27 Javascript Random Number Generator Between Range
Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. Generate random whole numbers within a range javascript Nov 19, 2020 - Whether for performance testing or any other purpose, it is sometimes useful to generate pseudorandom numbers.
Generate Random Number In Range In Javascript
How to generate random number between two numbers using JavaScript
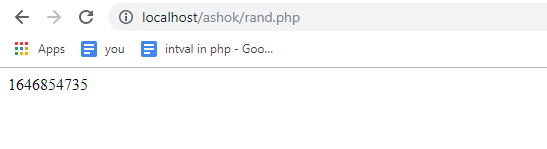
Javascript random number generator between range. 2/1/2020 · Generate a Random Number in Range With JavaScript/Node.js. by Marcus Pöhls on January 02 2020, tagged in , 3 min read. JavaScript has built-in methods to generate a single, random number. You can leverage this feature to create your own method generating a random number in a range between two other numbers. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. this is my take on a random number in a range, as in I wanted to get a random number within a range of base to exponent. e.g. base = 10, exponent = 2, gives a random number from 0 to 100, ideally, and so on. if it helps use it, here it is:
A mathematically correct random number generator library for JavaScript. - GitHub - ckknight/random-js: A mathematically correct random number generator library for JavaScript. A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded): This article describes how to generate a random number using JavaScript. Method 1: Using Math.random () function: The Math.random () function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range.
Random Number Generated : 0.2894437916976895. The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive).This random number can then be scaled according to the desired range. Syntax: Math.random(); Parameters: This function does not accepts any parameter. Let us see some of the examples to generate random numbers: 1. Use of Math.random () function. We have the Math. random () function in JavaScript to deal with the random numbers. This number always return less than 1 as a result. This will always give the result in the form of a decimal point. 2. Math.random() generates our random number between 0 and ≈ 0.9. Before multiplying it, it resolves the part between parenthesis (myMax - myMin + 1) because of the grouping operator ( ) . The result of that multiplication is followed by adding myMin and then "rounded" to the largest integer less than or equal to it (eg: 9.9 would result in 9)
A lot of times you will need to create a random number in JavaScript within a given range. In fact, let's say you need to randomly calculate a basketball result, you know that score will be between 70 and 130 (for example ;)). So how do you generate numbers from a range. The function below will give you a random number (float) within a range: A formula for generating a random number within a given range using JavaScript is as follows: Math.floor(Math.random() * (UpperRange - LowerRange + 1)) + LowerRange; So to generate a random number between 25 and 75, the equation would be Math.random () in JavaScript generates a floating-point (decimal) random number between 0 and 1 (inclusive of 0, but not 1). Let's check this out by calling: console .log ( Math .random ()) This will output a floating-point number similar to: 0.9261766792243478.
So now, to get a random value you would do the following: const x = Math.random () * (max - min) Here x is the random value. Currently, max is excluded from the interval. To make it inclusive, add 1. Also, you need to add the min back that was subtracted earlier to get a value between [min, max). const x = Math.random () * (max - min + 1) + min. 5/10/2009 · Use this function to get random numbers between given range. function rnd(min,max){ return Math.floor(Math.random()*(max-min+1)+min ); } JavaScript: get a random number from a specific range - random.js
18/9/2020 · Generating n random numbers between a range - JavaScript. We are required to write a JavaScript function that takes in a number, say n, and an array of two numbers that represents a range. The function should return an array of n random elements all lying between the range provided by the second argument. In JavaScript, you can generate a random number with the Math.random () function. Math.random () returns a random floating-point number ranging from 0 to less than 1 (inclusive of 0 and exclusive of 1) The above program will show an integer output between min (inclusive) to max (inclusive). First, the minimum and maximum values are taken as ... Home JavaScript Generate random integer between two numbers using JavaScript September 13, 2017 Random number generation is the generation of a sequence of numbers or symbols that cannot be reasonably predicted better than by a random chance, usually through a random-number generator (RNG).
Cool. In Ruby this would be as simple as random(16), but in JavaScript we have to write this function ourselves. And we just did! One second though. What I wanted was a number between one and fifteen, not zero and fifteen. I can achieve that by adding one to the whole thing and subtracting one from the top end: function myRandomIntByMax(n ... 19/8/2020 · How to create a random number between a range JavaScript. Javascript Web Development Object Oriented Programming. Our job is to create a function, say createRandom, that takes in two argument and returns a pseudorandom number between the range (max exclusive). The code for the function will be −. Questions: Is there a way to generate a random number in a specified range (e.g. from 1 to 6: 1, 2, 3, 4, 5, or 6) in JavaScript? Answers: If you wanted to get ...
To generate a random number in JavaScript, simply use the following code: ? 1. var randomnumber=Math.floor (Math.random ()*11) where 11 dictates that the random number will fall between 0-10. To increase the range to, say, 100, simply change 11 to 101 instead. MOD produces a value in the range of zero to <ModLimit> -1. RANDOM generates a random number within the limits imposed by the other functions. For example, to generate a random number between 1 and 100, use the following expression: ABS(MOD(RANDOM(1), 100)) +1 . Note: To truly guarantee a random number across large numbers of rows, calculate ... 2. Generating Random Numbers in a Range. 2.1. Math.random. Math.random gives a random double value that is greater than or equal to 0.0 and less than 1.0. Let's use the Math.random method to generate a random number in a given range [min, max): public int getRandomNumber(int min, int max) { return ( int) ( (Math.random () * (max - min)) + min); }
Apr 16, 2021 - So, to create a random number between 0 and 10: ... Math.random() is a useful function, but on its own it doesn’t give programmers an easy way to generate pseudo-random numbers for specific conditions. There may be a need to generate random numbers in a specific range that doesn’t start ... generate random number in javascript in range; JS can math.random end on 1; javascript generate random number between 1000 and 9999; generate(6, 1, 49); in js; generate random number between 1 and 3 javascript; jas random range; Math.floor(Math.random() * MAX_HINTS)\ random number in javascript in range; js randrange May 17, 2020 - In JavaScript, we can generate random numbers using the Math.random() function. Unfortunately, this function only generates floating-point numbers between 0 and 1. In my experience, it's much more common to need a random integer within a certain range. For example, a random number between 10 and 20.
In this example, 1 is considered the starting number, and 5 is the number of the possible results (1 + start (5) - end (1)). Describing Math.random()and Math.floor()¶ Math.random() is used for returning return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). It can then be scaled in line with the ... Apr 01, 2018 - For many situations ranging from coin toss operations to procedural animations, you will want to work with numbers whose values are a bit unpredictable. You will want to work with numbers that are random. Generating a random number is easy. It is built-in to JavaScript and exposed via the infamous ... Generate random no. between two numbers in javascript ... Write a JavaScript function to generate a random integer.
The random () method returns a random number from 0 (inclusive) up to but not including 1 (exclusive). 2/10/2017 · JavaScript is a front-end scripting language. Many a times we need to generate random numbers between a range, say for an input. JavaScript has its own built in Math library and under that we have random function, which is like 'Math.random()', but it generates floating numbers between 0 and 1. So, manipulating it with some other Math functions and simple maths logic, we can generate random numbers between a range. The fastest way to obtain a random integer number in a certain range [a, b], excluding one value c, is to generate it between a and b-1, and then increment it by one if it's higher than or equal to c. This solution only has a complexity of O (1). One possibility is not to add 1, and if that number comes out, you assign the last possible value.
Generate a Random Number Between 1 and User-Defined Value in JavaScript Generate a Random Number Between Two Numbers in JavaScript This tutorial will learn how to generate a random number between two numbers in JavaScript. We will use the method Math.random() that generates a random floating number between 0.0 and 0.999. Apr 01, 2021 - There was a recent feature request at work that called for picking a random number to represent an index for an array of objects. We needed this logic to create a new, smaller collection of randomly… Random Number between Range in JavaScript. December 28, 2020 Red Stapler 0. Math.random () is a function that returns a pseudo-random floating numbers between 0 and 1 (0 is inclusive, 1 is exclusive) We usually multiply the result with other numbers to scale the randomized value.
Aug 28, 2018 - A short guide to .ceil, .floor, and .round. “Using Math.random() in JavaScript” is published by Joe Cardillo. 9/2/2011 · This function can generate a random integer number between (and including) min and max numbers: function randomNumber(min, max) { if (min > max) { let temp = max; max = min; min = temp; } if (min <= 0) { return Math.floor(Math.random() * (max + Math.abs(min) + 1)) + min; } else { return Math.floor(Math.random() * (max - min + 1)) + min; } } The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range. The implementation selects the initial seed to the random number generation algorithm; it cannot be chosen or reset by the user.
Javascript queries related to "javascript generate random number between 1000 and 9999" random number between 1 and 4 in javascript; three random numbers between 1 and 10 javascript how to do a random number generator in js. C1 = Math.floor (Math.random () * 100 + 1); random number between 0 and 2 javascript. javascript random number between 1 and 300. random number in jquery. generate random number between 1 and 100 javas. generate random number between 1 and 100 javascript. rand function js. Nov 19, 2020 - Generating an array of random integers in JavaScript is surprisingly easy. Here are 3 ways to do it.
How To Select A Random Element From Array In Javascript
Why Is Randomness So Hard In Javascript Adtmag
How To Create Random Numbers In Your Html5 Game Dummies
Random Number Generator In Javascript Top 6 Examples To Learn
Github Mikemcl Bignumber Js A Javascript Library For
Create A Random Number Generator With Javascript Orangeable
Basic Javascript 105 111 Generate Random Whole Numbers Within A Range Freecodecamp
How To Generate Random Date Between Two Dates Quickly In Excel
Random Number Generator Idea Bubble Forum
Random Number Generator In Python Examples Of Random Number
How To Generate A Random Number Between Two Numbers In Javascript
Generate Random Whole Numbers Within A Range Freecodecamp Basic Javascript
Java Random Number Generator How To Generate Integers With
Adding Javascript Javascript Guidelines Document Write
How To Implement A Random Number Generator In Php Edureka
Random Number In Javascript Practical Example
A Simple Low Latency Real Time Certifiable Quantum Random
Excel Formula Random Number Between Two Numbers Exceljet
How To Generate Random Numbers With Decimals In Excel
How To Generate Gaussian Distributed Numbers Alan Zucconi
React Native Generate Random Number Between 1 To 100 In
Math Random Javascript Between Two Numbers Design Corral
Number Guessing Game Using Javascript Geeksforgeeks
How To Build A Random Quote Generator With Javascript And
0 Response to "27 Javascript Random Number Generator Between Range"
Post a Comment