31 Transform Object In Array Javascript
Introduction to JavaScript Array map () method Sometimes, you need to take an array, transform its elements, and include the results in a new array. Typically, you use a for loop to iterate over the elements, transform each individual one, and push the results into a new array. Let's take a look at an example. Let's say we have the following object of objects that contains rating of some Indian players, we need to convert this into an array of objects with each object having two properties namely name and rating where name holds the player name and rating holds the rating object −. Following is our sample object −. const playerRating = { 'V ...
Convert Javascript Array To Object Of Same Keys Values
Just want to point out - if you already have an array of sorted properties from the original object, using the spread operator is what will turn that array directly into a new object: { ...[sortedArray]} - HappyHands31 Jul 26 '19 at 15:18
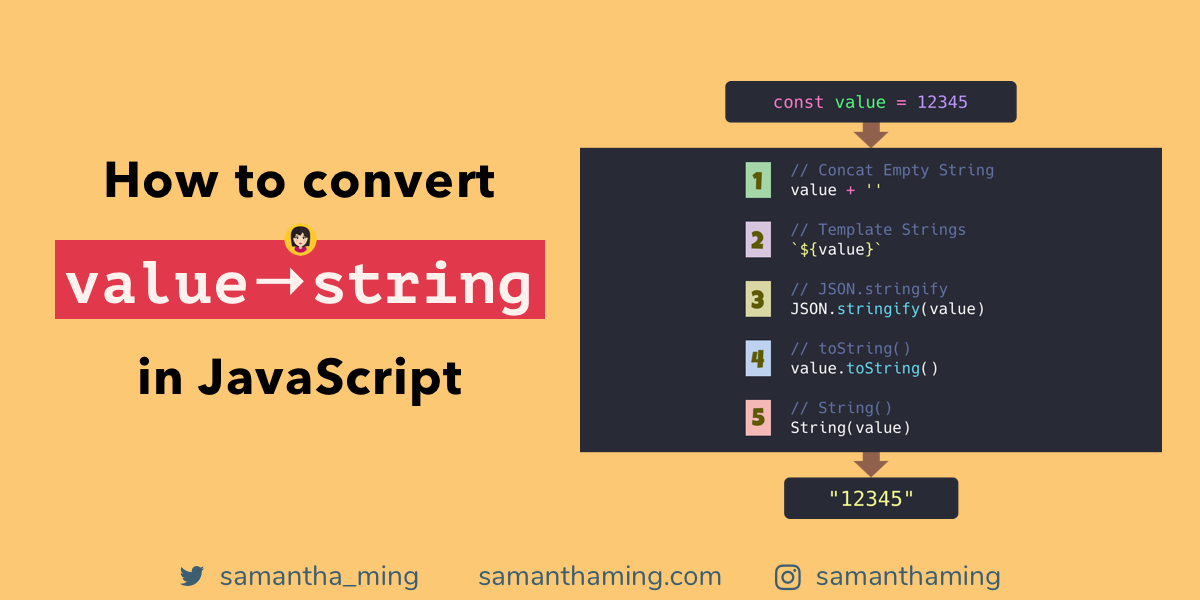
Transform object in array javascript. Both Object.assign () and object spread operator methods are the latest addition to JavaScript and only works in modern browsers. For more browsers support, you should simply use the for loop to iterate over the array elements, and add them to an object: 26/8/2021 · how to convert array to object in javascript Use loop statements const newArray = []; let counter = 1; for (let name of authors) { newArray.push({ id: counter, name, }); counter += 1; } console.log(newArray); No loop statement. Avoid loop statements by using the map methods of the array How to convert Set to Array in JavaScript? Last Updated : 21 Jul, 2021. A set can be converted to an array in JavaScript by the following way-. By using Array.from () method: This method returns a new Array from an array like object or iterable objects like Map, Set, etc. Syntax. Array.from (arrayLike object); Example-1.
Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... The task is to convert an Object {} to an Array [] of key-value pairs using JavaScript. Introduction: Objects, in JavaScript, is it's most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript's primitive data-types(Number, String, Boolean, null, undefined and symbol). 9/11/2020 · How can we transform our our services property object to an array? Transforming with plain JavaScript permalink. One obvious way is to do it in plain JavaScript. Like this. const transform = obj => Object.keys(obj).map(id => ({ id, ...obj[id] })); console.log(trasform(obj.services));
To access key=7, we need to traverse through each element of this array and then compare 7 with the key property of every element. So, this approach is not feasible at all. So, the solution to this problem is something you can convert this array of objects into a single object using javascript functions like reduce. I've just generated array with unique names and empty series array at first (result), this one is pushing all the series objects data into these empty series arrays in result array. It's just going through original array (arr) to pick series object and push it in result series array - names properties has to match hence y.name===x.name 24/8/2018 · Transform array of objects to a different array of objects - Javascript/Angular. I have an array of object that looks like this. I made it look that way using .groupBy with lodash. I need it to look like this: stateList:StateDropdownItem [] = [ { label: 'USA', items: [ {label: 'AL', value: 'Alabama'}, {label: 'AK', value: 'Alaska'}, ] }, . .
I want to create an array that holds the values of the object. The keys (key names) can be disregarded: [24, 23, 33, 12, 31] The order of the values is NOT important! One solution (obviously) would be do have a function that takes the values and puts them into an array: var arr = valuesToArray(obj); I will accept such a function as the answer. to convert an Object {} to an Array [] of key-value pairs in JavaScript If you are using lodash, it could be as simple as this: var arr = _.values (obj); How to convert an Object {} to an Array [] of key-value pairs in JavaScript 3/1/2020 · To convert property’s values of the person object to an array, you use the Object.values() method: const propertyValues = Object .values(person); console .log(propertyValues); Code language: JavaScript ( javascript )
Arrays of objects don't stay the same all the time. We almost always need to manipulate them. So let's take a look at how we can add objects to an already existing array. Add a new object at the start - Array.unshift. To add an object at the first position, use Array.unshift. JavaScript Object.assign() method: Here, we are going to learn how to convert arrays to objects in JavaScript using the Object.assign() method? Submitted by Siddhant Verma , on December 14, 2019 Let's say you have the following array, Given an array of objects and the task is to convert the object values to an array with the help of JavaScript. There are two approaches that are discussed below: Approach 1: We can use the map () method and return the values of each object which makes the array. Approach 2: The Object.keys () method is used to get the keys of object and then ...
We will use the Object methods i.e. Object.keys (), Object.values () and Object.entries () for convert an object into an array in JavaScript. For understanding this concept, it is very important that you have a clear understanding of an Object and Array in Javascript. It may be the chance that you are working on plain and vanilla Javascript. Convert object to array javascript - Một câu hỏi tuy đơn giản nhưng đây là một câu hỏi với số lượng search trên google tại Việt Nam lên đến gần 300 lượt mỗi tháng. Điều đó có nghĩa là nhu cầu sử dụng array trong javascript rất nhiều. Vì thế, tipjs sẽ giới thiệu một số cách chuyển từ object sang array javascript. To convert JavaScript object to array, we can use two functions - Object.keys () and Object.values (). Suppose, our javascript object is -. var jsObj = { key1 : 'value 1', key2 : 'value 2', }; Now, if you want to for loop through this object, you will need to first convert it into array. Because looping through array is possible using count ...
Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. Transform data from a nested array to an object in JavaScript Javascript Web Development Front End Technology Object Oriented Programming Suppose, we have the following array of arrays − The Object.fromEntries() method takes a list of key-value pairs and returns a new object whose properties are given by those entries. The iterable argument is expected to be an object that implements an @@iterator method, that returns an iterator object, that produces a two element array-like object, whose first element is a value that will be used as a property key, and whose second element ...
How to Convert JavaScript Objects to Arrays. The simplest way to convert an Object to an Array is to use Object.entries (). Object.entries () returns an array of arrays containing the object's key/value pairs. That's a bit of a mouthful and may be easier expressed in code, 21/11/2020 · Transforming array to object JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose we have an array of strings like this −. const arr = [ 'type=A', 'day=45' ]; We are required to write a JavaScript function that takes in one such array. The function should construct an object based on this array. Convert Object to Array in JavaScript using Object.entries () Method To convert the enumerable string-keyed properties of an object to an array, you use the Object.entries () method.
To convert an object to an array in JavaScript, you can use one of the following three methods: Object.keys (), Object.values (), and Object.entries (). The Object.keys () method was introduced in ES6 or ECMAScript 2015. # Converting Object to an Array. Finally, with ES2017, it's official now! We have 3 variations to convert an Object to an Array 🎊. Array has an array of methods (sorry, bad pun 😝). So by converting the object into an array, you have access to all of that. Woohoo 🥳 However, one key difference between Arrays and Array-like Objects is that Array-like objects inherit from Object.prototype instead of Array.prototype. This means that Array-like Objects can't access common Array prototype methods like forEach() , push() , map() , filter() , and slice() :
To convert an object into an array in Javascript, you can use different types of methods. Some of the methods are Object.keys (), Object.values (), and Object.entries (). Consider the below examples to understand the above methods. Method 1: Object.keys () How to convert Object to an Array in JavaScript. javascript1min read. In this tutorial, we are going to learn about three different ways to convert an object into an array in JavaScript. Using the Object.keys() method. The Object.keys() takes the object as an argument and returns the Object properties in an array. Convert an Array to an Object. Previously, converting from an object to an array was the only direction with built-in methods for the Object class. However as of ES2019/ES10, the .fromEntries() method was introduced, which converts an array of two-item arrays to an object—effectively the reverse of the .entries() method.. To see this in action, let's start with a simple example.
Javascript Array Of Objects Tutorial How To Create Update
How To Convert Object To Array In Php Php Fundamentals
Convert String With Commas To Array Stack Overflow
Transform Array Of Objects To A Different Array Of Objects
Convert Object To Array Javascript Js Example Codez Up
How To Convert An Object To An Array In Javascript
How To Use Map Filter And Reduce In Javascript
Converting Object To An Array In Javascript Dev Community
5 Ways To Convert Array Of Objects To Object In Javascript
Javascript Convert Array To Json Object
How To Convert An Array To An Object In Javascript
Converting Object To An Array Samanthaming Com
Transforming A Simple Guid Object To A Complex Object Within
Convert Array To Json Object Javascript Tuts Make
Javascript Sort Array Of Objects Code Example
Converting Object To An Array Samanthaming Com
How To Transform Javascript Objects The Power Of Object
Javascript Converting An Object To An Array Of Objects By
Hacks For Creating Javascript Arrays
5 Ways To Convert A Value To String In Javascript By
Typescript Convert Object To Array Because Ngfor Does Not
Solved Converting Json Object To Array Power Platform
Javascript Array To Object How To Convert An Array Into An
Converting An Array To Json Object In Javascript Qvault
Data Binding Revolutions With Object Observe Html5 Rocks
Pivot A Javascript Array Convert A Column To A Row Techbrij
How To Transform An Object To An Array Of Objects By
How To Use Gson Gt Fromjson To Convert The Specified Json
Js Array From An Array Like Object Dzone Web Dev
0 Response to "31 Transform Object In Array Javascript"
Post a Comment