27 Get Type Of Element Javascript
12/9/2019 · The task is to get the type of DOM element by having its object reference. Here we are going to use JavaScript to solve the problem. Approach 1: First take the reference of the DOM object to a variable(Here, In this example an array is made of IDs of the element, then select random ID and select that particular element). The tagName read-only property of the Element interface returns the tag name of the element on which it's called.
Find Element And Findelements In Selenium Differences
There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let's show you each of them separately and point the differences. The first method uses document.getElementById ('textboxId').value to get the value of the box:
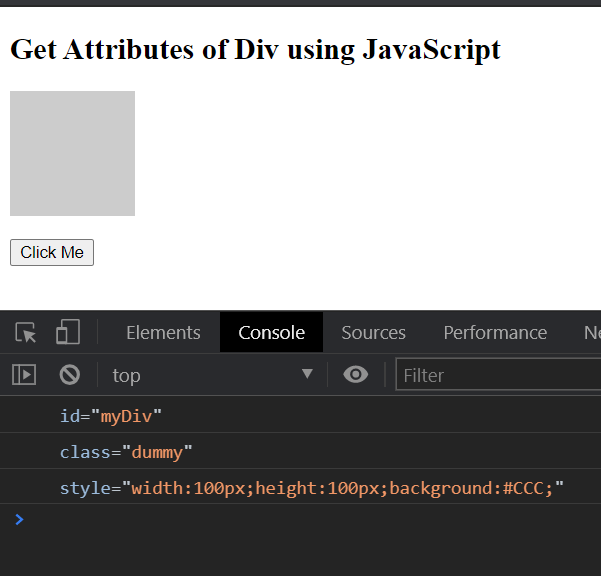
Get type of element javascript. JavaScript HTMLElement.style - To change style of HTML Element programmatically, assign custom style value HTMLElement.style. Example : document.getElementById('idv').style = 'color:#f00;'. Syntax and Try Online Examples are provided to get hands on. In JavaScript, sometimes, you might want to retrieve CSS styles applied to an element through inline styles or external style sheets. There are various ways available to do this, depending on whether you want to fetch inline styles or rendered styles. Libraries like jQuery introduced us to the idea of using CSS selectors to grab elements from the DOM, something that didn't really exist at the time. Eventually, JavaScript started to catch up by expanding beyond getElementById () to allow for selecting elements by way of things like class and tag names. Get Element by ID
JavaScript's method works with the help of the HTML DOM. It is useful in working on manipulating or getting info from the element on to your document. It works in Google Chrome, Internet Explorer, Mozilla Firefox, Safari and Opera browsers. It works by returning the element object which represents an element with help of the specific ID but ... Get a specified element using document.getElementById in javascript, and get a class of elements use document.getElementsByTagName, which can be seen literally by tag name to get a class of elements. If the tag is img, then get all images tags in the page; if the tag name is input, all input elements are got, regardless of whether the type is ... Definition and Usage The getElementsByTagName () method returns a collection of an elements's child elements with the specified tag name, as a NodeList object. The NodeList object represents a collection of nodes. The nodes can be accessed by index numbers.
The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name(s). Just like in selenium web driver, javascript also provides some methods to find the elements: getElementById. getElementsByName. getElementsByClass. getElementsByTagName. Here if you observe carefully, we have only one single method (getElementById) which returns the webelement and the rest are returning the list of webelements. <html> <head> <title>Online Survey</title> <script type="text/javascript" language="javascript"> <!-- // function CheckCheckboxes(){ var elLength = document.MyForm ...
Get and Set Scroll Position of an Element. In JavaScript, it is quite simple and easy to get and set the scroll position of an element of JS and especially when a user is building a user interface in the web browser.As when creating a user interface, we need to have an element that can be scrolled and for which it is required that you know its horizontal and vertical scrolling. Dec 24, 2020 - Help to translate the content of this tutorial to your language! ... DOM navigation properties are great when elements are close to each other. What if they are not? How to get an arbitrary element of the page? Introduction to JavaScript Get Element by Class. Whenever we want to access and get an element present in the DOM of HTML in javascript, we can get the element either based on its id, class or name. There are predefined methods and functions provided to access HTML elements in javascript that are mentioned in the Document interface.
Finishing touches. At this point, you have two options: Keep the script simple, and just get every parent element every time. Provide an option to filter parent elements by a selector (only returning parent elements that have a class of .pick-me, for example.; 1. Jun 17, 2020 - This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ... To get computed styles of an element with JavaScript, we can use the window.getComputedStyle method. For instance, if we have: We get the div with document.querySelector. Then we call window.getComputedStyle with element as the argument. Therefore, the console log should show all the computed CSS properties with their values for the div.
In this tutorial, we are going to find out, that javascript get multiple elements and how to get multiple elements by id using js.Sometimes we need to get the value of the same ID of the multiple elements.But We cannot use the ID to get multiple elements because ID is only used to get the First Element if the same ID is declared to the multiple elements. The querySelector() method of the Element interface returns the first element that is a descendant of the element on which it is invoked that matches the specified group of selectors. tagName A string that specifies the type of element to be created. The nodeName of the created element is initialized with the value of tagName.Don't use qualified names (like "html:a") with this method. When called on an HTML document, createElement() converts tagName to lower case before creating the element. In Firefox, Opera, and Chrome, createElement(null) works like createElement("null").
20/10/2016 · Use the typeof operator to get the type of an object or variable in JavaScript. The typeof operator also returns the object type created with the "new" keyword. As you can see in the above example, the typeof operator returns different types for a literal string and a string object. Map.get () Method in JavaScript The Map.get () method in JavaScript is used for returning a specific element among all the elements which are present in a map. The Map.get () method takes the key of the element to be returned as an argument and returns the element which is associated with the specified key passed as an argument. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties. Property-attribute synchronization When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa. Summary. The firstChild and lastChild return the first and last child of a node, which can be any node type including text node, comment node, and element node.; The firstElementChild and lastElementChild return the first and last child Element node.; The childNodes returns a live NodeList of all child nodes of any node type of a specified node. The children return all child Element nodes of a ... The "nodeType" property. The nodeType property provides one more, "old-fashioned" way to get the "type" of a DOM node. It has a numeric value: elem.nodeType == 1 for element nodes, elem.nodeType == 3 for text nodes, elem.nodeType == 9 for the document object, there are few other values in the specification. For instance:
1 week ago - The getElementsByTagName method of Document interface returns an HTMLCollection of elements with the given tag name. 10/11/2020 · JavaScript type checking is not as strict as other programming languages. Use the typeof operator for detecting types. There are two variants of the typeof operator syntax: typeof and typeof(expression) . JavaScript DOM — Get the children of an element. To get all child nodes of an element, you can use the childNodes property. This property returns a collection of a node's child nodes, as a NodeList object. By default, the nodes in the collection are sorted by their appearance in the source code. You can use a numerical index (start from 0) to ...
Apr 28, 2021 - Note when called on an <input> element, both the tagName or nodeName property returns INPUT, which doesn’t tell whether the input is a text box or a checkbox or a radio button. However, you can use the type attribute for this purpose: ... With jQuery, you can use the .prop() method to get the value of ... The getElementsByName() method of the Document object returns a NodeList Collection of elements with a given name attribute in the document. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Get the first child element in JavaScript HTML DOM. There is another method to get the child element which I am going to discuss now. The HTML DOM firstElementChild property can return the first child element of a specific element that we provide. It doesn't matter how many child elements are there, it will always return the first one. Here's how to get element in a HTML. Get Current Script Element document.currentScript Return the current script element. [see DOM: Get Current Script Element] Get Element by Matching the Value of the "id" Attribute document.getElementById(id_string) Return a non-live element object. Returns null if not found. In the first implementation of JavaScript, JavaScript values were represented as a type tag and a value. The type tag for objects was 0. null was represented as the NULL pointer (0x00 in most platforms). Consequently, null had 0 as type tag, hence the typeof return value "object".
The querySelectorAll definition is similar to getElementsByTagName, except it returns a new type: NodeListOf. This return type is essentially a custom implementation of the standard JavaScript list element. Arguably, replacing Output. _blank. How it works: First, select the link element with the id js using the querySelector () method. Second, get the target attribute of the link by calling the getAttribute () of the selected link element. Third, show the value of the target on the Console window. The following example uses the getAttribute () method to get the value ... Nov 19, 2020 - The typeof statement is really useful in JavaScript for data validation and type checking, but it has some odd features to be aware of. Specifically, there are two instances when typeof returns…
1 week ago - The Document method querySelector() returns the first Element within the document that matches the specified selector, or group of selectors. If no matches are found, null is returned. 13/1/2020 · The JavaScript getElementByName () is a dom method to allows you to select an element by its name. The following syntax to represents the getElementsByName () method: 1 let elements = document.getElementsByName (name);
Javascript Get Element By Id Name Class Tag Value Tuts Make
Using Getelementbyid In Javascript
How To Get All Textboxes In A Form Using Javascript
Tools Qa What Is Dom In Javascript How To Access Dom
How To Get The Class Of A Element Which Has Fired An Event
Methods For Accessing Elements In The Dom File With
Javascript Get Element By Class Searching For Elements
Javascript Dom Tutorial 2 Get Element By Id
How To Get Last Element Of An Array In Javascript
Change An Element S Class With Javascript Add An Element S
How To Get Html Elements In Javascript Simple Examples
Get The Closest Element By Selector
Get The Closest Element By Selector
Is There A Way To Get Element By Xpath Using Javascript In
Vanilla Javascript Get All Elements In A Form
Get All The Elements In A Div With Specific Text As Id Using
Vanilla Javascript Get All Elements In A Form
How To Add And Remove Classes In Vanilla Javascript
Jquery Find Nested Parent Child Elements Html Dom Pakainfo
Manipulating Documents Learn Web Development Mdn
How To Get Css Values In Javascript Zell Liew
How To Get The Type Of Dom Element Using Javascript
Javascript Dom Get The Children Of An Element
Get Class Of Clicked Element Using Javascript
How To Get Attributes Of Div Element In Javascript
Javascript Map How To Use The Js Map Function Array Method
0 Response to "27 Get Type Of Element Javascript"
Post a Comment