27 Remove Last Letter From String Javascript
The last character could be removed by specifying the ending index to be one less than the length of the string. This extracts the string from the beginning of the string to the second to last character. Syntax: // Removing the first character string.splice(1); // Removing the last character string.splice(0, string.length - 1); Example: In this article, we're going to have a look at how to remove last 3 characters from string in JavaScript. 1. String slice() method example. This approach allows to get substring by using negative indexes. So by using 0 and -3 indexes as range we get <0, text.length - 3> text range.
C Program To Find The Last Character Of A String Codevscolor
Javascript provides a wide array of string-handling functions. Removing the last character from a string is a simple task in Javascript. There are two very straightforward ways to go about this ...
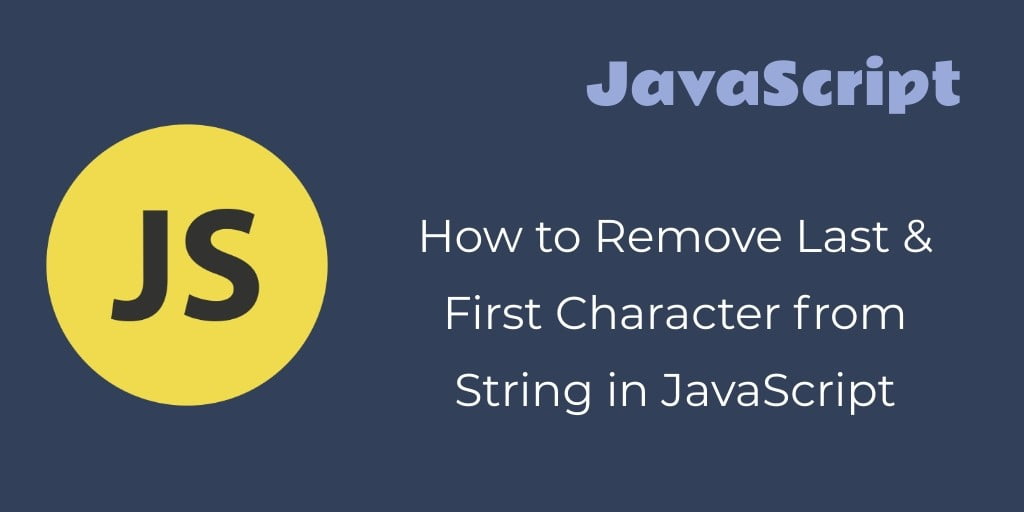
Remove last letter from string javascript. In this article, we're going to show you how to remove the last character of a string no matter if it's a simple comma, a newline character or even a complex unicode character. Using substring(). We can simply use substring() to get the entire string except for the last character like so: . const str = 'foo,bar,baz,'; const newStr = str.substr(0, str.length - 1); console.log(newStr); // output ... Summary: Learn two simple Windows PowerShell methods to remove the last letter of a string.. Hey, Scripting Guy! I have, what should be a simple question. I need to be able to remove only the last character from a string. For example, if I have a string the scripts, I want the string to be the script instead. If I have the number 12345, I want the number to be 1234. You can, in fact, remove the last arr.length - 2 items of an array using arr.length = 2, which if the array length was 5, would remove the last 3 items. Sadly, this does not work for strings, but we can use split() to split the string, and then join() to join the string after we've made any modifications.
Remove last character from string JavaScript. Javascript provides a wide array of string-handling functions. There are two very straightforward ways to go about this task, and either one works fine. Using substring (): The substring function in Javascript takes two arguments, the starting point of the substring, and the ending point of the ... Removing the First and Last Character of Each Word in a String. We can also remove or delete the first and last character of each word in a string. To remove the first and last character, we use the following steps: Split (break) the String based on the space. For each word, run a loop form the first to last letter. How can you remove the last character from a string? The simplest solution is to use the slice() method of the string, passing 2 parameters. THe first is 0, the starting point. The second is the number of items to remove. Passing a negative number will remove starting from the end. This is the solution: const text = 'abcdef' const editedText = text.slice(0, -1) //'abcde' Note that the slice ...
In this article I want to show you how you can remove the last character from any string with the help of JavaScript. Before I start with the explanations, let's first take a look at the code required for this. Here is how to do it: var strold = '1,2,3,'; var strnew = strold.substr (0, strold.length - 1); alert (strnew); 4/4/2020 · You can see a negative number to select from the end of the string. You can use the following code to remove the last character from the string. Syntax str.slice(start, end); Example var str= "Hello World!"; var newstr = str.slice(0, -1); console.log(newstr); Output. We prefer to use the slice() function to remove the last character from the string. 3. 15/7/2018 · Use the substring () function to remove the last character from a string in JavaScript. This function returns the part of the string between the start and end indexes, or to the end of the string. Syntax: JavaScript. str.substring (0, str.length - 1);
Use the replace() Method to Remove the Last Character From a JavaScript String. We can also remove the last character from a string by using the replace() function. It takes a regular expression as an input and the replacement for the results of the regular expression. $ character is used to match the end of the input. Use the JavaScript substring () function to remove the last character from a string in JavaScript. with the using javascript trim string functions to string slice. This function String returns the part of the string between the start part as well as end indexes, or to the end of the string. The nchar () function returns the string's length so that 1, nchar (name)-2 specifies the substring range from the beginning to the third last character. The above example code removes the last two characters from the given string. We can also pass a string vector or a column name to the substr () function.
Javascript remove last comma (',') from a string using slice () The slice (beginIndex, endIndex) method of javascript returns a copy as a new string. The beginIndex and endIndex describe from where the extraction needs to be started and ended. If any of the indexes are negative, it is considered -> string. length - index. Use the substring () string function to remove the last character from a string in Java Script source code. This javascriptstring function simple returns the section of the string between the start and end indexes, or to the get the data string end of the string. Read Also: Vuejs Autocomplete Input Tags component The charAt () method returns the character at the specified index in a string. You can use this method in conjunction with the length property of a string to get the last character in that string.
16/2/2012 · var str = "I want to remove the last word."; var lastIndex = str.lastIndexOf(" "); str = str.substring(0, lastIndex); Get last space and then get sub string. The most common way to trim the last character is by using the JavaScript slice method. This method can take up to two indexes as parameters and get the string between these two values. To keep the whole string and remove the last character, you can set the first parameter to 0 and pass the string length - 1 as the second parameter. Using for loop to Remove Last Character From String in Python. We can also use the for loop to remove or delete the last character from the string. We will take out the length of the string from the len() function. Then we will take an empty string. After that, we will run the loop from 0 to l-2 and append the string into the empty string.
Another way to remove the last character from a string is by using a regular expression with the replaceAll () method. This method replaces all occurrences of the matched string with the given string. The replaceAll () method takes two input parameters: the regular expression and the replacement string. In the above code, we first remove the first character from a string using substring() method then we chain it to slice() method to remove the last character.. Using replace method. The replace method takes two arguments, the first argument is a regular expression and the second argument is replacement.. Example: As you can see from the above function, in the input string value there is whitespace at the end of the string which is successfully removed in the final output. Using slice () method slice () method retrieves the text from a string and delivers a new string. Let's see how to remove the first character from the string using the slice method.
If you wanted to remove say 5 characters from the start, you'd just write substr(5) and you would be left with IsMyString using the above example.. Remove the last character. To remove one character off of the end of a string, we can use the String.slice method. The beautiful thing about slice is it accepts negative numbers, so we don't need to calculate the length like many other ... 25/9/2019 · In this tutorial, we are going to learn about different ways to remove the last character from string in JavaScript. First way: slice method. In JavaScript, we have slice() method by using that we can remove the last character from a string instead of modifying the original string. Example: I'm trying to remove last 3 zeroes here: 1437203995000 How do I do this in JavaScript? I'm generating the numbers from new date() function. Stack Overflow. ... Remove last 3 characters of string or number in javascript. Ask Question Asked 6 years, 1 month ago. Active 3 months ago. Viewed 135k times
The easiest way is to use the built-in substring() method of the String class.. In order to remove the last character of a given String, we have to use two parameters: 0 as the starting index, and index of the penultimate character. We can achieve that by calling String's length() method and subtracting 1 from the result.. However, this method is not null-safe and if we use an empty string ... The slice () method extracts the text from a string between the specified indexes and returns a new string. You can use the following code, which removes the last character from the string: 1 2 There are many methods to solve this problem some of them are discussed below: Method 1: Using charAt () function: This function returns the character at given index. First count number of characters in a given string by using str.length function. Since the indexing starts from 0 so use str.charAt (str.length-1) to get the last character of string.
Use the start and end parameters to specify the part of the string you want to extract. The first character has the position 0, the second has position 1, and so on. Tip: Use a negative number to select from the end of the string. Method 1: Using slice() Method: The slice() method extracts the part of a string and returns the extracted part in a new string. If we want to remove the first character of a string then it can be done by specifying the start index from which the string needs to be extracted. We can also slice the last element. Syntax:
Java String Replace Replacefirst And Replaceall Method
Php Functions Remove Last Character From String In Php
Write A C Program To Delete First And Last Element In String
Removing The Last Character From A String Using Salesforce
Write A C Program To Delete First And Last Element In String
Two Simple Powershell Methods To Remove The Last Letter Of A
Javascript Basic Remove A Character At The Specified
Notepad Remove First Or Last Character Of Every Line Rochcass
How To Remove A Particular Character From A String In C
Remove The Last 2 Characters Of A String When The 2nd To Last
Javascript Chop Slice Trim Off Last Character In String
How To Remove The Last Character Of A String In Javascript
Php Functions Remove Last Character From String In Php
How To Remove Last Character From A String In Swift Dev
Javarevisited How To Remove First And Last Character Of
Remove N Number Of Characters From The End Of The Strings In
How To Remove First And Last Character S From A String In
Remove Leading And Trailing Whitespace From Javascript String
How To Remove Last Character From String Jquery
Remove Character At Index From String Javascript Code Example
11 Ways To Check For Palindromes In Javascript By Simon
Javascript Remove First Last Specific Character From String
C Java Php Programming Source Code Javascript Remove
Java Program To Remove First Character Occurrence In A String
How To Remove Portion Of A String After Certain Character In
Cut Off End Of String Javascript Code Example
0 Response to "27 Remove Last Letter From String Javascript"
Post a Comment