28 Email Validation Using Javascript
Email validation! Since my day job is in marketing, we build a lot of pages with forms, and the least we need is an email address. So how do we make sure the input is at least a valid email type in JavaScript? HTML Structure For today's work we'll use a very simple form, with only a email input and a button to submit. 27/9/2018 · The best option to validate an email address is by using a Regular Expression. There is no universal email check regex. Everyone seems to use a different one, and most of the regex you find online will fail the most basic email scenarios, due to inaccuracy or to the fact that they do not calculate the newer domains introduced, or internationalized email addresses
Javascript Email Validation Form Using Regular Expressions Part 1 Of 2
Different Methods to validate Email in JavaScript. There are so many ways you can validate email addresses in JavaScript. And you don't have to worry because am going to walk you through with an easy way of doing it. But mostly, below are the popular methods to perform email validation: Using the regex or regular expression (my preferred way)
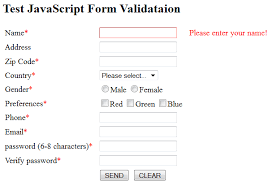
Email validation using javascript. Validating email and password - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have this dummy array that contains the login info of two of many users of a social networking platform −. const array = [ { email: 'usman@gmail ', password: '123' }, { email: 'ali@gmail ', password: '123 Through JavaScript, we can validate name, password, email, date, mobile numbers and more fields. Client-side validation prevents the client from knowing whether the form is okay before reloading a page. Whereas, Server-side validation is important due to the fact that client-side validation can be completely bypassed by turning off JavaScript. JavaScript is used to create beautifully designed web applications. Email validation in JavaScript is an important part of the user experience of a web application. Validation assists in inputting forms and makes sure that only valid information is passed to the server from the client-side of the application.
This is a very important step while validating an HTML form. In this post, we will discuss the process of email validation using JavaScript. Now we will look at the three ways to validate an email address. They are -. Using regular expression. Simple email validation by checking '@'and '. In the earlier blog, I've shared how to create Login & Registration Form using HTML & CSS and now it's time to validate the login form using JavaScript. Form Validation in HTML means to check that the user's entered credential - Email, Username, Password is valid and correct or not. Email validation using Regular Expressions The most common way to validate an email with JavaScript is to use a regular expression (RegEx). Regular expressions will help you to define rules to validate a string. If we summarize, an email is a string following this format:
Methods to validate Email in JavaScript. There are many ways to validate email address, the three most popular ways are: Using the regex expression. Using HTML in-built email validation. Using a third party library. As using regex expression is most popular, we will take a look at how we can validate an email address with a regex expression. Email Validation in JavaScript Email validation is one of the vital parts of authenticating an HTML form. Email is a subset or a string of ASCII characters, divided into two parts using @ symbol. Its first part consists of private information, and the second one contains the domain name in which an email gets registered. 20/9/2019 · Given an email id and the task is to validate the email id is valid or not. The validation of email is done with the help of Regular Expressions. Approach 1: RegExp – It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.) It is allowing every special symbol in the email-id (like, !, #, $, %, ^, &, *) symbols in the Email-Id but not allowing the second @ …
Javascript (JS) Email Validation without Regex. In this version of email validation we will be validating Email without using Regex In javascript, we can do this by using HTML5 Email attribute and by creating custom JavaScript, considering some points: Email Address string must contain "@" sign. "." (dot) must be last character in string to test. Enroll My Course : Next Level CSS Animation and Hover Effectshttps://www.udemy /course/css-hover-animation-effects-from-beginners-to-expert/?referralCode=... Example: Using Regex // program to validate an email address function validateEmail(email_id) { const regex_pattern = /^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/; if (regex_pattern.test(email_id)) { console.log('The email address is valid'); } else { console.log('The email address is not valid'); } } …
JavaScript email validation It is important to validate the form submitted by the user because it can have inappropriate values. So, validation is must to authenticate user. JavaScript provides facility to validate the form on the client-side so data processing will be faster than server-side validation. Email validation helps to check if a user submitted a valid email address or not. Using regular expression in JavaScript, you can easily validate the email address before submitting the form. We'll use the regular expression for validate an email address in JavaScript. 18/3/2020 · Introduction to Email Validation in JavaScript. Email Validation in JavaScript is defined as validating user credentials according to user requirements. Validation is a process to authenticate the user in any programming language. As with other programming languages. JavaScript provides the feature to validate the page elements on the client side.
29/7/2021 · Validating email is a very important point while validating an HTML form. In this page we have discussed how to validate an email using JavaScript : An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is [email protected] The length of the personal_info part may be up to 64 characters long and domain name … Phone Number validation. The validating phone number is an important point while validating an HTML form. In this page we have discussed how to validate a phone number (in different format) using JavaScript : At first, we validate a phone number of 10 digits with no comma, no spaces, no punctuation and there will be no + sign in front the number. Form Validation Using JavaScript Form validation is done to check the accuracy of the user's entered information before they could submit the form. What validation checks in the form? It's meant to check the following things:
Email validation using Javascript. Javascript is very useful in validating user data. It can be a name, number, email, credit card number, password etc., Validating data as soon as the user starts to enter it is the trend these days. It is an interactive process that saves a lot of time wasted on debugging. Java script can check the regular expression pattern for valid email address. We have different regular expression patterns for validating email address. In this article, I am sharing some useful cross browsers regular expression patterns for validating email address with java script and jquery. Simple regular expression to validate email address To get a valid email id, the best way is using regex: Watch a video course JavaScript - The Complete Guide (Beginner + Advanced)
A good practice is to validate your data on the client, but double-check the validation on the server. With this in mind, you can simply check whether a string looks like a valid email address on the client and perform the strict check on the server. Here's the JavaScript function I use to check if a string looks like a valid mail address: <input type='text' id='txtEmail'/> <input type='submit' name='submit' onclick='Javascript:checkEmail ();'/> Now when the button is clicked then the JavaScript function SubmitFunction () will be called. Now write the bellow code in this function. JavaScript Form Validation HTML form validation can be done by JavaScript. If a form field (fname) is empty, this function alerts a message, and returns false, to prevent the form from being submitted:
Validate Email with Javascript Email validation using the Regex Expression. The easiest way to check if an email address is valid is to use regular expressions. There are no universal regular expressions for verifying an email address. The majority of regular expressions found online are not robust even in the most basic cases. 🔥 Full Stack Web Development Training: https://www.edureka.co/masters-program/full-stack-developer-trainingThis Edureka video on Email Validation in JavaSc... You can add your comment about this article using the form below. Make sure you provide a valid email address else you won't be notified when the author replies to your comment
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Validation In Javascript For Registration Form Html Example
Javascript Custom Email Validation For Company Email Only
Master Javascript Form Validation By Building A Form From Scratch
Custom Html5 Form Validator In Vanilla Javascript Just
Javascript Form Validation Script More Features Javascript
Javascript Form Validation The Email Function
Email Validation Using Javascript Tutorial Teachucomp Inc
Javascript Html Form Email Validation By Hamdi Fersi
How To Validate Email Address In Javascript Codespot
Concise Form Validator In Vanilla Javascript
The Javascript Ecosystem 3 Dynamic Input Validation Using
Email And Form Validation Using Javascript With Example
Javascript Email Validation Using Javascript With Or
How To Validate Email Address In Javascript With Regex
Javascript Email Validation Regex Code Example
Javascript Form Validation The Email Function
Email Validation Check In Html Css Amp Javascript Dev Community
Complete Validation Form In Javascript Form In Javascript
Javascript Email Validation With Css Ui Email Validate Js
Data Validation Using Javascript
Javascript Form Validation Mobile Number Email Html Chillyfacts
How To Creating A Custom Email Validation Attribute In Mvc
Email Validation In Html Css Amp Javascript
Node Js Form Validation With An Example Codingstatus
Form Validation Using Javascript
0 Response to "28 Email Validation Using Javascript"
Post a Comment