20 Pass This To Javascript Function
And something I will add to this is that this feature alone - being able to pass JavaScript functions as arguments or variables or the like - is the feature that makes JavaScript so powerful and so great to code in. - TheHans255 Dec 9 '15 at 22:16. 3. In this tutorial learn about the Function Arguments, Parameters, and Argument Objects in JavaScript. We learned how to create a function in JavaScript. The parameters are the way we pass values to a JavaScript function. We learn the difference between argument and parameters, setting Default Parameters & learn about Argument Object.
How To Rewrite A Callback Function In Promise Form And Async
javascript by Grepper on Jul 31 2019 Donate Comment. 5. //passing a function as param and calling that function function goToWork (myCallBackFunction) { //do some work here myCallBackFunction (); } function refreshPage () { alert ("I should be refreshing the page"); } goToWork (refreshPage); xxxxxxxxxx. 1.
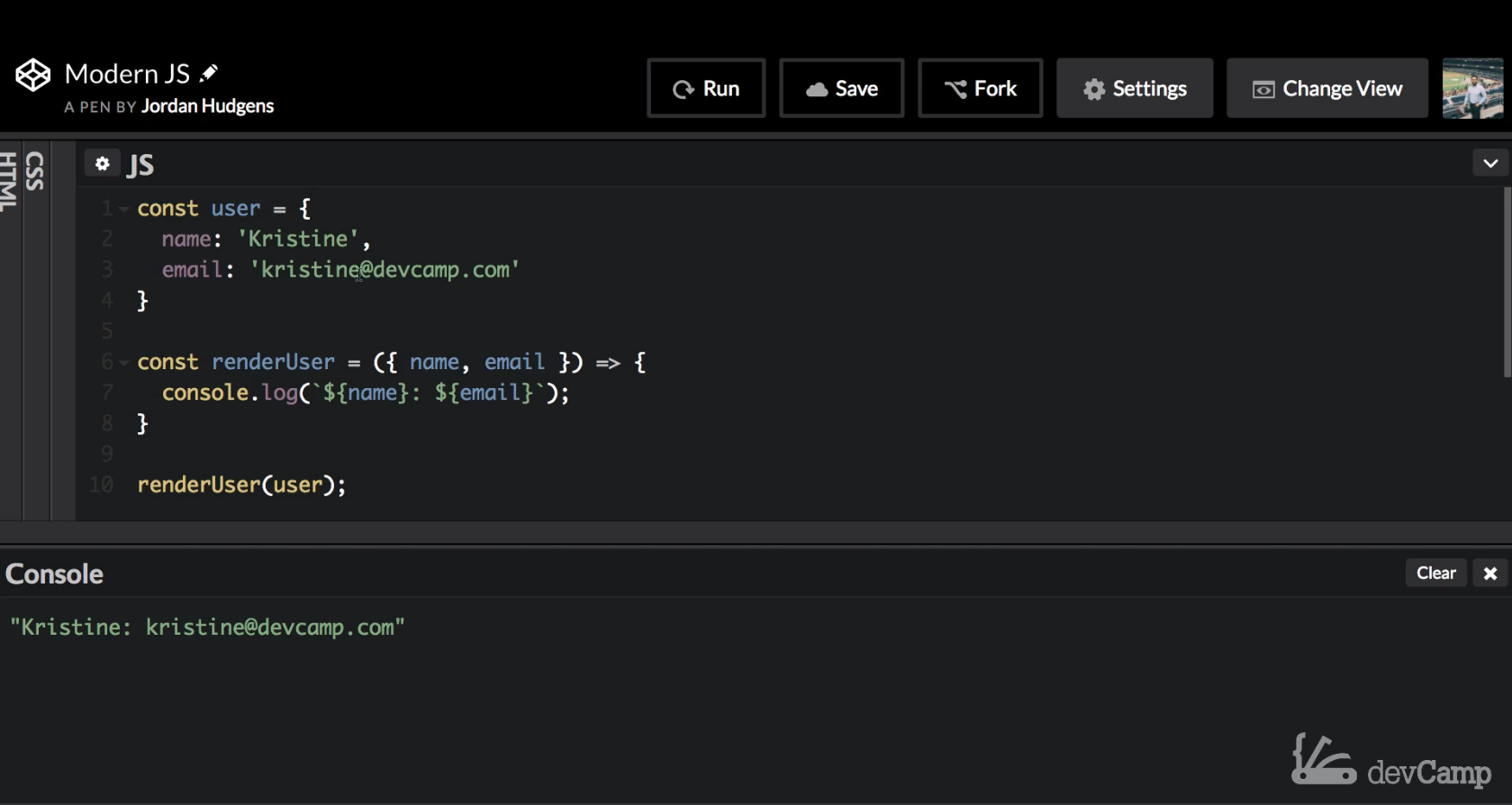
Pass this to javascript function. As I mentioned the javascript is generic and I don't want to manipulate the form inside it! I could create a helper function in the middle to get the form values with jQuery (for example) and then call the function but I was wondering if I can do it without the helper function. Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. To use a function, you must define it ... Feb 02, 2016 - This is how you can pass functions to other functions in JavaScript. In this case, functionTwo can use functionOne inside of it.
The json_encode() function in PHP provides an easy way to pass an array to JavaScript. Using json_encode() function, you can pass both single dimentional and multidimentional array to the JavaScript function. The following example code shows you how to pass PHP array to JavaScript using json_encode() function. In JavaScript, you cannot pass parameters by reference; that is, if you pass a variable to a function, its value is copied and handed to the function (pass by value). Therefore, the function can’t change the variable. If you need to do so, you must wrap the value of the variable (e.g., in ... Aug 27, 2020 - This tutorial explains how JavaScript pass by value works and gives you some examples of passing primitive and reference variables to a function.
The this keyword cannot be set in JavaScript. It is automatically generated by JavaScript and "always refers to the "owner" of the function we're executing, or rather, to the object that a function is a method of". Passing data is quite important in functional programming languages like JavaScript. When there are multiple functions (which is most of the time), there needs to be a way to pass data between the functions. This is done by passing values in parenthesis: myFunction (myData). Jan 17, 2019 - I have found that it’s better ... to always only pass a single argument called params to my functions, and embed everything in there. Before I try to convince you this is a good idea, let’s take a look at how argument handling works in JavaScript....
If the event is raised by the button, you will not have the text input object to pass to the function without getting it first, getting it by id is the best way. You can use the id of the text box: var searchValue = document.getElementById ('ad_search_query').value; in your function, or use Ahsan Rathod's method to use this code as parameter. Apr 24, 2019 - In order to understand this, you just need two more pieces of information. First, arguments is an Array-like object that represents all of the arguments passed into a function. Second, the apply method works exactly like the call primitive, except that it takes an Array-like object instead ... Pass a Checkbox Value to Javascript Function . Home. Programming Forum . Web Development Forum . Discussion / Question . ajwei810192 10 Junior Poster in Training . 11 Years Ago. Hi, I am a newbie in Ajax, and I am trying to figure out how to pass the value of an item from my HTML form through Javascript/Ajax.
Output: Here, we just take input by statically or dynamically and pass it to JavaScript variable using the assignment operator. The PHP code in the JavaScript block will convert it into the resulting output and then pass it to the variable x and then the value of x is printed. Program 2: This program passes the variables and data from PHP to ... How to pass an array as a function parameter in JavaScript ? Method 1: Using the apply () method: The apply () method is used to call a function with the given arguments as an array or array-like object. It contains two parameters. The this value provides a call to the function and the arguments array contains the array of arguments to be passed. Below is jqeury to call action and pass parameters that you can use in external JS file. var param2 = '@ViewBag.RetrieveObj1'; $ ("#lnkSumbit").bind ( "click", function() { PopulateObject(id, param2); }); Hope this helps. Thanks a lot, yes, I should avoid in line js , I will change all my js in code to jQuery file later.
Send. Bear Bibeault wrote: Or, use the JSP to create a JavaScript object with the same properties. JSON is an easy way to handle that, Yes,I've used JSON to fetch some of the data in my jsp. (e.g. I've used JSON to hold the city names list for a particular statename) Jun 04, 2011 - Your JavaScript code can register a handler function which is triggered when a particular event occurs. Most browsers pass a single object to the function which contains information about the event, i.e. what keys were pressed, the position of the cursor, etc. In order for JavaScript functions to be able to do the same thing with different input, they need a way for programmers to give them input. The difference between parameters and arguments can be confusing at first. Here’s how it works: Parameters are the names you specify in the function ...
Nov 07, 2011 - I thought this would be something I could easily google, but maybe I'm not asking the right question... How do I set whatever "this" refers to in a given javascript function? for example, like with May 31, 2019 - Now, as promised, we are back to ... that is passed as an argument into another function. Clutch your head and weep no more, because there are tricks to be learned — both for identifying callback ‘this’ and, if all else fails, how to just go ahead and force an explicit ‘this’ binding. ... When a function is invoked, the JavaScript interpreter ... How to pass a PHP array to a JavaScript function? Passing PHP Arrays to JavaScript is very easy by using JavaScript Object Notation (JSON). Method 1: Using json_encode () function: The json_encode () function is used to return the JSON representation of a value or array. The function can take both single dimensional and multidimensional arrays.
Mar 29, 2019 - Share Copy sharable link for this gist. Clone via HTTPS Clone with Git or checkout with SVN using the repository’s web address. ... Javascript is extremely flexible, encouraging us to find alternatives to almost any possible expression or statement. For example, passing a callback function to ... The parameters, in a function call, are the function's arguments. JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function. See the Pen JavaScript - Pass a JavaScript function as parameter-function-ex- 28 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous:Write a JavaScript function that returns the longest palindrome in a given string. Next: Write a JavaScript function to get the function name.
Output: Arguments Pass by Value: In a function call the parameters are called as arguments. The pass by value sends the value of variable to the function. It does not sends the address of variable. If the function changes the value of arguments then it does not affect the original value. When you pass an array into a function in JavaScript, it is passed as a reference. Anything you do that alters the array inside the function will also alter the original array. for example this ... Aug 01, 2021 - Functions provide a built-in method bind that allows to fix this. ... The result of func.bind(context) is a special function-like “exotic object”, that is callable as function and transparently passes the call to func setting this=context.
A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when "something" invokes it (calls it). Example. function myFunction (p1, p2) {. return p1 * p2; // The function returns the product of p1 and p2. } Jan 18, 2006 - If you don’t know the method of the object being passed in then you need to pass both the method and the object as parameters and use the call method. call is part of the JavaScript specification and allows a function to run in the context of another object. As a result, the this keyword will ... This is generally not a desired effect and can cause people to use globals and other funky methods of accessing parent objects in anonymous functions. Below I will show you a simple way to access the parent object. Always remember objects in Javascript are passed by reference!
JavaScript Program to Pass a Function as Parameter. In this example, you will learn to write a JavaScript program that will pass a function as a parameter. To understand this example, you should have the knowledge of the following JavaScript programming topics: Function That Wraps Document.Write, Adding a Line Break: 3. setTimeout() with a pointer to a function: 4. Define function in JavaScript: 5. Funciton with arguments: 6. Pass variables to a function, and use these variable values in the function: 7. Function that returns a value: 8. A function with arguments, that returns a value: 9. Oct 30, 2018 - For functions like forEach, map, ... to be passed into their callback functions (e.g. the current value in a collection being iterated over, or the value of a resolved promise), you can take advantage of JavaScript’s ability to use tacit or ‘point-free’ programming. This involves ...
And a very simple JS function is here : function myFunc(id){ alert(id); } You can see in JsFiddle. The problem is : I have no idea, maybe doesn't pass this.id to myFunc function, or some problem else. What's the problem ? Any help would be appreciated. It's very easy to Pass parameter to JavaScript function using the onClick () function. If sending a string value then use double" " or single " quote in the function. 1. Example Pass string parameter in onClick function I have successfully triggered the JavaScript function from code behind using the below statement. protected void btnOK_Click(object sender, EventArgs e) { ScriptManager.RegisterClientScriptBlock(this, GetType(), "MyKey", "myFunction();", true); } But when I tried to pass the values it didn't give me an output.
The JavaScript function resides in a .html page and so I cannot put the coldfusion function toScript() in the same html page. I will have a seperate Coldfusion page that will pass the current server time in a specific format to another .html page. I need to grab current server time when the page loads. Javascript queries related to "pass input value to javascript function" javascript run function passe with parameter; input value into function js So, there are we pass one parameter in the JavaScript function using the Html button onClick function. After that, pass an argument with the onClick event function. Then we implement this function script code in the below section of the body. Secondly, mention in the script tags the function coll and then get parameter.
JavaScript Function and Function Expressions. Javascript setTimeout () The setTimeout () method executes a block of code after the specified time. The method executes the code only once. The commonly used syntax of JavaScript setTimeout is: setTimeout (function, milliseconds); Its parameters are: function - a function containing a block of code. Aug 20, 2016 - This variable is then passed to the function, and modifying it will affect the original object. ... In a strongly typed language, we have to specify the type of parameters in the function declaration, but JavaScript lacks this feature. In JavaScript, it doesn’t matter what type of data or ... Run Next, let's look at an example where a function object is passed around like a regular object. The negate function takes a function as its argument. A new function is returned from the negate call that invokes the passed in function and returns the logical negation of its return value.. Following the function declaration we pass the built-in isNaN function to negate and assign the function ...
Jul 20, 2021 - Note: If this arg is passed to call, bind, or apply on invocation of an arrow function it will be ignored. You can still prepend arguments to the call, but the first argument (thisArg) should be set to null. Mar 23, 2021 - There are naturally trade-offs with this, but I'm glad that the JavaScript specification calls for function arguments to be passed by value rather than reference. And the workaround isn't too much trouble when you have the need (which is pretty rare because mutability makes programs harder ...
Passing Unlimited Parameters Into Javascript Function Parameter Less Function
How To Pass Javascript Objects As Function Arguments By
How To Pass Value From Javascript To Html In Laravel Stack
How To Pass Parameter In Javascript Function From Html Code
Functions Returning Functions In Javascript By Christian
Dynamics 365 Customer Engagement Passing Parameters To
Vaadin 12 Pass Object To Javascript S Function Can T Encode
Can You Pass Crm Parameters Into A Javascript Web Resource
Serverless Node Js Code With Azure Functions Azure
How To Pass Parameter In Javascript Function From Html
Javascript Quiz What Parseint Function Return If Pass String
Mastering This In Javascript Callbacks And Bind Apply
Solved Pass Selected Listelement To Javascript Function
Pass Javascript Session To Server Side
Javascript Pass Function As Parameter Code Example
Javascript Function Pass A Javascript Function As Parameter
Callback Functions In Javascript What Is A Callback Function
How To Pass A String As Argument Javascript Function Stack
0 Response to "20 Pass This To Javascript Function"
Post a Comment