22 Javascript Sort An Object
Given an array of objects and the task is to sort the array elements by 2 fields of the object. There are two methods to solve this problem which are discussed below: Approach 1: First compare the first property, if both are unequal then sort accordingly. If they are equal then do the same for the second property. JavaScript Program to Sort Array of Objects by Property Values In this example, you will learn to write a JavaScript program that will sort an array of objects by property values. To understand this example, you should have the knowledge of the following JavaScript programming topics: JavaScript Array sort ()
Sort Array Of Objects By String Property Value In Javascript
Jul 08, 2019 - Arrays in JavaScript come with a built-in function that is used to sort elements in alphabetical order. However, this function does not directly work on arrays of numbers or objects. Instead a custom function, that can be passed to the built-in sort() method to sort objects based on the ...
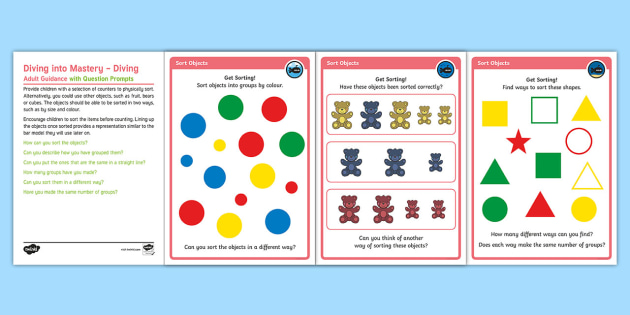
Javascript sort an object. It feels like such a waste having to iterate through, in my case, an api output containing id's and value's only and saving them in a javascript object only to iterate through them again because the javascript object is now sorted by key, and I need to present the user with an alphabetical list of values, with no way to sort by value. We are required to write a JavaScript function that takes in this object and returns a sorted array like this − const arr = [11, 23, 56, 67, 88]; Here, we sorted the object values and placed them in an array. When we return 1, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning -1 would do the opposite.. The callback function could calculate other properties too, to handle the case where the color is the same, and order by a secondary property as well:
Deeper: The actual sort algorithm used is dependent on JavaScript implementation and will vary from platform to platform. ... Sorting objects by a single property is great but we want a little more versatility. Let's take a look at these students and see if we can sort them by grade, then by ... We are required to write a JavaScript function that takes in one such array and sorts the sub-objects on the basis of the 'position' property of sub-objects (either in increasing or decreasing order). Sort by date (release date) movies.sort (function (a, b) { var dateA = new Date (a.release), dateB = new Date (b.release); return dateA - dateB; }); Sort by works because JavaScript lets you compare and/or do arithmetic on date objects, which are automatically converted to numeric representations first.
21/1/2021 · However, there are no in-built JavaScript methods to sort object properties. Here we have an object, pets. The key is a string which represents the type of pet. The value is an integer which represents the number of the specified pet. There are 3 object methods that can be used to parse through the data. JS: Sort a JavaScript object by key in alphabetical order case insensitive. Thanks to Arne Martin Aurlien and Ivan Krechetov for inspiration. #snippet - sortObj.js Testing sort () method on Object.entries () method. As you can see we got an array of arrays sorted by values as the result. Object.entries (grossaryList).sort ((a,b) => b -a), where a meens...
JSON Object does not maintain order of insertion. This post discusses how to sort JSON object by key or value. In order to sort JSON objects, we have to take help of Arrays. Arrays maintain an order of insertion. Moreover, it provides native sort() method to sort array elements. Sort JSON Object by Key: Jan 25, 2021 - Sort Array of Objects By Two Properties in JavaScript ... I was recently working on a way to sort an array of objects alphabetically based on two columns. This means that both levels of alphabetical sorting should be maintained. See the example below. The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana").
When we return a positive value, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning a negative value will do the opposite. The sort() method returns a new sorted array, but it also sorts the original array in place. Thus, both the sortedActivities and activities arrays are now sorted. One option to protect the original array from being ... If you need to sort an array of objects into a certain order, you might be tempted to reach for a JavaScript library. But before you do, remember that you can do some pretty neat sorting with the... Sep 01, 2020 - You should never make assumptions about the order of elements in a JavaScript object. An Object is an unordered collection of properties. The answers below show you how to "use" sorted properties, using the help of arrays, but never actually alter the order of properties of objects themselves.
JavaScript has a built-in sort () method which helps us to sort an array alphabetically. Given an object and the task is to sort the JavaScript Object on the basis of keys. Here are a few of the most used techniques discussed with the help of JavaScript. Approach 1: By using.sort () method to sort the keys according to the conditions specified in the function and get the sorted keys in the array. Introduction to JavaScript- This tutorial introduces to you the very basics of JavaScript
Mar 25, 2021 - Here we are going to sort an array of objects using keys that are available in that objects. For run... Tagged with javascript, sort, object, key. The sort method of JavaScript arrays allows the use of a callback function. For many sort features, this is necessary. In this tutorial we look at sorting an... How to Sort JavaScript Object by Key. In this tutorial, we will share a very simple and functional method to sort an array of objects by key. Here is a ES5 functional method of sorting. The Object.keys gives a list of keys in provided object, then you should sort those using default sorting algorithm, after which the reduce () method converts that ...
When the sort () function compares two values, it sends the values to the compare function, and sorts the values according to the returned (negative, zero, positive) value. If the result is negative a is sorted before b. If the result is positive b is sorted before a. If the result is 0 no changes are done with the sort order of the two values. A protip by davidcollingwood about multiple, javascript, and sort. The sort () method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values. The time and space complexity of the sort cannot be guaranteed as it depends on the implementation.
Home» Sort an Array of Objects in JavaScript Sort an Array of Objects in JavaScript Summary: in this tutorial, you will learn how to sort an array of objects by the values of the object’s properties. To sort an array of objects, you use the sort()method and provide a comparison function that determines the order of objects. Introduction to JavaScript Array sort () method The sort () method allows you to sort elements of an array in place. Besides returning the sorted array, the sort () method changes the positions of the elements in the original array. Sorting an array of objects in JavaScript can be a nightmare if you don't know about the right logic to do it. The preferred way to sort an array is using its sort method, a method that sorts the elements of an array in place. The default sort order is according to the string Unicode code points.
To sort or order keys in JavaScript objects, we can use the Object.keys method to get the keys of the object in a string array. Then we call sort on the array to sort the string keys. And then we use the reduce method to reconstruct the object with the sorted keys and return it. For instance, we can write: const notSorted = { b: false, a: true ... The sort( ) method will sort the item based on the values of the elements in case the compare function is omitted:. If the comparison is less than zero, the sort( ) method sorts to a lower index than b.; If the comparison is greater than zero, the sort( ) method sort b to a lower index than a.; If the comparison returns zero, the sort( ) method considers that a equals b and does not change ... 13/8/2021 · To sort an object by value in JavaScript, we can use the Object.values method to get the property values from the object in an array. Then we can use the JavaScript sort method to sort the array values. We create the dict object with values we want to sort. Then we call Object.values with dict to return an array of dict property values.
Feb 20, 2021 - Also, we’ve to do the same thing in the reduce callback to get the object value from ages . And at the end, sorted should be the same as in the previous example. ... We can use native JavaScript object and array methods to let us sort properties by their values. JavaScript has an inbuilt sort method which helps us to sort the array of the objects easily; we don't need to write our own custom sort methods. consider we have an array of objects with name and price properties. Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor.
Javascript Sort Object Properties By Value Numeric Or
How To Sort An Array Of Objects By Multiple Fields Stack
Javascript Sorting Array Objects Parallelcodes
How To Sort Array Of Objects By Date In Javascript Onthecode
Year1 White Rose Maths Sorting Objects Primary Maths
Sorting Objects In Javascript By Jennifer Yoo Jan 2021
Sort Array Of Objects Function With Localecompare Code Example
Javascript Sort Object By Key Number Code Example
Sort Javascript Object By Key Stack Overflow
Mongodb Sorting Sort Method Amp Examples Bmc Software Blogs
Sort An Object Array By Date In Javascript Geeksforgeeks
Sort Arrays With Javascript Methods
How To Sort An Array Of Object By Two Fields In Javascript
React How To Dynamically Sort An Array Of Objects Using The
Kotlin Sort List Of Custom Objects Bezkoder
Sort Array Of Objects By String Property Value In Javascript
How To Sort Objects In Javascript Simple Examples
Sort Array Of Objects By String Property Value In Javascript
Javascript Sort Array Of Object By Property Code Example
Javascript Array Sort How To Sort Array In Javascript
Sorting Objects In Javascript By Jennifer Yoo Jan 2021
0 Response to "22 Javascript Sort An Object"
Post a Comment