32 Concatenate A String In Javascript
JavaScript's join () method is handy for turning elements in an array into a string. JavaScript arrays can contain values of different types. If you only want to concatenate strings, you can filter out non-string values using filter () and typeof as shown below. Name Array.join( ): concatenate array elements to form a string — ECMAScript v1 Synopsis array.join( ) array.join(separator) Arguments separator An optional character or string used … - Selection from JavaScript: The Definitive Guide, 5th Edition [Book]
Javascript Concatenate String And Variable Simple Example Code
JavaScript String - concat() Method, This method adds two or more strings and returns a new single string.
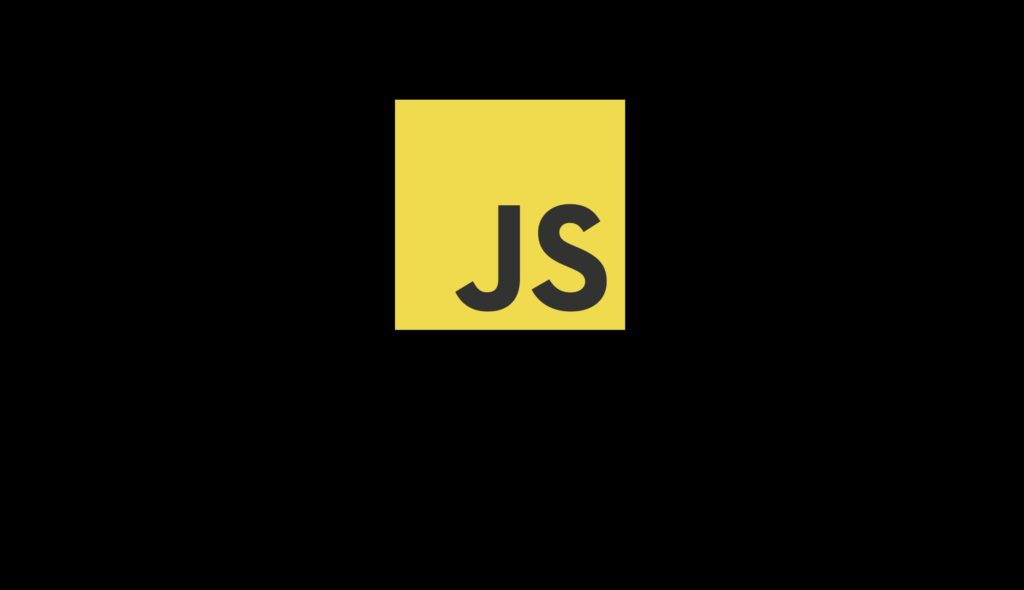
Concatenate a string in javascript. Introduction to the JavaScript String concat () method. The String.prototype.concat () method accepts a list of strings and returns a new string that contains the combined strings: If the arguments are not strings, the concat () converts them to strings before carrying the concatenation. It's recommended that you use the + or += operator for ... This method does not alter the input/given strings, instead, it returns a new string that has both the input strings concatenated. Syntax: ... Here, the parameters string1, string2, ..., stringN are the strings that to be concatenated. Example: var str1 = "Example of "; var str2 = "Javascript "; ... JavaScript offers us three different ways to concatenate or joining strings. The first way is using the plus + operator to join two strings. The second way is using the string interpolation with the help of es6 string literals. The third way is using the += operator to assign a string to the existing string.
I showed a few examples of using the different ways of concatenating strings. Which way is better all depends on the situation. When it comes to stylistic preference, I like to follow Airbnb Style guide. When programmatically building up strings, use template strings instead of concatenation. eslint: prefer-template template-curly-spacing Dec 11, 2019 · 2 min read In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. To concatenate a string, you add a plus sign+ between... Sep 22, 2019 - Alternatively you can also use ... a new string concatenating the one you call this method on, with the argument of the method: ... I generally recommend the simplest route (which also happen to be the faster) which is using the + (or +=) operator. Download my free JavaScript Beginner's ...
Aug 11, 2016 - I have some experience with Java and I know that strings concatenation with "+" operator produces new object. I'd like to know how to do it in JS in the best way, what is the best practice for it? The concat () function concatenates the string arguments to the calling string and returns a new string. Changes to the original string or the returned string don't affect the other. If the arguments are not of the type string, they are converted to string values before concatenating. 3 weeks ago - Concatenate is a fancy programming word that means "join together". Joining together strings in JavaScript uses the plus (+) operator, the same one we use to add numbers together, but in this context it does something different. Let's try an example in our console.
JavaScript add strings with concat. The concat method concatenates the string arguments to the calling string and returns a new string. Because the concat method is less efficient than the + operator, it is recommended to use the latter instead. concat.js. let a = 'old'; let c = a.concat (' tree'); console.log (c); The example concatenates two ... Functionally, it looks like it allows you to nest a variable inside a string without doing concatenation using the + operator. I'm looking for documentation on this feature. Example: var string = 'this is a string'; console.log (`Insert a string here: $ {string}`); javascript string variables concatenation. Share. It may seem that the previous approach creates a new string whenever a piece is added to str. Older JavaScript engines do it that way, which means that you can improve the performance of string concatenation by collecting all the pieces in an array first and joining them as a last step:
JavaScript String concat () The JavaScript String concat () method concatenates given arguments to the given string. The syntax of the concat () method is: str.concat (str1, ..., strN) Here, str is a string. String concatenation "+" operator In Javascript, you can do that in a multitude of ways, and one of them is by using the String concatenation operator, or the "+" operator. Now, this "+" operator, if used with numbers, like this: var a = 1 + 2; JavaScript Strings: Concatenation. "Concatenation" is the process of joining things together. In JavaScript, concatenation is most commonly used to join variable values together, or strings with other strings, to form longer constructions. Somewhat confusingly, the most common JavaScript concatenation operator is +, the same as the addition ...
To concatenate multiple string variables, use the JavaScript concatenation operator.ExampleYou can try to run the following code to concatenate variablesLive De ... Plan A for string concatenation: using the + operator. string1 + string2. If you ask a Javascript programmer how to concatenate a string, this is likely the method he'll respond with. It simply uses the + operator to concatenate the right operand with the left operand. This method used to be fairly slow, but has recently been optimized enough ... Dec 29, 2020 - For example, you may have two lists of employees stored as strings, one for each branch in your company. You may want to merge them all into one list of all your employees. ... Using the concatenation operator (+). Using the concat() method. In this tutorial, we are going to discuss how to concatenate a JavaScript ...
Jul 07, 2017 - Every now and again I get into a discussion with colleagues about the best way to concatenate strings in Javascript. That’s because I live an action packed life. Extracting String Parts. The methods listed below are used for extracting a piece of a string: slice (start, end) substring (start, end) substr (start, length) JavaScript slice () is used to extract a piece of string and return it as a new string. There are two parameters: a start position index and an end one. JavaScript String Operators The + operator can also be used to add (concatenate) strings.
Sep 01, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Dec 11, 2019 - In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. String#concat () JavaScript strings have a built-in concat () method. The concat () function takes one or more parameters, and returns the modified string. Strings in JavaScript are immutable, so concat () doesn't modify the string in place.
Using plus operator to concatenate strings is supported by many languages. We can put variables into a statement without plus operator by using "`". It is called Template Literal which was called Template String before. Fundamentals of String Concatenation in JavaScript. Concatenation is the operation that forms the basis of joining two strings. Merging strings is an inevitable aspect of programming. Before getting into "String Concatenation in JavaScript", we need to clear out the basics first. When an interpreter executes the operation, a new string is ... In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string.
This video details three different ways that you can combine multiple strings and variables with string values in JavaScript.Code GIST: https://gist.github.c... Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript program to concatenate two strings and return the result. If the length of the strings are not same then remove the characters from the longer string. Concatenation, or joining strings, is an important facility within any programming language. It's especially vital within web applications, since strings are regularly used to generate HTML output....
JavaScript String object has a built-in concat () method. As the name suggests, this method joins or merges two or more strings. The concat () method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings. This works by using the .concat method like so: var str1 = "Hello "; var str2 = "world!"; var res = str1.concat (str2); Interestingly you could also convert the strings to an array and use array operations like [push] [2] to concatenate strings. A performance evaluation (by jsperf) can be found here. Our catalog of all the courses we offer. Browse by topic or difficulty. Sign up today and get access to our entire library. Treehouse students get access to workshops, bonus content, conferences, and more.
Concatenate strings in Javascript with a separator. Earlier, when we used the join() and concat() methods, we found out that the resulting string has a separator with every value. The separator was a comma that is the default separator of these two methods. But while concatenating strings using the (+) sign, we should use some kind of ... Code language: JavaScript (javascript) The concat () accepts a varied number of string arguments and returns a new string containing the combined string arguments. If you pass non-string arguments into the concat (), it will convert these arguments to a string before concatenating. Being able to concatenate (join) two or more strings together is pretty useful - you may be combining user input for storage in a single database column or doing the reverse - combining data from multiple database columns into a single string for display or output.. There are several ways to concatenate strings in Javascript, and we'll cover these methods below, along with some useful ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
String Operations In Programs Ap Csp Article Khan Academy
Concatenate One Or More Strings To Another And Returns The
Tcs Coding Practice Question Concatenate 2 Strings
Javascript Strings Find Out Different Methods Of String
Basic Js Concatenating Strings With Plus Operator
Postgresql Concat Function W3resource
Python Concatenate Strings Step By Step Guide Career Karma
Best Of Jsperf 2000 2013 Part 1 3 Sitepoint
Concatenate Array Elements In String Js Code Example
Visual Basic String Concat Method Tutlane
String Concatenation In C Without Using Strcat Code Example
Javascript Lesson 22 Concat Method In Javascript Geeksread
Javascript Concatenate Strings Es6 String Concat Method
Javascript Concat String How Does Concat Function Work In
Concatenate Char To String In Java
Concatenate Char To String In Java
Concatenating Strings With Plus Operator Freecodecamp Basic Javascript
4 Ways To Concatenate Strings In Javascript
4 Different Ways To Concat String In Javascipt Tutorials
How Can We Concatenate 2 Or More Strings In Javascript By
Why Does Javascript Handle The Plus And Minus Operators
Jsnoob Push Vs Concat Basics And Performance
How To Work With Strings In Jmeter Blazemeter
Javascript Concatenate Strings Es6 String Concat Method
Faq Variables String Concatenation With Variables
How To Concatenate Strings Javascript Code Example
Javascript Concat String Does Not Work As Expected Stack
Understanding The Concatenation Of Strings In Java
How To Concatenate A String In Python And More Android
0 Response to "32 Concatenate A String In Javascript"
Post a Comment