20 Biggest Number In Array Javascript
Return an array consisting of the largest number from each provided sub-array. For simplicity, the provided array will contain exactly 4 sub-arrays. I am getting output [27,27,39,1001] but the output should be [27,5,39,1001]. function largestOfFour(arr) { // You can do this! Jul 24, 2021 - The Math.max() function returns the largest of the zero or more numbers given as input parameters, or NaN if any parameter isn't a number and can't be converted into one.
Find The Smallest And Biggest Numbers In An Array Help
Jul 01, 2019 - In this article, I’m going to explain how to solve Free Code Camp’s “Return Largest Numbers in Arrays” challenge. This involves returning an array with the largest numbers from each of the sub arrays. There are the three approaches I’ll cover: with a FOR loopusing the reduce(
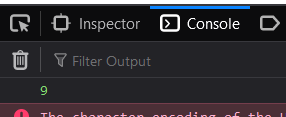
Biggest number in array javascript. Aug 16, 2017 - Or with the new spread operator, getting the maximum of an array becomes a lot easier. ... According to MDN the apply and spread solutions had a limitation of 65536 that came from the limit of the maximum number of arguments: But beware: in using apply this way, you run the risk of exceeding the JavaScript ... Apr 30, 2019 - This article is a part of the series freeCodeCamp Algorithm Scripting. Jul 02, 2018 - Tell us what’s happening: Can return ‘ar’ and ‘len’ right but returns nothing for ‘arg’. The syntax seems right and it’s no runtime error. I don’t understand why ‘len’ isn’t ‘pushed’ to ‘arg’. Your code so far functi…
We are going to write a Javascript program to find out:The Second Largest Number/Element in an unsorted Array. Javascript Web Development Front End Technology We are required to write a JavaScript function that takes in an array of numbers as the first and the only argument. The function should string together the numbers present in the array such that form the greatest possible number that can be formed from those given set of numbers. Aug 30, 2017 - Recently, I published an article on: How I built an Interactive 30-Day Bitcoin Price Graph with React and an API. One of the key ingredients you need to build a graph is a minimum and maximum value…
The Math.max() function returns the largest of zero or more numbers, and we can pass any number of arguments. console.log(Math.max(4,5,1,3)); // logs 5 But you can't pass an array of numbers to ... Nov 19, 2020 - The Fastest Way to Find Minimum and Maximum Values in an Array in JavaScript. JavaScript offers several ways to find the smallest and largest numbers in a list, including the built-in Math functions and sorting the…. Here, getLargest JavaScript function is used to calculate the largest of five numbers i.e. the numbers we are taking as parameters. Inside this function, we have five if conditions. Each condition compares each of these numbers with the other numbers and returns that number if it is largest of them all.
See the Pen javascript-math-exercise-6 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to format a number up to specified decimal places. Next: Write a JavaScript function to find the lowest value in an array. In this tutorial, you'll learn how to find the largest number in an array with JavaScript which is a common coding exercise asked at Junior Developer intervi... 12/3/2021 · You can use for loop or reduce () method to get the largest number in array JavaScript. But the simplest way is Math.max () function. 3 Way to find largest number in array JS:
This JavaScript code will read three numbers in a text box and it will return the largest number from given/input three numbers. Submitted by Aleesha Ali, on March 25, 2018 Given three numbers and we have to find its largest number using JavaScript. JavaScript code to find largest of three numbers Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation. There are multiple methods to find the smallest and largest numbers in a JavaScript array, and the performance of these methods varies based on the number of elements in the array. Let's discuss each of them separately and give the testing results in the end.
If we want the largest one number in an array, we can get that using JavaScript's Math.max () function. Simply place the array of values (without square brackets) into the parenthesis of the function. Output: 98 93 91. Optimized Solution: We first sort the array in increasing order and then we run the loop for the length equal to n and print the first n largest elements. Implementation: Hello everyone!!,This is my first post on medium feeling good! lets get into topic, find the second largest number in a array, let's write a function to find "Second largest number" from a array. In the main function we will use Math.max() and we will also use Array.splice() and Array.indexof() methods
9/9/2018 · Then from the nested array [2, 3, 603], add 603 at indices from 2 to 3. So the zero array becomes [0,603,603,0]. Then the second nested array is used. This is done till all the nested arrays are used. After that's done, I need to find the biggest number in the zeroArray (its value now is … We have to write a simple function in JavaScript that takes in an array of Numbers (nested to any level) and return the greatest number present in the array. For example: If the input array is −. const arr = [ 34, 65, 67, [ 43, 76, 87, 23, 56, 7, [ 54, 7, 87, 23, 79, 994, 2 ], 54 ], 54, 4, 2 ]; Then the output should be −. Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation.
Javascript Web Development Front End Technology Object Oriented Programming We are required to write a JavaScript function that takes in an array that contains some numbers, some strings and some falsy values. Our function should return the biggest Number from the array. Return an array consisting of the largest number from each provided sub-array. For simplicity, the provided array will contain exactly 4 sub-arrays. Remember, you can iterate through an array with a simple for loop, and access each member with array syntax arr [i]. I am trying math.max() but that doesn't seem to be working. When I console.log() the result of it I get only the first subarray. I tried sort(), imagining that I could select the largest number through bracket notation, but sort() just copied arr as well as the sorted arr into a larger array.
3/2/2018 · How to find the largest number contained in a JavaScript array? Javascript Web Development Front End Technology To get the largest number in the array, you can try to run the following code. It returns the largest number 530 − First, I would change the category from general to javascript. Here is my pseudocode. Define a variable and store a number. Run a forloop over your array and compare stored number with each value in the array. If the current value is bigger than the stored value, replace the stored value with current value. Repeat. You can use the spread operator to pass each array element as an argument to Math.min () or Math.max (). Using this method, you can find the smallest and largest number in an array:
Jul 01, 2019 - In this article, I’m going to explain how to solve Free Code Camp’s “Return Largest Numbers in Arrays” challenge. This involves returning an array with the largest numbers from each of the sub arrays. There are the three approaches I’ll cover: with a FOR loopusing the reduce( That takes an array as a parameter and returns the biggest number. Here is an array const array = [-1, 0, 3, 100, 99, 2, 99] What I try in my JavaScript code: Feb 01, 2018 - JavaScript Arrays — Finding The Minimum, Maximum, Sum, & Average Values · Plug and play options for working with Arrays of Numbers ... In this article we’ll explore four plug and play functions that allow you to easily find certain values in an arrays of numbers.
Sep 04, 2018 - https://learn.freecodecamp /javascript-algorithms-and-data-structures/basic-algorithm-scripting/return-largest-numbers-in-arrays/ I have done this challenge and came up with a way that it does return you a new array consists of all the biggest numbers from the sub-arrays. We are going to write a function called largestOfFour that will accept an array (arr) as an argument. We are given an array that contains 4 sub-arrays. The goal of the function is to output an array that contains the largest numbers from each of the sub-arrays. Here is an example: We want #2! Simplest Case: a sorted array. If you know the array is already sorted, getting the second largest number is simple: const arr = [1, 2, 3]; function findSecondLargest(arr) {return arr[arr.length - 2];} This will r eturn the element that is at the position of the length of the array, minus 2. That's 2, the second largest number.. Slightly more complex: unsorted array
Enter first number: -7 Enter second number: -5 Enter third number: -1 The largest number is -1. In the above program, parseFloat() is used to convert numeric string to number. If the string is a floating number, parseFloat() converts the string into floating point number. 9/7/2021 · There are two ways you can find the largest number from a JavaScript array: Using the forEach () array method Using the reduce () array method This tutorial will help you learn how to do both. Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript function to find the highest value in an array.
We can find the largest number in an array in java by sorting the array and returning the largest number. Let's see the full example to find the largest number in java array. public class LargestInArrayExample {. public static int getLargest (int[] a, int total) {. int temp; const arr = [ 15, 24, [ 29, 85, 56, [ 36, 14, 6, 98, 34, 52 ], 22 ], 87, 60 ]; and return the greatest number present in the array. In this video series, Stephen Mayeux (stephenmayeux ) walks through the algorithmic challenges at Free Code Camp.**Return Largest Numbers in an Array** --...
Simple trick on how to find highest element in an array. We`re gonna use FOR loop an IF statement insie it Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Many programming languages support arrays with named indexes. Arrays with named indexes are called associative arrays (or hashes). JavaScript does not support arrays with named indexes. In JavaScript, arrays always use numbered indexes.
largest = array.sort((a,b)=>a-b)[array.length - 1]; UPD, all code above is sucks when you add for example 9 in array my guess because by default numbers treated as strings in sort, there is better version. var array = [3 , 6, 2, 56, 32, 5, 89, 32, 9], largest; array.sort(function(a, b) { largest = a > b ? a: b; });
Find The Largest Number In Array Javascript Example Code
Find Largest And Second Largest Number In C
C Program To Find Largest And Second Largest Number In An
Java Program To Find The Largest Number In An Array Edureka
Third Maximum Number Leetcode Solution Tutorialcup
How To Find Largest And Smallest Number In Unsorted Array
C Program To Find Maximum Element In Array
How To Get The Highest And Lowest Number From An Array In
Find The Second Largest Number In Array Javascript Example Code
Javascript Basic Get The Largest Even Number From An Array
Find The Smallest And Biggest Numbers In An Array Help
Find The Smallest And Biggest Numbers In An Array Help
Find The Second Largest Number In An Array By Javascript
Java Program To Find Largest And Smallest Number In An Array
Find Smallest And Largest Number In Unsorted Array O N
How To Find The Nth Largest In Excel Small And Large
Javarevisited How To Find Largest And Smallest Of N Numbers
Find The Largest Number In An Array Javascript Tutorial
0 Response to "20 Biggest Number In Array Javascript"
Post a Comment