26 Using Arrays In Javascript
Sep 10, 2016 - To kick your JavaScript skills into outer space, everything you see here and more (with all its casual clarity!) is available in both paperback and digital editions. ... The popular way all the cool kids create arrays these days is to use an open and close bracket. In JavaScript, an array is a variable and it can hold different data type elements in it. Different types of data mean, it may contain elements of different data types. An element data type can be numbers, strings, and objects, etc. Declaration of an Array
Array In Javascript Cyberncode Com
Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements both to/from the beginning or the end. In computer science the data structure that allows this, is called deque. Methods that work with the end of the array:
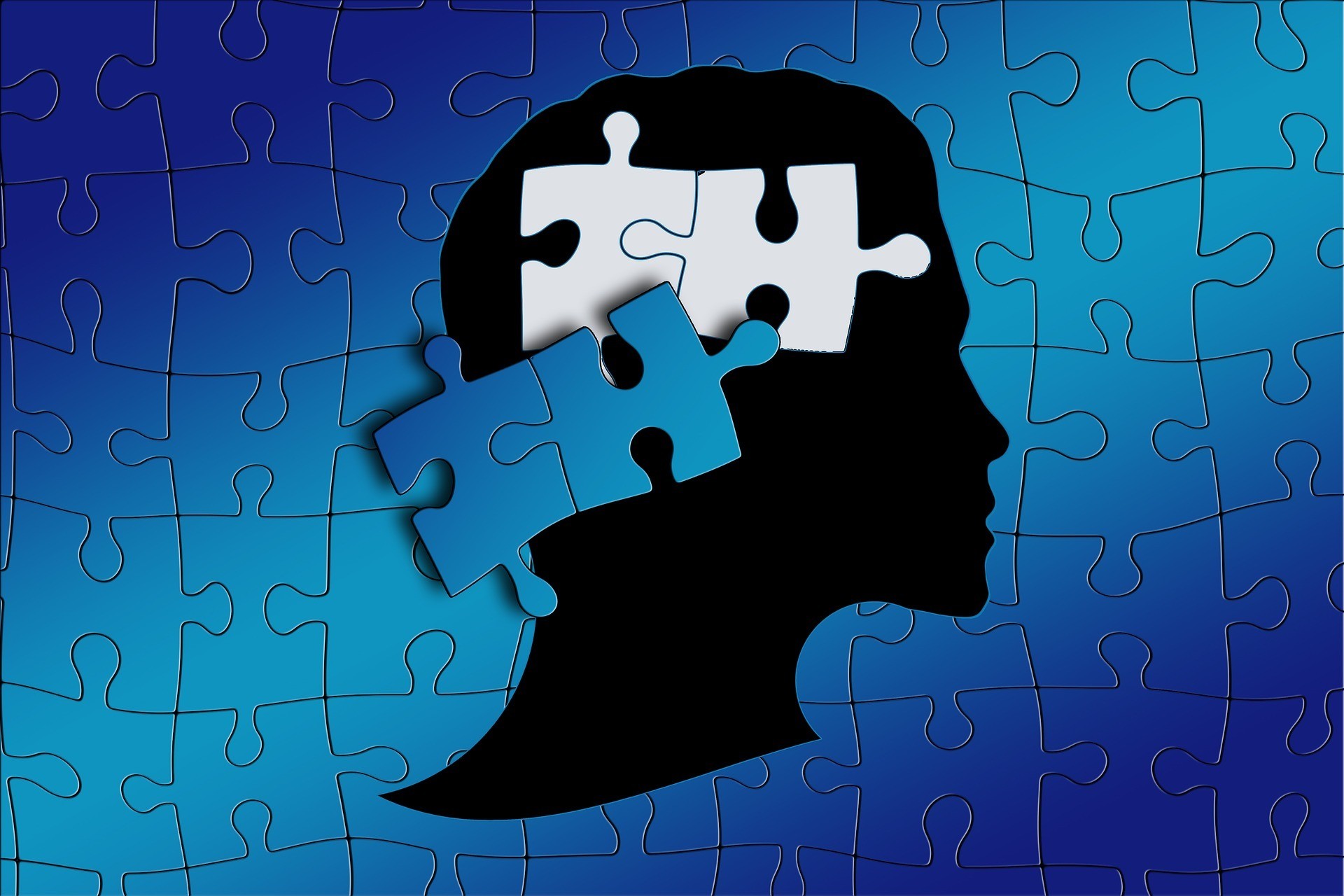
Using arrays in javascript. The JavaScript method toString () converts an array to a string of (comma separated) array values. Aug 28, 2017 - Arrays are an extremely versatile ... in JavaScript. In this tutorial, we learned how to create an array, how arrays are indexed, and some of the most common tasks of working in arrays, such as creating, removing, and modifying items. We also learned two methods of looping through arrays, which is used as a common ... JavaScript arrays are used to save multiple values in a single variable. The array is a single variable that is used to store different elements. It is often used when we want to store a list of elements and access them by a single variable. So, let's define arrays in Javascript.
You can also create an array using JavaScript's new keyword. const array2 = new Array("eat", "sleep"); In both of the above examples, we have created an array having two elements. Note: It is recommended to use array literal to create an array. 9/8/2017 · In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable that stores multiple elements. Declaration of an Array Best way to find if an item is in a JavaScript array? 0. If statement that loops through array not working. Related. 7626. How do JavaScript closures work? 6718. How do I remove a property from a JavaScript object? 5721. How do I include a JavaScript file in another JavaScript file? 7941.
Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person[0] returns John: Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the array, JavaScript arrays are not guaranteed to be dense; this depends on how the programmer chooses to use them. JavaScript Array Methods The Array object has many properties and methods which help developers to handle arrays easily and efficiently. You can get the value of a property by specifying arrayname.property and the output of a method by specifying arrayname.method().
15 Common Operations on Arrays in JavaScript (Cheatsheet) The array is a widely used data structure in JavaScript. The number of operations you can perform on arrays (iteration, inserting items, removing items, etc) is big. The array object provides a decent number of useful methods like array.forEach (), array.map () and more. Jul 20, 2021 - The Array.from() static method creates a new, shallow-copied Array instance from an array-like or iterable object. In Javascript, there are only 6 data types defined - the primitives (boolean, number, string, null, undefined) and object (the only reference type). Arrays do not belong to this list because they are objects as well. This is a common confusion among developers who assume that arrays are a special data type in Javascript.
Learn what is an array and how to use it in JavaScript. Also, learn how to access values from an array. The JavaScript Array.find method is a convenient way to find and return the first occurence of an element in an array, under a defined testing function. When you want a single needle from the haystack, reach for find()!. When to Use Array.find. The function and syntax of find() is very much like the Array.filter method, except it only returns a single element. Here's an optimized array comparison function that compares corresponding elements of each array in turn using strict equality and does not do recursive comparison of array elements that are themselves arrays, meaning that for the above example, arraysIdentical(a, b) would return false.
Jun 09, 2021 - In programming, an array is a collection of elements or items. Arrays store data as elements and retrieve them back when you need them. The array data structure is widely used in all programming languages that support it. In this handbook, I'll teach you all about arrays in JavaScript. You'll The method arr.concat creates a new array that includes values from other arrays and additional items. The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Jul 04, 2020 - I've been told not to use for...in with arrays in JavaScript. Why not? Multidimensional arrays are known in JavaScript as arrays inside another array as they are created by using another one-dimensional array. They can have more than two dimensions. A two-dimensional array is also called a matrix or a table of rows and columns. A good use for array methods like push () and pop () is when you are maintaining a record of currently active items in a web app. In an animated scene for example, you might have an array of objects representing the background graphics currently displayed, and you might only want 50 displayed at once, for performance or clutter reasons.
Arrays were an add-on afterthought to javascript; for that reason you can find various ways of using them listed on programming websites, including references to an Array object. The 'Array' object is however basically obsolete now; in this tutorial we'll look at current best practices. Jul 21, 2021 - In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable ... The Difference Between Array() and []¶ Using Array literal notation if you put a number in the square brackets it will return the number while using new Array() if you pass a number to the constructor, you will get an array of that length.. you call the Array() constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument ...
JavaScript provides many functions that can solve your problem without actually implementing the logic in a general cycle. Let's take a look. Find an object in an array by its values - Array.find Let's say we want to find a car that is red. 18 hours ago - You will learn different ways to loop or iterate JavaScript arrays, including how to use associative arrays or objects to find the values you want. One of the most fundamental programming constructs is the for loop. All languages have some implementation of the for loop and forEach and JavaScript ... JavaScript support various types of array, string array is one of them. String array is nothing but it's all about the array of the string. The array is a variable that stores the multiple values of similar types. In the context of a string array, it stores the string value only.
1) JavaScript array literal The syntax of creating array using array literal is given below: var arrayname= [value1,value2.....valueN]; As you can see, values are contained inside [ ] and separated by, (comma). There are two ways to create arrays in JavaScript. One is by using an array literal method. The other is by using the new keyword. Let's take a closer look at both. Array Literal. All you have to do to create an array using the array literal method is to write everything according to the proper syntax described above! That doesn't seem too ... You can also create an array using JavaScript's new keyword. ... In both of the above examples, we have created an array having two elements. Note: It is recommended to use array literal to create an array.
Sep 11, 2020 - Second, the length of an array is dynamically sized and auto-growing. In other words, you don’t need to specify the array size upfront. ... JavaScript provides you with two ways to create an array. The first one is to use the Array constructor as follows: Array in JavaScript Array is a collection of data items stored under a single name. Array provides a framework for announcing and accessing multiple data Objects should have only one identifier, thereby simplifying the task of data management. We use an array when we have to deal with multiple data items. Arrays in JavaScript are used to store an information set, but it is often more helpful for storing a set of variables of the same type. An array is a single variable in JavaScript that is used to store various elements. When we want to store a list of elements and access them through a single variable, it is often used.
In this tutorial, we'll learn the usage of Array Destructuring in JavaScript ES6 with examples. Destructuring Assignment is a special syntax introduced in JavaScript ES6 that allows us to extract multiple items from an array or object and assign them to variables, in a single statement. Also Read: Object Destructuring 2 days ago - The for...of statement creates a loop iterating over iterable objects, including: built-in String, Array, array-like objects (e.g., arguments or NodeList), TypedArray, Map, Set, and user-defined iterables. It invokes a custom iteration hook with statements to be executed for the value of each ... Cloning Arrays: The Challenge. In JavaScript, arrays and objects are reference types. This means that when a variable is assigned an array or object, what gets assigned to the variable is a reference to the location in memory where the array or object was stored.
Hacks For Creating Javascript Arrays
Javascript Array Methods Declaration Properties Cronj
3 Pragmatic Uses Of Javascript Array Slice Method
Javascipt Arrays How To Create An Array In Javascript
How To Create Array In Javascript Code Example
Javascript Array A Complete Guide For Beginners Dataflair
Javascript Array Distinct Ever Wanted To Get Distinct
Javascript Merge Array Of Objects By Key Es6 Reactgo
How To Search In An Array Of Objects With Javascript
Javascript Array A Complete Guide For Beginners Dataflair
Arrays In Javascript How To Create Arrays In Javascript
Creating Unique Merged Arrays Using Javascript S Set And
How To Merge Two Arrays Using Javascript Stack Overflow
4 Ways To Convert String To Character Array In Javascript
9 Ways To Remove Elements From A Javascript Array
How To Find Even Numbers In An Array Using Javascript
Let S Get Those Javascript Arrays To Work Fast Gamealchemist
How To Use Arrays To Integrate Javascript With Html Dummies
How To Use Javascript To Add To An Array The Web Developer
Javascript Array With Examples
Javascript Array Complete Reference Learnersbucket
Array Can Be Declared Using In Javascript
Everything You Need To Know About Javascript Array Methods
How To Sum Two Arrays Data In Javascript Stack Overflow
Data Structures Objects And Arrays Eloquent Javascript
0 Response to "26 Using Arrays In Javascript"
Post a Comment