27 Parse Str In Javascript
The parseInt () function parses a string and returns an integer. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. The JSON.parse () method parses a string and returns a JavaScript object. The string has to be written in JSON format. The JSON.parse () method can optionally transform the result with a function.
Javascript 8 Reasons Every Php Developer Should Love It
Example 1: how to convert string to int a array in javascript var a = "1, 2, 3, 4"; var b = a.split(', ').map(function(item) { return parseInt(item, 10); }); Example
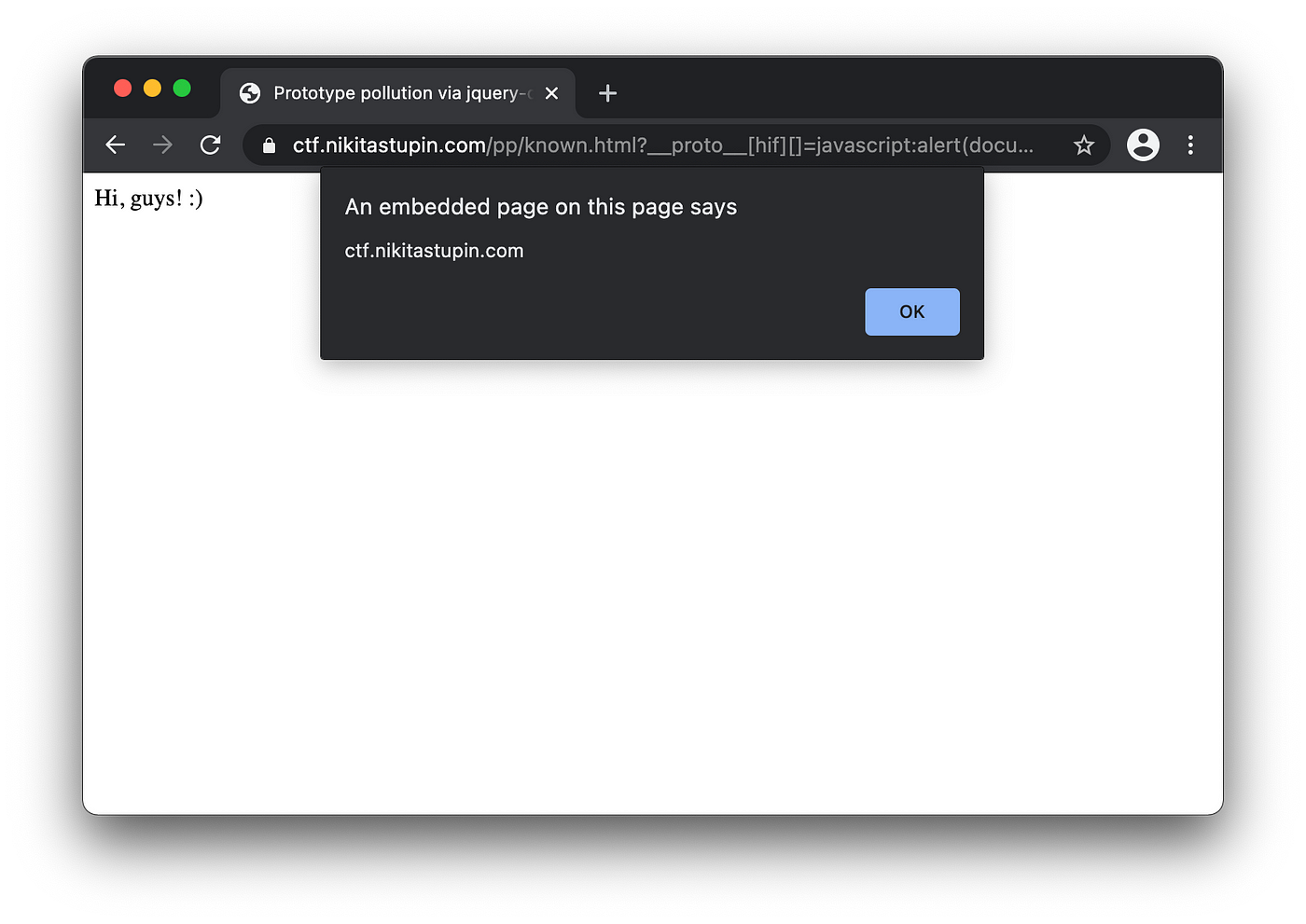
Parse str in javascript. parse string javascript; concat no and string in javascript; how to change string to array in javascript; javascript case insensitive regex; react replace all line breaks with br; javascript regex not in a set of characters; Limit text to specified number of words using Javascript; string to code javascript; javascript get text between two strings First, the json is to read the user data from the web servers and displayed the data in the web page first is converted into the string format using JSON.stringify () and next is again converted string into arrays using JSON.parse () method.This method parses the JSON string and returns the value for javascript is equal for whatever the user ... In the code examples below, we will take a string containing XML and parse it into an XMLDocument object. Converting it into an XMLDocument object allows us to easily read and manipulate the XML. Take a look at the following JavaScript example: //A simple XML string. var xmlString = '<reminder><date>2020-08-01T09:00:00</date><heading>Meeting ...
To convert a string back into a JavaScript object, use the JSON.parse() method like this: var obj = {'foo': 'bar'}; var string = JSON. stringify (obj); var obj2 = JSON. parse (string) console. log (obj2. foo); bar An Alternative way of Converting to Strings. Another way to convert a thing to a string in JavaScript is to use the String ... Jul 15, 2016 - I'm just curious how I go about splitting a variable into a few other variables. For example, say I have the following JavaScript: var coolVar = '123-abc-itchy-knee'; And I wanted to split that ... The value to parse. If this argument is not a string, then it is converted to one using the ToString abstract operation. Leading whitespace in this argument is ignored. An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) of the string . If radix is undefined or 0, it is assumed to be 10 except when ...
Date.parse () The Date.parse () method parses a string representation of a date, and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC or NaN if the string is unrecognized or, in some cases, contains illegal date values (e.g. 2015-02-31). It is not recommended to use Date.parse as until ES5, parsing of strings was entirely ... Type of Array in JavaScript with Example. There are two types of string array like integer array or float array. 1. Traditional Array. This is a normal array. In this, we declare an array in such a way the indexing will start from 0 itself. 0 will be followed by 1, 2, 3, ….n. slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0.
Mar 03, 2021 - There are a few examples, including the following on string formatting. ... There are also some other interesting libraries related to parsing that are not part of a common category. ... There is one special case that could be managed in more specific way: the case in which you want to parse JavaScript ... Aug 01, 2021 - JSON is a built-in javascript object and its parse() method parses a string as JSON and converts it into an object corresponding to the given text. So, if the string value is ‘true’, it is converted to a boolean object with value as true and false otherwise. In JavaScript, we do not have specialized methods or classes. However, we have a powerful mechanism which is regular expressions . Let's take a string as follows " Splitting String Into Tokens in JavaScript " and I try to extract only word tokens from it, i.e. Splitting , String , Into etc. Note that the given string has some leading and ...
Examples to Implement JavaScript Parse String. Using JSON.parse on JSON is derived from the array, the method returns javascript array instead of an object. Let us consider JSON array which consists of Car models, we shall pass content as an array to a JSON object. Json_demo_array.txt is the content file that consists of data of car models. The best string format for string parsing is the date ISO format together with the JavaScript Date object constructor. Examples of ISO format: YYYY-MM-DD or YYYY-MM-DDTHH:MM:SS . But wait! Jul 20, 2021 - The split() method divides a String into an ordered list of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern; where the pattern is provided as the first parameter in the method's call.
Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. The toString () method takes an integer or floating point number and converts it into a String type. There are two ways of invoking this method. If the base number is passed as a parameter to toString (), the number will be parsed and converted to it: Front End Technology Javascript Object Oriented Programming To convert a string to an integer parseInt () function is used in javascript. parseInt () function returns Nan (not a number) when the string doesn't contain number. If a string with a number is sent then only that number will be returned as the output.
The simplest way would be to use the native Numberfunction: var x = Number("1000") If that doesn't work for you, then there are the parseInt, unary plus, parseFloat with floor, and Math.roundmethods. Mar 30, 2020 - JavaScript function that generates all combinations of a string. The URL () constructor is handy to parse (and validate) URLs in JavaScript. new URL (relativeOrAbsolute [, absoluteBase]) accepts as first argument an absolute or relative URL. When the first argument is relative, you have to indicate the second argument as an abolsute URL that serves the base for the first argument.
Mar 30, 2020 - JavaScript function that generates all combinations of a string. JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. In contexts where a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup. 4 weeks ago - If no signs are found, the algorithm ... and runs the number-parsing on the rest of the string. A value passed as the radix argument is coerced to a Number (if necessary), then if the value is 0, NaN or Infinity (undefined is coerced to NaN), JavaScript assumes the follo...
Using Ajax to implement php inside of javascript loop 2 ; sending data of a dropdown list from one html page to another without data base 5 ; Help me out with this imple code pls 7 ; JavaScript dynamic table SUM problem 5 ; javascript code 7 ; mysql_num_rows warning 11 ; problem in passing value from jsp to javascript 16 ; Javascript LOCATION ... Mar 03, 2021 - Guide to JavaScript Parse String. Here we discuss an introduction, how does it works and examples to implement with proper codes and outputs. PHP's parse_str in JavaScript Here's what our current JavaScript equivalent to PHP's parse_str looks like. module.exports = function parse_str (str, array) { const strArr = String (str).replace (/^&/, '').replace (/&$/, '').split ('&')
The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following: Definition and Usage. The split() method splits a string into an array of substrings, and returns the new array.. If an empty string ("") is used as the separator, the string is split between each character. The split() method does not change the original string. convert integer to string in javascript. set number to string javascript. js from int to string. javascript get the number from a string. int to sting in javascript. convert a number to string ain javascript. converting numbers into string in java =script. int to string javascrit. convert anumber to string i n js.
How to delete a particular element from a JavaScript array? JavaScript construct an array with elements repeating from a string; Find the Smallest element from a string array in JavaScript; How to parse a JSON string using Streaming API in Java? How to remove text from a string in JavaScript? How to check that a string is parse-able to a double ... There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them. The + Operator. The same + operator you use for adding two numbers can be used to concatenate two strings.. const str = 'Hello' + ' ' + 'World'; str; // 'Hello World'. You can also use +=, where a += b is a shorthand for a = a + b. ... This is what's called grapheme clusters - where the user perceives it as 1 single unit, but under the hood, it's in fact made up of multiple units. The newer methods spread and Array.from are better equipped to handle these and will split your string by grapheme clusters 👍 # A caveat about Object.assign ⚠️ One thing to note Object.assign is that it doesn't actually produce a pure array.
For example, say I have the following JavaScript: var coolVar = '123-abc-itchy-knee'; And I wanted to split that into 4 variables, how does one do that? To wind up with an array where. array[0] == 123 and array[1] == abc etc would be cool. Or (a variable for each part of the string) A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse (), and the data becomes a JavaScript object. Example - Parsing JSON Now JavaScript's date and time format is a subset of ISO-8601 format, except that JavaScript's Date always has a time component, while ISO-8601 has the concept that value may only be as specific as it is precise. That is, 2020-04-10 in JavaScript is a specific date and time, but in ISO-8601, it is just the date without time. Date and Time¶
Example of String-Parse: JavaScript program to take a float input from the user and convert it into integer and string in a respective manner. Sep 18, 2020 - In this tutorial, you'll learn how to use the JavaScript split() method to split a string into an array of substrings.
Dapp Automatic Detection And Analysis Of Prototype Pollution
Github Puritys Nodejs Phplike Porting Php To Node Js
A Simple Post And Recall Ajax Query Javascript Help Php
Disable Tax For Company And Specific Country In Woocommerce
Javascript中使用php 超好用的php Js Php Extensions In Javascript
Active Questions Tagged Javascript Stack Overflow
Time Loop For Woocommerce Checkout Select Option From Date
Multiple File Upload Using Fine Uploader Phppot
Abusing Php Query String Parser To Bypass Ids Ips And Waf
红日安全 代码审计day7 Parse Str函数缺陷 Freebuf网络安全
Php Free Online Tutorials Page 6
How To Generate A Shift Jis Sjis Percent Encoded String In
Using Jquery Nestedsortable Plugin To Sort Hierarchical Lists
P A S Fork V 1 0 A Web Shell Revival Security Boulevard
Php Get Url Parameter Code Example
Javascript中使用php 超好用的php Js Php Extensions In Javascript
How To Send A Nested Array Through Get Or Post Just
How To Pass An Array Within A Query String Stack Overflow
Control Wut Web Io With Ajax And Php
Use Jquery To Send Javascript Array To Php Stack Overflow
Adactio Journal Tagged With Javascript
0 Response to "27 Parse Str In Javascript"
Post a Comment