33 Append Class In Javascript
JavaScript classes are one of the game-changing features that came with the ES6 version of the language. A class can be described as a blueprint that is used to create objects. In this tutorial article, you'll learn how to create and manipulate objects using JavaScript classes. JavaScript Class Structure Aug 26, 2009 - Receives the index position of the element in the set and the existing class name(s) as arguments. Within the function, this refers to the current element in the set. It's important to note that this method does not replace a class. It simply adds the class, appending it to any which may ...
Javascript To Check That Two Fields Are Equal Laserfiche
By using JavaScript classList property’s Add method, you may add the CSS class to different elements. In the following example, a raw table without any CSS is created as the web page loads. Again, as you click the button, the CSS style class will be added to the table by using JavaScript: See online demo and code
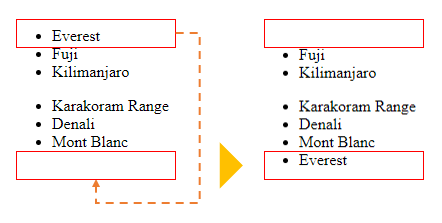
Append class in javascript. Feb 14, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Luckily enough, the JQuery library has a method called addClass, which can be used to add CSS classes to an element. Let's modify the example above to use JQuery instead of vanilla JavaScript: setTimeout (function () { //Add the CSS class using the JQuery addClass method. $ ('#intro').addClass ('newClass'); }, 5000); The code snippet above ... 1 week ago - If the given child is a reference to an existing node in the document, appendChild() moves it from its current position to the new position (there is no requirement to remove the node from its parent node before appending it to some other node). This means that a node can't be in two points ...
Sep 29, 2020 - To add a piece of text to a <div>, you can use the innerHTML property as the above example, or create a new Text node and append it to the div: // create a new div and set its attributes let div = document.createElement('div'); div.id = 'content'; div.className = 'note'; // create a new text ... How to add/remove class to/from an element in JavaScript. A quick tutorial on how to add, remove and toggle classes in JavaScript. In JavaScript, the standard way of selecting an element is to use the document.getElementById("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows:
Add Class Attribute To The Image In JavaScript. Unlike ID attribute, you can add multiple class names in a single image element or the same class name in multiple image elements or combinations of ... Element.classList. The Element.classList is a read-only property that returns a live DOMTokenList collection of the class attributes of the element. This can then be used to manipulate the class list. Using classList is a convenient alternative to accessing an element's list of classes as a space-delimited string via element.className. The example of using the .className property is given as follows.. Example - Adding the class name. In this example, we are using the .className property for adding the "para" class to the paragraph element having id "p1".We are applying the CSS to the corresponding paragraph using the class name "para".. We have to click the given HTML button "Add Class" to see the effect.
classList property in javascript. classList property is used to return the class attribute present in a particular element. In Javascript using classList property, class attributes and there values of an element can be accessed. Unlike className, this property has more methods. Syntax. Adding a class to an element ought to move the class name to the sharp end of the list, if it exists already. document.addClass= function(el, css){ var tem, C= el.className.split(/\s+/), A=[]; while(C.length){ tem= C.shift(); if(tem && tem!= css) A[A.length]= tem; } A[A.length]= css; return el.className= A.join(' '); } 2/12/2019 · How to add a class to DOM element in JavaScript? Javascript Front End Technology Object Oriented Programming. To add a class to a DOM element, you first need to find it using a querySelector like querySelector, getElementById, etc. Then you need to add the class. For example, if …
Javascript append single item. Please, take into account that push () changes your original array. For creating a new array, you should implement the concat () method, like this: const animals = [ 'dog', 'cat', 'mouse' ]; const allAnimals = animals.concat ( 'rabbit' ); console .log (allAnimals); Also notice that the concat () method doesn't ... Javascript queries related to “append class name to existing class jquery” · add javascript class to an element with a class jquery ... Apply a class to <a> element. Apply class to an a element. Apply class to an element. Apply class element. screen change find same name class and addclass in ... CSS class names can be removed or added using the classList method, or they can modified straight with the className property. Using the classList method. classList is pretty smart and the most modern system of manipulating CSS class names in elements via JavaScript. Remove class names. Here's how to remove a single class name:
Feb 15, 2021 - From there you can easily derive ... just append a space followed by the new class to the element's className property. Knowing this, you can also write a function to remove a class later should the need arise. ... I think it's better to use pure JavaScript, which we can ... Jul 24, 2019 - Showing results for div id javascript id selector combine with class Search instead for div id javascript id selector cobine with class How to combine class and ID in JQerry selector The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass";
Aug 28, 2018 - The leading space is important, because the className property treats the css classes like a single string, which ought to match the class attribute on HTML elements (where multiple classes must be separated by spaces). Incidentally, you're going to be better off using a Javascript library like ... JavaScript JavaScript Reference ... jQuery Get jQuery Set jQuery Add jQuery Remove jQuery CSS Classes jQuery css() jQuery Dimensions ... The append() method inserts specified content at the end of the selected elements. Tip: To insert content at the beginning of the selected elements, use the prepend() method. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Dec 01, 2019 - How to add class to element in JavaScript, Element.classList.add modern JavaScript answer on Code to go 18/9/2019 · In this video, you will learn how to add and remove class onclick in javascript. Also, you will learn how to check if class exists, toggle class and add or ... Code language: JavaScript (javascript) The append () method will insert DOMString objects as Text nodes. Note that a DOMString is a UTF-16 string that maps directly to a string. The append () method has no return value.
It's important to note that this method does not replace a class. It simply adds the class, appending it to any which may already be assigned to the elements. Before jQuery version 1.12/2.2, the .addClass() method manipulated the className property of the selected elements, not the class attribute. Once the property was changed, it was the ... TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. If you know javascript, you are more than half way there. TypeScript consists of a few parts. The first is the TypeScript language — this is a new language which contains all JavaScript features . Check out the specs for more information. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
Since .append() can accept any number of additional arguments, the same result can be achieved by passing in the three <div>s as three separate arguments, like so: $('body').append( $newdiv1, newdiv2, existingdiv1 ). The type and number of arguments will largely depend on how you ... In modern JavaScript, it makes no sense to load the complete jQuery library just to do some simple DOM manipulations. In this article, you'll learn how to add, remove, and toggle CSS classes in vanilla JavaScript without jQuery. Using className Property. The simplest way to get as well as set CSS classes in JavaScript is by using the className ... Here is syntax for JavaScript Append method is as follows: document. getElementById ("div_name").innerText += "data" ; We can use jQuery too, for appending content at the end of the selected elements, by using following syntax : $ (selector).append (content , function (index.html)) Into the above syntax content is the required parameter ...
Summary: in this tutorial, you will learn how to use the JavaScript appendChild() method to add a node to the end of the list of child nodes of a specified parent node. Introduction to the JavaScript appendChild() method. The appendChild() is a method of the Node interface. How to get current formatted date dd/mm/yyyy in Javascript and append it to an input? 28, May 19. Implement prepend and append with regular JavaScript. 24, Jun 19. How to append some text to all paragraphs using jQuery ? ... How to append class if condition is true in Haml? 15, May 20. How to append a string in PHP ? 02, Jun 20. Article ... Javascript Web Development Object Oriented Programming. To add an active class to the current element with JavaScript, the code is as follows −.
Learn how to add a class name to an element with JavaScript. Add Class. Click the button to add a class to me! Add Class. Step 1) Add HTML: Add a class name to the div element with id="myDIV" (in this example we use a button to add the class). Example. <button onclick="myFunction()">Try it</button>. <div id="myDIV">. const element = document.querySelector("#box"); element.classList.add("active", "highlighted"); Sep 22, 2018. 1. Jad Joubran. See answer. 1. Jad Joubran. Element.classList on MDN. Oct 10, 2020 - Found the internet! ... I'm sooo sorry if this is a massively newbie question, but I'm not quite sure how to search for this. Everything I find shows me how to append 1 class to an element. Essentially what I'm trying to do is convert this HTML to Javascript DOM:
jQuery's append() method can add a DOM element or a HTML string into a given DOM element, and makes things quite easy. But the same can be done with vanilla Javascript also. Appending an Element with appendChild. The appendChild method requires 2 DOM elements :. The DOM element into which appending is done. The DOM element which is to be appended Jun 02, 2017 - This solved the issue with the class names, but it didn’t solve the issue of the<div class="nav__expand">....</div> issues. ... I’m not great at Javascript but I’ve become more and more comfortable when dabbling with jQuery over the years. First I had to target the instances where the ... Class methods are non-enumerable. A class definition sets enumerable flag to false for all methods in the "prototype". That's good, because if we for..in over an object, we usually don't want its class methods. Classes always use strict. All code inside the class construct is automatically in strict mode.
In the example above, the addNewClass() function adds a new class highlight to the DIV element that already has a class box without removing or replacing it using the className property.
Append Remove Class For Li Vps And Vpn
How To Append Html Code To A Div Using Javascript
Setting Css Styles With Javascript Soshace Soshace
How To Append Child Of Child In Javascript Stack Overflow
Class Amp Amp Interface Angular
Cardio Beginner Javascript Wes Bos
Javascript Add An Html Class To Div If Statement Bootstrap
Only Javascript Please For Every Front End Developer By
Javascript Adding A Class Name To The Element Geeksforgeeks
How To Append Html Css And Javascript To Your Whitelabel
Mixing Replace And Append Javascript Basics
Javascript Jquery How To Append The State City Fields
Add A Map To Your Website Javascript
How To Add If Or Else Condition In Javascript Content Stack
How To Add A Class To An Element Using Javascript Javatpoint
Setting Css Styles With Javascript Soshace Soshace
Javascript Append How Does Javascript Append Work In Html
How To Add Live Support Chat To Your Web Application With
Javascript Project How To Create A To Do List Using
How To Append Data To Lt Div Gt Element Using Javascript
Javascript Appendchild By Practical Examples
How To Add Remove And Toggle Class By Javascript And Jquery
Object Oriented Javascript For Beginners Learn Web
Javascript Append String Dynamically To Script Element
Javascript Append How Does Javascript Append Work In Html
Add Paragraph Javascript Code Example
How To Manipulate Classes Without Jquery By Using Html5 S
Call A Function With Parameters In Javascript Append Stack
Adding Or Removing Css Class In Lightning Web Component
4 Ways To Convert String To Character Array In Javascript
Jquery Addclass With Html Table Button Links And List Demos
0 Response to "33 Append Class In Javascript"
Post a Comment