26 How To Get Array Object Value In Javascript
Summary: in this tutorial, you will learn how to convert an object to an array using Object's methods.. To convert an object to an array you use one of three methods: Object.keys(), Object.values(), and Object.entries().. Note that the Object.keys() method has been available since ECMAScript 2015 or ES6, and the Object.values() and Object.entries() have been available since ECMAScript 2017. We can also use destructuring with for-of loops like below example. This approach helps us in getting the current array index as well as distinct value for the same index. 1let numbers = [ 'zero', 'ten', 'hundred', 'thousand']; 2for (const [index, element] of numbers.entries()) {. 3 console.log(index, element); 4}
Find Object By Id In An Array Of Javascript Objects
In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf () method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease.
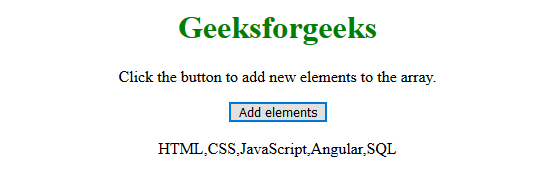
How to get array object value in javascript. Use Object.entries (obj) to get an array of key/value pairs from obj. Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries (array) on the resulting array to turn it back into an object. For example, we have an object with prices, and would like to double them: Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: JavaScript Array of Objects Tutorial - How to Create, Update, and Loop Through Objects Using JS Array Methods ... Creating an array of objects. But let's get back to cars. Let's take a look at this set of cars: ... Find an object in an array by its values - Array.find. Let's say we want to find a car that is red.
I want to extract a field from each object, and get an array containing the values, for example field foowould give array [ 1, 3, 5 ]. I can do this with this trivial approach: function getFields(input, field) { var output = []; Oct 12, 2017 - Function map is a good choice when dealing with object arrays. Although there have been a number of good answers posted already, the example of using map with combination with filter might be helpful. In case you want to exclude the properties which values are undefined or exclude just a specific ... The Object.entries() and Object.values() methods were introduced to JavaScript Object constructor with the release of ECMAScript 2017 (ES8). Let us have a quick look at these useful methods. Object.entries() Method. The Object.entries() method takes an object as argument and returns an array with arrays of key-value pairs:
Object.values() The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well). Inside this function, we can check if the name matches Roy Daniel and return the boolean value true if it matches and the boolean value false if not. the findIndex () method returns the index of the object in the array. It can be done like this, // Array of objects const objsArr = [ { name: "John Doe", age: 23 }, { name: "Roy Daniel", age: 25 ...
Search should return the object that match the search should return undefined becuase non of the objects in the array have that value should return undefined becuase our array of objects dont have ids 3 passing (12ms) Old answer - removed due to bad practices ... Get JavaScript object from array of objects by value of property. 0. 5/6/2021 · The Array.prototype.filter () method returns a new array with all elements that satisfy the condition in the provided callback function. Therefore, you can use this method to filter an array of objects by a specific property's value, for example, in the following way: // ES5+ const employees = [ { name: 'John', department: 'sales' }, { name: ... The task is to convert an Object {} to an Array [] of key-value pairs using JavaScript. Introduction: Objects, in JavaScript, is it's most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript's primitive data-types(Number, String, Boolean, null, undefined and symbol).
reason: When next().done=true or currentIndex>length the for..of loop ends. See Iteration protocols.. Value: there are no values stored in the array Iterator object; instead it stores the address of the array used in its creation and so depends on the values stored in that array. Two array methods to check for a value in an array of objects. 1. Array.some () The some () method takes a callback function, which gets executed once for every element in the array until it does not return a true value. The some () method returns true if the user is present in the array else it returns false. Jul 27, 2021 - Object.values(obj) – returns an array of values.
Oct 16, 2018 - Write cleaner and more readable code by making use of modern JavaScript array and object methods. Never touch a for/while loop again! but I just got bunch of peaks array with value (instead of all intervalId, time, value) and not the max value. ... Find object by id in an array of JavaScript objects. 1917. Copy array by value. 5111. For-each over an array in JavaScript. Hot Network Questions Has Peter Thiel said "Your mind is software. Program it. Second Method - javaScript sum array values using reduce () method. Next, we will use the reduce method of javascript for the sum of array values in javascript. You can see the example the below: 1. 2. 3. var numArr = [10, 20, 30, 40] // sums to value = 100. var sum = numArr.reduce (function(a, b) {return a+b;})
The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. Javascript Object.values () is a built-in function that returns the array of the given Object's enumerable property values. The Object values () takes an object as an argument of which enumerable own property values are to be returned and returns the array containing all the enumerable property values of the given Object. Array of states for each friend. Getting The Unique List of States. The next step is to use a JavaScript Set.. JavaScript Set. The Set object lets you store unique values of any type, whether primitive values or object references.. The key word to make note of in the description is unique.. The syntax to create a new Set is,
Nov 19, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Mar 10, 2021 - In the above code, we have used the Javascript indexOf method. So, this is how to search value in an array in javascript. Find the object in the JavaScript array. Object.values () takes the object as an argument of which the enumerable own property values are to be returned and returns an array containing all the enumerable property values of the given object.
The destructuring assignment is a cool feature that came along with ES6. Destructuring is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. That is, we can extract data from arrays and objects and assign them to variables. Why is In JavaScript, getting the keys and values that comprise an object is very easy. You can retrieve each object’s keys, values, or both combined into an array. The examples below use the following object: const obj = { name: 'Daniel', age: 40, occupation: 'Engineer', level: 4 }; Getting an object’s keys. The Object.keys() method returns an array of strings containing all of the object’s keys, sorted by … 12/9/2020 · To get only specific values in an array of objects in JavaScript, use the concept of filter(). Example
Different methods to obtain key-value pairs. Now that we know how to get keys & values in an array, let us look at the different methods to obtain key-value pairs. Let us first store the previously extracted keys & values in separate arrays. var keys = [ "one", "two", "three" ]; var values = [ 1, 2, 3 ]; You can use the Object.getOwnPropertySymbols to get the symbols of an object to enumerate over. The new Reflect API from ECMAScript 2015 provides Reflect.ownKeys returning a list of property names and symbols. Object.values¶ This just adds a method to object. Using fat-arrow functions can be a one-liner: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
@Gothdo is right. To get object we can use Array.find: var result = jsObjects.find(function( obj ) { return obj.b === 6; }); – kolodi Apr 27 '16 at 9:49 | Feb 20, 2021 - The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ...
First, that's not an array. Second, what you're calling the "index" is normally called the property name or key. There's no way to get the value except by the property. Though any expression can be placed inside the []and its return value will be used as the property name.- user2437417Jul 14 '13 at 1:58 Sort an Array of Objects in JavaScript. Summary: in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. Most values in JavaScript have properties, the exceptions being null and undefined. Properties are accessed using value.prop or value["prop"]. Objects tend to use names for their properties and store more or less a fixed set of them. Arrays, on the other hand, usually contain varying amounts ...
Let's see what utility functions provide JavaScript to extract the keys, values, and entries from an object. 1. Object.keys () returns keys. Object.keys (object) is a utility function that returns the list of keys of object. Let's use Object.keys () to get the keys of hero object: Object.keys (hero) returns the list ['name', 'city'], which ... Finally with ES2017, it's official now! We have 3 variations to convert an Object to an Array in JavaScript The structure of this array is fixed and I know for a fact that I'll always have key and value as the fields in each object in the array. When I try to validate the form submitted (additional server side validation), I'd like to cross-reference the value provided for a field against all the values for "key" in the array (blah, foo, bar, baz).
Definition and Usage. The find() method returns the value of the array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values)
How To Locate A Particular Object In A Javascript Array
Javascript Array Contains A Step By Step Guide Career Karma
Js Array From An Array Like Object Dzone Web Dev
10 Rendering Lists Part 2 Iterating Over Objects Java
Javascript Pushing An Object In An Array Is What By
Javascript Lesson 25 Difference Between Arrays And Objects
Javascript Group An Array Of Objects By Key By Edison
Looping Javascript Arrays Using For Foreach Amp More
Hacks For Creating Javascript Arrays
How To Group An Array Of Objects In Javascript By Nikhil
Hacks For Creating Javascript Arrays
Javascript Map How To Use The Js Map Function Array Method
Data Structures Objects And Arrays Eloquent Javascript
Javascript Find An Object In Array Based On Object S
How To Check If Variable Is An Array In Javascript By
Get Object From Array Based On Property Using Lodash Find
Convert Javascript Array To Object Of Same Keys Values
Javascript Array Of Objects Tutorial How To Create Update
How To Get The Value Of An Array Of Objects Present Inside An
Indexed Collections Javascript Mdn
How To Add An Object To An Array In Javascript Geeksforgeeks
Converting Object To An Array Samanthaming Com
Find A Value In An Array Of Objects In Javascript Stack
Javascript Array Methods How To Use Map And Reduce
Javascript Find Array Object By Property Value
0 Response to "26 How To Get Array Object Value In Javascript"
Post a Comment