21 Javascript Functional Programming Performance
23/3/2020 · On my machine it takes about 400 milliseconds to run one million iterations. Hence, the functional program is only about 10x slower than the imperative program. In conclusion, don't use the functional programming paradigm with imperative data structures like objects and arrays. It's slow and it's messy. Use functional data structures instead. Note: This is part of the "Javascript and Functional Programming" series on learning functional programming techniques in JavaScript ES6+. Checkout the previous post on function currying <Part 4>. Start from the beginning here. Let's get practical! Previously, we examined higher order functions.
Measuring The Performance Of Javascript Functions
Functional programming works really well for high performance and processors as you can run it on multiple processors simultaneously. If you have too many things like characters in game and few operation then OOP is good choice
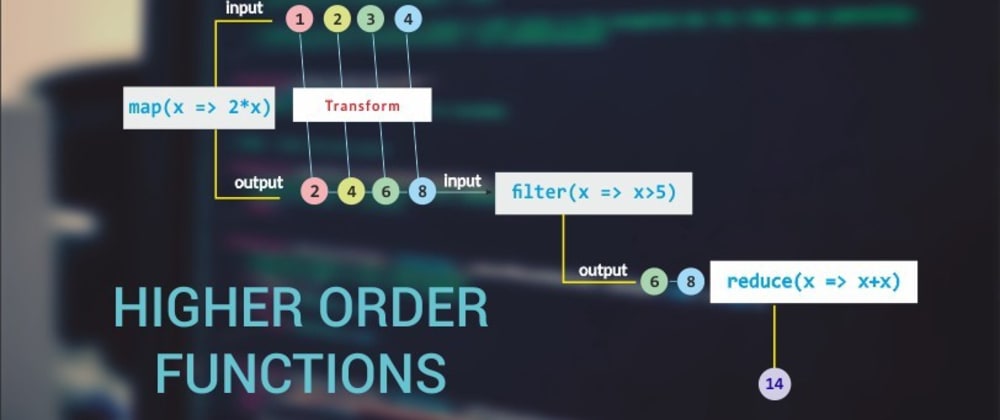
Javascript functional programming performance. 18/2/2020 · 2 Answers2. Active Oldest Votes. 6. Your benchmark is wrong because you're building a new transducer chain on each run. const inc = x => x + 1; const isEven = x => x % 2 === 0; // simplest, shortest way I would be comfortable with if performance wasn't an issue const mapFilter = xs => xs.filter (isEven).map (inc); // transducers way // ... Functional Programming was possible in Javascript just because of the first-class usage. It made the code more readable and boost the execution. Examples of functional programming boosting the execution performance are angular and react. The procedural loops can be replaced with functions that make coding, reading, and debugging more easier. Functional programming (often abbreviated FP) is the process of building software by composing pure functions, avoiding shared state, mutable data, and side-effects. Functional programming is...
Functional programming is a programming paradigm—a style of building the structure and elements of computer programs—that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. Hence in functional programming, there are two very important rules. No Data mutations: It means a data object ... Functional Programming in JavaScript teaches you techniques to improve your web applications: their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. Functional Programming and Function Chaining in JavaScript. Functional Programming is a declarative programming paradigm that has existed for a long time and the internet is full of articles trying to depict it as OOPs powerful counterpart. It only takes you to search functional vs OOP to see the raging opinion war.
In JavaScript, function composition plays an important part in implementing lazy evaluation. Lazy evaluation is a "call by need" execution strategy whereby a function is invoked only when needed. Better Code: let l = arr.length; for (let i = 0; i < l; i++) {. The bad code accesses the length property of an array each time the loop is iterated. The better code accesses the … Libraries that support functional programming lodash - A JavaScript utility library delivering consistency, modularity, performance, & extras. ramda A practical functional library for Javascript programmers. lazy.js Like Underscore, but lazier.
Avi is a full-stack developer skilled with Python, JavaScript, and Go and is also a multiple-time Google Summer of Code participant. Functional programming is a paradigm of building computer programs using expressions and functions without mutating state and data. In this article, we will talk about ... 22/8/2021 · Performance Of Javascript Foreach Map And Reduce Vs For Mastering Javascript Functional Programming In Depth Guide Where Programming Ops Ai And The Cloud Are Headed In 2021 The next function uses the "of" loop, and is FOUR TIMES FASTER than the functional version. I wasn't really expecting this level of performance boost. The last function 'aggregate_imperative_idx', uses "old" JavaScript things, no spread operator, no "of" loop, just a indexed loop. If not for the "let" and "const" keywords, it should run on ...
Functional programming is not a new approach to coding, but it has grown in popularity in recent years. This is because, once programmers understand the basics behind the technique (and are able to write clean and reliable code using it), applications written using a functional approach are much easier to work with. JavaScript is a multi-paradigm language that allows you to freely mix and match object-oriented, procedural, and functional paradigms. Recently there has been a growing trend toward functional programming. In frameworks such as Angular and React, you'll actually get a performance boost by using immutable data structures. 8/3/2018 · Common JavaScript performance problems. 1. Too many interactions with the host; 2. Too many dependencies; 3. Poor event handling; 4. Inefficient iterations; 5. Unorganized code; 20 Tips and best practices for improving JavaScript performance. 1. Use HTTP/2; 2. Use pointer references; 3. Trim your HTML; 4. Use document.getElementById() 5. Batch your DOM changes; 6. Buffer your DOM; 7.
6 best JavaScript Functional programming libraries to extend JavaScript's capabilities. So let's get started with the list. 1. Lodash. Lodash is a modern JavaScript utility library delivering modularity, performance, & extras. It makes JavaScript easier by taking the hassle out of working with arrays, numbers, objects, strings, etc. Lodash ... The importance of software performance (2) No one likes to wait for their software. Low performance software = lots of waiting. Better performing software is more popular. See e.g. Google's Chrome and their focus on performance. (Nice chapter 'High Performance Networking in Chrome' in 'The performance of Open Source Applications') Applying Functional Programming in React with Bit. Now that we know what Functional Programming is, I'll demonstrate how we use pure functions with Bit's tooling. Bit is an amazing tool to share your components in the open-source community as well as share the components you've made with your team and other teams that are looking forward to reusing existing solutions (modules on Bit's ...
Functional Programming for JavaScript People ... my real goal is to instill in you some of the core concepts and patterns behind functional programming. ... This is a huge performance loss and ... About the Book Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. You'll find plenty of JavaScript functional programming libraries on NPM. One of most notable is Ramda. It's a kind of "lodash" or "Underscore," but with FP-first in mind. Ramda gives you a few dozens of functions to process your data and compose functions.
In other words, it should not create new bugs. Therefore, let's start off with Pure Functions and further elaborate about functional programming with examples of JavaScript programs. function addition(num1,num2) {. return num1 + num2; } addition(3,4); // output is 7 regardless of the number of the number of times being called. Functional Programming in JavaScript Explained in Plain English. Joel P. Mugalu. One of the hardest things you have to do in programming is control complexity. Without careful consideration, a program's size and complexity can grow to the point where it confuses even the creator of the program. In fact, as one author put it: Functional Programming is becoming increasingly popular and relevant with each year that goes by. With so much discussion around languages such as F#, Haskel...
Exploring performance differences between classes and factories (and functional mixins). This article is part of a series starting with Examples in JavaScript Functional Programming: Part 1 . Rather than being standalone articles, they are written as a (unofficial) companion to Eric Elliot's series on functional programming. The third module, Functional Programming in JavaScript, will help you to write real-world applications by utilizing a wide range of functional techniques and styles. It explores the core concepts of functional programming common to all functional languages, with examples of their use in JavaScript. Style and approach Although Javascript doesn't have tail call optimization, recursion is often the best way to go. And sincerely, except in edge cases, you're not going to get call stack overflows. Performance is something to keep in mind, but premature optimization too. If you think that recursion is more elegant than iteration, then go for it.
JavaScript can support a wide range of programming styles simultaneously within the same codebase, so it's up to you to make the right choices for maintainability, readability, and performance.... Star 4.5k. Code Issues Pull requests. vสvr (formerly called Javaslang) is a non-commercial, non-profit object-functional library that runs with Java 8+. It aims to reduce the lines of code and increase code quality. java functional-programming java8 javaslang immutable-collections object-functional persistent-collections vavr. Updated on Jul 15. One of the great benefits of FP (Functional Programming) is how it encourages a declarative style of code rather than imperative. It has these three very common functions that are widely known as map, filter, and reduce.
Explore this second edition updated to cover features like async functions and transducers, as well as functional reactive programming ; Enhance your functional programming (FP) skills to build web and server apps using JavaScript ; Use FP to enhance the modularity, reusability, and performance of apps; Book Description. Functional programming ...
Functional Programming Vs Object Oriented Programming Dev
Functional Programming Vs Oop Top 8 Useful Differences To Know
Functional Programming In Javascript Is An Antipattern
Functional Programming Using Underscore Js Speaker Deck
Lazy Loading Javascript For High Speed Webpage Performance
Introduction To Functional Programming In Javascript
Functional Programming By Charles Scalfani Pdf Ipad Kindle
Higher Order Functions From Scratch Functional Js Dev
Transduction Functional Programming In Javascript
Javascript Performance Beyond Bundle Size Full Stack Feed
Understanding Functional Programming In Javascript A
Javascript Rest Parameter Geeksforgeeks
Functional Programming Vs Object Oriented Programming Oop
Functional Programming In Javascript Es6 Map Filter By
How Functional Programming Achieves No Runtime Exceptions
Programming Languages Trends In 2021 The Future Of Tech Codica
Two Years Of Functional Programming In Javascript Lessons
An Introduction To Functional Programming In Javascript
Functional Programming In Javascript Learn Pure Amp Impure
20 Best Practices For Improving Javascript Performance Keycdn
0 Response to "21 Javascript Functional Programming Performance"
Post a Comment