29 How To Loop Backwards In Javascript
I've a simple FOR statement like this: var num = 10, reverse = false; for(i=0;i<num;i++){ console.log(i); } when reverse is false I want it to return something like [0,1,2,3,4,5,6,7,8,9]. but, when reverse is true, it should return [9,8,7,6,5,4,3,2,1,0]. Which is the most efficient way to get this result, without checking every time if reverse is true or false inside the loop? The break and the continue statements are the only JavaScript statements that can "jump out of" a code block. Syntax: break labelname; continue labelname; The continue statement (with or without a label reference) can only be used to skip one loop iteration. The break statement, without a label reference, can only be used to jump out of a loop ...
Reverse A String Using String Method Reverse A String Using
Mar 25, 2020 - Write a javascript program to find roots of quadratic equation.
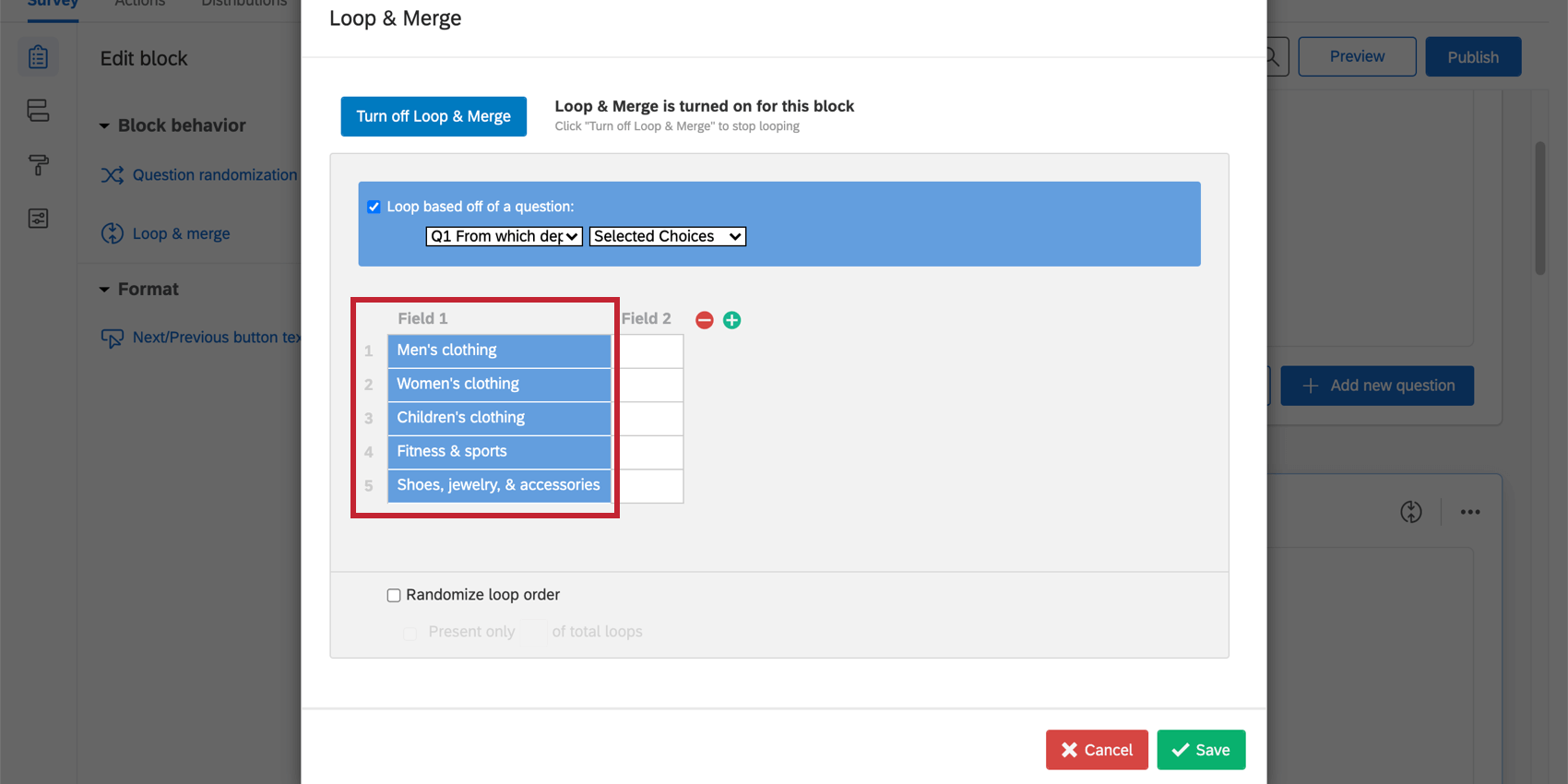
How to loop backwards in javascript. How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource · File C:\Users\Tariqul\App... There is no way to loop through an object backwards, but if you recreate the object in reverse order then you are golden! Be cautions however, there is nothing that says the order of the object will stay the same as it changes and so this may lead to some interesting outcome, but for the most part it works... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Efficiently Looping A Javascript Array Backwards. Most of the time when you're looping over an array of elements, you'll do it sequentially. However, I recently needed to iterate through an array of objects in reverse just using a plain old for loop. This will not be a highly informative or groundbreaking post but, I thought I'd share ... May 01, 2020 - Get code examples like "how to loop backwards through array js" instantly right from your google search results with the Grepper Chrome Extension. Jan 19, 2016 - Connect and share knowledge within a single location that is structured and easy to search. ... I've heard this quite a few times. Are JavaScript loops really faster when counting backward? If so, why? I've seen a few test suite examples showing that reversed loops are quicker, but I can't ...
The continue statement breaks one iteration (in the loop) if a specified condition occurs, and continues with the next iteration in the loop. The difference between continue and the break statement, is instead of "jumping out" of a loop, the continue statement "jumps over" one iteration in the loop. However, when the continue statement is ... How to Reverse an Array in JavaScript with the Spread Operator You can use a combination of the spread operator and the reverse method to reverse an array without changing the original. First, you put the elements returned from the spread operator into a new array by enclosing the spread syntax with square brackets []: java2s | © Demo Source and Support. All rights reserved
All my thinking about some code challenge and Free Code Camps - CodeChallenge/Count Backwards With a For Loop.md at master · EQuimper/CodeChallenge Learn More about Javascript below:http://amzn.to/2azP34wThis is a tutorial about how to reverse a for loop with an array. For loops are the most used loops in any language. But there is more than one way to iterate using a for loop. These are the ways you can use the for loop in JavaScript. The advantages and disadvantages are given too.
Reverse a String With a Decrementing For Loop function reverseString (str) { // Step 1. Create an empty string that will host the new created string var newString = ""; // Step 2. The For Loop. The for loop has the following syntax: for ( statement 1; statement 2; statement 3) {. // code block to be executed. } Statement 1 is executed (one time) before the execution of the code block. Statement 2 defines the condition for executing the code block. Statement 3 is executed (every time) after the code block has been executed. loop array backwards javascript . javascript by Confused Sphere on Jan 15 2021 Donate Comment . 3. Source: www.techiedelight . how to reverse loop in javascript . javascript by Dark Dunlin on Mar 25 2020 Comment . 2. js loop backwards . javascript by ...
How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource · File C:\Users\Tariqul\App... Loop through an array backwards in JavaScript, In order to loop through an array backwards using forEach method, we have to reverse the array. To a avoid modifying the original array, first create copy of the The reverse method transposes the elements of the calling array object in place, mutating ... A while loop evaluates the expression inside the parentheses each time through the loop. When that expression gets to a falsey value, the loop will stop. Examples of falsey values are: false 0 undefined NaN null ""
Explanation. In the first line the array is defined. Then with the size variable, we will store the length of the array. After that, we traverse the loop in reverse order, which is starting with "size-1" index, which is 4 in our case and we are iterating till the 0th index. Inside the loop, we print or work on our element. There is two popular way to make browsers go back to the previous page by clicking JavaScript event, both methods are described below: Method 1: Using history.go () method: The go () method of the window.history object is used to load a page from the session history. It can be used to move forward or backward using the value of the delta parameter. Learn to code with interactive screencasts. Our courses and tutorials will teach you React, Vue, Angular, JavaScript, HTML, CSS, and more. Scrimba is the fun and easy way to learn web development.
The reverse function contains a loop that runs until the number (entered by the user) becomes 0. The value will be set to zero in the starting and then gets multiplied by ten consecutively. The number is then increased by the number mod 10. loop array backwards javascript . javascript by Confused Sphere on Jan 15 2021 Donate Comment . 3. Source: www.techiedelight . how to reverse loop in javascript . javascript by Dark Dunlin on Mar 25 2020 Comment . 2. Add a Grepper Answer ... The best way to reverse a string is by using three different JavaScript built-in methods: split (), reverse () and join (). split () - It splits a string into an array of substrings using a separator, and returns the new array. reverse () - This method reverses the order the elements in an array.
Dealing with arrays is everyday work for every developer. In this article, we are going to see 6 different approaches to how you can iterate through in Javascript. for Loop. The for loop statement has three expressions: Initialization - initialize the loop variable with a value and it is executed once; Condition - defines the loop stop condition The arr.reverse() method is used for in-place reversal of the array. The first element of the array becomes the last element and vice versa. Syntax: arr.reverse() Parameters: This method does not accept any parameter. Return value: This method returns the reference of the reversed original array. var arr = [1, 2, 3, 4, 5]; for (var i = arr.length - 1; i >= 0; i--) { console.log(arr[i]); }
Oct 10, 2017 - The instructions state the following… We need to make three changes to our for loop: Edit the start condition (var i = 0), to set i equal to the length of the vacationSpots array. Then, set the stop condition ( i < vacationSpots.length) to stop when i is greater than or equal to 0. Jul 20, 2021 - The reverse() method reverses an array in place. The first array element becomes the last, and the last array element becomes the first. loop array backwards javascript . javascript by Confused Sphere on Jan 15 2021 Donate Comment . 3. Source: www.techiedelight . Add a Grepper Answer . Javascript answers related to "reverse array using without loop javascript" javascript reverse array; forward and reverse loop one by one js ...
May 01, 2020 - How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource · File C:\Users\Tariqul\App... Apr 28, 2021 - This post will discuss how to loop through an array backward in JavaScript... The standard approach is to loop backward using a for-loop starting from the end of the array towards the beginning of the array. Aug 31, 2018 - A typical JavaScript challenge or interview question has to do with reversing arrays in-place and out-of-place. Specifically, take this problem from Eloquent JavaScript, 2nd Edition: I worked through…
First, Use the split method to split a string into individual array elements. Then use the array reverse () method to reverse elements. In the last join into a single string using the join () method. loop array backwards javascript . javascript by Confused Sphere on Jan 15 2021 Donate Comment . 3. Source: www.techiedelight . Add a Grepper Answer . Javascript answers related to "array backwards loop" array reverse algorithm in js; array reverse in javascript ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
I had the need to reverse a JavaScript array, and here is what I did. Given an array list: const list = [1, 2, 3, 4, 5] The easiest and most intuitive way is to call ... Java - Reverse loop versus Forward loop in Performance. This article is using the endTime - startTime method to measure the performance of a loop, it ignores the JVM warm up optimization, the result may not consistent or accurately. A better way is using the OpenJDK JMH framework to do the benchmark testing, because it will take care of the ... Get code examples like "javascript loop backwards" instantly right from your google search results with the Grepper Chrome Extension.
The continue statement can be used to restart a while, do-while, for, or label statement.. When you use continue without a label, it terminates the current iteration of the innermost enclosing while, do-while, or for statement and continues execution of the loop with the next iteration. In contrast to the break statement, continue does not terminate the execution of the loop entirely. There are many methods to reverse a string in JavaScript some of them are discussed below: ... Loop through the array from the end to the beginning and push each and every item in the array revArray[]. Use join() prebuilt function in JavaScript to join the elements of an array into a string. Aug 15, 2017 - But what if we want to iterate over the array backwards? The usual way would be to use a regular for or while loop, like : But this way we lose the convenience of using a for..of loop. In order to…
Loop backward in array of objects JavaScript. Javascript Web Development Object Oriented Programming. We have an array of objects like this − ...
Example Of While Loop In Javascript With Array Length Code
Applied Sciences Free Full Text A Review On The Lifecycle
Write A Program To Reverse An Array Or String Geeksforgeeks
Python Program To Iterate Over The List In Reverse Order
Basic Javascript Count Backwards With A For Loop Issue
How To Reverse A For Loop In Javascript
Javascript Performance For Vs Foreach
Count Backwards With A For Loop Freecodecamp Basic Javascript
C Program To Print Natural Numbers In Reverse Order
For Loop Backwards Javascript Codecademy Forums
Best Practices For Reversing A String In Javascript C Amp Python
How To Reverse A Number In Java Javatpoint
Loops And Debugging In Javascript
C Iterate Over A Vector In Reverse Order Backward
How To Reverse A String In Javascript Step By Step
How To Knit A Backwards Loop Cast On 9 Steps With Pictures
How To Read Recursively A Directory In Node Js Our Code World
Backward Loop Increase How To Do M1bl For Beginners Video
How To Iterate For Loop In Reverse Order In Swift Stack
Solution Using A While Loop To Reverse String In Javascript
Javascript Reverse Array Tutorial With Example Js Code
0 Response to "29 How To Loop Backwards In Javascript"
Post a Comment