29 Change Keys Of Object Javascript
Let's see what utility functions provide JavaScript to extract the keys, values, and entries from an object. 1. Object.keys () returns keys. Object.keys (object) is a utility function that returns the list of keys of object. Let's use Object.keys () to get the keys of hero object: Object.keys (hero) returns the list ['name', 'city'], which ... in JavaScript it's the most easy, just because JSON is native valid JavaScript syntax, can copy a JSON object into a JavaScript source code and assign to a variable, it just works, even no need ...
In the loop, we'll create a new key from the value of the key in the original object. We'll assign the value of that key the property we're currently looking at in our iteration. That's all there is to inverting the keys and values! Of course, we'll return the newly generated object. Use Cases. Okay, so we can invert k, v pairs.
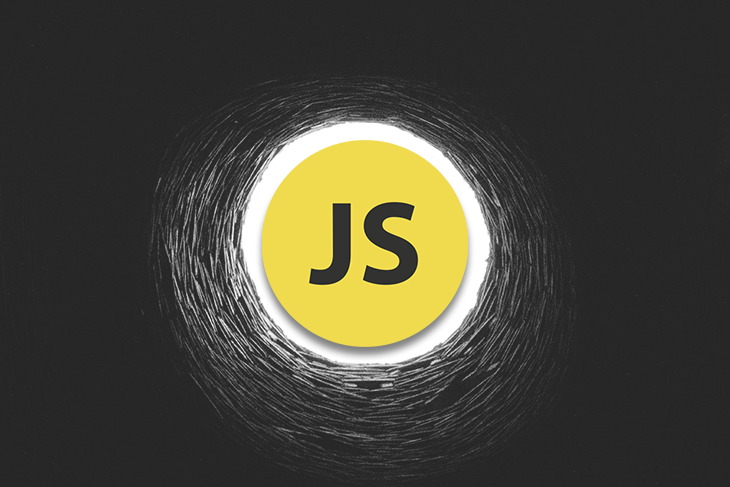
Change keys of object javascript. Object.keys () Method The Object.keys () method was introduced in ES6. It takes the object that you want to iterate over as an argument and returns an array containing all properties names (or keys). You can then use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of each property. In JavaScript, getting the keys and values that comprise an object is very easy. You can retrieve each object's keys, values, or both combined into an array. The examples below use the following object: const obj = { name: 'Daniel', age: 40, occupation: 'Engineer', level: 4 }; If we'd like to apply them, then we can use Object.entries followed by Object.fromEntries: Use Object.entries (obj) to get an array of key/value pairs from obj. Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries (array) on the resulting array to turn it back into an object.
To add a new key-value pair to an object, the simplest and popular way is to use the dot notation: foods. custard = '🍮'; console.log( foods); Alternatively, you could also use the square bracket notation to add a new item: foods ['cake'] = '🍰'; console.log( foods); As you can see above, when you add a new item to an object, it usually ... This is the basic object syntax. But there are a few rules to keep in mind when creating JavaScript objects. Object Keys in JavaScript. Each key in your JavaScript object must be a string, symbol, or number. Take a close look at the example below. The key names 1 and 2 are actually coerced into strings. Introduction. An object in JavaScript is a data type that is composed of a collection of names or keys and values, represented in name:value pairs.The name:value pairs can consist of properties that may contain any data type — including strings, numbers, and Booleans — as well as methods, which are functions contained within an object.. Objects in JavaScript are standalone entities that ...
Object.keys () method is used to return an array whose elements are strings corresponding to the enumerable properties found directly upon an object. The ordering of the properties is the same as that given by the object manually in a loop is applied to the properties. Object.keys () takes the object as an argument of which the enumerable own ... Edit: See how to rename many object keys here. If you're okay with mutating data, renaming an object's key is easy. obj = { name: 'Bobo' } obj.somethingElse = obj.name. delete obj.name. If you ... When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
JavaScript: replacing object keys with an array We are required to write a JavaScript function that takes in an object and an array of literals. The length of the array and the number of keys in the object will always be equal. Our function should replace the corresponding keys of the object with the element of the array. I would like to listen about the ways to improve the following code: function toLowerCaseKeys(obj) { return Object.keys(obj).reduce Stack Exchange Network Stack Exchange network consists of 178 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their ... How to merge an array with an object where values are arrays - JavaScript; How to change an object Key without changing the original array in JavaScript? How to remove a function from an object in JavaScript? How to access an object value using variable key in JavaScript? Previous Page Print Page. Next Page . Advertisements
The Object.keys () method accepts one parameter: the name of the Object whose keys you want to retrieve. This method returns the names of all keys in the Object you have specified, stored as a JavaScript list. Notice that the method itself is called Object.keys (). This is because keys () is a method of Object. change key in object javascript; new object with a name key; change key names in object; how to rename object key js; how to replace the key's name of an objetc; change object key name javascript es6 children; better way to update all keys in an object javascript; Although it is a common belief that a regular JavaScript Object can already handle Numbers, booleans, and other primitive data types as keys, this is actually not the case, because Objects change all keys to strings. As an example, initialize an object with a numerical key and compare the value for a numerical 1 key and a stringified "1" key:
Converting Object Keys from Snake Case to Camel Case with Javascript. I run into a situation frequently when dealing with APIs where my JavaScript frontend expects camel case object keys while APIs tend to return snake case keys. I've settled on a few utility methods for converting these that are worth sharing here. That's the same, because Object.fromEntries expects an iterable object as the argument. Not necessarily an array. And the standard iteration for map returns same key/value pairs as map.entries().So we get a plain object with same key/values as the map.. Set. A Set is a special type collection - "set of values" (without keys), where each value may occur only once. JavaScript Program to Add Key/Value Pair to an Object In this example, you will learn to write a JavaScript program that will add a key/value pair to an object. To understand this example, you should have the knowledge of the following JavaScript programming topics:
Object.values() returns an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by looping over the property values of the object manually. JavaScript doesn't provide an inbuilt function to rename an object key. So we will look at different approaches to accomplish this in this article. Keys: In JavaScript, objects are used to store collection of various data. It is a collection of properties. A property is a "key:value" pair. Keys are known as 'property name' and are ... Move object (image) with arrow keys using JavaScript function - In this example we will learn how to move object/image using arrow keys (left, top, right and down). We will call function in Body tag on "onkeydown" event, when you will down arrow keys object will be moved on the browser screen.
Definition and Usage. The keys () method returns an Array Iterator object with the keys of an array. keys () does not change the original array. That's easy! Lets create function to do that: ```js const renameKey = (object, key, newKey) => { con. JetRockets Case studies Services ... 29, 2019 Rename the key name in the javascript object. Alexander BlinovFrontend Developer at JetRockets. How to rename the key name in the javascript object? That's easy! Lets create function to do that: ... Personally, the most effective way to rename keys in object without implementing extra heavy plugins and wheels: var str = JSON.stringify(object); str = str.replace(/oldKey/g, 'newKey'); str = str.replace(/oldKey2/g, 'newKey2'); object = JSON.parse(str); You can also wrap it in try-catch if your object has invalid structure. Works perfectly :)
keyis just a local variable, it's not an alias for the actual object key, so assigning it doesn't change the object. 30 Seconds of Code is a brilliant collection of JavaScript snippets, digestible in ≤ 30 seconds. Anyone looking to master JavaScript should go through the entire thing. The list didn't contain a function to rename multiple object keys, however, so I created a pull request that thankfully got merged! Here' So, when you pass the key "programmer" to the object, it returns the matching value that is 2. Aside from looking up the value, you may also wish to check whether a given key exists in the object. The object may have only unique keys, and you might want to check if it already exists before adding one.
Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object.The ordering of the properties is the same as that given by looping over the properties of the object manually.
3 Ways To Add Dynamic Key To Object In Javascript Codez Up
Object Keys Function In Javascript The Complete Guide
How To Create Modify And Loop Through Objects In Javascript
How To Get A Key In A Javascript Object By Its Value
Change Object Studio Pro 9 Guide Mendix Documentation
Methods For Deep Cloning Objects In Javascript Logrocket Blog
How To Merge A List Of Maps By Key In Immutable Js Pluralsight
Javascript Merge Array Of Objects By Key Es6 Reactgo
Objects Puzzles Reference Soft8soft
Comparing Objects Why Am I Getting Different Results Using
Create Key Signature Changes In Logic Pro Apple Support
How To Rename Object Keys In React Javascript Using Lodash
Introduction To Keyboard Events In Javascript Engineering
Data Structures Objects And Arrays Eloquent Javascript
Modifying Json Data Using Json Modify In Sql Server
How To Create Key Value Object In Javascript Code Example
Introducing Amazon S3 Object Lambda Use Your Code To
Javascript Fundamental Es6 Syntax Create A New Object From
Javascript Objects In Nutshell Learnjavascript
5 Ways To Log An Object To The Console In Javascript By Dr
Netscape Certificate Management System Administrator S Guide
Responsive System Principle Of Vue Js
Rename Object Key In Javascript Geeksforgeeks
Never Confuse Json And Javascript Object Ever Again By
Javascript Object Rename Key Stack Overflow
Rewriting Javascript Converting An Array Of Objects To An
Javascript Object Dictionary Examples Dot Net Perls
0 Response to "29 Change Keys Of Object Javascript"
Post a Comment