20 Convert Json Into Array In Javascript
JSON (JavaScript Object Notation) is a lightweight data-interchange format. As its name suggests, JSON is derived from the JavaScript programming language, but it's available for use by many languages including Python, Ruby, PHP, and Java and hence, it can be said as language-independent. ... Converting JavaScript Arrays into CSVs and Vice ... This array contains the values of JavaScript object obtained from the JSON string with the help of JavaScript. There are two approaches to solve this problem which are discussed below: Approach 1: First convert the JSON string to the JavaScript object using JSON.Parse() method and then take out the values of the object and push them into the ...
Node Js Transform File Buffer To Array Of Object From Html
Just double-checking: is the array you want to send to the page a JavaScript array or is it on the server? - Ian Oxley Feb 19 '10 at 10:22 it's a Javascript array, I will be sending it to a Python script and Python will use the JSON string and work with that. - dotty Feb 19 '10 at 10:24
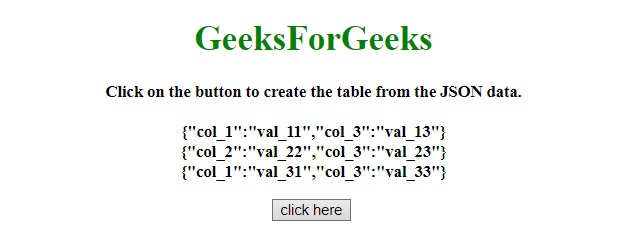
Convert json into array in javascript. Convert JSON object to javascript array. by leealdrich. on Jun 9, 2016 at 15:51 UTC. Solved Web Development. 1. Next: Do i need SSl certification for integrate? Get answers from your peers along with ... But how do I then convert this into an array? My original PHP code, btw, is: I need to convert JSON object string to a JavaScript array. This my JSON object: {"2013-01-21":1,"2013-01-22":7} And I want to have: var data = new google.visualization.DataTable(); data.addCol... How to convert Set to Array in JavaScript? Last Updated : 21 Jul, 2021. A set can be converted to an array in JavaScript by the following way-. By using Array.from () method: This method returns a new Array from an array like object or iterable objects like Map, Set, etc. Syntax. Array.from (arrayLike object); Example-1.
Use the JSON.parse () Expression to Convert a String Into an Array. The JSON.parse () expression is used to parse the data received from the web server into the objects and arrays. If the received data is in the form of a JSON object, it will convert it into a JavaScript object. [code]var JSONItems = []; $.getJSON( "js/settings.json", function( data){ JSONItems = data; console.log(JSONItems); }); [/code]Or [code]var JSONItems = []; $.get( "js ... This blog post covers how to convert Array to JSON and JSON to Array in typescript with examples. Before Doing conversion, Always understands Structure of JSON, apply logic as per JSON structure. typescript is an extended version of javascript. Below examples works on javascript environment also. How to Convert Array of Objects to JSON with ...
Created: July-24, 2021 . This tutorial will discuss converting an array to JSON using the JSON.stringify() function in JavaScript.. Convert an Array to JSON Using the JSON.stringify() Function in JavaScript. We use JSON to send and receive data from a server, and the data should be in a string format. Convert a JSON Response into an Array of Objects. Ask Question Asked 2 years, 6 months ago. Active 2 years, ... usually im not that stupid in terms of JS but never had todo with JSON yet, will defenetly read myself into it for the future. - Badgy Feb 8 '19 at 12:30 ... For-each over an array in JavaScript. 6102. Hi. I got my output in JSON... Now I need to convert those data into javascript.. How to write the code in javascript? I have to display the images to the browser.. it is possible only by writing the code in javascript. Help me.. My JSON output is..
You convert the whole array to JSON as one object by calling JSON.stringify () on the array, which results in a single JSON string. To convert back to an array from JSON, you'd call JSON.parse () on the string, leaving you with the original array. To convert each item in an array into JSON format, then you'll want to loop over the array. Javascript Object Notation. In layman's terms, we use the JSON.stringfy(ARRAY) function to turn an array or object into a JSON encoded string - Then store or transfer it somewhere. We can then retrieve this JSON encoded string, do the opposite of JSON.parse(STRING) to get the "original" array or object back. JSON parsing is the process of converting a JSON object in text format to a Javascript object that can be used inside a program. In Javascript, the standard way to do this is by using the method JSON.parse(), as the Javascript standard specifies.. Using JSON.parse() Javascript programs can read JSON objects from a variety of sources, but the most common sources are databases or REST APIs.
Given an HTML document containing JSON data and the task is to convert JSON data into a HTML table. Approach 1: Take the JSON Object in a variable. Call a function which first adds the column names to the < table > element.(It is looking for the all columns, which is UNION of the column names). Traverse the JSON data and match key with the ... JSON to Array converter simplest. This free online tool lets you convert a JSON file into a Array file.Just paste your JSON in the form below and it will instantly get converted to Array. No need to download or install any software. Free . JSON to Array converter examples. JSON You should avoid using functions in JSON, the functions will lose their scope, and you would have to use eval() to convert them back into functions. Previous Next NEW
Summary: in this tutorial, you will learn how to convert an object to an array using Object's methods.. To convert an object to an array you use one of three methods: Object.keys(), Object.values(), and Object.entries().. Note that the Object.keys() method has been available since ECMAScript 2015 or ES6, and the Object.values() and Object.entries() have been available since ECMAScript 2017. convert json array to javascript array. 1. How to convert a JSON-like (not JSON) string to an object? 0. ... Load a json file into an array in javascript. 0. With JavaScript or jQuery, how can I convert a name-value pair string into an object? 2. parsing JSON to javascript array. 0. 22/10/2020 · JavaScript Convert an array to JSON; Search by id and remove object from JSON array in JavaScript; Removing property from a JSON object in JavaScript; Building a frequency object from an array JavaScript; Build tree array from JSON in JavaScript; How to turn a JSON object into a JavaScript array in JavaScript ? How to convert JSON text to ...
The below approaches can be followed to solve the problem. Approach 1: In this approach, we create an empty object and use the Array.forEach () method to iterate over the array. On every iteration, we insert the first item of the child array into the object as a key and the second item as the value. Then it returns the object after the iterations. The array is passed as a parameter to the stringify() function. It converts the array into a plain string. But to convert the array into JSON format, we need to pass it to the stringify() function enclosed in curly brackets accompanied by the spread operator. Observe the following code. Convert JSON file to Array using jQuery .getJSON() Method. Now, let's see how you can accomplish the same task, that is, converting data in JSON file to an array, using jQuery. The jQuery .getJSON() method is ideal for this kind of task. That's what I am using here in my second example.
20/11/2020 · How to convert a JSON string into a JavaScript object? Converting two arrays into a JSON object in JavaScript; How to turn a String into a JavaScript function call? Convert JSON array into normal json in JavaScript; How to turn JavaScript array into the comma-separated list? How to convert a date object's content into json in JavaScript? JSON, or "JavaScript Object Notation", is an extremely popular data exchange format, especially in web development. Let's go over a few simple ways to convert an array to JSON data. Sorry for the interruption! Use the JavaScript function JSON.stringify () to convert it into a string. const myJSON = JSON.stringify(obj); The result will be a string following the JSON notation. myJSON is now a string, and ready to be sent to a server: Example. const obj = {name: "John", age: 30, city: "New York"}; const myJSON = JSON.stringify(obj);
Here, 1.JSON.stringify () and Object.assign () method convert array to JSON string. 2.JSON.parse () method convert string to JSON object in javascript. 2. Converting an Object to an Array. When converting an object to an array, we'll use the .entries () method from the Object class. This will convert our object to an array of arrays. We can convert an Object {} to an Array [] of key-value pairs using methods discussed below: Method 1: In this method, we will use Object.keys () and map () to achieve this. Approach: By using Object.keys (), we are extracting keys from the Object then this key passed to map () function which maps the key and corresponding value as an array, as ... To convert the array from the local JSON file to the JavaScript-based object, you can use the ES6 import statement to import the local JSON file and use it in the existing components. Any JSON file can be imported from the local directory. 1 import Students from "./Students"; jsx. Here in the above import statement, the Students will represent ...
How To Parse Custom Json Data Using Excel The Excel Club
How To Iterate Through Jsonarray In Javascript Crunchify
Reshaping Json With Jq Programming Historian
Working With Json Data In Python Python Guides
Convert Json String To Data Array Using Javascript Or C
How To Convert Json Data To A Html Table Using Javascript
Modifying Json Data Using Json Modify In Sql Server
How To Convert Json Array Property Value From Arrays To Keys
Json Loop A Multidimensional Array To With Node Js Stack
How To Parse Json Data With Python Pandas By Ankit Goel
How To Import Export Json Data Using Sql Server 2016
Convert Array To Json Object Javascript Tuts Make
How To Parse Custom Json Data Using Excel The Excel Club
Python Read Json File How To Load Json From A File And
Converting Json With Nested Arrays Into Csv In Azure Logic
Vuejs Json Array Object String Parse Example Pakainfo
How To Parse Custom Json Data Using Excel The Excel Club
Correct Way To Parse Json File Into Typescript Array Object
How To Convert A Javascript Array To Json Format By Dr
0 Response to "20 Convert Json Into Array In Javascript"
Post a Comment