27 Merge Two Objects Javascript
Merging of two or more objects using object destructuring Using es6 object destructuring, it is very easy to merge two objects. And of course as soon as I publish the post I find another great use of the spread operator while I tinker with Babel and React: merging multiple objects' properties into one object! The JavaScript. Wrap any objects you'd like merged into one with braces ({}):
Merge Duplicate Array Of Object Key To Single Array Stack
14/6/2020 · In vanilla JavaScript, there are multiple ways available to combine properties of two objects to create a new object. You can use ES6 methods like Object.assign () and spread operator (...) to perform a shallow merge of two objects. For a deeper merge, you can either write a custom function or use Lodash's merge () method.
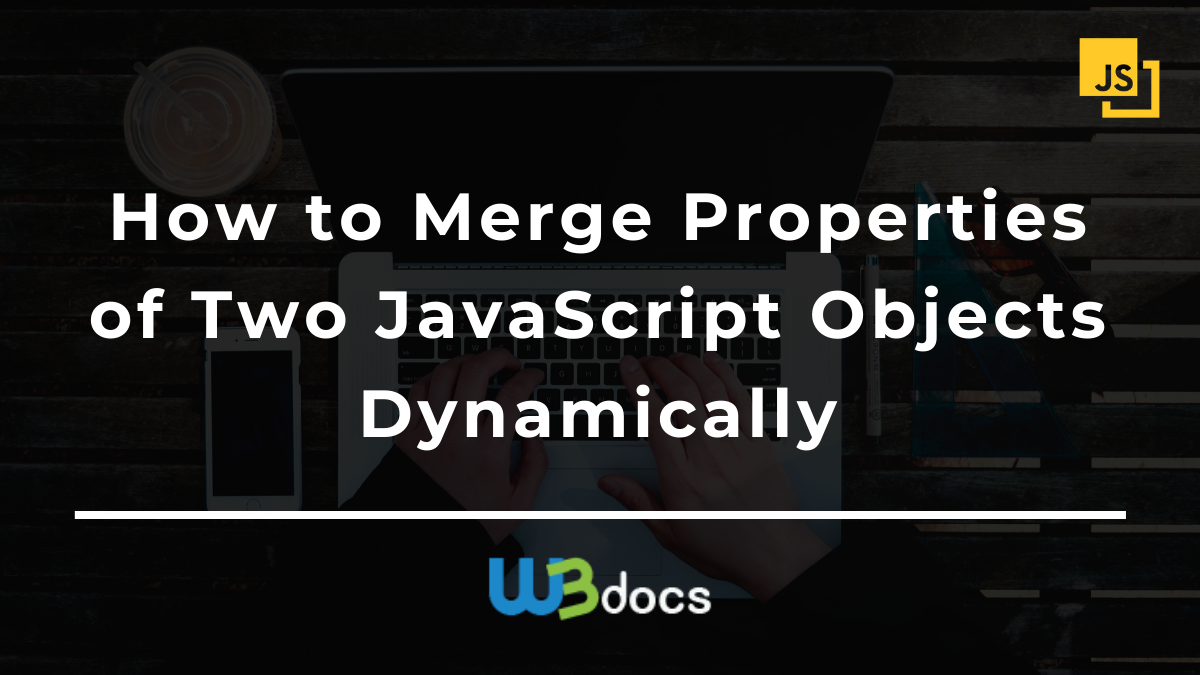
Merge two objects javascript. Jan 17, 2019 - This lesson looks at three methods that can be used to shallow merge two objects in javascript: Object.assign, both with mutating the original object and without mutation, and the spread operator. It also covers the potential danger of doing a shallow merge instead of a deep merge, by showing ... 31/8/2021 · How to merge two objects in JavaScript The easiest way to merge two objects in JavaScript is with the ES6 spread syntax / operator (... Merge JavaScript Objects Using the Spread Operator We can merge different objects into one using the spread operator (...). This is also the most common method of merging two or more objects.
If both objects have a property with the same name, then the second object property overwrites the first. The best solution in this case is to use Lodash and its merge () method, which will perform a deeper merge, recursively merging object properties and arrays. See the documentation for it on the Lodash docs. One thing that I have to do rather often when writing JavaScript code is to combine properties from two objects into a single object. UPDATE: This article was originally called Combining two objects in lodash. I've updated it to cover more ways of combining objects in JavaScript. For example, given these two objects: To know the basics of the Spread operator you can refer Javascript Spread Operator. Just like the Spread operator provides a shorter syntax to merge arrays, it can also be used to merge objects. However only enumerable properties of the object can be copied.
If you are merging two objects that contain other objects or arrays, then you probably want to deeply merge those objects, instead of just shallow merging them. In this lesson, we'll look at three different ways to deeply merge objects, depending on what you want to accomplish: using the spread ... Following is the code to merge two JavaScript objects together −Example Live Demo<!DOCTYPE html> ×. Home. Jobs. Tools. Coding Ground . Current Affairs. UPSC Notes. Online Tutors ... In this tutorial we will learn how to merge two JSON objects using JavaScript. We will assume that we are starting from two JSON strings and, at the end, we want to obtain a single JSON string with the merged objects. Nonetheless, we will do the merging operation over the parsed objects, on their JavaScript representation.
JavaScript code to Merge Multiple Objects. The above code will give the output in the console that you can see below: You can clearly see that the output contains all the value or items from our three objects. That means we are able to merge the three objects into a single one just by using three dots before each object variable. Merge Objects. Merging objects in JavaScript is possible in different ways. Two common approaches are Object.assign() or the … spread operator.. Merge Objects With Object.assign. The outcome of Object.assign() and the … spread operator are the same. Using Object.assign() copies properties of one or many source objects into a target object. The target object is the first one in the ... Now we need to merge the two array of objects into a single array by using id property because id is the same in both array objects. Note: Both arrays should be the same length to get a correct answer.
Mar 05, 2021 - The result of this is exactly the same as the result of Object.assign(). The major difference between the two approaches is control over how the merging occurs (lines 16-18). How to merge properties of two JavaScript Objects dynamically? 0 8 . Online Business Ideas for Aspiring Writing Entrepreneurs . 0 31 . Categories. The Object.assign method or the spread operator lets us shallow merge JavaScript objects. This means that only the first level is merged but the deeper are levels are still referencing the original object. Deep merging ensures that all levels of the objects we merge into another object are copied instead of referencing the original objects.
Feb 22, 2021 - This guide will show you how to merge two or more JavaScript objects into a new object. The new object will contain the properties of all the objects that were merged together. JavaScript provides… The Object.assign () method only copies enumerable and own properties from a source object to a target object. It uses [ [Get]] on the source and [ [Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties. JavaScript program to merge two objects into a single object and adds the values for same keys. We have to write a function that takes in two objects, merges them into a single object, and adds the values for same keys. This has to be done in linear time and constant space, means using at most only one loop and merging the properties in the pre ...
var person={"name":"Billy","age":34}; ... the two object ... const a = { b: 1, c: 2 }; const d = { e: 1, f: 2 }; const ad = { ...a, ...d }; // { b: 1, c: 2, e: 1, f: 2 } ... let obj1 = { foo: 'bar', x: 42 }; let obj2 = { foo: 'baz', y: 13 }; let clonedObj = { ...obj1 }; // Object { foo: "bar", x: 42 } let mergedObj = { ...obj1, ... Using Object.assign As you can see, the location property from the update object has completely replaced the previous location object, so the country property is lost. This is because Object.assign does a shallow merge and not a deep merge. A shallow merge means it will merge properties only at the first level and not the nested level. In JavaScript, it is possible to merge the properties of two or more objects and make a new object by using the spread operator. The spread operator was standardised in ECMAScript 2018 and is represented by ... (three dots) preceding an object. Let's have a look at this in action. Using the Spread Operator
Merge objects using the Lodash Merge method (deep merge) If you want to move a bit further with the JavaScript object merge, you can use the Lodash merge method. This method allows you to deep merge objects together. It means the merge is made recursively, and all values of complex objects are copied. I need to be able to merge two (very simple) JavaScript objects at runtime. For example I'd like to: var obj1 = { food: 'pizza', car: 'ford' } var obj2 = { animal: 'dog' } obj1.merge(obj2); //obj1 now has three properties: food, car, and animal Does anyone have a script for this or know of a built in way to do this? In this tutorial, you'll learn how to merge two objects in JavaScript. You'll use two ways to do it. One using Object.assign and another one using ES6 Spread operator. Merge Two Objects Using Object.assign
// program to merge property of two objects // object 1 const person = { name: 'Jack', age:26 } // object 2 const student = { gender: 'male' } // merge two objects const newObj = Object.assign(person, student); console.log(newObj); Output { name: "Jack", age: 26, gender: "male" } In the above example, two objects are merged into one using the Object.assign() method. Merge the contents of two or more objects together into the first object. The concat method creates a new array consisting of the elements in the object on which it is called, followed in order by, for each argument, the elements of that argument (if the argument is an array) or the argument itself (if the argument is not an array). It does not recurse into nested array arguments. The concat method does not alter this or any of the arrays provided as arguments but ...
There are many ways of merging arrays in JavaScript. We will discuss two problem statements that are commonly encountered in merging arrays: Merge without removing duplicate elements. Merge after removing the duplicate elements. Merge without removing duplicate elements: We will first begin with three arrays and merge them. Later, we will ... There are other libraries available which you can use to merge or two objects like Jquery's $.extend () method $.extend(deep, copyTo, copyFrom) can be used to make a complete deep copy of any array or object in javascript. Lodash merge () method 3 Ways To Merge Objects In Javascript - Simple Examples By W.S. Toh / Tips & Tutorials - Javascript / December 24, 2020 January 30, 2021 Welcome to a tutorial on how to merge or combine two objects together in Javascript.
In this quick tutorial, we'll learn how to combine two or more JSON object into one object in JavaScript. Object.assign() The Object.assign is the most straightforward approach to add two or more objects. 3/1/2020 · Summary: in this tutorial, you will learn how to merge two or more JavaScript objects and create a new object that combines the properties of all the objects. To merge objects into a new one that has all properties of the merged objects, you have two options: Use a spread operator ( ...) Use the Object.assign() method Jul 14, 2019 - There are two methods to merge properties of javascript objects dynamically. They are
May 16, 2020 - Write a shell script that takes two strings as input and determines whether two strings are the anagram. ... as;dlkfja;slkdjf;lakjsdfjhatl;kndflkjatojn ;kojt;oknbsd;lk 0p9tjdn,mvnklizchflkjt52897sd984235jsdfvhuaiwetr kjhfdoiu twhfoah a ... MERN stack implementing Sign in with Google. ... javascript... Jul 06, 2019 - Almost everything in JavaScript is object, but still it does not have any good methods to merge two or more different objects. After ES6 there are new methods added which can be used to merge objects. Merging Two JSON Objects using jQuery. In order to merge the json objects we have to use jQuery's extend () function which takes up multiple parameters. Here is the syntax for the method. jQuery.extend ( [deep], target, object1 [, objectN]) Where 'deep' when set as TRUE, makes the merging process recursive (optional).
Copying Properties From One Object To Another Including
Merging Javascript Objects A Short Guide To Creating A New
2 Ways To Merge Arrays In Javascript Dev Community
How To Merge Two Json Objects Into One Using Jquery
Combine Two Javascript Objects Code Example Amp Live Demo
How To Merge Two Arrays In Javascript
How To Merge A List Of Maps By Key In Immutable Js Pluralsight
Solved Merging Arrays Power Platform Community
How To Merge Amp Concat Objects Using Javascript Object Assign
Merge Two Or More Objects In Javascript Example Codez Up
How To Merge Two Different Object From Json Data Stack Overflow
Merge Two Different Json Files In Python Codespeedy
How To Combine Merge Shapes In Illustrator Makiplace
How Can I Merge These Two Spherical Objects Into A Single
Deep Merge Objects In Javascript With Spread Lodash And
How To Merge Properties Of Two Javascript Objects Dynamically
Javascript Merge Array Of Objects By Key Es6 Reactgo
Javascript Merge Two Objects Code Example
Merge Two Array Of Objects Ui Javascript Codesandbox
3 Ways To Combine Javascript Objects By Corey Butler
Javascript Merge Array Of Objects By Key Es6 Reactgo
How To Merge Two Array Of Objects With Reactjs Stack Overflow
Everything About Javascript Objects By Deepak Gupta
How To Merge Objects In Javascript
How To Merge Two Objects In Javascript Latest Javascript
Javascript Fundamental Es6 Syntax Create A New Object From
0 Response to "27 Merge Two Objects Javascript"
Post a Comment