35 How To Check Integer Value In Javascript
In the above program, the passed value is checked if it is an integer value or a float value. The typeof operator is used to check the data type of the passed value. The isNaN() method checks if the passed value is a number. The Number.isInteger() method is used to check if the number is an integer value. javascript check value is Number; javascript check if type is string and parsable to number; javascript if string is 1; is string in javascript; javascript value is string or object; js check if a string fucntions; if var = string; javascript how to check if a stirng is a nubmer; check if number javascript string; how to determine if an ...
How To Check A String Is Numeric In Javascript Typescript
Use the JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... ExpressionChangedAfterItHasBeenCheckedError: Expression has changed after it was checked. Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'.
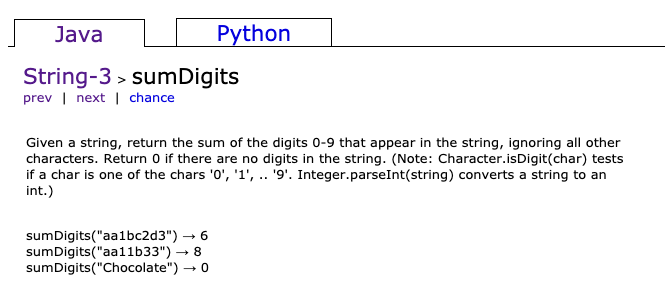
How to check integer value in javascript. Oct 03, 2020 - Let’s say we have the following variables −var value1 = 10; var value2 = 10.15;Use the Number() condition to check that a number is float or integer −Numb ... Javascript isInteger () JavaScript isInteger () is an inbuilt method that resolves whether the passed value is an integer. The isInteger () method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise, it returns False. May 21, 2020 - Get code examples like "js how to check if number is integer" instantly right from your google search results with the Grepper Chrome Extension.
Numbers can be positive or negative integers. However, integers are floating-point values in JavaScript. Integers value will be accurate up to 15 digits in JavaScript. Integers with 16 digits onwards will be changed and rounded up or down; therefore, use BigInt for integers larger than 15 digits. Number.isInteger () Method In JavaScript Number.isInteger () method works with numbers, it will check whether a value an integer. This method returns true if the value is of the type Number and an integer. Otherwise, it will return false. javaScript check if variable is a number: isNaN () JavaScript - isNaN Stands for "is Not a Number", if a variable or given value is not a number, it returns true, otherwise it will return false. typeof JavaScript - If a variable or given value is a number, it will return a string named "number". In JavaScript, the typeof operator ...
A value in JavaScript can be primitive such as a number or string. Or it can be an object. This tutorial shows you to check if an array contain a value, being a primtive value or object. 1) Check if an array contains a string. To check if an array contains a primitive value, you can use the array method like array.includes() The following ... Jan 18, 2020 - JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... how to write a function that checks the number of strings in the string and returns it in javascript Write a javascript function named is_integer which checks if the passed argument is an integer. You can use any mathematical operator or functions defined in the Math object. ... Write a function that takes in an input and returns true if it's an integer and false otherwise. ... Use the JavaScript documentation to ...
There's lots of websites doing this wrong. Here's how to do it right. JavaScript exercises, practice and solution: Write a JavaScript program to check whether two given integer values are in the range 50..99 (inclusive). Return true if either of them are in the said range. 15/10/2019 · Getting the Number Rounded Downwards to the Nearest Integer. The Math.floor() method rounds the number downwards to the next integer (having lower value). Going by the name it returns the "floor" integer of a number. // 11 Math.floor(11.25) // 11 Math.floor(11.99) // -12 // -12 has lower value than -11.25 Math.floor(-11.25) // 11 Math.floor(11)
How to check if an R matrix column contains only duplicate values? Check if object contains all keys in JavaScript array; How to check if a variable is an integer in JavaScript? Java Program to Check if An Array Contains a Given Value; Check if the given array contains all the divisors of some integer in Python The MAX_SAFE_INTEGER constant has a value of 9007199254740991 (9,007,199,254,740,991 or ~9 quadrillion). The reasoning behind that number is that JavaScript uses double-precision floating-point format numbers as specified in IEEE 754 and can only safely represent integers between - (2^53 - 1) and 2^53 - 1. Safe in this context refers to the ... Another method is document.getElementsByName ('name') [wholeNumber].value which returns a live NodeList which is a collection of nodes. It includes any HTM/XML element, and text content of a element: Use the powerful document.querySelector ('selector').value which uses a CSS selector to select the element:
JavaScript Numbers are Always 64-bit Floating Point. Unlike many other programming languages, JavaScript does not define different types of numbers, like integers, short, long, floating-point etc. JavaScript numbers are always stored as double precision floating point numbers, following the international IEEE 754 standard. August 7th, 2020. JavaScript. In JavaScript, there are different ways to check whether a value is a number or something else. The most common way is to use the typeof operator: const value = 5 console.log(typeof value) One way you can use it in a practical context is to check if a form is filled out correctly, by using typeof in a conditional ... 31/1/2013 · To check if integer like poster wants: if (+data===parseInt (data)) {return true} else {return false} notice + in front of data (converts string to number), and === for exact. Here are examples: data=10 +data===parseInt (data) true data="10" +data===parseInt (data) true data="10.2" +data===parseInt (data) false.
@HunanRostomyan Good question, and honestly, no, I do not think that there is. You are most likely safe enough using variable === null which I just tested here in this JSFiddle.The reason I also used ` && typeof variable === 'object'` was not only to illustrate the interesting fact that a null value is a typeof object, but also to keep with the flow of the other checks. In this chapter, you will learn about how to check variable is an integer or not in Javascript., here I am listing the possible ways to check if the value is an integer or not. Solution 1: Number.isInteger (): To check if the variable is an integer or not, use the method Number.isInteger (), which determines whether the passed value is an integer. Feb 26, 2020 - Javascript function to check if a field in a html form contains all numbers or not.
To convert a string to an integer parseInt() function is used in javascript.parseInt() function returns Nan( not a number) when the string doesn't contain number.If a string with a number is sent then only that number will be returned as the output. This function won't accept spaces. If any particular number with spaces is sent then the part of the number that presents before space will be ... Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function to check whether a value is an integer or not. In JavaScript, there are two ways to check if a variable is a number : isNaN () - Stands for "is Not a Number", if variable is not a number, it return true, else return false. typeof - If variable is a number, it will returns a string named "number".
The Number.isInteger () method in JavaScript is used to check whether the value passed to it is an integer or not. It returns true if the passed value is an integer, otherwise, it returns false. If isNaN () returns false, the value is a number. Another way is to use the typeof operator. It returns the 'number' string if you use it on a number value: typeof 1 //'number' const value = 2 typeof value //'number' The Number.isInteger () method determines whether a value an integer. This method returns true if the value is of the type Number, and an integer (a number without decimals). Otherwise it returns false.
Nov 19, 2020 - Infinity is indeed a value in JavaScript, representing mathematical infinity (∞). Be prepared for when it pops ups in… ... To check if variable A is an integer I could use the loose equality operator == to see if the parsed value equals itself. Mar 30, 2020 - Get code examples like "how to check number is integer or not in javascript" instantly right from your google search results with the Grepper Chrome Extension. If the target value is an integer, return true, otherwise return false. If the value is NaN or Infinity, return false. The method will also return true for floating point numbers that can be represented as integer.
The isNaN () function determines whether a value is an illegal number (Not-a-Number). This function returns true if the value equates to NaN. Otherwise it returns false. This function is different from the Number specific Number.isNaN () method. The global isNaN () function, converts the tested value to a Number, then tests it. Aug 25, 2020 - JavaScript documentation to find a method on the built-in Number object that checks if a number is an integer. ... how to write a function that checks the number of strings in the string and returns it in javascript The return value of text input field is string. First try parseInt () to convert the value to integer. If the type casting fails, it parseInt () will return NaN, so we can use isNaN () to check the result of conversion. The following is demo of above idea:
26/5/2014 · Checking via Math.round () #. A number is an integer if it remains the same after being rounded to the “closest” integer. Implemented as a check in JavaScript, via Math.round (): function isInteger(x) { return Math.round (x) === x; } This function works as it should: > isInteger (17) true > isInteger (17.13) false. It's a string. The point of this function is to test whether a value is a Javascript numeric value that has no fractional part and is within the size limits of what can be represented as an exact integer. If you want to check a string to see if it contains a sequence of characters that represent a number, you'd call parseFloat()first. In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf() method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.. indexOf() Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf() method.
Javascript Validate Float Number Html Cod Example Eyehunts
Javascript Basic Check Whether Two Given Integer Values Are
How To Convert Integer To Binary In Javascript Spursclick
Count The Number Of Digits In C Javatpoint
How To Check Whether A Value Is Numeric Or Not Using Jquery
Myfib Write A Javascript Function Using Recursion To Chegg Com
How To Filter Out Only Numbers In An Array Using Javascript
How To Check Whether A Value Is Numeric Or Not Using Jquery
Java Program To Check If Given Number Is Perfect Square
Python Check Integer Number In Range Using Multiple Ways
Python Program To Check If A Number Is Odd Or Even
Set Validation Rules For Your Schema Mongodb Compass
The Problem Of A Parseint Function Found By Javascript Random
Javascript Math Check Whether A Value Is An Integer Or Not
Number Truncation In Javascript Samanthaming Com
5 Ways To Convert A Value To String In Javascript By
Jquery Allow Numbers And Decimal Only In Textbox Numeric
Javascript Numbers Get Skilled In The Implementation Of Its
How To Convert A String To A Number In Javascript
Parseint 08 Returns 0 With Javascript The Electric
Check If Variable Is A Number In Javascript Mkyong Com
Javascript Basic Check Two Given Integers Whether One Is
Javascript How To Sum Two Numbers Code Example
Apache Jmeter User S Manual Functions And Variables
How To Check For A Number In Javascript By Dr Derek Austin
How To Check For A Number In Javascript By Dr Derek Austin
Data Types In Power Bi Desktop Power Bi Microsoft Docs
Javascript Typed Arrays Javascript Mdn
Javascript Check If A Number Is An Integer Code Example
How To Convert Strings To Numbers Using Javascript
Javascript Limit Integer To Min Amp Max Using One Line Of Code
Why 1 7 11 Map Parseint Returns 1 Nan 3 In
Number Isinteger Javascript Method Check Var Value Is An
Program To Count Digits In An Integer 4 Different Methods
0 Response to "35 How To Check Integer Value In Javascript"
Post a Comment