35 Javascript Add To Array
Apr 28, 2021 - Sometimes you need to append one or more new values at the end of an array. In this situation the push() method is what you need. The push() method will add one or more arguments at the end of an array in JavaScript: In the same directory where the JavaScript file is present create the file named code.json. After creating the file to make the work easier add the following code to it: { "notes": [] } In the above code, we create a key called notes which is an array. The Main JavaScript Code. In the upcoming code, we will keep adding elements to the array.
It add the element to the existing array not over ride, Ex: let arrName = ['xxx', 'yyy', 'zzz']; arrName. · If arrName has more than 3 elements you are ...20 answers · Top answer: You want the splice function on the native array object. arr.splice(index, 0, item); will ...
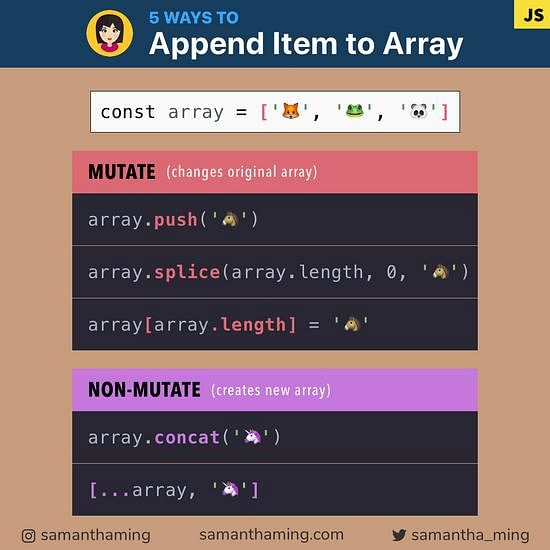
Javascript add to array. Last Updated : 06 Mar, 2019. There are several methods for adding new elements to a JavaScript array. push (): The push () method will add an element to the end of an array, while its twin function, the pop () method, will remove an element from the end of the array. If you need to add an element or multiple elements to the end of an array, the ... Method 3: unshift () The unshift () function is one more built-in array method of JavaScript. It is used to add the objects or elements in the array. Unlike the push () method, it adds the elements at the beginning of the array. " Note that you can add any number of elements in an array using the unshift () method." Method 1: push () method of Array. The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. The object is hence added to the end of the array.
Jul 17, 2021 - We can also call it without arguments: arr.slice() creates a copy of arr. That’s often used to obtain a copy for further transformations that should not affect the original array. ... The method arr.concat creates a new array that includes values from other arrays and additional items. In this article, I would like to discuss some common ways of adding an element to a JavaScript array. The Push Method. The first and probably the most common JavaScript array method you will encounter is push(). The push() method is used for adding an element to the end of an array. How to append an array to an existing JavaScript Array? How do you append an array to another array in JavaScript? Other ways that a person might word this question: Add an array to another; Concat / Concatenate arrays; Extend an array with another array; Put the contents of one array into another array
Notice that this method will not mutate your original array, but will return a new one. Describing Arrays¶ JavaScript arrays are a super-handy means of storing multiple values in a single variable. In other words, an array is a unique variable that can hold more than a value at the same time. Arrays are considered similar to objects. The main ... JavaScript gives us four methods to add or remove items from the beginning or end of arrays: pop(): Remove an item from the end of an array let cats = ['Bob', 'Willy', 'Mini']; cats.pop(); // ['Bob', 'Willy'] pop() returns the removed item. push(): Add items to the end of an array This page will walk through how to add elements in JavaScript Array. We will provide examples to add elements at start of the Array, at end of the Array, adding elements at given index and joining Arrays. We will use following Array methods in our example. unshift(): Inserts one or more elements at the start of the Array.
If you want to append a single value into an array, simply use the push method. It will add a new element at the end of the array. But if you intend to add ...30 answers · Top answer: Use the Array.prototype.push method to append values to the end of an array: // initialize ... 20 Jul 2021 — The elements to add to the front of the arr . Return value. The new length property of the object upon which the method was called. Description. 20 Jul 2021 — The concat() method is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array.
1 week ago - Javascript array push() an built-in method that adds a new item to the end of an array and returns the new length of an array. Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array. #2 unshift - Insert an element at the beginning of the array. #3 spread operator - Adding elements to an array using the new ES6 spread operator. #4 concat - This can be used to append an array to ... Nov 11, 2019 - Insert an element in specific index in JavaScript Array. Learn how to insert an element in specific index in array. ... We have some in-built methods to add at elements at the beginning and end of the array.
In JavaScript, by using push () method we can add an element/elemets to the end of an array. EXAMPLE: Adding element to the end of an array. In the above code snippet we have given Id as " myId "to the second <p> element in the HTML code. There is a function myFunction () in the <script> block which is connected to the onclick of the HTML ... Introduction to Dynamic Array in JavaScript. Dynamic Array in JavaScript means either increasing or decreasing the size of the array automatically. JavaScript is not typed dependent so there is no static array. JavaScript directly allows array as dynamic only. We can perform adding, removing elements based on index values. The push method appends values to an array.. push is intentionally generic. This method can be used with call() or apply() on objects resembling arrays. The push method relies on a length property to determine where to start inserting the given values. If the length property cannot be converted into a number, the index used is 0. This includes the possibility of length being nonexistent, in ...
Javascript add array to array. To add an array to an array in JavaScript, use the array.concat() or array.push() method. The array concat() is a built-in method that concatenates two or more arrays. The concat() function does not change the existing arrays but returns a new array containing the values of the joined arrays. Arrays are carefully tuned inside JavaScript engines to work with contiguous ordered data, please use them this way. ... The similar thing happens with unshift: to add an element to the beginning of the array, we need first to move existing elements to the right, increasing their indexes. In Example # 2, we create an array literal, but it is empty. (var arr = []; works just fine, but it is an empty array.) When we check the length property and try to inspect the object with console.dir(arr), we can clearly see that it is empty. Then we add elements to the array, and add named properties (e.g. arr["drink"] = "beer").
20 Jul 2021 — The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. Say you want to add an item to an array, but you don't want to append an item at the end of the array. You want to explicitly add it at a particular place of the array. That place is called the index. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. Turning a 2D array into a sparse array of arrays in JavaScript; How to add new value to an existing array in JavaScript? How to compare two arrays in JavaScript and make a new one of true and false? JavaScript; Can we convert two arrays into one JavaScript object? Add two consecutive elements from the original array and display the result in a ...
In the above program, the splice () method is used to add an object to an array. The splice () method adds and/or removes an item. The first argument represents the index where you want to insert an item. The second argument represents the number of items to be removed (here, 0). The third argument represents the element that you want to add to ... The concat() method concatenates (joins) two or more arrays. The concat() method does not change the existing arrays, but returns a new array, containing the ... There are various ways to add or append an item to an array. We will make use of push, unshift, splice, concat, spread and index to add items to an array. Let's discuss all the 6 different methods one by one in brief. The push() method. This method is used to add elements to the end of an array. This method returns the new array length.
How to use JavaScript push, concat, unshift, and splice methods to add elements to the end, beginning, and middle of an array. Dec 11, 2020 - An array is a linear data structure and arguably one of the most popular data structures used in Computer Science. Modifying an array is a commonly encountered operation. Here, we will discuss how to add an element in any position of an array in JavaScript. An element can be added to an array ... push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ...
1 week ago - Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the ... Output. In the above program, the splice () method is used to add a new element to an array. The first argument is the index of an array where you want to add an element. The second argument is the number of elements that you want to remove from the index element. The third argument is the element that you want to add to the array. May 25, 2018 - Find out the ways JavaScript offers you to append an item to an array, and the canonical way you should use ... Notice that concat() does not actually add an item to the array, but creates a new array, which you can assign to another variable, or reassign to the original array (declaring it ...
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: Then you don't want to mutate it. If it is not needed, you can mutate it directly, which is usually faster than creating a copy. Said there are data structures where creating a copied of the array is as cheap as mutate it (or comparable cheap) and those are very cool but not so widespread in the JavaScript ... There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end. ARRAYA.concat (ARRAYB) will join two arrays together.
Adding and Deleting Array Elements We’ve already seen the simplest way to add elements to an array: just assign values to new indexes: a = [] // Start with an … - Selection from JavaScript: The Definitive Guide, 6th Edition [Book] Tip: To add items at the beginning of an array, use unshift(). ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS Tutorial JavaScript ... Feb 11, 2021 - You can add new items to an existing array with ease. This tutorial shows you three ways of appending new items to the end of an existing array. ... JavaScript comes with the Array#push method allowing you to push one or more items to the end of the array. You may also append new items by creating ...
A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the arrayLength parameter below). To add items and objects to an array, you can use the push () function in JavaScript. The push () function adds an item or object at the end of an array. For example, let's create an array with three values and add an item at the end of the array using the push () function. See the code below. Adding an element at a given position of the array. Sometimes you need to add an element to a given position in an array. JavaScript doesn't support it out of the box. So we need to create a function to be able to do that. We can add it to the Array prototype so that we can use it directly on the object.
How To Use Javascript Array Sort Method
5 Way To Append Item To Array In Javascript Laptrinhx
Javascript How To Add X To An Array X Times Stack Overflow
Javascript Lesson 25 Difference Between Arrays And Objects
3 Ways To Add Dynamic Key To Object In Javascript Codez Up
How To Add Elements Of An Array That Is A Return Of Another
Beginner Javascript Tutorial 33 Add Array Elements Using A Loop
Pivot A Javascript Array Convert A Column To A Row Techbrij
5 Way To Append Item To Array In Javascript Samanthaming Com
Adding Elements To Array In Javascript Tech Funda
Javascript Add New Elements At The Beginning Of An Array
Using Powershell To Split A String Into An Array
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Push Vs Unshift Methods Explained With 4
How To Add Object In Array Using Javascript Javatpoint
Javascript Array Splice Delete Insert And Replace
How To Check If Array Includes A Value In Javascript
Javascript Array Push How To Add Element In Array
How To Add An Object To An Array In Javascript Geeksforgeeks
25 Javascript Tutorial Add Two Arrays Into One Array بالعربي
4 Ways To Convert String To Character Array In Javascript
How To Add Item To An Array At A Specific Index In Javascript
Tools Qa Array In Javascript And Common Operations On
How To Append Element To Array In Javascript Tecadmin
Javascript Array Distinct Ever Wanted To Get Distinct
How To Insert An Item Into Array At Specific Index In
Javascript Array Insert How To Add To An Array With The
Add Or Remove Array Value With Javascript And Jquery
How To Add An Object To An Array In Javascript Geeksforgeeks
Chapter Preview Arrays Tech Career Booster
Javascript Tutorial Adding Elements To Array In Javascript
13 Things You Can Do With Javascript Arrays
0 Response to "35 Javascript Add To Array"
Post a Comment