20 Add Element To Array Javascript Es6
The splice () method adds and/or removes array elements. splice () overwrites the original array. This would mean that I have to concat the buffer to the array and shift everything. However, concatenation is slow so I was wondering if there is a fast way of doing this? Example: var array = [1,2,3,4,5,6]; var stream = [7,8,9]; array = magicalFunction(array,stream); // outputs [4,5,6,7,8,9] The array function is used for plotting with ChartJS.
Tools Qa Array In Javascript And Common Operations On
push() method appends the given element(s) in the last of the array and returns the length of the new array. Syntax array.push(element1, ..., elementN); Parameter Details. element1, ..., elementN: The elements to add to the end of the array. Return Value. Returns the length of the new array. Example
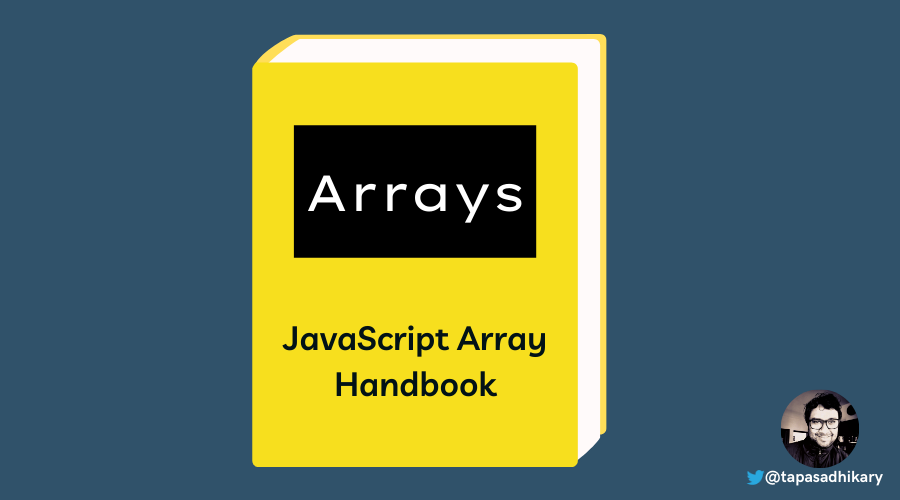
Add element to array javascript es6. This will return a new array with only unique elements like we wanted. Using Array.filter The next method we will be using is using ES6 Array.filter function. The Array.filter function takes a callback whose first parameter is the current element in the operation. It also takes an optional index parameter and the array itself. Combining these, we can use Array.filter to ensure only unique ... One of the most frequently used javascript methods has to be push. It does a simple thing. Push -> Adds an element to the end of the array. Couldn't be any simpler right. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
JavaScript ES6 Arrays. Arrays are used to store collections of values in a sequential order. You can assign arrays to variables just like you can assign strings or any other data type. Arrays are useful for storing collections of elements where order is important. Arrays start counting from 0. Method 1: push () method of Array The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. Learn how to add an element to an array if it doesn't exist JavaScript/Vue.js/ES6. In this example, there's an array of objects. items: [ { id: 1, text: "Item 1" }, { id: 2, text: "Item 2" }, { id: 3, text: "Item 3" } ] Our task is to add a new item "Item 4" with an id 4 to the array of items. Vue.js
The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array #2 unshift - Insert an element at the beginning of the array #3 spread operator - Adding elements to an array using the new ES6 spread operator join() method joins all the elements of an array into a string. Syntax array.join(separator); Parameters. separator − Specifies a string to separate each element of the array. If omitted, the array elements are separated with a comma. Return Value. Returns a string after joining all the array elements. Example
Spreading elements together with an individual element. Beside simply spreading elements into an empty array, you can also spread elements together with other standalone elements. One example could be to create an array where you first add a simple name, then spread the elements from names at the end. Output: The main array : [10, 20, 30, 40, 50] The modified array: [40, 50] Note: The filter() method is widely compatible in IE because it belongs to ES5 and find() is introduced in ES6 so only the latest browsers (IE 11) are compatible with it. Find() Method: This method can be used when you want to find out the element which passes a certain condition. ES6 − Array Methods. Following are some new array methods introduced in ES6. Array.prototype.find. find lets you iterate through an array and get the first element back that causes the given callback function to return true. Once an element has been found, the function immediately returns.
Adding an element at a given position of the array. Sometimes you need to add an element to a given position in an array. JavaScript doesn't support it out of the box. So we need to create a function to be able to do that. We can add it to the Array prototype so that we can use it directly on the object. Insert One or Multiple Elements at a Specific Index Using Array.splice () The JavaScript Array.splice () method is used to change the contents of an array by removing existing elements and optionally adding new elements. This method accepts three parameters. JavaScript: Add an element to the end of an array. This is a short tutorial on how to add an element to the end of a JavaScript array. In the example below, we will create a JavaScript array and then append new elements onto the end of it by using the Array.prototype.push() method.
Method of adding array in ES6. Time:2021-3-8. ES6 new array operation method. ... Pass in a callback function, find the first element in the array that meets the search rule, return it and terminate the search. ... Several methods of judging array in JavaScript; push () ¶ The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. Returns the last (greatest) index of an element within the array equal to an element, or -1 if none is found. Array.prototype.map() Returns a new array containing the results of calling a function on every element in this array. Array.prototype.pop() Removes the last element from an array and returns that element. Array.prototype.push()
To double every element of this array a for loop is used which runs from zero (because the index of an array starts from zero) to one less than the length of the array. Now on the third line, each element is extracted from the array and is multiplied by 2, hence doubling the values. DOM Element. accessKey ... Previous JavaScript Array Reference Next ... Definition and Usage. The push() method adds new items to the end of an array. push() changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift(). Browser Support. 5 Way to Append Item to Array in JavaScript. Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍.
Spread syntax can be used when all elements from an object or array need to be included in a list of some kind. In the above example, the defined function takes x, y, and z as arguments and returns the sum of these values. An array value is also defined. When we invoke the function, we pass it all the values in the array using the spread syntax ... See the Pen JavaScript: Add items in a blank array and display the items - array-ex-13 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: Write a JavaScript program to compute the sum and product of an array of integers. The spread operator ..., introduced first in ES6 became one of the most popular and favourite feature among the developers.. A separate RFC was made for this much widely accepted feature to extend its functionalities to objects, prior it only worked on arrays. This tutorial describes how to add element to the ending of an array using spread operator in ES6
I think the term "splice" makes sense. Splice means to join or connect, also to change. You have an established array that you are now "changing" which would involve adding or removing elements. You specify where in the array to start, then how many old items to remove (if any) and lastly, optionally a list of new elements to add. Use unshift. It's like push, except it adds elements to the beginning of the array instead of the end. unshift / push - add an element to the beginning/end of an array shift / pop - remove and return the first/last element of an array The push method appends values to an array.. push is intentionally generic. This method can be used with call() or apply() on objects resembling arrays. The push method relies on a length property to determine where to start inserting the given values. If the length property cannot be converted into a number, the index used is 0. This includes the possibility of length being nonexistent, in ...
Es6 Remove Item From Array Code Example
Javascript Array Distinct Ever Wanted To Get Distinct
Everything You Need To Know About Javascript Array Methods
Vanillacartjs Es6 Javascript Shopping Cart
The Javascript Array Handbook Js Array Methods Explained
Javascript Array Splice Delete Insert And Replace
Remove Duplicates In A Javascript Array Using Es6 Set And
Js Array From An Array Like Object Dzone Web Dev
Get The First Element Of An Array W3resource
Use Es6 Sets To Improve Javascript Performance
Javascript Es6 The Spread Syntax By Brandon Morelli
Find The Min Max Element Of An Array In Javascript Stack
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Javascript Array Methods Declaration Properties Cronj
Append List Of Elements Through Foreach Javascript Code Example
Es6 Destructuring An Elegant Way Of Extracting Data From
0 Response to "20 Add Element To Array Javascript Es6"
Post a Comment