35 Javascript Var To String
JavaScript - Convert Function to String and Back February 4, 2017 December 21, 2020 Karl San Gabriel This post demonstrates how to convert a JavaScript function to a string and using the same string to declare and execute a new method dynamically. 1 week ago - So, for example, you could define a variable as follows: ... Because JavaScript is dynamically typed, this assignment does not cause an error message. ... In expressions involving numeric and string values with the + operator, JavaScript converts numeric values to strings.
How To Check If A String Contains Substring Or A Word Using
Passing a java property to a javascript variable. / javascript "); %> var collegename = ''; Java Script code: alert ("collegename...Passing a java property to a javascript variable i want to assign a java property to a js variable : action class: collegename have a property. JavaScript display variable in alert.
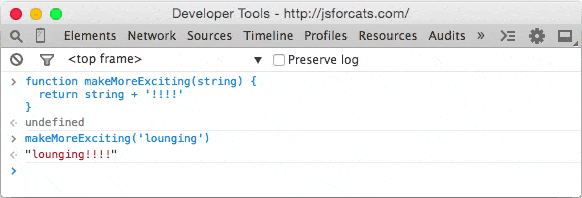
Javascript var to string. Oct 04, 2020 - Get code examples like "convert a variable to string javascript" instantly right from your google search results with the Grepper Chrome Extension. Convert string to variable name in JavaScript . Posted by: admin November 18, 2017 Leave a comment. Questions: I've looked for solutions, but couldn't find any that work. I have a variable called onlyVideo. "onlyVideo" the string gets passed into a function. The difference between the String() method and the String method is that the latter does not do any base conversions. How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type:
To convert a string to an integer parseInt() function is used in javascript.parseInt() function returns Nan( not a number) when the string doesn't contain number.If a string with a number is sent then only that number will be returned as the output. This function won't accept spaces. If any particular number with spaces is sent then the part of the number that presents before space will be ... In JavaScript, toString () is a string method that is used to return a string representation or primitive value of an object. Because the toString () method is a method of the String object, it must be invoked through a particular instance of the String class. JSON is the javascript object notation is one of the web request and response format in the server-side. We can convert the json request into the string format using javascript predefined methods like stringfy () it's converted into a javascript instance or values to be shifted into the json strings. The values are replacing optionally ...
JavaScript cannot add a number and a string without converting the number to a string first. JavaScript converts 3 to "3" and then concatenates the two strings. 3 can be converted to "3" with this code: String(3); Round the number 4.87 to the nearest integer. JavaScript Data Types. JavaScript variables can hold numbers like 100 and text values like "John Doe". In programming, text values are called text strings. JavaScript can handle many types of data, but for now, just think of numbers and strings. Strings are written inside double or … Return Value: The num.toString () method returns a string representing the specified number object. Below are some examples to illustrate the working of toString () method in JavaScript: Converting a number to a string with base 2: To convert a number to a string with base 2, we will have to call the toString () method by passing 2 as a parameter.
2 weeks ago - Template literals are literals delimited with backticks (`), allowing embedded expressions called substitutions. The function produces, as promised, a string (a primitive). The constructor produces an instance of the type String (an object). The latter is hardly ever useful in JavaScript, which is why you can usually forget about String as a constructor and concentrate on its role as converting to string. A minor difference between ""+value and String(value) Dec 24, 2020 - You don't have to type globalThis.String, you can just type the unqualified String. The corollary, in non-strict mode, is that assignment to unqualified identifiers will, if there is no variable of the same name declared in the scope chain, assume you want to create a property with that name ...
Mar 02, 2018 - Connect and share knowledge within a single location that is structured and easy to search. ... I was wondering if this same thing is possible in JavaScript as well. Using variables inside strings without using concatenation — it looks more concise and elegant to write. HTML CSS JAVASCRIPT SQL PYTHON PHP BOOTSTRAP HOW TO W3.CSS JAVA JQUERY C++ C# R React Kotlin ... JS HOME JS Introduction JS Where To JS Output JS Statements JS Syntax JS Comments JS Variables JS Let JS Const JS Operators JS Arithmetic JS Assignment JS Data Types JS Functions JS ... In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. To concatenate a string, you add a plus sign+ between the strings or string variables you want to connect. let myPet = 'seahorse'; console.log('My favorite animal is the ' + myPet + '.'); // My favorite animal is the seahorse.
Use sprintf () to Insert Variable Into String in JavaScript Before Template Literals in ES6 of Javascript, the development community followed the feature-rich library of sprintf.js. The library has a method called sprintf (). sprintf.js is an open-source library for JavaScript. It has its implementations for Node.js and browsers. Append a String to Another String Using the concat () Function in JavaScript To append one string with another string, we can use the predefined concatenation function concat () in JavaScript. This function concatenates two given strings together and returns the one string as output. Nov 03, 2019 - Managing data is one of the fundamental concepts of programming. Because of this, JavaScript offers plenty of tools to parse various data types, allowing you to easily interchange the format of data. Particularly, I'll be covering how to convert a Number to a String in this article.
1 week ago - Before ECMAScript 2015, typeof was always guaranteed to return a string for any operand it was supplied with. Even with undeclared identifiers, typeof will return 'undefined'. Using typeof could never generate an error. However, with the addition of block-scoped let and const, using typeof on let and const variables ... The String () function converts the value of an object to a string. Note: The String () function returns the same value as toString () of the individual objects. Next, we'll turn our attention to strings — this is what pieces of text are called in programming. In this article, we'll look at all the common things that you really ought to know about strings when learning JavaScript, such as creating strings, escaping quotes in strings, and joining strings together.
Nov 05, 2020 - // How to create string with multiple spaces in JavaScript var a = 'something' + '\xa0\xa0\xa0\xa0\xa0\xa0\xa0' + 'something'; JavaScript is a "dynamically typed language", which means that, unlike some other languages, you don't need to specify what data type a variable will contain (numbers, strings, arrays, etc). For example, if you declare a variable and give it a value enclosed in quotes, the browser treats the variable as a string: let myString = 'Hello'; In JavaScript, a variable stores the data value that can be changed later on. Use the reserved keyword var to declare a variable in JavaScript. Syntax: var < variable-name >; var < variable-name > = < value >; A variable must have a unique name. The following declares a variable. Example: Variable Declaration.
May 23, 2020 - JavaScript function that generates all combinations of a string. How to Use the toString Method in JavaScript To use the toString () method, you simply need to call the method on a number value. The following example shows how to convert the number value 24 into its string representation. Notice how the value of the str variable is enclosed in double quotation marks: Variables in JavaScript are named containers that store a value, using the keywords var, const or let. We can assign the value of a string to a named variable. const newString = "This is a string assigned to a variable."; Now that the newString variable contains our string, we can reference it and print it to the console.
Syntax: parseInt (Value, radix) It accepts string as a value and converts it to specified radix system and returns an integer. Program to convert string to integer: Example-1: <script>. function convertStoI () {. var a = "100"; var b = parseInt (a); Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The JSON.stringify () method converts an object or value to a JSON string. JSON.stringify skips some JavaScript-specific objects, such as properties storing undefined, symbolic properties, and function properties. The toString () method is called without arguments and should return a string.
What's the best way to do insert variables in a string in JavaScript? I'm guessing it's not this: var coordinates = "x: " + x + ", y: " + y; In Java, Strings are immutable and doing something like the above would unnecessarily create and throw away Strings. Ruby is similar and has a nice way of doing the above: coordinates = "x: #{x}, y: #{y}" 9/4/2020 · The typeof operator. We can use the typeof operator to check if a given variable is a string. The typeof operator returns the type of a given variable in string format. Here is an example: let user = 'gowtham'; if (typeof user === 'string') { console.log('user is a string'); } else { console.log('user is not a string'); } 1.I want to now use the name I have extracted, displayed through the variable text above, and create a string (or label as described below) from that variable - so that it can be then sent to a ...
{{CODE_Includes}} The following is a module with functions which demonstrates how to get the name of a variable as a string using vanilla JavaScript. 1. Simple Variable The example below demonstrat… 17/10/2011 · As of node.js >4.0 it gets more compatible with ES6 standard, where string manipulation greatly improved. The answer to the original question can be as simple as: var s = `hello ${my_name}, how are you doing`; // note: tilt ` instead of single quote ' Where the string can spread multiple lines, it makes templates or HTML/XML processes quite easy. To declare a variable and initialize it at the same time, you use the following syntax: var variableName = value; Code language: JavaScript (javascript) For example, the following statement declares the message variable and assign it a value "Hello". var message = "Hello"; Code language: JavaScript (javascript)
The concat() method is used to join two or more strings. This method does not change the existing strings, but returns a new string containing the text of the joined strings. ... var str1 = "Hello "; var str2 = "world!"; var str3 = " Have a nice day!"; var res = str1.concat(str2, str3); Jul 21, 2021 - Get access to ad-free content, doubt assistance and more! ... In programming, dynamic variable names don’t have a specific name hard-coded in the script. They are named dynamically with string values from other sources. Dynamic variables are rarely used in JavaScript. Dec 02, 2015 - I found three ways to cast a variable to String in JavaScript. I searched for those three options in the jQuery source code, and they are all in use. I would like to know if there are any differences between them:
May 27, 2019 - Let’s check out the different ways of converting a value to a string in JavaScript. The preferred way from Airbnb’s style guide is… Well, it is possible, and here in this simple tutorial, I am going to show you how to convert any string into a variable in JavaScript. To do this task, we will use the JavaScript eval () function. Well, this is the function that will play the main role to create variable name from our string. Now see the example below: Jul 28, 2021 - It is far too easy for a bad actor to run arbitrary code when you use eval(). See Never use eval()!, below. The eval() function evaluates JavaScript code represented as a string. ... A string representing a JavaScript expression, statement, or sequence of statements. The expression can include variables ...
Storing The Information You Need Variables Learn Web
Javascript Lesson 6 Variables In Javascript Geeksread
Reverse In Javascript Logic To Find Out Reverse In
4 Ways To Convert String To Character Array In Javascript
Converting An Object To A String Stack Overflow
Angularjs Expressions Array Objects Eval Strings Examples
How To Replace All Occurrences Of A String In Javascript
Javascript String Contains Step By Step Guide Career Karma
How To Convert A String To A Number In Javascript
Beginner Javascript Tutorial 5 Using Variables With Strings
Writing New Functions Javascript For Cats
Javascript Find A Variable String And Save It Into Another
Javascript Object Returns A Subset Of A String W3resource
Javascript Variables A To Z Guide For A Newbie In
Javascript Chapter 10 Strings And Arrays
Strings Math And Dates Javascript 2 Objectives How To
Javascript Strings Properties And Methods
Javascript String Contains Check If A String Contains A
Javascript Variables Progracoding
Valid Javascript Variable Names In Es5 Mathias Bynens
Javascript Console Log With Examples Geeksforgeeks
How To Get Numbers From A String In Javascript
What Will Happen When Object String Array Ware Frozen By
Tools Qa What Is A String In Javascript And Different
Javascript Compare Strings Examples Tuts Make
String Format For Javascript Chuvash Eu
5 Ways To Convert A Value To String In Javascript
Check If A Variable Is A String In Javascript Stack Overflow
Javascript Execute Function By String Name Code Example
How To Declare A Variable In Javascript With Pictures Wikihow
When Is It Advisable To Declare String Number And Boolean As
How To Check If A Variable Is An Array In Javascript
How To Call Function From It Name Stored In A String Using
0 Response to "35 Javascript Var To String"
Post a Comment