23 Javascript Class Within Class
Class inheritance is a way for one class to extend another class. ... (inside our constructor only). For instance, let our rabbit autohide when stopped: ... In JavaScript, there's a distinction between a constructor function of an inheriting class (so-called "derived constructor") and other functions. ... TypeScript is object oriented JavaScript. TypeScript supports object-oriented programming features like classes, interfaces, etc. A class in terms of OOP is a blueprint for creating objects. A class encapsulates data for the object. Typescript gives built in support for this concept called class. JavaScript ES5 or earlier didn't support classes.
Local Class In Abap Java And Javascript Sap Blogs
Jul 20, 2021 - The class declaration creates a new class with a given name using prototype-based inheritance.
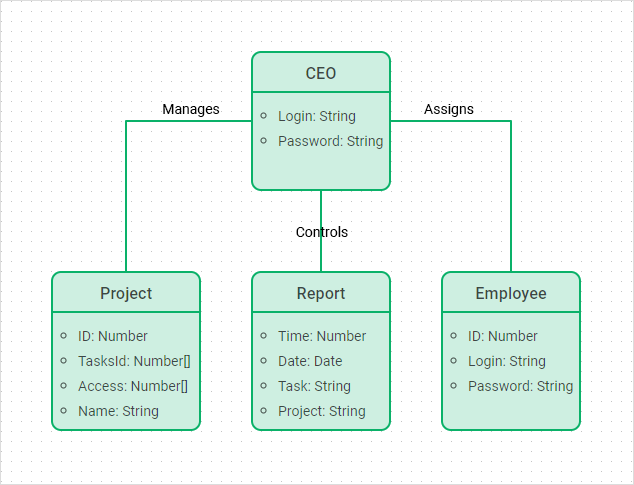
Javascript class within class. In JavaScript, there are some approaches to add a class to an element. We can use the.className property or the.add () method to add a class name to the particular element. Now, let's discuss the approaches to add a class to an element. A JavaScript class is a blueprint for creating objects. A class encapsulates data and functions that manipulate data. Unlike other programming languages such as Java and C#, JavaScript classes are syntactic sugar over the prototypal inheritance. In other words, ES6 classes are just special functions. Apr 28, 2021 - This post will discuss how to get elements by class name using JavaScript and jQuery. ... In jQuery, you can use the class selector ('.class') to select all elements with the given class. ... Note that we can apply the same class to multiple elements within the document.
Java Inner Classes In Java, it is also possible to nest classes (a class within a class). The purpose of nested classes is to group classes that belong together, which makes your code more readable and maintainable. To access the inner class, create an object of the outer class, and then create an object of the inner class: Add all methods of the class, such as sayHi(), to Person.prototype property. Afterward, when we call any method of the object, it is taken from the prototype property. Take a look at this article to learn more about JavaScript classes. Summary. Objects are an important part of JavaScript language. Almost everything in JavaScript is an object. Apr 15, 2017 - The goal is to have prototype ... defined within BobsGarage.Car are not accessible outside the constructor of BobsGarage.Car but instances of them are accessible to derived classes, as shown in the test code. Note: I am referring to the concept of Class in Javascript as defined ...
Mar 29, 2020 - The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively. constructor (name, year) {. this.name = name; this.year = year; } } The example above creates a class named "Car". The class has two initial properties: "name" and "year". A JavaScript class is not an object. It is a template for JavaScript objects. version added: 1.0 jQuery ( ".class" ) class: A class to search for. An element can have multiple classes; only one of them must match. For class selectors, jQuery uses JavaScript's native getElementsByClassName () function if the browser supports it.
1 week ago - The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name(s). A class in JavaScript is created with the special word: function , using this syntax: className = function () { // code of the className class } A class can contain public and private variables (called also properties) and functions (also called methods). The private variables, and functions are defined with the keyword " var ". Apr 16, 2018 - Jeff Mott takes an in-depth look at ES6 classes starting with the basics, then moving on to discuss inheritance, encapsulation, polymorphism and much more.
The class syntax does not introduce the new object-oriented inheritance model to JavaScript. Define a class in Javascript. One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code. Sometimes, we want to select an item with a class within a div with JavaScript. In this article, we'll look at how to select an item with a class within a div with JavaScript. Use document.querySelector. We can use document.querySelector on to select a div and then select an element within it with the given class after that. Class basic syntax In object-oriented programming, a class is an extensible program-code-template for creating objects, providing initial values for state (member variables) and implementations of behavior (member functions or methods). In practice, we often need to create many objects of the same kind, like users, or goods or whatever.
Nov 06, 2019 - Classes in JavaScript are a special syntax for its prototypical inheritance model that is a comparable inheritance in class-based object oriented languages. Classes are just special functions added… 1 week ago - The className property of the Element interface gets and sets the value of the class attribute of the specified element. Nov 23, 2018 - JavaScript is an oddball of a language with numerous approaches to almost any problem. Did ES6 classes help or just muddy the waters? Find out what veteran JavaScript developer Justen Robertson thinks about OOP in JS.
TypeScript offers full support for the class keyword introduced in ES2015. As with other JavaScript language features, TypeScript adds type annotations and other syntax to allow you to express relationships between classes and other types. May 04, 2018 - Understanding prototypical inheritance is paramount to being an effective JavaScript developer. Being familiar with classes is extremely helpful, as popular JavaScript libraries such as React make frequent use of the class syntax. A class is a type of function with keyword class. Class is executed in strict mode, So the code containing the silent error or mistake throws an error. JavaScript classes can have a constructor method, which is a special method that is called each time when the object is created.
If you run the JavaScript above, you will see that "newClass" is added to "intro" after five seconds. It is important to note that the classList.add approach will not work in Internet Explorer version 9 and below. If you do need to support older browsers, then you can use the following approach instead: Dec 13, 2019 - These private static fields are accessible only within the User class. Nothing from the external world can interfere with the limits mechanism: that’s the benefit of encapsulation. ... The fields hold data. But the ability to modify data is performed by special functions that are a part of the class: the methods. The JavaScript ... A class in JavaScript is a type of function, which can be initialized through both function keyword as well as through class keyword. In this article, we will cover the inner class in javascript through the use of a function keyword. Here's an example of the class using the function keyword.
JavaScript is different from other object-oriented languages. It is based on constructors and prototypes rather than on classes. For a long time classes were not used in JavaScript. They were introduced in ECMAScript 2015. Array class in JavaScript: class Array {. constructor () {. this.length = 0; this.data = {}; } } In the above example, create a class Array which contains two properties i.e. length and data, where length will store the length of an array and data is an object which is used to store elements. Function in Array: There are many functions in array ... class ClassWithInstanceField {baseInstanceField = 'base field' anotherBaseInstanceField = this. baseInstanceField baseInstanceMethod {return 'base method output'}} class SubClassWithInstanceField extends ClassWithInstanceField {subInstanceField = super. baseInstanceMethod ()} const base = new ClassWithInstanceField const sub = new SubClassWithInstanceField console. log (base. …
Define Class in JavaScript JavaScript ECMAScript 5, does not have class type. So it does not support full object oriented programming concept as other languages like Java or C#. However, you can create a function in such a way so that it will act as a class. 13/12/2011 · You’ll have to use an index number, to indicate which one of the found elements with that class you want to target. This will select the first one: document.getElementById('theId').getElementsByClassName('theClass')[0]; I’ve never figured out how to select all classes with getElementsByClassName, so that’s why jQuery is so great. One may argue that JavaScript does not have completely nested classes -- there is no way for a class to use parent class scope, for instance, nor would it be meaningful for anything but parent class properties (static declarations), but a class in JavaScript is just an object like any other, so you may as well define one and refer to it with a property on another class:
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. 1 week ago - The Element method getElementsByClassName() returns a live HTMLCollection which contains every descendant element which has the specified class name or names. It can lead to more readable and maintainable code: Nesting small classes within top-level classes places the code closer to where it is used. Inner Classes. As with instance methods and variables, an inner class is associated with an instance of its enclosing class and has direct access to that object's methods and fields.
Dec 11, 2020 - Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics. May 22, 2017 - You said you want to select an element with a specific class and that's what we showed you how to do it. Either you have to provide more information (including an explanation what does not work) or better, learn how to debug JavaScript code: netmagazine /tutorials/javascript-debugging-beginners. Classes in JavaScript There are no classes as such in JavaScript, like there are in Java. Functions can be used to simulate classes to a large degree, but they can't replace classes entirely. Objects in JavaScript, however, can implement the concept of inheritance that is found in other class oriented languages like Java.
In JavaScript, the standard way of selecting an element is to use the document.getElementById("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows: Nov 23, 2018 - Stack Overflow is the largest, most trusted online community for developers to learn, share their programming knowledge, and build their careers. class. A class is a type of function, but instead of using the keyword 'function', keyword 'class' is used to initiate it, and the properties are assigned inside a constructor() method. The constructor() method is called each time the class object is initialized.. Example-1. In the following example, a class called 'company' is created and inside a constructor() method the name of the company ...
Class inheritance, Class inheritance is a way for one class to extend another class. For instance, a function call that generates the parent class: function The call () method is a predefined JavaScript method. It can be used to invoke (call) a method with an owner object as an argument (parameter). A non-static nested class is a class within another class. It has access to members of the enclosing class (outer class). It is commonly known as inner class. Since the inner class exists within the outer class, you must instantiate the outer class first, in order to instantiate the inner class. Apr 05, 2018 - To not break instanceof define Node outside of the scope of a single getter, either in the constructor (disabling the Huffman.Node class property and causing instanceof to work within the namespace of a single Huffman instance, and break outside that), or define Node in a sibling or ancestor ...
Javascript Class Find Out How Classes Works Dataflair
Private Class Fields And Methods In Javascript Classes
Es6 Classes In Javascript Javascript Classes Private
Inheritance In Javascript Classes Akanksha S Blog
Differences In Defining Es6 Class Methods Cmichel
How To Create A Javascript Uml Class Diagram Dhtmlx Diagram
How To Write Classes In Javascript Programmerhumor
Classes In Javascript Samanthaming Com
Javascript Create Class And Call Function Within The Class
Learn Javascript Classes In Depth Reactgo
Javascript Classes Under The Hood By Majid Tajawal Medium
Why Mdn Argues That Js Classes May Cause An Error Stack
Using Classes In Javascript Kirupa
Three Ways To Create A Javascript Class Learn Web Tutorials
Tools Qa How To Use Javascript Classes Class Constructor
Inheritance In Javascript Accelebrate
Tools Qa How To Use Javascript Classes Class Constructor
Private Properties And Methods In Javascript Classes
Private Variables In Javascript By Marcus Noble
How To Work With Classes In Typescript Packt Hub
Constructor In Javascript Two Main Types Of Constructor In
Example Of Class In Javascript Es6 Learn Web Tutorials
0 Response to "23 Javascript Class Within Class"
Post a Comment