ... Yea, but why bother. Sending, receiving, stringify and parse json over compressed HTTP steam isn't going to be much slower than any other way. wss.on('connection', function ... { ws.send(JSON.stringify({ type: 'server', method: req.method, headers: req.headers, body: msg }, null, '\t')); }); }); ... /** * @param {string} message */ send(message) { if (this._ws.readyState === WebSocket.OPEN) { protocolDebug('>', ... 28/2/2019 · Let's start with the lengths and copy the strings: let i = 0 buffer [i] = nameBuffer.length buffer.set (i += 1, nameBuffer) buffer [i += nameBuffer.length] = typeBuffer.length buffer.set (i += 1, typeBuffer) buffer [i += typeBuffer.length] = sizeLength. Then the file size must be written as the appropriate Int type:
5. Next, we implement a WebSocket connection logic. The market_price_app.js receives a user input for Refinitiv Real-Time Advanced Distribution Server IP Address and WebSocket port from the index.html web page, then market_price_app.js sends the WebSocket URL to ws_wokers.js file with the command 'connect' to establish a connection via postMessage() function. Sep 05, 2017 - Therefore every message is a simple JavaScript object encoded into JSON. ... Node.js itself doesn’t have support for WebSocket but there are already some plugins that implement WebSocket protocols. I’ve tried two of them: In today's video I'll be showing you how to send JSON data over WebSockets between the client and server - this is very simple to do and provides a convenien...
The web_app.ts file initiates the ItemRequestMsg object based on user input from the web UI and sends it to Real-Time Advanced Distribution Server via WebSocket.send() function. This item request message object will be compiled to a standard Item Request JSON message in the compiled JavaScript ... WebSockets support sending and receiving: strings, typed arrays (ArrayBuffer) and Blobs. Javascript objects must be serialized to one of the above types before sending. To send an object as a string you can use the builtin JSON support: ws.send(JSON.stringify(object)); In JavaScript you can extend a built-in functions, just like we extend structs, classes or protocols in Swift. We are extending the WebSocket object with a helper method to send JSON messages with the client UUID encoded as blob data (sendJsonBlob). When the main.js file is loaded all the top level code gets executed.
Learn how to send and receive data in JSON in WebSocket communication. Realize multiple paths for WebSocket communication using JSON. Premise. In this article, I will mainly describe how to handle JSON. For an explanation of socket communication, please refer to past articles and googled pages. ⇒ Implement the server program in Java and the ... Mar 27, 2018 - Simply enter the name of the WebSocket JavaScript variable, here it is webSocket and the console will print a JSON representation of the object. The readyState property of the WebSocket object stores the status of the connection. A value of 1 means that the connection is open and ready to receive and send ... How websockets work. For this tutorial, we will assume you are familiar with Node.JS. Websockets are essentially constant connections made between the server and your computer. When you access a website, it can send a GET request to the server, to initiate a websocket connection between the user and the server. Websockets vs REST API
JavaScriptでのJSON送受信. JavaScriptに関しては特筆すべきことはない。 前述した通り、JSON.stringify()メソッド及びJSON.parse()メソッドで簡単にオブジェクト ⇔ JSON間の変換が可能。 クライアントから送信前にエンコード、受信後にデコードすれば問題無い。 Real-time data streaming using FastAPI and WebSockets. We have several options for real-time data streaming in web applications. We can use polling, long-polling, Server-Sent Events and WebSockets. The last two can be used for server-push scenarios where we want to send data to a browser without any specific request from the client. Writing WebSocket client applications. WebSocket client applications use the WebSocket API to communicate with WebSocket servers using the WebSocket protocol. Note: This feature is available in Web Workers. Note: The example snippets in this article are taken from our WebSocket chat client/server sample.
The WebSocket.send() method enqueues the specified data to be transmitted to the server over the WebSocket connection, increasing the value of bufferedAmount by the number of bytes needed to contain the data. If the data can't be sent (for example, because it needs to be buffered but the buffer is full), the socket is closed automatically. Jun 12, 2013 - JSON WebSocket. Simple abstraction on top of websockets to send 'typed' JSON objects and emit received messages by type. Suitable for use in Node or the browser. A JSON event-based convention for WebSockets. This post was originally published on the New Bamboo blog, before New Bamboo joined thoughtbot in London. HTML5 WebSockets are cool. Given a compliant server - and browser - all you need to do is instantiate your socket object and start listening to server-pushed data.
May 10, 2017 - Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community · By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails The protocols property is used to specify the sub-protocol that the WebSocket connection should use. You can pass either a string, or an array of strings. The retryProcessTimePeriod property is used to help buffer the time between trying to resend a message over a WebSocket connection. By default it is a number, 50 (for 50 miliseconds). You can adjust this value in the client instance. WebSockets Send Message ... To create a WebSocket and open the connection to FME Server use getWebSocketConnection method. The result is an open WebSocket you can ...
To send a message to the WebSocket server, first encode the desired message as a JSON string. In JavaScript, for example, you can use the JSON stringify() function and then send the message using the WebSocket send function: //ws is a connected WebSocket object var backgroundMessage = JSON. stringify ... WebSocket JSON javascript/jquery. 567. November 22, 2016, at 12:28 PM. I'm sending JSON via a websocket, but some of the created messages are empty. function chVol(vol) { var send = {}; send["payload"] = {}; send["payload"] ["volume"] = vol; ws.send(JSON.stringify(send)); } If vol has the value 0 or 1, the messages are correct and at some other ... This is some pseudocodish JavaScript: ... we want to send something to the other client websocket.send(JSON.stringify({ to: "client2", data: "foo" }));.1 answer · Top answer: As I understand it, you want the server be able to send messages through from client 1 to client 2. You cannot directly connect two clients because one ...
WebSocket .send() method can send either text or binary data. A call socket.send(body) allows body in string or a binary format, including Blob, ArrayBuffer, etc. No settings required: just send it out in any format. When we receive the data, text always comes as string. And for binary data, we can choose between Blob and ArrayBuffer formats.
Spring Boot Websocket Tutorial
How Do I Send An Array Of String Issue 1740 Websockets
Building Real Time Apps With Websockets Amp Server Sent Events
Esp32 Arduino Websocket Server Receiving And Parsing Json
Esp32 Arduino Websocket Server Receiving And Parsing Json
Java Web Application Sending Json Messages Through Websocket
Websocket Implementation With Spring Boot And Stomp Toptal
Json Handling In Websockets With Play Framework
Playing With Websockets For Fun And Profit Debugging Remote
How To Send Server Side Notifications Through Websockets In
Getting Started Websockets 9 1 Documentation
Html Over Websockets Testdriven Io
Websockets Tutorial Creating A Real Time Websocket Server
Websockets And Node Js Testing Ws And Sockjs By Building A
Websocket Vs Rest Learn The 8 Important Differences
Websockets Support In Asp Net Core Microsoft Docs
Getting Started With Node Js And Websockets By Mike Cronin
0 Response to "22 Javascript Websocket Send Json"
Post a Comment