30 Timestamp 1 Day Javascript
May 01, 2020 - Despite the JavaScript Date warts, it’s straightforward to add day(s) to a date in JavaScript. While it would be very easy to reach The date and time is broken up and printed in a way that we can understand as humans. JavaScript, however, understands the date based on a timestamp derived from Unix time, which is a value consisting of the number of milliseconds that have passed since midnight on January 1st, 1970. We can get the timestamp with the getTime () method.
Demystifying Datetime Manipulation In Javascript Toptal
Curto e grosso: + new Date() Um operador + dispara o mĂ©todo valueOf no objeto Date e retorna o timestamp (sem qualquer alteraĂ§Ă£o).. Detalhes: Em quase todos os navegadores atuais, vocĂª pode usar Date.now() para obter o UTC timestamp em milissegundos; uma exceĂ§Ă£o notĂ¡vel Ă© o IE8 e anterior (veja tabela de compatibilidade).. Para obter o timestamp em segundos, vocĂª pode usar:
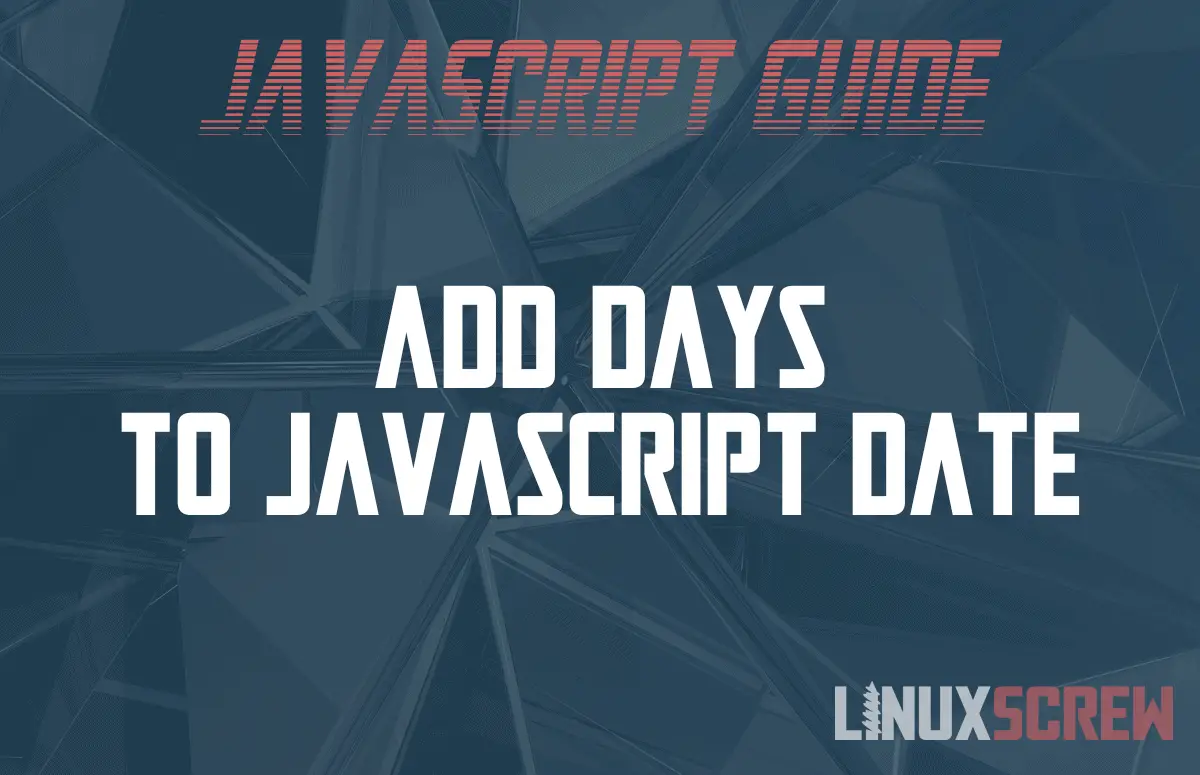
Timestamp 1 day javascript. JavaScript stores dates as number of milliseconds since January 01, 1970, 00:00:00 UTC (Universal Time Coordinated). Zero time is January 01, 1970 00:00:00 UTC. Now the time is: milliseconds past January 01, 1970 JavaScript Date objects represent a single instance in time in a platform-independent format. They contain a number that represents milliseconds since January 1, 1970, UTC. The getDate () instance method returns the day of the month for the defined date. 2/4/2012 · Here is an example of how i would do it: var current = new Date(); //'Mar 11 2015' current.getTime() = 1426060964567var followingDay = new Date(current.getTime() + 86400000); // + 1 day in msfollowingDay.toLocaleDateString(); imo this insures accuracy.
A template was not provided. This is likely because you're using an outdated version of create-react-app · There might be a problem with the project dependency tree. It is likely not a bug in Create React App, but something you need to fix locally · Error: A cross-origin error was thrown. Date.now Method¶. This method can be supported in almost all browsers. The Date.now method is aimed at returning the number of the milliseconds, elapsed since the Unix Epoch. As now() is considered a static method, it is not capable of creating a new instance of the Date object. The example below demonstrates it:
An integer number, between 0 and 6, corresponding to the day of the week for the given date, according to local time: 0 for Sunday, 1 for Monday, 2 for Tuesday, and so on. Examples Using getDay() Feb 13, 2018 - To subtract days to a JavaScript Date object, use the setDate() method. Under that, get the current days and subtract days. JavaScript date setDate() method sets the day of the month for a specified date according to local time. ... You can try to run the following code to subtract 10 days from ...
The Unix epoch (or Unix time or POSIX time or Unix timestamp) is the number of seconds that have elapsed since January 1, 1970 (midnight UTC/GMT), not counting leap seconds (in ISO 8601: 1970-01-01T00:00:00Z). Literally speaking the epoch is Unix time 0 (midnight 1/1/1970), but 'epoch' is often used as a synonym for Unix time. 2 hours ago - This is implemented as moment(timestamp * 1000), so partial seconds in the input timestamp are included. ... Note: Despite Unix timestamps being UTC-based, this function creates a moment object in local mode. If you need UTC, then subsequently call .utc(), as in: ... You can create a Moment with a pre-existing native Javascript ... A JavaScript date is fundamentally specified as the number of milliseconds that have elapsed since midnight on January 1, 1970, UTC. This date and time are not the same as the UNIX epoch (the number of seconds that have elapsed since midnight on January 1, 1970, UTC), which is the predominant base value for computer-recorded date and time values.
how to write a program that displays a message “It’s Fun day” if it's Saturday or Sunday today in javascript These methods can be used for getting information from a date object: Method. Description. getFullYear () Get the year as a four digit number (yyyy) getMonth () Get the month as a number (0-11) getDate () Get the day as a number (1-31) 17/11/2019 · Javascript's Date object accepts timestamp in milliseconds (not as seconds) getFullYear() method returns the full 4 digit year for the provided timestamp. getMonth() method returns the month for the provided timestamp (0-11). This needs to be incremented by 1 to get the actual calendar month.
8/31 + 1 day will become 9/1. The problem with using setDate directly is that it's a mutator and that sort of thing is best avoided. ECMA saw fit to treat Date as a mutable class rather than an immutable structure. How to subtract time from a timestamp? How to subtract seconds from a times. javacodex . Java Examples. Subtracting Time From A Timestamp. Here is an example on how to subtract time from a timestamp in Java. Source: (SubtractTime.java) ... DAY_OF_MONTH, -20); timestamp = new Timestamp (cal. getTime (). getTime () ... Nov 16, 2020 - This tutorial explains how to add days to current date in Javascript
Jul 23, 2021 - The setDate() method changes the day of the month of a given Date instance, based on local time. There are also special considerations to keep in mind when adding time that crosses over daylight saving time. If you are adding years, months, weeks, or days, the original hour will always match the added hour. Adding a month will add the specified number of months to the date. moment([2010, 1, ... An integer number representing the number of milliseconds that has passed since the beginning of 1970 is called a timestamp. It's a lightweight numeric representation of a date. We can always create a date from a timestamp using new Date(timestamp) and convert the existing Date object to a timestamp using the date.getTime() method (see below).
Date.UTC () returns a time value as a number instead of creating a Date object. If a parameter is outside of the expected range, the UTC () method updates the other parameters to accommodate the value. For example, if 15 is used for month, the year will be incremented by 1 ( year + 1) and 3 will be used for the month. This will return the Unix Timestamp in microseconds, which is why we further divide it by 1000 to get the number of seconds - unix = Math.floor(unix / 1000); The rest of the calculations is pretty straightforward - One day has 60 seconds X 60 minutes X 24 hours = 86400 seconds. Since the JavaScript Date timestamp is in the unit of millisecond while the Unix timestamp is in the unit of second, we can multiply 1000 to convert the Unix timestamp to JavaScript timestamp. If the Unix timestamp is 1607110465, then the JavaScript timestamp is 1607110465000.
Add 1 day to timestamp. alexeynl over 6 years ago. Is there any way to substact add 1 day to timestamp: 1. On FlexConnector side. 2. On Manager side using variables. I tried to use such monstrous expression in FlexConnector properties file to achive this with no luck: Definition and Usage. The getTime() method returns the number of milliseconds between midnight of January 1, 1970 and the specified date. Example JavaScript setDate Method Output Wed Dec 20 2017 11:20:25 GMT+0530 (India Standard Time)
JavaScript has a built-in Date object that stores the date and time and provides methods for handling them. To create a new instance of the Date object, use the new keyword: const date = new Date (); The Date object contains a Number that represents milliseconds passed since the Epoch, that is 1 January 1970. Mar 11, 2015 - I have a current Date object that needs to be incremented by one day using the JavaScript Date object. I have the following code in place: 27/2/2009 · function toTimestamp ( year, month, day, hour, minute, second){. var datum = new Date(Date. UTC( year, month -1, day, hour, minute, second)); return datum. getTime()/1000; } Notice that when adding in the month you need to minus the value by 1. This is because the function requires a month value between 0 and 11.
Sep 09, 2010 - I need to increment a date value by one day in JavaScript. For example, I have a date value 2010-09-11 and I need to store the date of the next day in a JavaScript variable. How can I increment a... To offer protection against timing attacks and fingerprinting, the precision of Date.now() might get rounded depending on browser settings. In Firefox, the privacy.reduceTimerPrecision preference is enabled by default and defaults to 20µs in Firefox 59; in 60 it will be 2ms. If you click the save button, your code will be saved, and you get a URL you can share with others · By clicking the "Save" button you agree to our terms and conditions
Hi, in this tutorial, we are going to talk about the different ways through which we can get the current date-time or timestamp in javascript. Get Timestamp in javascript. There are a lot of ways through which one can easily get the current timestamp using built-in modules as well as using external libraries like lodash, moment, underscore, and ... select current_timestamp - 1 ts from dual; TS ----- 01/06/2010 10:02:06 AM 0 · Share on Twitter Share on Facebook Frank Kulash Member, Moderator Posts: 40,814 Red Diamond Mar 15, 2006 - For a better experience, please enable JavaScript in your browser before proceeding. You are using an out of date browser. It may not display this or other websites correctly. You should upgrade or use an alternative browser. ... hi all, how would i increment the timestamp by one day. i currently ...
Javascript answers related to "javascript subtract 1 month from date". 3 letter months javascript array. add month date now javascript. add one month to date javascript. get day first 3 letters name in js. Get day first 3 letters name js. get month in two digit in javascript date. 25/3/2020 · Increment Date by 1 Day in JavaScript. // Current date is 25th March 2020 var currDate = new Date(); console.log('Today the date is '+ currDate); // now increment it by 1 day currDate.setDate(currDate.getDate() + 1); console.log('Tomorrow the date is '+ currDate); Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor.
We would like to know how to add days to current date · The code above is rendered as follows: Date.getDate() returns the day index for the current date object. In your example, the day is 30. The final expression is 31, therefore it's returning 31 milliseconds after December 31st, 1969. A simple solution using your existing approach is to use Date.getTime() instead. Then, add a days worth of milliseconds instead of 1. For example, TIMESTAMPADD () function. MySQL TIMESTAMPADD () adds time value with a date or datetime value. The unit for the interval as mentioned should be one of the following : FRAC_SECOND (microseconds), SECOND, MINUTE, HOUR, DAY, WEEK, MONTH, QUARTER or YEAR.
Dec 02, 2020 - A quick article to learn how to add days to the JavaScript Date object. Use the Math.abs() Function to Subtract Datetime in JavaScript. This procedure is similar to the first one except that it returns the absolute value. You will need to define the two dates then subtract the two variables using the Math.abs() function as follows: const date = new Date (Date.now () + (3600 * 1000 * 24)) console.log (date) to get the current date and time in milliseconds with Date.now. Then we add 3600 * 1000 * 24 milliseconds, which is the same as 1 day to it. And we pass that to the Date constructor to get the date 1 day after the current date.
The timezone offset can vary, and getTimezoneOffset gets the offset for the date instance. For example, I am in US Eastern Timezone, and new Date().getTimezoneOffset() is 300 for today's date, but new Date("6/1/2016") is 240 because of change of daylight savings. Your code may give unexpected results if the date represented by the timestamp has a different timezone offset from the current date.
Customized Scheduling For Integrations In Sap Cloud
Lightweight Human Readable Date Amp Time Library Timeago Js
Serverless Analytics Benchmark Of Aws Aurora Performance
Moment Js Parse Date Time Ago Stack Overflow
Get The Day Of The Week Name And Number In Power Bi Using Dax
A Complete Guide To Javascript Dates And Why Your Date Is
How To Group Daily Summary Data By Date Data Queries
Jquery Relative Date Plugins Jquery Script
Python Datetime Timedelta Strftime Format With Examples
Top 5 Best Open Source Javascript Time Ago Libraries Our
Get Day Of Month From Date Javascript Code Example
How To Get The Current Timestamp In Javascript
Timestamp Subtract 1 Day Js Code Example
How Do I Convert A Firestore Date Timestamp To A Js Date
Javascript Convert A Unix Timestamp To Time W3resource
The Definitive Guide To Javascript Dates
Javascript Extract Date From String Code Example
Demystifying Datetime Manipulation In Javascript Toptal
Working With Dates And Timezones In Javascript A Survival Guide
Adjusting Dates By Adding Date Time Parts In Angular 11 0 0
How To Find Unique Dates In An Array In Javascript By Dr
Add Days Or Minutes Or Hours To Javascript Date Or Subtract
Working With Timestamp With Time Zone In Your Amazon S3 Based
A Human Friendly Way To Display Dates In Typescript
Absolute Vs Relative Timestamps When To Use Which
How To Manipulate Date And Time In Javascript
Front End Developer Handbook 2019 Learn The Entire
0 Response to "30 Timestamp 1 Day Javascript"
Post a Comment