27 Prevent Event Bubbling Javascript
Now, check the console: Stop Propagation vs. Stop Immediate Propagation. As we have already learned, both prevent events from bubbling. event.stopPropagation() does not cancel the execution of all events of the same type attached to the element If the DOM node has two click events attached, both will continue to execute. Note: The event.stopPropagation method stops the move upwards bubbling (on one event only), but all the other handlers still run on the current element. In order to stop the bubbling and also prevent the handlers from running on the current element, we can use event.stopImmediatePropagation method. It is another method that stops the bubbling ...
Event Bubbling And Event Capturing In Javascript By Vaibhav
We use a method of the Event interface called stopPropagation (). Essentially, stopPropagation () does what the name describes: it stops the propagation of a bubbling event from going further up the tree of elements that it's occurring in. (It's important to note that stopPropagation () doesn't stop the default action of an element from occurring.
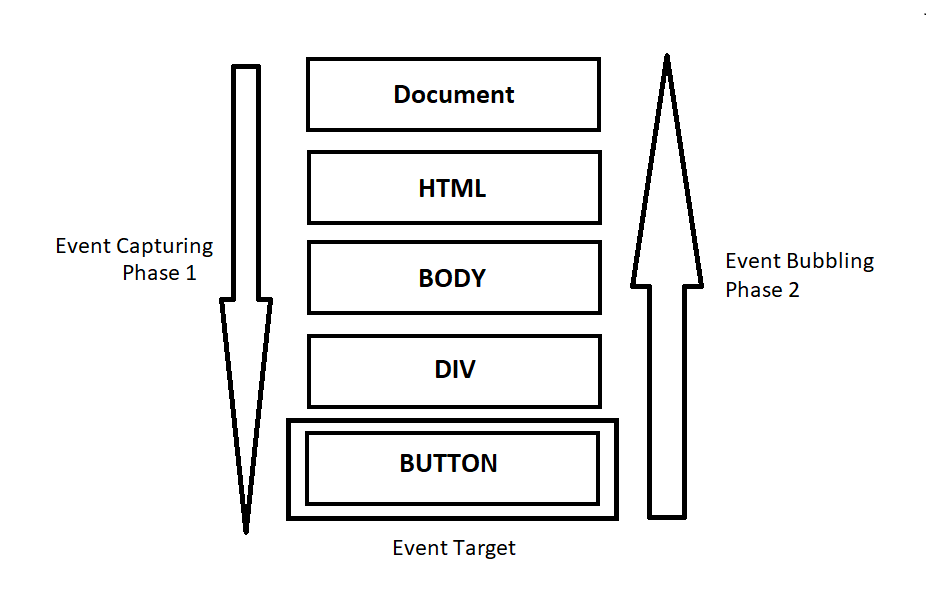
Prevent event bubbling javascript. event.target. A handler on a parent element can always get the details about where it actually happened. The most deeply nested element that caused the event is called a target element, accessible as event.target.. Note the differences from this (=event.currentTarget):. event.target - is the "target" element that initiated the event, it doesn't change through the bubbling process. stopPropagation(): event.stopPropagation() will stop the event from bubbling up the DOM tree. When one of the child elements handling the event uses stopPropagation , the event stops and the parent element will no longer handle the event. stopPropagation while useful, can be a double-edged sword. Note: We can prevent this behavior by adding two lines in our listeners. event.stopPropagation(); The event.stopPropagation() method stops the bubbling of an event to parent elements, preventing any parent event handlers from being executed. So, I conclude this article here.
Event Bubbling - Dealing with the child's parent. There are two ways of event propagation in DOM i.e. Event Capturing and Event Bubbling. In this article, we'll talk about Event Bubbling and leave Event Capturing for some other article. Let's just take a simple analogy, you might have played with bubbles in your childhood …it's ok if ... The event.stopPropagation () method stops the bubbling of an event to parent elements, preventing any parent event handlers from being executed. Tip: Use the event.isPropagationStopped () method to check whether this method was called for the event. Event bubbling in JavaScript. Event bubbling is a method of event propagation in the HTML DOM API when an event is in an element inside another element, and both elements have registered a handle to that event. It is a process that starts with the element that triggered the event and then bubbles up to the containing elements in the hierarchy.
17/1/2018 · It is caused due to event bubbling.Triggering an event on child propagates upward toward its parent.In your case click event on image also triggers click event on parent div.use event.stopPropagation() to prevent this from happening. HTML : pass the event as parameter to event … event.stopPropagation() This method is used to prevent the propagation of an event as defined in the capturing/bubbling phase of the flow of an event in the browser. JavaScript. Copy. The above example is the same as the Event bubbling example, but the difference is enabling the event capturing flow by adding the third optional argument (boolean value) set to "true" for the "addEventListener ()" function. By default it is false. target.addEventListener( type, listener, useCapture);
- Prevents the event from bubbling up the DOM tree, preventing any parent handlers from being notified of the event. - For example, if there is a link with a click method attached inside of a DIV or FORM that also has a click method attached, it will prevent the DIV or FORM click method from firing. Sometimes you'll want to stop an event from bubbling all the way to the top. Maybe you know for sure you're finished processing the event, or perhaps in some case you don't want the event to be triggered on the parent. We can stop bubbling with event.stopPropagation(). Taking the example we're using throughout this guide, we can test this out: Question: How do I cancel event bubbling or stop event propagation? Answer: Sometimes, event bubbling may have unintended consequences. For example, in the event bubbling demo clicking the Reset button triggers not only the button's own event handler function resetTable(), but also another, higher-level onclick event handler, which has the unintended consequence of colorizing the entire page.
The stopPropagation() method of the Event interface prevents further propagation of the current event in the capturing and bubbling phases. It does not, however, prevent any default behaviors from occurring; for instance, clicks on links are still processed. If you want to stop those behaviors, see the preventDefault() method. Prevents the event from bubbling up the DOM, but does not stop the browsers default behaviour. For an in-depth explanation of event bubbling, I'd recommend this article about event propagation . Stop Event Bubbling : If you want to stop the event bubbling, this can be achieved by the use of the event.stopPropagation() method. If you want to stop the event flow from event target to top element in DOM, event.stopPropagation() method stops the event to travel to the bottom to top.
2/7/2019 · Event bubbling By default, events bubble in JavaScript. Event bubbling is when an event will traverse from the most inner nested HTML element and move up the DOM hierarchy until it arrives at the element which listens for the event. This move is also popularly known as Event Propagation or Event Delegation. Output: If we clicked on child div, the propagation is stopped on parent div and does not move to grandparent div.Hence, the event bubbling is prevented. Note: The event capturing can also be prevented using the same way. Important points to remember: If we do not mention any third parameter in addEventListener(), then by default event bubbling will happen. Event bubbling is pretty simple to understand if you know event capturing. It is the exact opposite of event capturing. Event bubbling will start from a child element and propagate up the DOM tree until the topmost ancestor's event is handled. Omitting or setting the useCapture argument to 'false' inside addEventListener () will register ...
Event bubbling is a type of event propagation where the event first triggers on the innermost target element, and then successively triggers on the ancestors (parents) of the target element in the same nesting hierarchy till it reaches the outermost DOM element or document object (Provided the handler is initialized). It is one way that events are handled in the browser. JavaScript Event Bubbling and Capturing. Among the most used terminology in JavaScript at the time of event flow are bubbling and capturing. In general, the event flow process is completed by the following three concepts: event capturing, event target, and event bubbling. Before starting to explain the concept of bubbling, let's consider a case. what is event bubbling in javascript with example; how to make a stopwatch using js; how to prevent event capturing in javascript; bubbling in javascript; ... Get code examples like"how to stop bubbling in javascript". Write more code and save time using our ready-made code examples.
To prevent this situation you can stop event from bubbling up the DOM tree using the event.stopPropagation() method. In the following example click event listener on the parent elements will not execute if you click on the child elements. Definition and Usage. The stopPropagation () method prevents propagation of the same event from being called. Propagation means bubbling up to parent elements or capturing down to child elements. Stop Propagation of Events. To stop an event from further propagation in the capturing and bubbling phases, you call the Event.stopPropation()method in the event handler. Event.stopPropagation(); Code language:CSS(css) Note that the event.stopPropagation()method doesn’t stop any default behaviors of the element e.g., link click, checkbox checked.
event.stopPropagation() Stops the bubbling of an event to parent elements, preventing any parent handlers from being notified of the event. event.preventDefault() Prevents the browser from executing the default action. Use the method isDefaultPrevented to know whether this method was ever called (on that event object). Event bubbling is a term you might have come across on your JavaScript travels. It relates to the order in which event handlers are called when one element is nested inside a second element, and ... Block event of cancels the browser's default behavior for the event and prevents it from propagating ; Talking about the default behavior of js stop event bubbling blocking browser of blocking hyperconnection ; JavaScript prevents event bubbling example sharing
A Simplified Explanation Of Event Propagation In Javascript
20 What Is The Difference Between Stoppropagation And Preventdefault
How To Stop Event Bubbling With Jquery Live Stack Overflow
Event Bubbling And Capturing In Javascript Javatpoint
Event Bubbling In Javascript Geeksforgeeks
The Dangers Of Stopping Event Propagation Css Tricks
Disable Clipboard Events Override Rawsec
Javascript Events Bubbling Capturing And Propagation
Event Bubbling And Event Capturing In Javascript Edureka
Event Bubbling And Event Capturing In Javascript By Vaibhav
Event Propagation With Jquery Ilovecoding
Preventdefault Event Method Geeksforgeeks
Understanding Javascript Events
Overview Of Event Propagation In Salesforce Lightning
Advanced Javascript Events These Are My Notes For Asim
Event Capturing And Bubbling In React Dev Community
Event Bubbling And Event Capturing In Javascript By Vaibhav
Phases Of Javascript Event Geeksforgeeks
Adding Or Attaching Eventlistener Hits Javascript Tutorial
Event Bubbling And Event Capturing In Javascript Explained
Event Flow Capture Target And Bubbling In Javascript
What Is The Preventdefault Event Method In Javascript
Introduction To Events Learn Web Development Mdn
0 Response to "27 Prevent Event Bubbling Javascript"
Post a Comment