32 Convert Blob To File Javascript
Blob. ArrayBuffer and views are a part of ECMA standard, a part of JavaScript. In the browser, there are additional higher-level objects, described in File API, in particular Blob. Blob consists of an optional string type (a MIME-type usually), plus blobParts - a sequence of other Blob objects, strings and BufferSource. In this tutorial I will show you how to retrieve a remote file and then create a blob (similar to file object). This can be useful if you want to analyze a text/binary remote file on frontend using JavaScript. This simple code converts a remote file to a blob object.
Nov 15, 2013 - I want to send image data via web socket and display it in a canvas, but it's very difficult to do. I tried so many ways to convert blob to image data, I just couldn't.
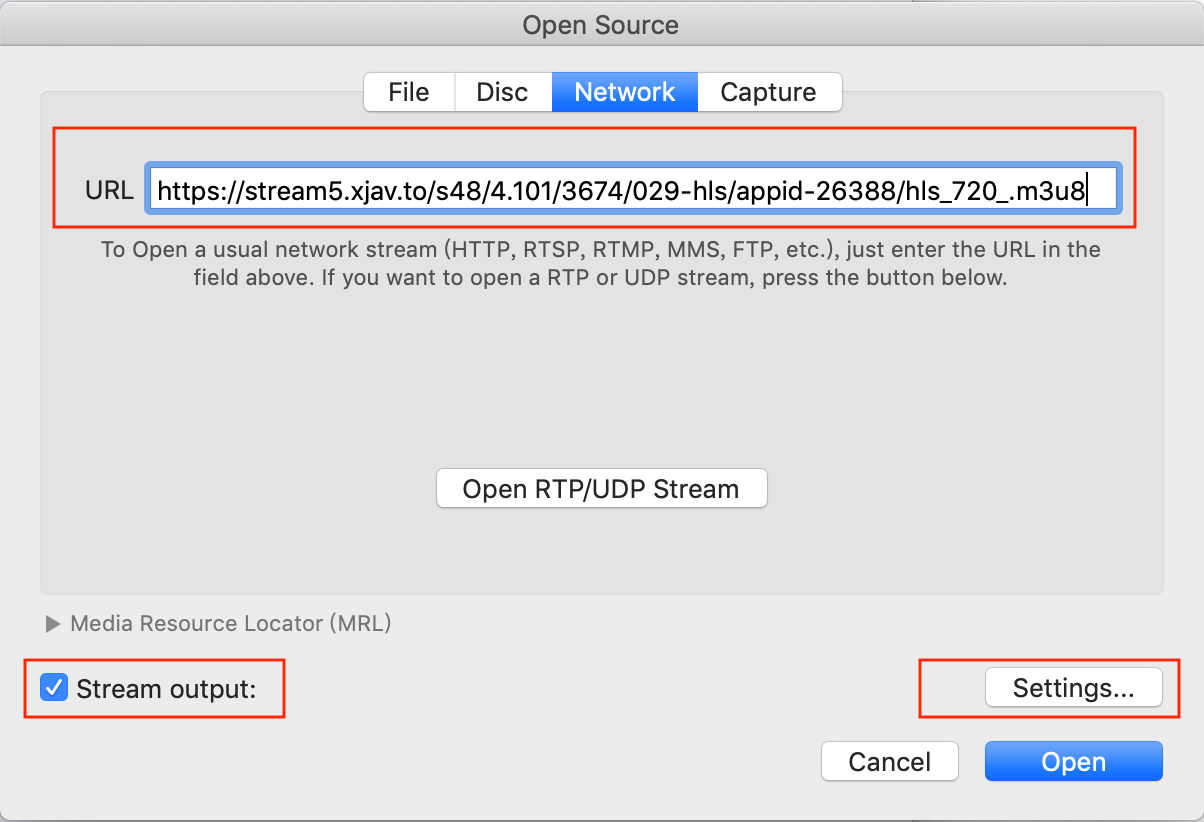
Convert blob to file javascript. This approach will be equivalent to the action that an user does when he drags and drop a file into a file input. Implementation. To get started, we need to convert a base64 string into a "file" using Javascript, to do that, we are going to convert a Base64 string to a Blob and that will be interpreted as a File in our server. Interactive API reference for the JavaScript Blob Object. Blobs are immutable objects that represent raw data. File is a derivation of Blob that represents data from the file system. Use FileReade Converting JavaScript file objects or blobs to Base64 strings can be useful, for example when we can only send string based data to the server. In this blog post, we'll explore how to use JavaScript to generate a Base64 string and a DataURL from a file object. Blob: Blob is a fundamental data type in JavaScript.
This function converts a Blob into a File and it works great for me. Vanilla JavaScript. function blobToFile(theBlob, fileName){ //A Blob() is almost a File() - it's just missing the two properties below which we will add theBlob.lastModifiedDate = new Date(); theBlob.name = fileName; return theBlob; } TypeScript (with proper typings) try { return !!new Blob(); The URL.createObjectURL() static method creates a DOMString containing a URL representing the object given in the parameter. The URL lifetime is tied to the document in the window on which it was created. The new object URL represents the specified File object or Blob object. To release an object URL, call revokeObjectURL().
Step 2: DataURI is converted into a Blob (Binary Large Object-Stores any kind of data in binary format such as images, audio, and video)object file. Step 3: Blob object file is appended in FormData object instance {key : value}. Example: Suppose there is a DataURI for an image of type 'gif'. Input: URI for an image. Program: While we are working with Javascript we may find our self in a situation where we need to let users download blob as File. So In this post, I'll share basic javascript function which allows us to download blob as a file in the browser. Suppose you have input type file on a form. <input type=file multiple>. Input type file used to upload a files from a browser. Multiple options enable to upload multiple files. FileList object holds the file information When user upload an file (s), Following is an example for reading the input type in pure javascript.
This function converts a Blob into a File and it works great for me. Vanilla JavaScript. function blobToFile(theBlob, fileName){ //A Blob() is almost a File() - it's just missing the two properties below which we will add theBlob.lastModifiedDate = new Date(); theBlob.name = fileName; return theBlob; } TypeScript (with proper typings) File is a sub-class of Blob, so all File instances are also valid Blobs. Please note that this requires a platform path, and not a file URL . Yes, FileReader is available to addons. We're first creating the file-like JavaScript Object with new Blob, passing the body of the document, in our case, is our Array buffer. Then we're composing our file name.
How to convert Blob to File in JavaScript, I need to upload an image to NodeJS server to some directory. I am using connect-busboy node module for that. I had the dataURL of the Insert Blob into Database Export Blob From SQL Server and save it as a file. For demo purpose, we want to save documents ... Programming Tips - How can I convert a Blob to a File?. Date: 2016may10 Language: javaScript Platform: web Q. How can I convert a Blob to a File? A. The File constructor accepts a Blob but not in all browsers so this seems like the best way: I want to convert it into file object ..and should be able to append in a Formdata. Answer. A Blob is a File like object, or more exactly, a File object is a Blob with a name property.. You can create a File object from a Blob thanks to the File(blob, name) constructor, but this will be useless in almost every use cases*.. All you can do with a File can be done with a Blob.
new File([], 'foo.txt').constructor.name === new Blob([]).constructor.name //false If you must convert a file object to a blob object, you can create a new Blob object using the array buffer of the file. See the example below. let file = new File(['hello', ' ', 'world'], 'hello_world.txt', {type: 'text/plain'}); FileReader.readAsDataURL () The readAsDataURL method is used to read the contents of the specified Blob or File. When the read operation is finished, the readyState becomes DONE, and the loadend is triggered. At that time, the result attribute contains the data as a data: URL representing the file's data as a base64 encoded string. As mentioned in the chapter Blob, FileReader can read not just files, but any blobs. We can use it to convert a blob to another format: readAsArrayBuffer(blob) - to ArrayBuffer, readAsText(blob, [encoding]) - to string (an alternative to TextDecoder), readAsDataURL(blob) - to base64 data url.
Converting JavaScript file objects or blobs to Base64 strings can be useful. For example when we can only send string based data to the server. In this tutorial we'll explore how to use JavaScript to generate a Base64 string and a DataURL from a file object. Convert Blob to String in JavaScript. Using the FileReader API readAsText method. John Paul Ada. Follow. Mar 27, ... A Blob object represents a file-like object of immutable, raw data. Blobs ... @Dave I edited my question to explain how the image file is saved. If however your server will not work with base64 encoding let me know and I'll include code how to convert it to a blob (binary encoding). You can upload it to your server via a simple ajax call, if you need help with that also let me know. - YAHsaves May 26 '18 at 0:18
JavaScript has two primary ways to represent binary data in the browser. ArrayBuffers/TypedArrays contain mutable (though still fixed-length) binary data which you can directly manipulate. Blobs contain immutable binary data which can only be accessed through the asynchronous File interface. The send method of the XMLHttpRequest has been extended to enable easy transmission of binary data by accepting an ArrayBuffer, Blob, or File object. The following example creates a text file on-the-fly and uses the POST method to send the "file" to the server. This example uses plain text, but you can imagine the data being a binary file instead. How to use js to convert blob objects or json objects to file objects---the front end automatically uploads json files to the server, Programmer Sought, the best programmer technical posts sharing site.
1 week ago - The Blob object represents a blob, ... data, or converted into a ReadableStream so its methods can be used for processing the data. Blobs can represent data that isn't necessarily in a JavaScript-native format. The File interface is based on Blob, inheriting blob functionality and expanding it to support files ... I was using Nextjs and trying to convert canvas to an image file. I just use the other guy's solution but the created file was empty. So if this is your problem you should mention the size property in the file object. new File([Blob], `my_image${new Date()}.jpeg`, { type: "image/jpeg", lastModified: new Date(), size: 2, }); Web browsers provide a variety of data primitives that web developers use to manage, manipulate, and store data - from plain text, to files, images, videos and more. However, using them correctly and effectively can be confusing. One such example is converting a base64 string to a blob using JavaScript. A blob represents binary data […]
If you need to send it over ajax, then there's no need to use a File object, only Blob and FormData objects are needed.. As I sidenote, why don't you just send the base64 string to the server over ajax and convert it to binary server-side, using PHP's base64_decode for example? Anyway, the standard-compliant code from this answer works in Chrome 13 and WebKit nightlies: Convert a Blob to a File using JavaScript March 10th, 2021. Sometimes you get a Blob object when instead you would like to have a File. For example, when you use blob() on a fetch response object. In this quick tutorial we'll explore how to turn a Blob into a File. Fetch. The FileReader method readAsText() is an older method that performs a similar function. It works on both Blob and File objects. There are two key differences: Blob.text() returns a promise, whereas FileReader.readAsText() is an event based API. Blob.text() always uses UTF-8 as encoding, while FileReader.readAsText() can use a different encoding depending on the blob's type and a specified ...
We'll get to URL.createObjectURL() in a bit, but the point is that you've seen that we can access file "data" using just Javascript. Blobs & Data URIs. I've been pretty vague in my use of the word "data" so far, so let's clear that up. We can represent file contents in two primary forms, depending on how you're using it: Aug 02, 2018 - I'm recording an audio into an empty file using Cordova Media. To upload it I need to have the content type on the file. I'm trying to convert the File into a Blob so I can set the content type, 2/6/2020 · Blob is a fundamental data type in JavaScript. Blob stands for Binary Large Object and it is a representation of bytes of data. Web browsers support the Blob data type for holding data. Blob is the underlying data structure for the File object and the FileReader API. Blob has a specific size and file type just like ordinary files and it can be stored and retrieved from the system memory.
Dec 19, 2014 - I need to convert a blob to file i javascript. ... This is return from a function reading a cropped images. The old upload function are using $files as input. I want to convert that blob to file, and then set the name and type in that object. How do I do this?? After you send your blob binary file to the backend, you can use node's ffmpeg library to convert your file to another format. First, run npm install ffmpeg , then copy the code below. Jan 01, 2021 - 1.6m members in the javascript community. All about the 𝚓𝚊𝚟𝚊𝚜𝚌𝚛𝚒𝚙𝚝 programming language!
You can apply a script from anywhere on the web to your Pen. Just put a URL to it here and we'll add it, in the order you have them, before the JavaScript in the Pen itself. If the script you link to has the file extension of a preprocessor, we'll attempt to process it before applying. How to convert Blob to File in JavaScript Tags: blob, file-upload, javascript, node.js. I need to upload an image to NodeJS server to some directory. I am using connect-busboy node module for that. I had the dataURL of the image that I converted to blob using the following code:
Html5 Blob Implementation File Download Function Example Code
Creating Files On The Client Side Via The Blob Api And
Use Azcopy To Upload Data To Azure Blob Storage
Downloading Text Using Blobs Url Createobjecturl And
Convert Image Into Blob Before Uploading Jquery Image Blob
Blob To Text Javascript Code Example
Several Binary Classes Blob Arraybuffer And Buffer In Js
Convert String To Blob Javascript Code Example
Several Binary Classes Blob Arraybuffer And Buffer In Js
Convert Blob Data To Raw Buffer In Javascript Or Node Issue
Retrieve Image And File Stored As A Blob From Mysql Table
Create A Blob Link From Csv Data Javascript Code Example
Convert Blob To Clob In Oracle
Convert Blob Url To File Object With Axios Stack Overflow
How To Convert Pdf To Blob Datatype Help Uipath Community
Pasting Images Into Your App Using File Blobs And Url
Sql Developer Quick Tip Blobs And Images
How To Read A Big Gt 300 Mb Image Or Video File Via Javascript
Blob To String Typescript Code Example
How To Download Video With Blob Url By Jameskrauser Lee
How To Convert Blob Data To Downloadable Excel Csv File In
Several Binary Classes Blob Arraybuffer And Buffer In Js
Upload Image Data In The Cloud With Azure Storage Microsoft
Exporting Multiple Blobs With Oracle Sql Developer
How To Download Video With Blob Url By Jameskrauser Lee
Blob Attributes Losant Documentation
Learn Javascript Blob Object With Examples Cloudhadoop
0 Response to "32 Convert Blob To File Javascript"
Post a Comment