25 Websocket Javascript Client Example
What is Websocket. According to MDN, The WebSocket API is an advanced technology that makes it possible to open a two-way interactive communication session between the user's browser and a server. With this API, you can send messages to a server and receive event-driven responses without having to poll the server for a reply, Simply WebSocket ... The code from the video, and also this article is available in my Node.js WebSocket Examples GitHub Repository.. First we'll go over some plain socket code, followed by WebSocket code. If you already serve assets with something like Express.js, Hapi, or the native Node.js HTTP library, we can jump into the socket code.. Socket Server JavaScript Code
Announcing Websocket Apis In Amazon Api Gateway Aws Compute
13/11/2018 · Using websockets directly might be troublesome, it's advised you use a framework to abstract this layer, so they can easily fallback to other methods when not supported in the client. For example, this is a direct implementation using Express js and Websockets directly. This example also allows you to use the same server for HTTP calls.
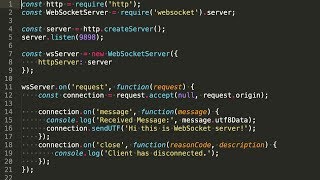
Websocket javascript client example. In terms of the problem I was originally trying to solve, I quickly realized that once I had a websocket server running, a simple HTML+JS client might be superior to a dedicated console-based websocket client app. JS has done a good job at making websockets ridiculously easy to use, and since the initial connection of a websocket is HTTP-based ... Web Browsers can use the http and websockets protocols but not MQTT. To be able to publish and subscribe to an MQTT broker with a browser you will need to u... WebSocket is a communications protocol that provides a full-duplex communication channels over a single TCP connection established between a web browser (client) and a web server (this take place ...
Apr 14, 2021 - This is a (mostly) pure JavaScript implementation of the WebSocket protocol versions 8 and 13 for Node. There are some example client and server applications that implement various interoperability testing protocols in the "test/scripts" folder. Because Node networking is event-driven, clients "run in a loop" by default—so to make it a use-once client, the client would have to explicitly call client.destroy() or submit data with client.end(), or we can let the server do the close. (Note how this differs from the Java and Python servers we saw earlier, where use-once was the ... In most cases you can refer to the first example to get the boilerplate code. Establishing the Connection. In order for a connection to be established between the client and server, the server must do two things: Hook in to the HTTP server to handle websocket connections; Serve up the socket.io.js client library as a static resource
Using WebSocket to build an interactive web application. This guide walks you through the process of creating a "Hello, world" application that sends messages back and forth between a browser and a server. WebSocket is a thin, lightweight layer above TCP. This makes it suitable for using "subprotocols" to embed messages. Tutorial programming for Node.js peer-to-peer, client-to-server, and server-to-client WebSocket data streaming.Learn how to open socket connections with Node... 3 days ago - Also see the provided example using express-session. ... A client WebSocket broadcasting to all connected WebSocket clients, including itself.
Apr 28, 2018 - WebSockets are an alternative to HTTP communication in Web Applications. They offer a long lived, bidirectional communication channel between client and server. Learn how to use them to perform network interactions This simple example creates a new WebSocket, connecting to the server at wss://www.example /socketserver. A custom protocol of "protocolOne" is named in the request for the socket in this example, though this can be omitted. var exampleSocket = new WebSocket("wss://www.example /socketserver", "protocolOne"); Sep 03, 2019 - It’s actually possible to transfer binary data, such as video and audio, but it may not be practical, as Javascript at client side has to encode them. ... Many features of HTML5 (Canvas, geo location, offline storage, etc) are already implemented in many of existing browsers, but WebSocket is ...
ws: a Node.js WebSocket library. ws is a simple to use, blazing fast, and thoroughly tested WebSocket client and server implementation. Passes the quite extensive Autobahn test suite: server, client. Note: This module does not work in the browser.The client in the docs is a reference to a back end with the role of a client in the WebSocket communication. The WebSocket protocol, described in the specification RFC 6455 provides a way to exchange data between browser and server via a persistent connection.. Once a websocket connection is established, both client and server may send the data to each other. WebSocket is especially great for services that require continuous data exchange, e.g. online games, real-time trading systems and so on. Leveraging Express.js and the ws NPM module, it is possible to create a Node Websocket Server in less than ten lines of code.. The Websocket protocol provides full-duplex communication channels over a single TCP connection. In the past, web clients had to employ long-polling or the repeated pinging of a server in order to achieve this kind of "push" functionality.
The WebSocket protocol, described in the specification RFC 6455 provides a way to exchange data between browser and server via a persistent connection. The data can be passed in both directions as "packets", without breaking the connection and additional HTTP-requests. WebSocket is especially great for services that require continuous data exchange, e.g. online games, real-time trading ... _client.websocket.client.on('connectFailed', function (error) { if (_client.connection.state == states.connection.retryingConnection 25/1/2015 · Simple Javascript Websocket Client. A very simple html / javascript WebSocket client, that can be used to test my Simple Websocket Server in Play!. This client illustrates how to connect to a websocket endpoint, send & receive data, close the connection. I wrote a presentation about WebSockets in Play!. Find it here on SlideShare.
Jun 10, 2021 - When we call it, we pass the two ... our websocket client instance once it's available. For the queryParams, here we're just passing some example data to showcase how this works. In the onMessage callback, we take in the message (remember, this will be a JavaScript object parsed ... 1 Answer1. To start with, both your code looks identical the Java and JavaScript one. Both work for what they are design to, but the facts is that you are trying to connect a WebSocket client to a socket server. As I know they are two different things regarding this answer. I have never tried it your way. The semantics are on top of the WebSockets and defines frames that are mapped onto WebSockets frames. Using STOMP gives us the flexibility to develop clients and servers in different programming languages. In this current example, we will use STOMP for messaging between client and server. 4. WebSocket Server
Dec 12, 2018 - Let’s see how opening a WebSocket connection looks like on the client side: WebSocket URLs use the ws scheme. There is also wss for secure WebSocket connections which is the equivalent of HTTPS. This scheme just starts the process of opening a WebSocket connection towards websocket.example . Sep 05, 2017 - But for server -> client it’s a bit more complex. We have to distinguish between 3 different types of message: ... Therefore every message is a simple JavaScript object encoded into JSON. ... Node.js itself doesn’t have support for WebSocket but there are already some plugins that implement ... _client.websocket.client.on('connectFailed', function (error) { if (_client.connection.state == states.connection.retryingConnection
A JavaScript MQTT client which works with Nodejs. See Using the Node.js MQTT Client-Starting Guide; An MQTT over Websockets JavaScript client which works in a web browser. Using the JavaScript Websocket Client. Note: If you prefer video then see Using the JavaScript MQTT client- Example 1. The first thing you will need to do is create a basic ... WebSocket Example. A WebSocket is a standard bidirectional TCP socket between the client and the server. The socket starts out as a HTTP connection and then "Upgrades" to a TCP socket after a HTTP handshake. After the handshake, either side can send data. Client Side HTML & JavaScript Code Below is a very simple example of how to create a basic websocket using node.js. Websockets are great for maintaining a server/client relationship without as much of the overhead of HTTP web traffic.
WebSockets - JavaScript Application. The following program code describes the working of a chat application using JavaScript and Web Socket protocol. The key features and the output of the chat application are discussed below −. To test, open the two windows with Web Socket support, type a message above and press return. This would enable the ... C# WebSockets Tutorial: Build a Multiplayer Game ... From a client perspective, all modern HTML5-compliant browsers support the WebSocket class. JavaScript like this creates a connection that send messages: var connection = new WebSocket ... Client Libraries for WebSocket communications. Below is the JavaScript source code of a file named wsclient.js which will be included in an HTML 5 page to enable it to open a WebSocket connection. The script contains the code to create a WebSocket client using the WebSocket interface See line 37 for how this class is used: . new WebSocket(webSocketURL)
Jan 15, 2020 - Seamless communication is a must on the modern web. As internet speeds increase, we expect our data in real time. To address this need, WebSocket, a popular communication protocol finalized in 2011, enables websites to send and receive data without delay. With WebSockets, you can build multiplayer ... 13/7/2020 · simple websocket example. Simple websocket example with javascript client and python Tornado server files. Websockets provide convenient server to client messaging. To work with websockets you will need a websocket enabled client/server pair. Check page below for client websocket support: http://caniuse /websockets This is a quick demonstration of Java WebSocket API with maven and embedded Jetty. The WebSocket specification allows bi-directional communication session between client and server. This is an HTML5 based solution for HTTP statelessness. Maven dependencies
The sample WebSocket server application is a virtual USD Exchange rate publishing server. A new exchange rate becomes available every 2 seconds and it is published to all registered clients. Aug 01, 2021 - Are you wondering why we publish all this free content that you always seem to stumble on when Googling stuff? Simple: trials! If you’re enjoying LogRocket’s blog, we bet you’ll love our product. LogRocket helps you reproduce bugs more quickly, understand frontend performance issues, ... The masking value in websocket-client is normally set using os.urandom in the websocket/_abnf.py file. However, this value can be customized as you wish. One use case, outlined in issue #473 , is to set the masking key to a null value to make it easier to decode the messages being sent and received.
Define a client-side WebSocket endpoint by using JavaScript; Operate on Plain Old Java Objects (POJOs), in real-time, with actions invoked from a web browser client; Time to Complete. Approximately 1 hour. Introduction. Modern web applications require more interactivity than ever before for client/server communications. 2 weeks ago - The WebSocket API is an advanced technology that makes it possible to open a two-way interactive communication session between the user's browser and a server. With this API, you can send messages to a server and receive event-driven responses without having to poll the server for a reply.
Github Dualcon Javascript Websocket Javascript Java Ee
Server And Client Side Example Of Websockets Using Node
How To Send Message To Specific Client Using Ws Node Js Code
Html5 Websocket Dzone Refcardz
Using The Javascript Mqtt Client With Websockets
Developing Websocket Clients Java Ee 8 Application Development
Create A Websocket Client In Javascript Pega Exchange
What Is Web Socket And How It Is Different From The Http
How To Build A Chat Application With Amazon Elasticache For
Websocket Basics An Introduction Article Treehouse Blog
Using Web Socket Aws Api Gateway To Allow Your Event Driven
Websocket Tutorial With Java Server Jetty And Javascript
Node Js Websocket Programming Examples Pubnub
Websockets Javascript Application
Github Pomelonode Pomelo Jsclient Websocket
Html5 Websocket Dzone Refcardz
Websocket Client Amp Server Unity Amp Nodejs By Ahmed Schrute
Node Js Websocket Programming Examples Pubnub
Node Js Websocket Programming Examples Pubnub
Writing A Chat Server Using Node Js Typescript And
Websockets In Node Js Mastering Js
Create A Websocket Client In Javascript Pega Exchange
0 Response to "25 Websocket Javascript Client Example"
Post a Comment