34 Javascript Array Of Fixed Size
Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the array, JavaScript arrays are not guaranteed to be dense; this depends on how the programmer chooses to use them. Mar 01, 2017 - Most of the tutorials that I've read on arrays in JavaScript (including w3schools and devguru) suggest that you can initialize an array with a certain length by passing an integer to the Array
Numpy Array Object Exercises Practice Solution W3resource
Typescript types for fixed size arrays. Contribute to mstn/fixed-size-array development by creating an account on GitHub.
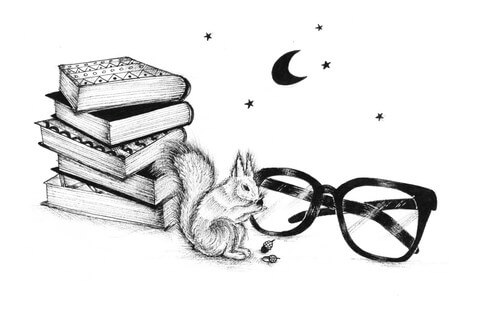
Javascript array of fixed size. Dec 21, 2016 - in javascript how would I create an empty array of a given size Psuedo code: X = 3; createarray(myarray, X, ""); output: myarray = ["","",""] In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable that stores multiple elements. Declaration of an Array Javascript Web Development Front End Technology The array is a container which can hold a fixed number of items and these items should be of the same type. It stores a fixed-size sequential collection of elements of the same type.
// Create the fixed queue array value. var queue = Array. apply (null, initialValues); // Store the fixed size in the queue. queue. fixedSize = size; // Add the class methods to the queue. Some of these have // to override the native Array methods in order to make // sure the queue lenght is maintained. queue. push = FixedQueue. push; queue. splice = FixedQueue. splice; > Array.from({length: 3}) [ undefined, undefined, undefined ] The parameter {length: 3} is an Array-like object with length 3 that contains only holes. It is also possible to instead use new Array(3), but that usually creates larger objects. Spreading into Arrays only works for iterable values and has a similar effect to Array.from(): Using Array literal notation if you put a number in the square brackets it will return the number while using new Array () if you pass a number to the constructor, you will get an array of that length. you call the Array () constructor with two or more arguments, the arguments will create the array elements.
Array is a fixed size data structure while ArrayList is not. One need not to mention the size of Arraylist while creating its object. Even if we specify some initial capacity, we can add more elements. // A Java program to demonstrate differences between array javascript create empty array of a given size, The length property of an object which is an instance of type Array sets or JavaScript Demo: Array.length Create empty array of fixed length. The parameter {length: 3} is an Array-like object with length 3 that contains only holes. Cloning Arrays: The Challenge. In JavaScript, arrays and objects are reference types. This means that when a variable is assigned an array or object, what gets assigned to the variable is a reference to the location in memory where the array or object was stored.
C# | declaring a fixed size array: Here, we are going to learn how to declare, initialize, input and print a fixed size array in C#? Submitted by IncludeHelp, on March 30, 2019 . In C#, an array can be declared with a fixed size length and a variable length. Sometimes it's required that we need to have an array of fixed length. Under the hood, array-backed data structures are used and engines use heuristics to figure out which implementation to use. So, if you create an array and set its millionth value to something, you don't actually create an array of that size. Instead, a sparse array of some sort will be used. See the Pen JavaScript - Get all possible subset with a fixed length combinations in an array-function-ex- 21 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function that generates a string id (specified length) of random characters.
JavaScript arrays, as implemented in modern browsers, are complicated beasts. There are sparse arrays and dense arrays: * The sparse ones are dynamically-resizable hash tables, with a fill level; when collisions are starting to become a problem, a... It is not possible to create a native javascript Array with a fixed length. But, you can create an object which will behave like a fixed-length Array. Following the suggestions in the comments, I've come up with 2 possible implementations, both with pros and cons. I haven't figured out which of the 2 I'm going to use in my project yet. Previous JavaScript Array Reference Next ... array.length = number. Technical Details. Return Value: A Number, representing the number of elements in the array object: JavaScript Version: ECMAScript 1: Related Pages. Array Tutorial. Array Const. Array Methods.
Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript JavaScript array length property returns an unsigned, 32-bit integer that specifies the number of elements in an array. An array is a fixed size in memory. If you need to grow it or add a single item to it, then you need to create a new array and copy all the values over from the old array. This sounds like a lot of work, however, PowerShell hides the complexity of creating the new array.
Definition and Usage. The toFixed() method converts a number into a string, rounding to a specified number of decimals. Note: if the desired number of decimals are higher than the actual number, zeros are added to create the desired decimal length. A dynamic array is a random access, variable-size list data structure that allows elements to be added or removed. It is supplied with standard libraries in many modern programming languages. Dynamic arrays overcome a limit of static arrays, which have a fixed capacity that needs to be specified ... Here are the TLDR common methods of defining arrays in TypeScript. [string] = Tuple (fixed size array) string [] = Array (most common array) Array = Array (same as the 2nd but preferred if you need different types in the array)
This is a more concise approach to chunking (grouping) the elements of an array. Here, we use the.splice () method to extract elements of the specified size from the array that is received. The **.splice ()** method is used to modify the content of an array by removing or replacing elements. Each element in a JavaScript array can itself be an array giving the equivalent of a multi-dimensional array but the lengths of the arrays iyou add for the second dimension are not forced to be the... An array in JavaScript is a type of global object that is used to store data. Arrays consist of an ordered collection or list containing zero or more data types, ... We can find out how many items are in an array with the length property. seaCreatures.length; Output. 5
Jul 20, 2016 - when using shift and push there ... or end of array before add or reject new item .. my fastest solution is that. if using just key for reaching array can be easy to control and expect fixed size behaviour. Both object and array can be used. ... Not the answer you're looking for? Browse other questions tagged javascript arrays or ... Nov 02, 2015 - Are they fixed arrays that are reinitialized? I know it doesn't matter but I'm starting to get curious about these under the hood stuff. And yeah, if you couldn't tell. ... No and no. All JavaScript objects are collections of key/value pairs called properties. 25/2/2021 · This approach of dividing an array into smaller chunks assumes a fixed chunk size. For example, we want to split the array [1, 2, 3, 4, 5, 6, 7, 8] into chunks of three items. The result of chunking the source array should look like this: [ [1, 2, 3], [4, 5, 6], [7, 8] ] JavaScript comes with the Array#splice method.
The fill() method changes all elements in an array to a static value, from a start index (default 0) to an end index (default array.length). It returns the modified array. The solution is using the memory keyword:. uint8[4][2] memory myArr; myArr will then return the default value uint8(0) for all indices.. It should also be noted, that in Solidity array size is defined in reverse lookup order. The last number in myArr would thus be uint[1][3].. Also, in memory Arrays, uint8 may actually be more costly than just using uint256, since the latter is used internally. Arrays in JavaScript enables multiple values to be stored in a single variable. It stores the same kind of element collection sequential fixed-size. Arrays in JavaScript are used to store an information set, but it is often more helpful for storing a set of variables of the same type.
In the first call, current_item will be the second item and partial_result will be the first item in the list. This is very convenient for some cases, including in our example. Javascript provides 2 reducer function: reduce () and reduceRight () - which iterates the elements starting from the end of the array -. 1. 13/12/2019 · My Objective is to create a fixed column array object of size 4 columns so that I can form a 2d array in the following format which I can use to print to a pdf later. The solution is working fine. But need help in figuring out if there is a better way to implement this. let input = [ { id:123, quantity: 4, value: "xxxx" }, { id:234, quantity: ... JavaScript fundamental (ES6 Syntax): Exercise-53 with Solution. Write a JavaScript program to Initialize a two dimension array of given width and height and value. Use Array.from() and Array.prototype.map() to generate h rows where each is a new array of size w. Use Array.prototype.fill() to initialize all items with value val.
Get code examples like "lodash fixed length array" instantly right from your google search results with the Grepper Chrome Extension. You could of course feature-test it: ... The accepted answer shows how this issue can now be solved using Object.seal which wasn't available at the time. ... So, it seems that the answer to the original question is simply 'No'. It is not possible to create a native javascript Array with a fixed ... Jul 28, 2019 - by Glad Chinda Hacks for Creating JavaScript ArraysInsightful tips for creating and cloning arrays in JavaScript.Original Photo by Markus Spiske on UnsplashA very important aspect of every programming language is the data types and structures available in the language.
Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the ... Jul 20, 2021 - The length property of an object which is an instance of type Array sets or returns the number of elements in that array. The value is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array. May 22, 2017 - JavaScript arrays are not fixed-size, so you can usually get away with not preallocating them. What have you tried? – Matt Ball Dec 10 '12 at 20:08
Mar 27, 2019 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. In many a cases we will not want to create the array with a fixed size or length. In such cases we can create an array with out passing length. This array will dynamically set its value as and when a new variable or entry is added. If start is negative, it is treated as array.length + start.; If end is negative, it is treated as array.length + end.; fill is intentionally generic: it does not require that its this value be an Array object.; fill is a mutator method: it will change the array itself and return it, not a copy of it.; If the first parameter is an object, each slot in the array will reference that object.
May 21, 2021 - You may think that it is correct "semantics", and it is. How else would you create an array of X length? The catch here is, that I've never needed to define a size in an array, since Javascript doesn't need to allocate memory for an array. They are more like...
Github Mstn Fixed Size Array Typescript Types For Fixed
Dynamic Array Data Structure Interview Cake
How Do Dynamic Arrays Work Geeksforgeeks
How To Replace An Element In Array In Java Code Example
Urgent Need Help Immediatelythis Is My Code Chegg Com
Data Structures In Javascript Arrays Hashmaps And Lists
How To Work With Jagged Arrays In C Infoworld
Github Mbrevoort Tailable Capped Array A Simple Fixed
Javascript Array A Complete Guide For Beginners Dataflair
Tools Qa Array In Javascript And Common Operations On
How To Define Js Fixed Array Size Stack Overflow
6 Javascript Data Structures You Must Know
Difference Between Length Of Array And Size Of Arraylist In
Serious Impact Slice In Golang For Speed And Memory Usage
Data Structures Objects And Arrays Eloquent Javascript
Javascript Array A Complete Guide For Beginners Dataflair
Vb Net Arrays String Dynamic With Examples
Javascript Array Fun Rick Strahl S Web Log
Bangle Js Creating A Smart Watch With Javascript
Javascript Api Frida A World Class Dynamic
Loops Amp Arrays Chapter Ppt Download
Working With Arrays In Javascript
Project Zero Trashing The Flow Of Data
How To Create Dynamic Length Array With Numbers And Sum The
Javascript Array A Complete Guide For Beginners Dataflair
How Do I Empty An Array In Javascript Stack Overflow
Vectors In Javascript Learn How A Vector Can Be Created In
Top 50 Array Interview Questions Amp Answers 2021 Update
Javascript Array Length Get Number Of Elements In Array
Hacks For Creating Javascript Arrays
C Vector Vs Array Learn The 8 Important Differences
0 Response to "34 Javascript Array Of Fixed Size"
Post a Comment