30 Javascript Remove Array Item
Required. The position to add/remove items. Negative values a the position from the end of the array. howmany: Optional. Number of items to be removed. item1, ..., itemX: Optional. New elements(s) to be added Remove the first element using array.splice () The splice () method is a sly way of removing, replacing, and/or adding items in the array. It works similarly to splice () methods in other languages. To remove the first item of an array, means we have to remove an item whose index is 0. Remember that arrays are zero-indexed in JavaScript.
The Fastest Way To Remove A Specific Item From An Array In
When you delete an array element, the array length is not affected. This holds even if you delete the last element of the array. When the delete operator removes an array element, that element is no longer in the array. In the following example, trees [3] is removed with delete. var trees = ['redwood', 'bay', 'cedar', 'oak', 'maple']; delete ...
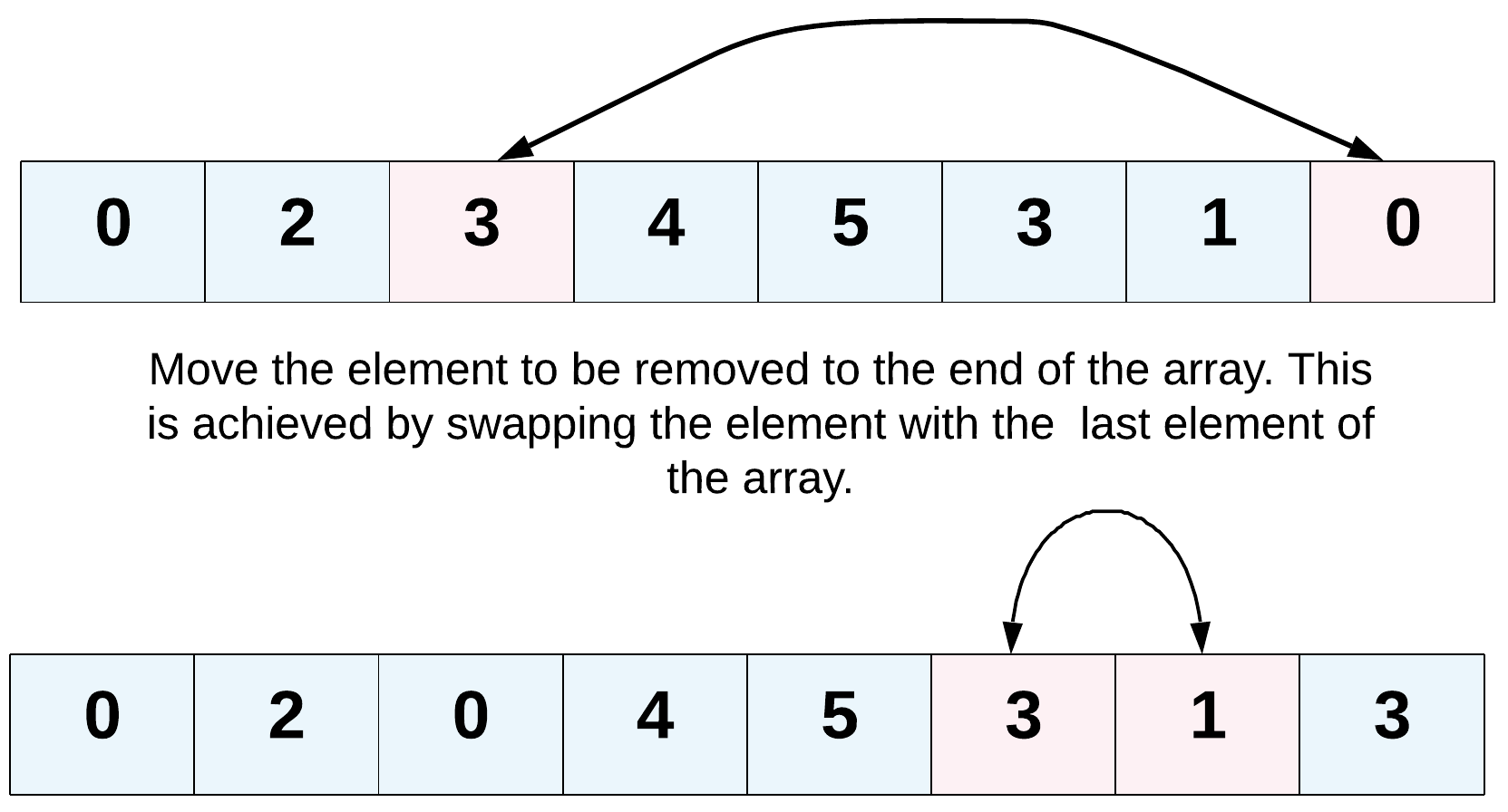
Javascript remove array item. Use the splice () Method to Remove an Object From an Array in JavaScript. The method splice () might be the best method out there that we can use to remove the object from an array. It changes the content of an array by removing or replacing existing elements or adding new elements in place. The syntax for the splice () method is shown below. 18/5/2019 · Returns a new Array, having the selected items. JavaScript Array pop() Method This method deletes the last element of an array and returns the element. Syntax: array.pop() Return value: It returns the removed array item. An array item can be a string, a number, an array, a boolean, or any other object types that are applicable in an array. JavaScript array splice() Javascript array splice() is an inbuilt method that changes the items of an array by removing or replacing the existing elements and/or adding new items. To remove the first object from the array or last object from the array, then use the splice() method.
The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: The pop() and shift() methods change the length of the array.. You can use unshift() method to add a new element to an array.. splice()¶ The Array.prototype.splice() method is used to change the contents of an array by removing or replacing the existing items and/or adding new ones in place. The first argument defines the location at which to begin adding or removing elements. Use the Underscore.js Library to Remove a Specific Element From JavaScript Array. Underscore.js is a very helpful library that provides us a lot of useful functions without extending any of the built-in objects. To remove target elements from a JavaScript array, we have to use the without() function. This function returns a copy of the array ...
How can I remove a specific item from an array? Related. 7626. How do JavaScript closures work? 8164. How do I check if an element is hidden in jQuery? 6730. How do I remove a property from a JavaScript object? 4435. How do I check if an array includes a value in JavaScript? 3493. How to insert an item into an array at a specific index (JavaScript) Removing Array Items By Value Using Splice. If you know the value you want to remove from an array you can use the "splice method".. First, you must identify the index of the target item. filter (): In a given JavaScript array, when we need to remove one or more than one item based on some condition then we use arr.filter () method to perform the operation. For example, lets take a numbers array is numArray = [4,33,42,7,52,84,11,8,]. Now from this array if we want values of more than 10 then we will use filter () method.
This will remove all objects with an id of 0.. It also creates a copy of the array and does not modify the original array. This is why we need to reassign the output. If you'd like to modify the array in place, then you might have to resort to good ol' for loops. Summary: in this tutorial, you will learn how to remove duplicates from an array in JavaScript. 1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Array.from() Creates a new Array instance from an array-like or iterable object.. Array.isArray() Returns true if the argument is an array, or false otherwise.. Array.of() Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments.
One traditional way of removing an item from JavaScript array is by using the built-in splice method. And that's what we did here. Last argument is set to 1 to make sure we only delete 1 item. You can specify any number here, but in this case we only need to delete 1. Leaving second argument empty will remove all items after the index. 1/1/2014 · Using Splice to Remove Array Elements in JavaScript. The splice method can be used to add or remove elements from an array. The first argument specifies the location at which to begin adding or removing elements. The second argument specifies the number of elements to remove. possible duplicate of Can you remove one array from another in javascript or jquery - you cannot have paid a lot of attention to the suggestions made when you wrote the question - mplungjan Nov 13 '13 at 15:18. ... Proper way to remove all elements contained in another array is to make source array same object by remove only elements:
11/5/2021 · The splice() method modifies the contents of an array by removing existing elements and/or adding new elements. While the filter() method does not modify the original array. Example : First, find the index of the item you want to delete, then delete it with splice(). 10/9/2020 · The splice () method This method can both remove and add items at a specified index of array. The first parameter of splice () takes an array index where you want to add or remove item. The second parameter takes number of elements to be removed from the specified index. In the above program, an item is removed from an array using a for loop. Here, The for loop is used to loop through all the elements of an array. While iterating through the elements of the array, if the item to remove does not match with the array element, that element is pushed to newArray. The push() method adds the element to newArray.
To remove an item from an array by value, we can use the splice () function in JavaScript. The splice () function adds or removes an item from an array using the index. To remove an item from a given array by value, you need to get the index of that value by using the indexOf () function and then use the splice () function to remove the value ... To remove an element from a JavaScript array using the Array.prototype.splice () method, you need to do the following: Pass the number of elements you wish to remove as the second argument to the method. Please be aware that the Array.prototype.splice () method will change/mutate the contents of the original array by removing the item (s) from it. Whatever you do, don't use delete to remove an item from an array. JavaScript language specifies that arrays are sparse, i.e., they can have holes in them. Using delete creates these kinds of holes. It removes an item from the array, but it doesn't update the length property. This leaves the array in a funny state that is best avoided.
In JavaScript, the Array.splice () method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice () returns the removed elements (if any) as an array. (In this case, the origin -1, meaning -n is the index of the nth last element, and is therefore equivalent to the index of array.length - n.) If array.length + start is less than 0, it will begin from index 0. deleteCount Optional. An integer indicating the number of elements in the array to remove from start. 20/5/2020 · JavaScript provides many ways to remove elements from an array. You can remove an item: By its numeric index. By its value. From the beginning and end of the array. Removing an element by index. If you already know the array element index, just use the Array.splice() method to remove it from the array. This method modifies the original array by removing or replacing existing elements and returns the removed elements …
To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. To remove null or undefined values do the following: In this lesson, we have discussed how to remove a specific item from an array in JavaScript. There are several methods offered by JavaScript to remove the specified element from the given array. Here we have used the three methods, firstly we have used the splice() method. With the help of this method, we can remove or replace the specified ... Here are a few ways to remove an item from an array using JavaScript. All the method described do not mutate the original array, and instead create a new one. If you know the index of an item. Suppose you have an array, and you want to remove an item in position i. One method is to use slice():
The array.splice () method is used to add or remove items from an array. This method takes in 3 parameters, the index where the element's id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted. This method actually deletes the element at index and shifts the remaining elements leaving no ...
Remove First Last Element From Array Javascript Tuts Make
Remove Elements From A Javascript Array Geeksforgeeks
How To Remove Items From The Array In Javascript Reactgo
Proful Sadangi On Twitter Javascript Basics 3 Ways To
How To Remove A Specific Javascript Array Element Splice
Javascript Remove The Last Item From An Array Geeksforgeeks
3 Ways To Use Array Slice In Javascript By Javascript Jeep
Javascript Array A Complete Guide For Beginners Dataflair
Java67 How To Remove A Number From An Integer Array In Java
Javascript Remove Object From Array If Value Exists In Other
Remove Element From Array Javascript First Last Value
How To Remove An Item From An Array In Javascript
Hacks For Creating Javascript Arrays
Javascript Remove Array Index Depending On Row Index
How Can I Remove Elements From Javascript Arrays O Reilly
Javascript Tutorial Removing A Specific Element From An Array
Typescript Remove Item From Array Learn The Examples And
How To Remove Array Duplicates In Es6 By Samantha Ming
C Program To Delete Duplicate Elements From An Array
How To Remove An Element From A Javascript Array By Eniola
Javascript How Can I Remove A Specific Item From An Array
Program To Delete An Element From Array In C C Programs
Javascript Array Remove An Element Javascript Remove Element
Remove An Array Element Without Mutation Jake Trent
How To Remove An Item From Array In Javascript Programmerhumor
9 Ways To Remove Elements From A Javascript Array
Add And Remove Array Items With Javascript Concat And Slice
0 Response to "30 Javascript Remove Array Item"
Post a Comment