30 Javascript Add To String
Use sprintf () to Insert Variable Into String in JavaScript Before Template Literals in ES6 of Javascript, the development community followed the feature-rich library of sprintf.js. The library has a method called sprintf (). sprintf.js is an open-source library for JavaScript. It has its implementations for Node.js and browsers. JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. In contexts where a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup.
How To Insert A String At A Specific Index In Javascript
In JavaScript, the plus + operator is used for numeric addition and string concatenation. To adds the number to a string Just use plus between the number and string in Javascript. String + Number = Concatenated string. Number + Number = The sum of both numbers. String - Number = The difference between (coerced string) and the number.
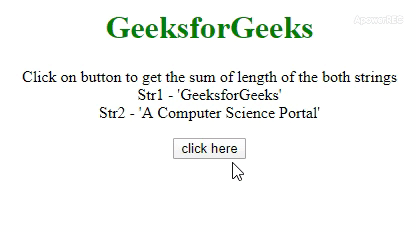
Javascript add to string. Strings Can be Objects. Normally, JavaScript strings are primitive values, created from literals: let firstName = "John"; But strings can also be defined as objects with the keyword new: let firstName = new String ("John"); Example. let x = "John"; let y = new String ("John"); // typeof x will return string. I need to add in a For Loop characters to an empty string. I know that you can use the function concat in Javascript to do concats with strings var first_name = "peter"; var last_name = "jones";... String#concat () JavaScript strings have a built-in concat () method. The concat () function takes one or more parameters, and returns the modified string. Strings in JavaScript are immutable, so concat () doesn't modify the string in place.
The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. In this article, I'll explain how to solve freeCodeCamp's "Repeat a string repeat a string" challenge. This involves repeating a string a certain number of times. There are the three approaches I'll cover: using a while loopusing recursionusing ES6 repeat() methodThe Algorithm Challenge DescriptionRepeat a given string In javascript, we can add a number and a number but if we try to add a number and a string then, as addition is not possible, ' concatenation' takes place. In the following example, variables a,b,c and d are taken. For variable 'a', two numbers (5, 5) are added therefore it returned a number (10).
The padStart () method pads the current string with another string (multiple times, if needed) until the resulting string reaches the given length. The padding is applied from the start of the current string. COMBINED = STRING-A + STRING-B: Concatenate 2 strings together. Click Here: COMBINED = `TEXT ${EXPRESSION} TEXT` Template literals, literally evaluate the expression and add it into the string. Click Here: STRING.repeat(N) Repeat the given string N times. Click Here: STRING.concat(STRING, STRING, ...) Combines multiple strings together. Click Here Let's check out the different ways of converting a value to a string in JavaScript. The preferred way from Airbnb's style guide is…
11 Years Ago. That will remove any preceding or trailing white space from the string. Also, you may want to phrase your initial question better :P You asked for a script to add a space 'after every 4 digits' which is what you got.. \w will match a-z, A-Z, 0-9 and underscores, so yes, replacing the d with a w should work if you want a space ... Just appending one string to another For just two strings, you can append as follows: Basically, you can think of it as concatenating str2 to str1. The left-hand side is getting the right-hand side added to it. In JavaScript, strings are objects used to represent and manipulate strings. [removed] There are many ways to add strings in the +operatorconcat method, and you can connect form strings JavaScript add strings with + operator The easiest way to concatenate strings is to use the + or + = operator.
The concat () method joins two or more strings. concat () does not change the existing strings, but returns a new string. P adding a string in JavaScript refers to filling a string up with a repeated character or string of characters, usually whitespace. The string is padded to a certain length, which is what is... In JavaScript, there are three ways you can create multi-line strings: Using new line characters (\n). Using string concatenation. Using template string literals. In this guide, we're going to talk about how to use each of these three approaches to write a multi-line string. We'll walk through examples of each approach so you can easily get ...
In JavaScript, Strings are values made up of text and can contain letters, numbers, symbols, punctuation, and even emojis! Single and Double Quotes in JavaScript Strings Strings in JavaScript are contained within a pair of either single quotation marks '' or double quotation marks "". Both quotes represent Strings but be sure to choose one and ... 17/2/2021 · JavaScript add strings with concat The concat method concatenates the string arguments to the calling string and returns a new string. Because the concat method is less efficient than the + operator, it is recommended to use the latter instead. Using the insertAdjacentHTML () method Using the innerHTML attribute: To append using the innerHTML attribute, first select the element (div) where you want to append the code. Then, add the code enclosed as strings using the += operator on innerHTML.
In javascript, we have a built-in method which is common for all the objects toString () to convert the object to the string datatype. This toString () method of an object is overridden by Array to convert the array object to string data type. While using the toString () method the array object remains intact and unchanged and a new object with ... In JavaScript and many other programming languages, the newline character is \n. To add a new line to a string, all you need to do is add the \n character wherever you want a line break. Concat String: 8. String escape: 9. Escape double quote: 10. Including a special character in a string: 11. String font Color: 12. String font Size() 13. String index Of: 14. Combine indexOf and substring methods to get the anchor part of a url: 15. Search the string and counts the number of e's: 16. String from Char Code: 17. String in italics ...
The toString() method is a built-in method of the JavaScript Number object that allows you to convert any number type value into its string type representation.. How to Use the toString Method in JavaScript. To use the toString() method, you simply need to call the method on a number value. The following example shows how to convert the number value 24 into its string representation. Description In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string. JavaScript Array join () Method: This method adds the elements of an array into a string and returns the string. The elements will be separated by a passed separator. The default separator is a comma (,).
Variables in JavaScript are named containers that store a value, using the keywords var, const or let. We can assign the value of a string to a named variable. const newString = "This is a string assigned to a variable."; Now that the newString variable contains our string, we can reference it and print it to the console. To keep long concatenation output readable, JavaScript provides two ways to create line breaks within your string. The first is the newline character ( \n). The newline character creates line breaks within the output of a string, be it simply text or JavaScript-generated HTML. Here is a long string without line breaks: var noBreaks = "Hello World. var string ="Maru"; string +="f" console.log(string); Here we declare a variable to contain a string and then we just used the + operator to add "f" to the end of the string. So we should get the output with the added character into the string. Output: Maruf. Here we are! So it's that such simple.
25/10/2018 · // use a negative index to insert relative to the end of the string. String.prototype.insert = function (index, string) { var ind = index < 0 ? this.length + index : index; return this.substring(0, ind) + string + this.substr(ind); };
Could Not Insert Script String From Ajax Response Stack
5 Way To Append Item To Array In Javascript Samanthaming Com
Add A New Method To String Class In Javascript
Javascript Tip Generating Random Code In Form Field Input
How To Insert Variable Into Javascript Action Questions
Python String Append Example Java Code Geeks 2021
Two Ways You Can Take Advantage Of Types In Javascript
Javascript Array Splice Delete Insert And Replace
How To Add Method To String Class In Javascript Geeksforgeeks
Using Enums Enumerations In Javascript
Overloading Methods With Javascript Control Add Ins Vjeko Com
Javascript Add Delete Array Flip Array Array Sorting String
Table Of Javascript String And Array Methods By Charlie
Concatenate Char To String In Java
Display Updating Javascript Triggered In Format String Of
String Interpolation In Javascript
4 Ways To Convert String To Character Array In Javascript
How To Append Element To Array In Javascript Tecadmin
How To Replace Number With String In Javascript
Javascript Concatenate Strings Es6 String Concat Method
When You Add A String To An Integer And Wait For An Error But
Javascript Connector Workato Docs
Why Javascript Innerhtml Add Quote Automatically To My String
Javascript Add String To Middle Of String Code Example
String Literals In Javascript Should I Use Double Quotes Or
Strings In Powershell Replace Compare Concatenate Split
0 Response to "30 Javascript Add To String"
Post a Comment