25 Javascript Position Of Character In String
The indexOf () method returns the position of the first occurrence of a specified value in a string. indexOf () returns -1 if the value is not found. indexOf () is case sensitive. Tip: Also look at the lastIndexOf () method. 10/12/2020 · The number is guaranteed to be smaller than the length of the array. The function should insert the character char after every n characters in the string and return the newly formed string. For example −. If the arguments are −. const str = 'NewDelhi'; const n = 3; const char = ' '; Then the output string should be −. const output = 'Ne wDe lhi';
Get First Character Of String Array Javascript Code Example
Characters in a string are indexed from left to right. The index of the first character is 0, and the index of the last character of a string called stringName is stringName.length - 1.
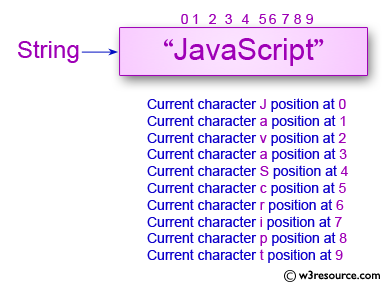
Javascript position of character in string. Description In JavaScript, lastIndexOf () is a string method that is used to find the location of a substring in a string, searching the string backwards. Because the lastIndexOf () method is a method of the String object, it must be invoked through a particular instance of the String class. Javascript strings are immutable, they cannot be modified "in place" so you cannot modify a single character. in fact every occurence of the same string is ONE object. - Martijn SchefferMay 7 '19 at 20:33 Add a comment | 25 Answers Given a string and a character, your task is to find the first position of the character in the string. These types of problem are very competitive programming where you need to locate the position of the character in a string. Let's discuss a few methods to solve the problem. Method #1: Using Naive Method
The indexOf function is a method of the String object, so to find the position of a string within the "foo" variable above we would use foo.indexOf (). This returns an integer value where 0 is the first character in the string. If the string to look for is not found, -1 is returned. slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0. In JavaScript, charAt() is a string method that is used to retrieve a character at a specific position in a string. Because the charAt() method is a method of the String object, it must be invoked through a particular instance of the String class.
charAt() is a method that returns the character from the specified index. Characters in a string are indexed from left to right. The index of the first character is 0, and the index of the last character in a string, called stringName, is stringName.length - 1. Syntax. Use the following syntax to find the character at a particular index. The indexOf () method returns the position of the first occurrence of specified character (s) in a string. Tip: Use the lastIndexOf method to return the position of the last occurrence of specified character (s) in a string. In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str):
13/11/2020 · If you need to find the exact position of the letter in the string, however, you need to use the indexOf() method: 'a nice string' . indexOf ( 'a' ) //0 'a nice string' . indexOf ( 'c' ) //4 If there are more than one occurrence, this method returns the position of the first one it finds, starting from the left. The lastIndexOf () method returns the position of the last occurrence of a specified value in a string. lastIndexOf () searches the string from the end to the beginning, but returns the index s from the beginning, starting at position 0. lastIndexOf () returns -1 if the value is not found. lastIndexOf () is case sensitive. 17/7/2020 · To grab one or more characters at a certain position in a string, the built-in JavaScript function substr() is really handy. It takes two arguments, the position where to start (as with arrays, the index starts with 0, so the third character in a string would be at position 2) and how many characters to extract.
The first character in the string is H, which corresponds to the index 0.The last character is ?, which corresponds to 11.The whitespace characters also have an index, at 3 and 7.. Being able to access every character in a string gives us a number of ways to work with and manipulate strings. String.prototype.charCodeAt () The charCodeAt () method returns an integer between 0 and 65535 representing the UTF-16 code unit at the given index. The UTF-16 code unit matches the Unicode code point for code points which can be represented in a single UTF-16 code unit. If the Unicode code point cannot be represented in a single UTF-16 code ... JavaScript String indexOf() method. The JavaScript string indexOf() method is used to search the position of a particular character or string in a sequence of given char values. This method is case-sensitive. The index position of first character in a string is always starts with zero. If an element is not present in a string, it returns …
26/2/2020 · "JavaScript We are doing some exercises." "We are doing some JavaScript exercises." Pictorial Presentation: Sample Solution:-HTML Code: <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Insert a string within a specific position in another string</title> </head> <body> </body> </html> JavaScript Code: Strings are used to store a series of characters. Each character of a string can be accessed by its index. The index starts at 0 and ends at length - 1, where length is the length of the string. For the first character, it is 0 and for the last character, it is length - 1. JavaScript String slice() method: This method gets parts of a string and returns the extracted parts in a new string. Start and end parameters are used to specify the part of the string to extract. First character starts from position 0, the second has position 1, and so on. Syntax: string.slice(start, end) Parameters: start: This parameter is ...
25/1/2020 · To find a character in a String has to use a includes () method in the JavaScript program. Another way to a string contains a certain character using a regular expression. They include () method finds the “contains” character in whole string, it will return a true. Example 1: Find a “t” character in the string. Strings In JavaScript, the textual data is stored as strings. There is no separate type for a single character. The internal format for strings is always UTF-16, it is not tied to the page encoding. Let's see the usage in an example. Suppose that we have the example string below: const myString = "I am learning JavaScript and it is cool!"; Now if we set the startIndex as 0 and the endIndex as 10, then we will get the first 10 characters of the original string: The first character's index is always 0.
The length of a string can be find out by reading the .length property in Javascript. We can also get the character at any position of a string using charAt method. str.charAt(i) returns the character at index i for the string str. The index starts at 0 for string characters. As per the output above, you can see that specified first and last characters are removed from the original string. With substr() method. substr() method will retrieve a part of the string for the given specified index for start and end position. Let's remove the first character from string using substr function in the below example. Given a URL and the task is to remove a portion of URL after a certain character using JavaScript. split () method: This method is used to split a string into an array of substrings, and returns the new array. Syntax: string.split (separator, limit) Parameters: separator: It is optional parameter.
Retrieving a specific string character On a related note, you can return any character inside a string by using square bracket notation — this means you include square brackets ([]) on the end of your variable name. If you've ever found yourself saying: "How do I figure out if one string contains a certain character or a sub-string?" then the JavaScript indexOf () method is for you. It returns the starting position of a string, even if it is one character. If that string does not exist, then it returns "-1". Can you please explain what you mean by get a character position in my text after a click on the character - ManavM Mar 24 '19 at 21:01 Click on the letter -> get the position of the letter relative to the text. - miha64 Mar 24 '19 at 21:08
The substr () method extracts parts of a string, beginning at the character at a specified position, and returns a specified number of characters. Tip: To extract characters from the end of the string, use a negative start number. substr () method does not change the original string. 5/10/2018 · If you know the string position of the character in the text, you can wrap the character in a <span> or similar element with an ID and find the x,y coords of that element. Easiest way to do this is with jQuery or another library, see this answer for a cross-browser, no-library method.
Add Remove Replace String In C
How To Index And Slice Strings In Python 3 Digitalocean
Indexed Collections Javascript Mdn
Strings In Powershell Replace Compare Concatenate Split
Javascript To Find The Position Of The Left Most Vowel In The
Javascript String Indexof How To Get Index Of String
Using Javascript Substring Substr Amp Slice To Pull Sub
Javascript Split A String At The Index Particular And Nth
Python Print The Index Of The Character In A String W3resource
In Java How To Get All Text After Special Character From
Counting The Number Of Characters In A String Java Code Example
Javascript Split A Step By Step Guide Career Karma
Basics Of Javascript String Charat Method By Jakub
Everything You Need To Know About Regular Expressions By
Javascript Remove First Character Design Corral
How To Find A Substring In A String With C Agnostic
Javascript Function Letter Count Within A String W3resource
How To Manipulate And Search Javascript Strings To Program
How To Remove A Character From String In Javascript
Javascript Es5 Interview Questions Amp Answers
Javascript Insert A String At Position X Of Another String
Javascript How To Get Middle Letters Of A String Code Example
0 Response to "25 Javascript Position Of Character In String"
Post a Comment