23 How To Apply Css Dynamically In Javascript
How to Use JavaScript to Change a Cascading Style Sheet (CSS) Dynamically by Christopher Heng, thesitewizard . It may be useful sometimes to provide your visitors with the option to change the appearance of your website. Perhaps you want to provide theme support for your site. Jun 18, 2013 - Insert css or javascript dynamically / That's a super simple function for adding new css or javascript files in the current document. I didn't test it in all the browsers, because I'm using it in the chrome extension and I need only Chrome supported.
Add Custom Javascript And Css To Wordpress Dynamic Drive Blog
In this tutorial, we will provide you how to apply CSS dynamically in JavaScript. JavaScript provides you to add CSS and change the look of webpage. This includes effects such as text change, background color change, text-color change, etc. on click on a button. And also it provides you to display something on click on a button.
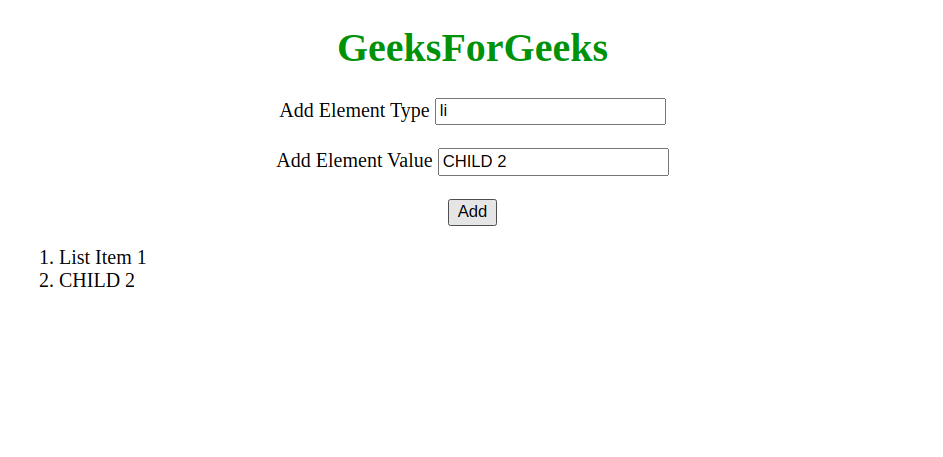
How to apply css dynamically in javascript. You might have the need to dynamically apply CSS properties to DOM elements. What are the APIs browser expose to do that? First, one of the cleanest ways is to add or remove classes from an element, and use classes styling in your CSS. const element = document.querySelector('#my-element') How To Use Pure CSS To Style Web Form Dynamically Plus 12 Awesome JavaScript Plugins August 25, 2009 in Tutorials by OXP Editorial Everybody knows about web forms and it has fast becoming part of our daily online interaction. Adding a CSS class using JQuery. Luckily enough, the JQuery library has a method called addClass, which can be used to add CSS classes to an element. Let's modify the example above to use JQuery instead of vanilla JavaScript: setTimeout(function(){ //Add the CSS class using the JQuery addClass method. $('#intro').addClass('newClass'); }, 5000);
CSS custom properties allow you to: Assign arbitrary values to a property with a name of your choice. Use the var () function to use these values in other properties. Although support for CSS custom properties is a bit of a rocky path at the moment, and some browsers support them under flags that need to be activated or set to true beforehand ... Sometimes, we want to add CSS styles dynamically into our web page with JavaScript. In this article, we'll look at ways to add CSS styles dynamically with JavaScript. Using the window.document.styleSheets Object and the insertRule Method. We can get the first stylesheet for a document with the window.document.styleSheets property. Nov 28, 2019 - An important part of turning static web pages into dynamic web apps is to dynamically change the styling of elements on the page. JavaScript lets us do this by exposing CSS properties that are part…
Apr 28, 2021 - This post will discuss how to dynamically add a CSS stylesheet to an HTML page using JavaScript and jQuery... If you work with jQuery, you may use the .appendTo() method to append a stylesheet at the end of the head element of the current document. Are you looking for applying dynamic CSS in Lightning web Component? Lightning Web Component doesn't allow computed expressions in html markup but there are ways to do so. The implementation of CSS for Lightning Web Components adheres to the W3C standard. You can create a style sheet in the CSS file, and it's automatically applied to the ... For the most part, this setup meets our needs just fine, though in the world of synchronous Ajax design patterns, the ability to also fire up JavaScript/ CSS on demand is becoming more and more handy. In this tutorial, lets see how it's done. Dynamically loading external JavaScript and CSS files
2. Include CSS file. Created a new addCSS() function which also takes the filename as a parameter.. Working of this function is similar to the above function the only difference is - defining a link type of element instead of a script.. Use the file name to define an element href attribute and define other attributes that are required.. Completed Code You can see that this view uses dynamic CSS and static CSS. It also uses one static JS file, and two dynamic JS files. Dynamic references has query string parameters which will impact the rendering of the resource. In DynamicWithModel.js I also wants to use model that I pass to the JS-view and which is respected during JS rendering. I have a javascript function which will add rows dynamically. But the problem is how should i apply css class to those dynamically generated rows.
Feb 25, 2016 - What is a good way to apply styling dynamically (i.e. the value of styles are created at runtime) to HTML elements with JavaScript? I'm looking for a way to package a JavaScript widget (JS, CSS and Change CSS Property With querySelector () in JavaScript querySelector () method is a superset of features offered by the element selection mechanisms. It has the combined power of both getElementsByClassName () and getElementById () methods. With this method, we can select the HTML element the same way while writing CSS classes. Click A Row In The Table Js Dynamically Add Css Style Style Javascript And Css Dynamic Styles And Layers Techotopia Add Edit And Delete Rows From Table Dynamically Using Css How To Dynamically Add And Remove Form Fields Formget
When you can't use a JavaScript or CSS concatenater, this method can be useful for adding scripts and styles to your site on the fly. ... be aware that other JavaScript files may be loaded after this if they are either loaded dynamically or are loaded at the end of the body. Now, in your controller.js file, add this function: controller.js ... Free source code and tutorials for Software developers and Architects.; Updated: 8 Mar 2016 One option to dynamically create and update CSS class in JavaScript: Using Style Element to create a CSS section Using an ID for the style element so that we can update the CSS
Next, we have a js script where we specified function named "addClass" that can take "id", and "className" as params. Inside the function, we iterate DOM to find an element with the id that we passed in button and add a class attribute with a class name that we added when fire "addClass" function. 24/2/2016 · Using Jquery. Use the css () function to apply style to existing elements where you pass an object containing styles : var styles = { backgroundColor : "#ddd", fontWeight: "" }; $ ("#myId").css (styles); You can also apply one style at the time with : $ ("#myId").css ("border-color", "#FFFFFF"); Nov 07, 2011 - I'm making a widget that will be added to external websites, and I have made a page that generates css for them to style it (text color, background color, font size, etc). I end up with a textarea ...
11/11/2009 · One option to dynamically create and update CSS class in JavaScript: Using Style Element to create a CSS section ; Using an ID for the style element so that we can update the CSS class..... HOME HTML5 CSS3 JAVASCRIPT JQUERY BOOTSTRAP4 PHP7 SQL REFERENCES EXAMPLES FAQ SNIPPETS Online HTML Editor ... HTML Tutorial CSS Tutorial JavaScript Tutorial jQuery Tutorial Bootstrap Tutorial PHP Tutorial SQL Tutorial insertRule () method: This method is use to insert some new CSS rule into the current CSS style sheet (bound with some restriction).
We could also use a CSS variable for the hue value and switch the color theme by changing a single value in the stylesheet or dynamically altering it with JavaScript. This is a screen shot of the ... The CSS Object Model is a set of APIs allowing the manipulation of CSS from JavaScript. It is much like the DOM, but for the CSS rather than the HTML. It allows users to read and modify [the] CSS style dynamically. The CSSStyleSheet.insertRule () method inserts a new CSS rule to a stylesheet. 7/5/2020 · This article consists of a sequence of screenshots depicting how CSS can be toggled dynamically using javascript. The CSS of the element is changed dynamically using javascript. 1. No CSS yet applied The following are the HTML elements that create the preceding page when the page is being run. <div id="dvMessage">.
1 week ago - The CSS Object Model (CSSOM), part of the DOM, exposes specific interfaces allowing manipulation of a wide amount of information regarding CSS. Initially defined in the DOM Level 2 Style recommendation, these interfaces forms now a specification, CSS Object Model (CSSOM) which aims at superseding ... In this post, we will learn how to dynamically add CSS class with JavaScript code. It is useful to add interactive dynamic functionality? Submitted by Abhishek Pathak, on October 16, 2017 JavaScript was built to provide interaction. With JavaScript and CSS combined user experience can be significantly improved. Apr 26, 2017 - You can use insertRule to add new CSS rules to a stylesheet using Javascript.
8/12/2020 · Sometimes you need to change the CSS styles of a page dynamically. JavaScript comes handy in such case. Let's start with a typical minimal test case with the following markup structure. <p>This should be green</p> <script><!-- JavaScript code --></script> The first approach we can try is adding a style element just before the target element. Aug 08, 2020 - You will learn how to do DOM manipulation related to the event. In this case, we’ll use the onclick method that we add to the button. 12/6/2020 · View the raw code as a GitHub gist. Then, you need to style those elements by setting a property on the .style object of that HTMLElement.Doing so adds a new inline style to them. “The style property is used to get as well as set the inline style of an element. When getting, it returns a CSSStyleDeclaration object that contains a list of all styles properties for that element with values ...
Action: A JavaScript demonstration. Make a new HTML document, doc5.html. Copy and paste the content from here, making sure that you scroll to get all of it: Make a new CSS file, style5.css. Copy and paste the content from here: Make a new text file, script5.js. Copy and paste the content from here: // JavaScript demonstration var square ... The HTML DOM allows you to execute code when an event occurs. Events are generated by the browser when "things happen" to HTML elements: An element is clicked on. The page has loaded. Input fields are changed. You will learn more about events in the next chapter of this tutorial. 25/11/2020 · In this article, we will see how to dynamically create a CSS class and apply to the element dynamically using JavaScript. To do that, first we create a class and assign it to HTML elements on which we want to apply CSS property. We can use className and classList property in JavaScript. Approach: The className property used to add a class in JavaScript.
Oct 27, 2016 - In this chapter we will begin to explore making dynamic style changes to web pages using JavaScript and CSS. We will begin with some simple changes such as changing the color of some text before moving on to more complex tasks such as hiding, showing and moving blocks of content. Introduction. At this point in the JavaScript section of the Web Standards Curriculum, you've already covered the real basics of JavaScript usage, looked at how to target elements using the DOM, and seen how to manipulate them once you've successfully targeted them.. In this article we will look at how to dynamically update the styling applied to your elements by manipulating your CSS at ... transitionend and its related events are quite helpful when manipulating CSS transitions and animations using JavaScript. Changing a CSS animation from its current values can be done by obtaining the stylesheets in JavaScript, but can be quite involved. In JavaScript, CSS transitions are generally easier to work with than CSS animations.
Unit 3 Dynamic Websites With Css And Javascript Objectives
Dynamically Add And Remove Rows In A Table Using Jquery
How To Dynamically Load Javascript Amp Css Simple Examples
Asynchronously Updating A Webpage In A Standard Html Css Js
Functional Css Dynamic Html Without Javascript By Dmitriy
Static And Dynamic Webpage Development With Html Css
Make Your Css Dynamic With Css Custom Properties Toptal
Add Css And Javascript Files Dynamically Inside Html Head
Forcing Css Animation On Dynamic Containers With Javascript
Override Important Style Property In Javascript
Customizable Dynamic Quiz Plugin With Jquery Quiz Js Free
How To Use Pure Css To Style Web Form Dynamically Plus 12
Dynamically Load And Apply Fonts With Javascript Andreas Wik
How To Dynamically Create And Apply Css Class In Javascript
Learn Javascript Amp Css For Beginners By Creating A Dynamic Page Switcher
How To Dynamically Load Javascript And Css With Ajax Drupal
Understanding How Dynamic Rendering Works Using Html And Css
Call Dynamic Js And Css In C Dzone Web Dev
Dynamically Add Amp Remove List Items Using Javascript Dev
Build A Dynamic App Using Javascript Html And Css By
How To Dynamically Create New Elements In Javascript
How To Do Dynamic Styling In Reactjs With Practical Example Part 12
0 Response to "23 How To Apply Css Dynamically In Javascript"
Post a Comment