24 Javascript Callback Extra Parameters
A callback function is a function that is passed as a parameter into another function. Callback functions are run within the function in which they are declared. When you execute a function, its callback function, if one is specified, will execute. Once it has run, the callback function will return a response to the main function. The above example is a synchronous callback, as it is executed immediately.. Note, however, that callbacks are often used to continue code execution after an asynchronous operation has completed — these are called asynchronous callbacks. A good example is the callback functions executed inside a .then() block chained onto the end of a promise after that promise fulfills or rejects.
Chapter 9 Asynchronous Programming With Callbacks And
15/2/2016 · If you reversed the parameters to print, you could do this a little more elegantly like so: function print(str, num) { .. }; array.forEach(print.bind(null, str)); str will be bound as the first parameter, any parameters that forEach passes when invoking the callback are passed in second, third etc. place.
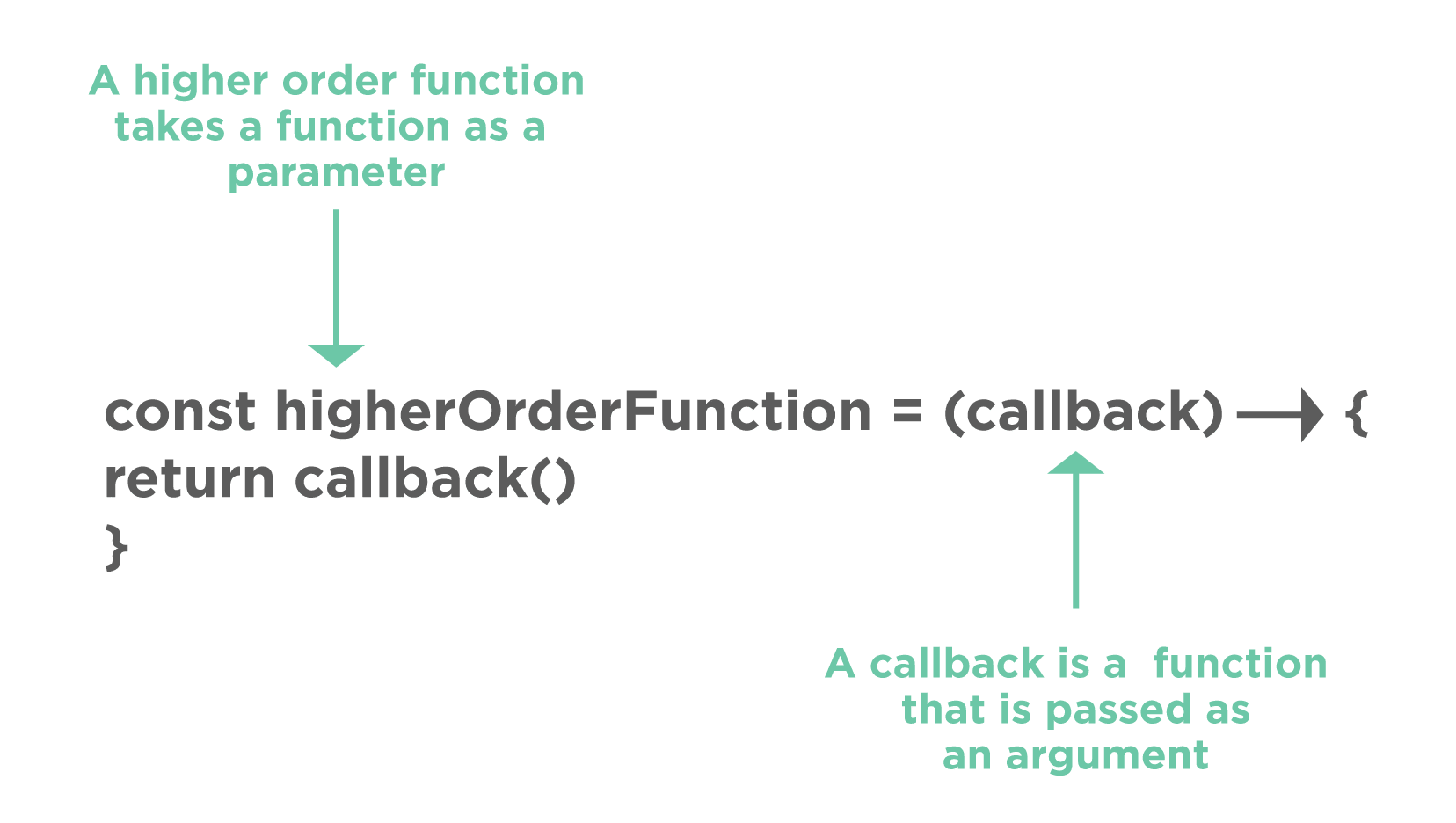
Javascript callback extra parameters. The event and callback structure is the fundamental mechanism by which JavaScript engines are able to efficiently handle overlapping tasks, like responding to I/O requests. This is why understanding JavaScript callbacks is essential to understanding asynchronous programming in JavaScript. JavaScript Callback function are the most special and important function of JavaScript whose main aim is to pass another function as a parameter where the callback function runs which means one function when infused into another function with the parameters is again called as per the requirement. The parameters passed by the function at the ... Using some extra node.js methods we can operate a callback-based method in promise way. Syntax: fs.writeFile (path, data, options) Note: Callback not required since we operate the method with promises. Parameters: Accepts three parameter path, data and options. The options is an optional parameter.
Note that the parameters given in the callback specification are used first, and then additional parameters from the call to the callback come afterward. ... When you use a method of a JavaScript object, a special variable called this is defined that refers to the object whose method is being ... Oct 22, 2012 - Lets say you're using jQuery ajax to do something, and you're passing it named callbacks. Here we have an onError callback that you might use to log or handle errors in your application. It conforms to the jQuery Ajax error callback signature, except for an extra parameter that you might have ... thisArg. The value to be passed as the this parameter to the target function func when the bound function is called. The value is ignored if the bound function is constructed using the new operator. When using bind to create a function (supplied as a callback) inside a setTimeout, any primitive value passed as thisArg is converted to object.
Jul 02, 2020 - This article gives a brief introduction to the concept and usage of callback functions in the JavaScript programming language. Functions are Objects The first thing we need to know is that in JavaScript, functions are first-class objects. As such, we can work with them in the same way we work ... Callback functions are a concept derived from functional programming and specifies the use of functions as arguments. In Javascript every function is a first class object, which means that every ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Now you have a strong type callback instead of just passing around function as we usually do in JavaScript. Generalize the callback type. We could also use generic to generalize the use case of callback with one parameter as below. By doing so, you don't have to define a new interface with a new name each time you need to use a callback with ... Nov 14, 2019 - Recently I was taking Node.js classes for company’s training batch. I was explaining about the code reusability & callback functions at that time. At that time came across an interesting problem due… Simply put: A callback is a function that is to be executed after another function (normally asynchronous) has finished executing — hence the name 'call back'. More complexly put: In ...
Sep 15, 2019 - Every function in JavaScript has two methods viz. Call and Apply. These methods are used to set this object inside the function to pass arguments to functions. Let’s see how easy are Call or Apply by applying it to the previous example. // note that we have added extra parameter called callbackObjFunction ... The callback function gets executed (called) inside the higher order function, but not necessarily immediately. It gets "called back" — hence the name — at whatever point serves the code's purpose. Getting REALLY Tricky: 'this' in Callback Functions. Many people find JavaScript callbacks challenging to begin with. Callbacks make sure that a function is not going to run before a task is completed but will run right after the task has completed. It helps us develop asynchronous JavaScript code and keeps us safe from problems and errors. In JavaScript, the way to create a callback function is to pass it as a parameter to another function, and then to call ...
Nov 22, 2015 - I am using drupal 7. I need to add extra arguments to my ajax callback function. I have five fieldsets in my form, and in all of them same there are same elements, just their IDs are different. For In JavaScript, functions are objects; that is, functions are of the type Object and they can be used in a manner like any other object since they are in fact objects themselves. They can be stored in variables, passed as arguments to functions, created within functions, and returned from functions. Because functions are objects, we can pass a function as an argument in another The nesting gives all callbacks in the second chain access to connection. You may have noticed that, in both the sync and the async code, synchronous exceptions thrown by db.open() won't be handled by catch (clause or callback). A follow-up blog post on Promise.try() shows how to fix that for the async code.
Callbacks in asynchronous functions. Asynchronous here means that, if JavaScript needs to wait for something to complete, it will execute the rest of the tasks given to it while waiting.. An example of an asynchronous function is setTimeout.It takes in a callback function to execute at a later time: Apr 27, 2017 - Now if I wanted to pass the grading_company variable to the callback function as a parameter is there a way to do that without having to add it as another parameter in the runAjax call? I'm trying to keep the runAjax function open to other usage so I don't want to pass in any extra parameters; ... Nov 08, 2011 - Is there a way to pass more data into a callback function in jQuery? I have two functions and I want the callback to the $.post, for example, to pass in both the resulting data of the AJAX call, a...
In this post, we'll break down the pros and cons of three of the most common patterns, Callbacks, Promises, and Async/Await and talk about their significance and progression from a historical context. Let's start with the OG of these data fetching patterns, Callbacks. Callbacks. I'm going to assume you know exactly 0 about callbacks. Nov 08, 2011 - A new version for the scenario ... and you want to add additional parameters. For example, let's pretend that you have a lot of nested calls with success and error callbacks. I will use angular promises for this example but any javascript code with callbacks would be the same ... Dec 02, 2014 - As you see in the preceding example, we pass a function as a parameter to the click method. And the click method will call (or execute) the callback function we passed to it. This example illustrates a typical use of callback functions in JavaScript, and one widely used in jQuery.
Of course, as I said, it's unpredictable when the callbacks will be executed and depends on multiple factors the JavaScript interpreter used, the function invoking the callbacks and it's input data. An example is an HTTP request with a success callback that won't be executed before the server sends a response, which could be any time interval ... The callback function runs after the completion of the outer function. It is useful to develop an asynchronous JavaScript code. In JavaScript, a callback is easier to create. That is, we simply have to pass the callback function as a parameter to another function and call it right after the completion of the task. Passing Extra Data to Callbacks. It's common to need to pass extra information to a callback method, but since all callback methods have only one parameter (the return code from the remote method) this can be tricky. ... callbackArg, and exceptionArg. For an alternative approach you may use a Javascript closure. Recommended Method.
JavaScript callback functions; another important concept you must need to understand to become a successful JavaScript developer. But I am sure that after reading this article thoroughly you will ... Callback Functions. A callback function is a function that is passed as an argument to another function, to be "called back" at a later time. A function that accepts other functions as arguments is called a higher-order function, which contains the logic for when the callback function gets executed. It's the combination of these two that ... Answer 7. If you are not sure how many params are you going to be passed into callback functions. Using apply. function tryMe (param1, param2) { alert (param1 + " and " + param2); } function callbackTester(callback,params) { callback.apply(this,params); } callbackTester(tryMe, ['hello','goodbye']); Answer 8.
In JavaScript, a callback is a function passed into another function as an argument to be executed later. Suppose that you the following numbers array: let numbers = [ 1, 2, 4, 7, 3, 5, 6 ]; Code language: JavaScript (javascript) To find all the odd numbers in the array, you can use the filter () method of the Array object. Nov 25, 2016 - Not the answer you're looking for? Browse other questions tagged javascript function parameters callback or ask your own question. Jan 16, 2016 - By default you cannot pass arguments to a callback function. For example:
Explain the callback Promise.finally in JavaScript; JavaScript Function Parameters; Can a C++ virtual functions have default parameters? What are default-parameters for function parameters in JavaScript? Is there an elegant way of passing an object of parameters into a function? Parameters & Arguments in JavaScript. Pseudo mandatory parameters ... Oct 29, 2013 - Consider a typical Express/node.js app, such as a web scraper, one that takes a user request, parses that url to make a request to the "real" website", scrapes or otherwi... JavaScript does not enforce a function’s arity: you can call it with any number of actual parameters, independent of what formal parameters have been defined. Hence, the number of actual parameters and formal parameters can differ in two ways: ... The extra parameters are ignored but can ...
JavaScript Callback Functions Explained. This is a Javascript function called sayHi. Whenever it is called/executed it will output the text "Hi!". function sayHi() { console.log("Hi!") } sayHi() // "Hi!" We can also pass arguments to our function. For example, now our function has one parameter x. A callback, as the name suggests, is a function that is to execute after another function has finished executing. As we know, in JavaScript, functions are objects. Because of this, functions can take functions as arguments, and other functions can also return it. Functions that take the additional function as a parameter are called higher-order ... Syntax: Above is an example of a callback variable in JavaScript function. "geekOne" accepts an argument and generates an alert with z as the argument. "geekTwo" accepts an argument and a function. "geekTwo" moves the argument it accepted to the function to passed it to. "geekOne" is the callback function in this case.
As we know that the callback function is asynchronous in nature. One of the simplest examples of how to use callbacks is timers. Timers are not part of JavaScript, but they are provided by the browser. Let me talk about one of the timers we have: setTimeout (). The setTimeout () function accepts 2 arguments: a function, and a number. JavaScript Callbacks. A callback is a function passed as an argument to another function. Using a callback, you could call the calculator function ( myCalculator ) with a callback, and let the calculator function run the callback after the calculation is finished: Example. function myDisplayer (some) {. Callbacks are a great way to handle something after something else has been completed. By something here we mean a function execution. If we want to execute a function right after the return of some other function, then callbacks can be used. JavaScript functions have the type of Objects. So, much like any other objects (String, Arrays etc.),
Everything You Need To Know About Foreach Loops In Js
How To Operate Callback Based Fs Readfile Method With
Using Callbacks And Closures In Javascript By John Au Yeung
How Not To Be Afraid Of Javascript Anymore Neil Kakkar
Mastering This In Javascript Callbacks And Bind Apply
Declarative Javascript Functions
Asynchronous Error Handling In Javascript Ruben Verborgh
Javascript Why Named Arguments Are Better Than Positional
Passing Arguments To Callback Functions
Functions And Callbacks In Javascript By Sakshat
Understanding Destructuring Rest Parameters And Spread
You Can Convert Your Callbacks To Promises Dev Community
Passing An Extra Parameters To Addeventlistener Code Example
How To Share Information Between Your Applications Twilio
Tools Qa What Are Callback Functions In Javascript And How
A Practical Guide To Es6 Arrow Functions By Arfat Salman
Promises Next Ticks And Immediates Nodejs Event Loop Part
Javascript Code Used By Group And Assignee In Incident Form
Javascript Function Multiple Parameters Code Example
Async Programming With Javascript Callbacks Promises And
Javascript Callback Function With Parameters Passing
0 Response to "24 Javascript Callback Extra Parameters"
Post a Comment