23 Search Element In Object Javascript
The 3 ways to check if an object has a property in JavaScript: hasOwnProperty() method, in operator, comparing with undefined. Dmitri Pavlutin. I help developers understand JavaScript and React. All posts Search About. 3 Ways to Check If an Object Has a Property in JavaScript. Javascript: Find Object in Array. To find Object in Array in JavaScript, use array.find () method. Javascript array find () function returns the value of the first item in the provided array that satisfies the provided testing function. The find () function returns the value of the first item in an array that pass the test (provided as a function).
How To Use Javascript Collections Map And Set
closest. Ancestors of an element are: parent, the parent of parent, its parent and so on. The ancestors together form the chain of parents from the element to the top. The method elem.closest(css) looks for the nearest ancestor that matches the CSS-selector. The elem itself is also included in the search.. In other words, the method closest goes up from the element and checks each of parents.
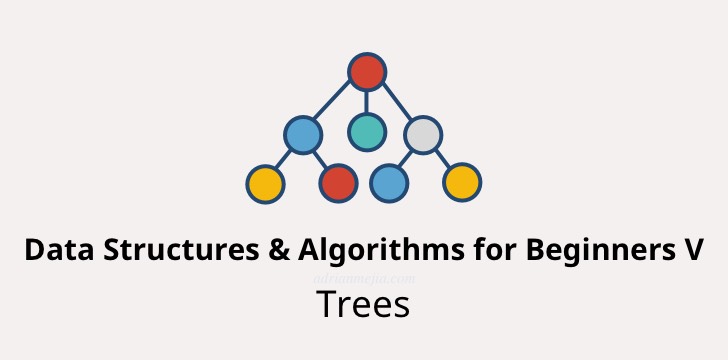
Search element in object javascript. Given a jQuery object that represents a set of DOM elements, the .find() method allows us to search through the descendants of these elements in the DOM tree and construct a new jQuery object from the matching elements. The .find() and .children() methods are similar, except that the latter only travels a single level down the DOM tree.. The first signature for the .find()method accepts a ... Just like in selenium web driver, javascript also provides some methods to find the elements: getElementById. getElementsByName. getElementsByClass. getElementsByTagName. Here if you observe carefully, we have only one single method (getElementById) which returns the webelement and the rest are returning the list of webelements. The find () method. The Array find () method returns the first matched element in array that satisfies a condition. The find () method takes a function as argument which returns true or false based on some condition. The find () method executes this function for each element of array. If the function returns true for any element then that ...
Below examples illustrate the JavaScript Array find () method in JavaScript: Example 1: Here the arr.find () method in JavaScript returns the value of the first element in the array that satisfies the provided testing method. <script>. var array = [10, 20, 30, 40, 50]; var found = array.find (function (element) {. The find method executes the callbackFn function once for each index of the array until the callbackFn returns a truthy value. If so, find immediately returns the value of that element. Otherwise, find returns undefined. callbackFn is invoked for every index of the array, not just those with assigned values. This means it may be less efficient for sparse arrays, compared to methods that only ... So i modified my answer to add a) trimming code (to trim the search string prior to searching and) and b) more complete array object search code to ensure we don't have duplicates in the result set. Now i guess it works the way you want. Please check my updated answer.
In the example above, the first array element has four items, the second array element has three items and the third array element has only one item. In each of these cases, only the first two items are used when forming an object. If there is only a single element present, the corresponding value of that element is undefined. Related Articles ... Definition and Usage. The find() method returns the value of the array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values) When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ...
Find Object In Array With Certain Property Value In JavaScript. If you have an array of objects and want to extract a single object with a certain property value, e.g. id should be 12811, then find () has got you covered. I want to find the object with an id of 12811. find () takes in a testing function. We want the object's id property to ... This works fine and returns an object from a nested "structure" by Id. I would like to know if you could suggest a better approach, possible a faster one. <script> var data = { item: [ ... Both indexOf and lastIndexOf take an optional second argument that indicates where to start searching. Another fundamental array method is slice, which takes start and end indices and returns an array that has only the elements between them. The start index is inclusive, the end index exclusive.
JavaScript provides a bunch of good ways to access object properties. The dot property accessor syntax object.property works nicely when you know the variable ahead of time. When the property name is dynamic or is not a valid identifier, a better alternative is square brackets property accessor: object[propertyName] . Nov 22, 2020 - So we must understand them first before going in-depth anywhere else. An object can be created with figure brackets {…} with an optional list of properties. A property is a “key: value” pair, where key is a string (also called a “property name”), and value can be anything. 1 week ago - The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well).
It adds the specified values to the Set object. clear() It removes all the elements from the Set object. delete() It deletes the specified element from the Set object. entries() It returns an object of Set iterator that contains an array of [value, value] for each element. forEach() It executes the specified function once for each value. has() Dec 22, 2020 - Say you have this object: const dog = { name: 'Roger' } To get the value of the name property, you can use the dot syntax, like this: dog.name Or you can use the square brackets property accessor syntax: dog['name'] This is especially useful when a property has not a valid variable name, like ... find() Parameters. The find() method takes in: callback - Function to execute on each element of the array. It takes in: element - The current element of array. thisArg (optional) - Object to use as this inside callback.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript is an object oriented scripting language, while Java is an object oriented programming language. There are major differences between the two (JavaScript can only be run inside browsers, for example), but they are both languages that revolve around the concept of manipulating objects to perform programming tasks. Most of the times when we're working with JavaScript, we'll be dealing with nested objects and often we'll be needing to access the innermost nested values safely. Let's take this nested object as an example. const user = {. id: 101, email: 'jack@dev ', personalInfo: {. name: 'Jack',
Method 2: Using the find method() to compare the keys: The Object.keys() method is used to return all the keys of the object. On this array of keys, the find() method is used to test if any of these keys match the value provided. The find() method is used to return the value of the first element that satisfies the testing function. The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) element is the current element. index the index of the current element. array the array that the find() was called upon. 2) thisArg. The thisArg is the object used as this inside the callback. Return value. The find() executes the callback function for each element in the array until the callback returns a truthy value.
Here, the if condition is testing if any object in the array is available with id and name equal to the given object. If found, it returns true and moves inside the block.. Using findIndex() : findIndex() method is used to find the index of first element in the array based on a function parameter. If the element is not found, it returns -1. Unlike Object.values() that creates an array of the values in the object, Object.entries() produces an array of arrays. Each inner array has two elements. The first element is the property; the second element is the value. Here is an example: In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf() method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.. indexOf() Method The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf() method.
On getting a true return value from the callback function, find() returns the value of that element in the array (an object in this case). However it would have been better if the return value would just be the index of the matched element instead of the whole element. For this Array.findIndex() is used. Method 3 — Using Array.findIndex() Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The in operator returns true if the specified property is in the specified object or its prototype chain.
Array.some will check if at least one value in the array matches the condition in our callback function and Array.every will check that ALL of the elements in the Array match that condition.. Replacing an element of an Array at a specific index Now that we know how to check if the Array includes a specific element, let's say we want to replace that element with something else. 1 week ago - The for...in statement iterates over all enumerable properties of an object that are keyed by strings (ignoring ones keyed by Symbols), including inherited enumerable properties. 4 weeks ago - The hasOwnProperty() method returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it).
Array.prototype.findIndex () The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1 , indicating that no element passed the test. See also the find () method, which returns the value of an array element, instead of its index. To search a particular object, we will use the Array prototype find method. This returns a value on a given criterion, otherwise, it returns 'undefined'. It takes two parameters, one required callback function and an optional object, which will be set as a value of this inside the callback function.. The callback function will be called for each element of the array until the given ... 1 week ago - The Object class represents one of JavaScript's data types. It is used to store various keyed collections and more complex entities. Objects can be created using the Object() constructor or the object initializer / literal syntax.
Recently while working I came across a scenario. I had to find index of a particular item on given condition from a JavaScript object array. In this post we will see how to find index of object from JavaScript array of object. Let us assume we have a JavaScript array as following, Now if we… Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Searchbar React Native Elements
Check If String Exists In An Array Java Code Example
Statistically Defined Visual Chunks Engage Object Based
Tree Data Structures In Javascript For Beginners Adrian
Javascript How Browser Consoles Displays The Object Key
Programmable Search Element Control Api Programmable Search
Injecting Javascript Into Custom Api And Replaying Ajax Calls
How To Remove Duplicates From An Array Of Objects Using
Find Object By Id In An Array Of Javascript Objects Stack
Users Lookup In Chat Search Breaks With Javascript Error
Javascript Search Json Array For Date Code Example
Data Structures Objects And Arrays Eloquent Javascript
Javascript Find An Object In Array Based On Object S
Javascript Array Of Objects Tutorial How To Create Update
2 Ways To Check If Value Exists In Javascript Object
Looping Javascript Arrays Using For Foreach Amp More
How To Check If A Key Exists In A Javascript Object
5 Working With Arrays And Loops Javascript Cookbook Book
The Json Query Function To Extract Objects From Json Data
0 Response to "23 Search Element In Object Javascript"
Post a Comment