32 Javascript Import Json File
TL:DR If you want to do a dynamic import of JSON with Webpack be sure to account for a JSON file not having a default export when you're trying to extract the value and call default on the returned module to return the whole JSON object. The JSON Format Evaluates to JavaScript Objects The JSON format is syntactically identical to the code for creating JavaScript objects. Because of this similarity, a JavaScript program can easily convert JSON data into native JavaScript objects.
Import Json File In React Webpack Config Error Stack Overflow
json is the only module type currently supported by import assertions. But there are plans to implement also CSS and HTML modules. Previous way of importing JSONs into JavaScript modules Before...
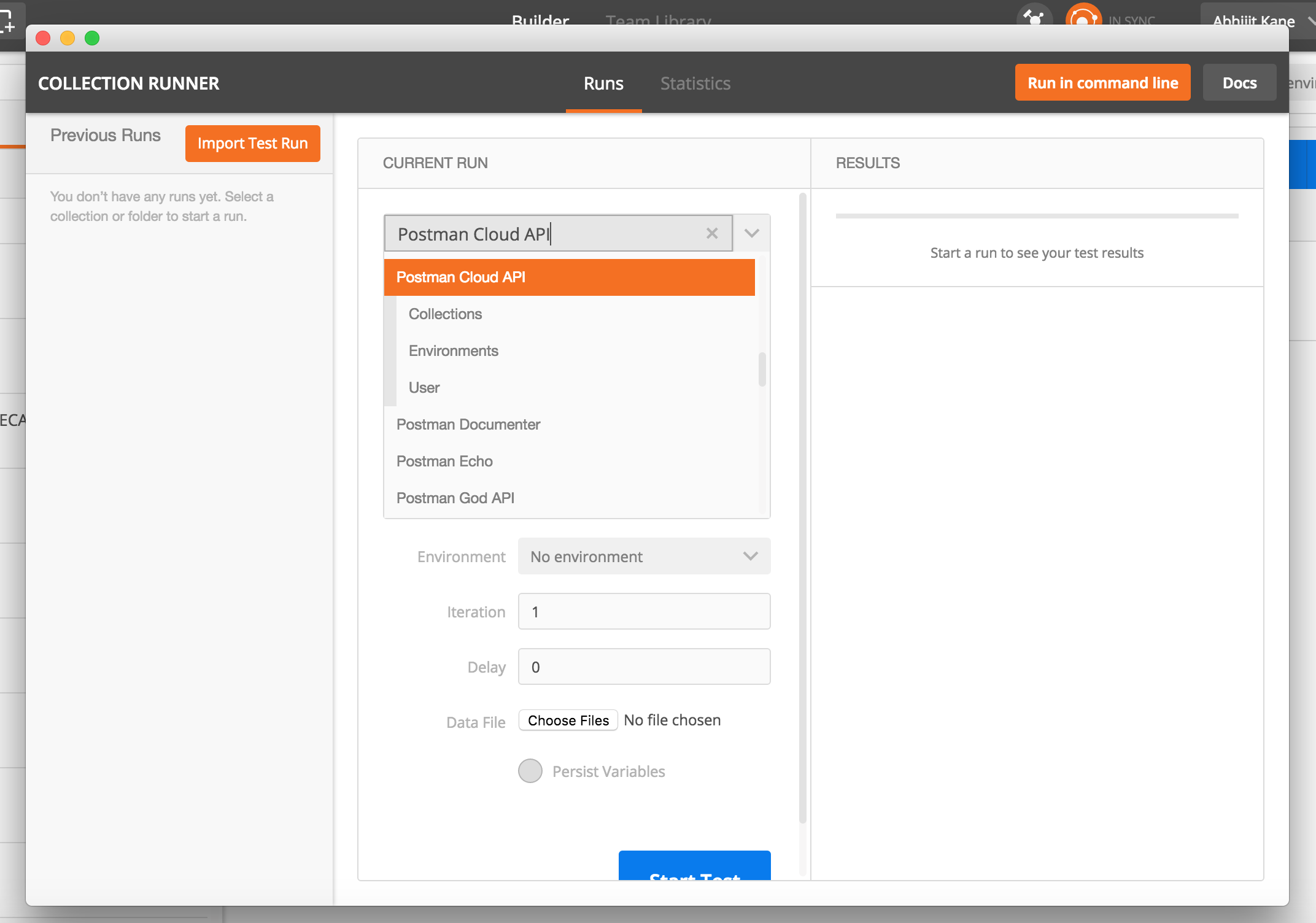
Javascript import json file. Example. Assuming we have following JSON content in names.txt file with json format content: Following is a simple example of a simple showing the usage of this method. And Save this text file as JSONExample.html. Click on the button to load result.html file: 28/11/2016 · [UPDATE] Solution: Typescript 2.9 supports JSON import! If you are using Typescript version 2.9, you don’t need to follow solution 2. Here is how you can do it: In your `tsconfig.json` file, under compiler options, add these two lines: Jun 21, 2020 - // example.json { "name": "testing" } // ES6/ES2015 // app.js import * as data from './example.json'; const {name} = data; console.log(name); // output 'testing' ... Error during serialization or deserialization using the JSON JavaScriptSerializer. The length of the string exceeds the value ...
Here we are fetching our people.json file. After the file has been read from disk, we run the then function with the response as a parameter. To get the JSON data from the response, we execute the json() function. The json() function also returns a promise. This is why we just return it and chain another then function. Jul 29, 2021 - Importing our Own Files: The require function can also be used to load our own JavaScript files. We have to provide a relative path to the file that we want to load script. ... Writing and reading JSON file: JavaScript provides two methods for working with JSON. Jun 16, 2021 - The Fetch API, an API based on Promises for downloading files in JavaScript code (line B and line C). The module metadata property import.meta.url (line A). fetch() has two downsides compared to JSON modules:
Option 1: Read and parse JSON files yourself. The Node.js documentation advises to use the fs module and do the work of reading the files and parsing it yourself. import { readFile } from 'fs/promises'; const json = JSON.parse( await readFile( new URL('./some-file.json', import.meta.url) ) ); 22/2/2021 · Method 2: Using ES6 import module (Web Runtime Environment only) If we want to access the json file while running JavaScript in browser, we can use the ES6 import syntax to do that. You can import json files into your js like this import data from './filename.json'.
resolveJsonModule was introduced in typescript 2.9. It allows importing JSON files directly in a typescript file. Once you have added this flag, you can import JSON files in any typescript file in the project like below : import * as data from './data.json'; The first sentence of the MDN docs plainly says import "..is used to import features into JavaScript modules that have been exported by another module." A JSON file is not a module. Additionally, under the covers, webpack's json-loader converts a json file to a proper export. This morning, a student of mine asked me how to use JavaScript to import a JSON file and do something with the data in it. I thought I'd write an article about it, because it's a really cool browser feature! Let's dig in. An example For this article, we're going to look at a form element with the #upload ID. It contains a single field, #file, with a type of file.
Jul 01, 2019 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. Automatically Reading a JSON file The code which can be used for making Node.js automatically read the JSON file is shown below: var obj = require ("../path/jsonfile.json"); Here, Node.js will parse the content to a particular JSON object and assign the same to the variable placed on the left hand side. Unfortunately ES6/ES2015 doesn't support loading JSON via the module import syntax. But... There are many ways you can do it. Depending on your needs you can either look into how to read files in JavaScript (window.FileReader could be an option if you're running in the browser) or use some ...
js. In the webpack config file, the loader rule accepts the NPM package to load any of the JSON files in your app. One package used is called json5-loader. Along with the loader rule, there are two other rules, one of which is test. 1 test: /\.json5$/i, js. That identifies the file format of the JSON or gives a warning at the time of compilation. Mar 22, 2017 - Access local JSON data with Javascript. GitHub Gist: instantly share code, notes, and snippets. Jun 06, 2021 - To import and load a JSON file in an ES module, you have to do a "window.fetch dance" to request the file and parse it yourself. It's not great... But there's hope! According to chromestatus , JSON Modules are shipping in Chrome 91. I gave it a quick try, and surprisingly, the following JavaScript ...
One nice way (without adding a fake.js extension which is for code not for data and configs) is to use json-loadermodule. If you have used create-react-appto scaffold your project, the module is already included, you just need to import your json: import Profile from './components/profile'; In this tutorial, we will see how to Load JSON file locally using pure Javascript. the proper way to include or load the JSON file in a web application, Instead of using JQuery we'll The correct method using XMLHttpRequest JavaScript Alternative To jQuery's Having a pure javascript alternative to jQuery's $.getJSON () and $.parseJSON (). How to import local json file data to my JavaScript variable? Javascript Web Development Object Oriented Programming We have an employee.json file in a directory, within the same directory we have a js file, in which we want to import the content of the json file.
<DATABASE> and <COLLECTION> refer to the name of the database and the collection, into which you want to import the JSON file . Finally, <FILENAME> is the full path and name of the JSON file you wish to import. You can even import various other file formats such as TSV or CSV using mongoimport. For ES6/ES2015 you can import directly like: // example.json { "name": "testing" } // ES6/ES2015 // app.js import * as data from './example.json'; const {name} = data; console.log(name); // output 'testing' If you use Typescript, you may declare json module like: Nov 18, 2020 - Bind Json file into a Datagridview in windows form using C# ... npx node-minify --silence --compressor jsonminify --input './json/*.json' --output './json/$1.min.json'`
JSON is a file format widely used for static storage and app config management with any of the frameworks and data servers. Any JSON file contains the key-value pair separated by the comma operator. JavaScript objects are an integral part of the React app, so they need to get accessed from JSON files/data to be uses in components. I am assuming 'without using Ajax' you mean by a local json data in your system. If that is the case you can easily import the local json data file into your HTML file by just including the json file in your head tag e.g. [code]<script type="text/... Apr 30, 2020 - dynamic import are Javascript Module - Import (Es Module) that are defined at runtime Doc: Type Json Example with: The whole Json file Return the whole object Return only a sub-part Typescript. If the import is really dynamic, typescript will complain and you need to
JSON Format JSON's format is derived from JavaScript object syntax, but it is entirely text-based. It is a key-value data format that is typically rendered in curly braces. When you're working with JSON, you'll likely see JSON objects in a.json file, but they can also exist as a JSON object or string within the context of a program. You can't mix CommonJS require and ES6 import in the same file (at least not easily), so if you're using ES6 import and wish to read a file, do so with fs.readFileSync or a similar method. If the file is a json string, you'll need to parse it with JSON.parse(). Jun 13, 2018 - How can I access a JSON file in ECMAScript 6? The following doesn't work: import config from '../config.json' This works fine if I try to import a JavaScript file.
Importing data from JSON files. JSON stands for JavaScript Object Notation and is one of the most commonly used formats for data exchange between different platforms and applications. With Power BI, you can export data from JSON files to create different types of visualization. To import JSON files, go to the Power BI dashboard and click the ... defaultExport. Name that will refer to the default export from the module. module-name. The module to import from. This is often a relative or absolute path name to the .js file containing the module. Certain bundlers may permit or require the use of the extension; check your environment. You cannot load a file with JSON.parse (). This function is only able to convert an existing string into an object (if content is in valid JSON format).
As long as you are dealing with Next.js and JSON files, then this post is for you. What we will do in this post is the following: We are going to load a JSON file to be used with Next.js. Based on its content, we will manually extend static pages and create pages completely dynamically. Thus, we will create a dynamic route handler and navigation. Export default. In practice, there are mainly two kinds of modules. Modules that contain a library, pack of functions, like say.js above.; Modules that declare a single entity, e.g. a module user.js exports only class User.; Mostly, the second approach is preferred, so that every "thing" resides in its own module. the JSON file and print the data in JavaScript? I have saved a JSON file in my local system and created a JavaScript file in order to read the ... 83684/how-to-read-an-external-local-json-file-in-javascript
Feb 01, 2021 - Import JSON from a local file and assign to variable in JavaScript. We can use require statements to import a JSON from a file to a variable. May 16, 2021 - Learn about the two ways to lead JSON files in Node.js ECMAscript modules (esm).
Read Locally Json File Use Fetch Method In Javascript By
Using External Js Files In Angular Franco Morales
Import Json File In Typescript 2 9 Issue 963 Aurelia
Ansible Read Json File Json File Parsing Devops Junction
Importing Data From Json Files And Power Bi Rest Apis Into
Import Json To Mysql Made Easy With The Mysql Shell Mysql
Import Json Data Into Sql Server
Json Data Shows In Webpack Server But Not Production Build
How Can I Select Data From Local Json File With Axios Api
Vanilla Javascript How To Read Local Json File Stack Overflow
Python Json Encode Dumps Decode Loads Amp Read Json File
How To Use An Es6 Import In Node Js Geeksforgeeks
Importing Data From Json Files And Power Bi Rest Apis Into
How To Import Data From A Json File And Parse It Javascript
Jquery Import Json File Code Example
Read Json File Using Python Geeksforgeeks
Viscourse Classic Import Export Mechanisms Through Editable
How To Fetch Data From Json File And Display In Html Table
How To Parse Custom Json Data Using Excel The Excel Club
How To Import Json Into Javascript Module Json Modules By
Import Local Json In Node Js V8 5 Experimental Modules Dev
How To Read A Json File With Javascript
Convert Text File To Json In Python Geeksforgeeks
Zabbix 5 2 How To Import Local Json File To Javascript
How To Import Json Files In Es Modules Node Js Dev Community
How To Read Local Json File In React Js By Rajdeep Singh
How To Read And Write Json File Using Node Js Geeksforgeeks
Using Csv And Json Data Files In The Postman Collection
Loading Json File With Es6 Import To Browser Issue 239
0 Response to "32 Javascript Import Json File"
Post a Comment